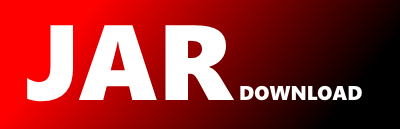
com.pulumi.azure.redhatopenshift.kotlin.inputs.ClusterWorkerProfileArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.redhatopenshift.kotlin.inputs
import com.pulumi.azure.redhatopenshift.inputs.ClusterWorkerProfileArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property diskEncryptionSetId The resource ID of an associated disk encryption set. Changing this forces a new resource to be created.
* @property diskSizeGb The internal OS disk size of the worker Virtual Machines in GB. Changing this forces a new resource to be created.
* @property encryptionAtHostEnabled Whether worker virtual machines are encrypted at host. Defaults to `false`. Changing this forces a new resource to be created.
* > **NOTE:** `encryption_at_host_enabled` is only available for certain VM sizes and the `EncryptionAtHost` feature must be enabled for your subscription. Please see the [Azure documentation](https://learn.microsoft.com/azure/virtual-machines/disks-enable-host-based-encryption-portal?tabs=azure-powershell) for more information.
* @property nodeCount The initial number of worker nodes which should exist in the cluster. Changing this forces a new resource to be created.
* @property subnetId The ID of the subnet where worker nodes will be hosted. Changing this forces a new resource to be created.
* @property vmSize The size of the Virtual Machines for the worker nodes. Changing this forces a new resource to be created.
*/
public data class ClusterWorkerProfileArgs(
public val diskEncryptionSetId: Output? = null,
public val diskSizeGb: Output,
public val encryptionAtHostEnabled: Output? = null,
public val nodeCount: Output,
public val subnetId: Output,
public val vmSize: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.redhatopenshift.inputs.ClusterWorkerProfileArgs =
com.pulumi.azure.redhatopenshift.inputs.ClusterWorkerProfileArgs.builder()
.diskEncryptionSetId(diskEncryptionSetId?.applyValue({ args0 -> args0 }))
.diskSizeGb(diskSizeGb.applyValue({ args0 -> args0 }))
.encryptionAtHostEnabled(encryptionAtHostEnabled?.applyValue({ args0 -> args0 }))
.nodeCount(nodeCount.applyValue({ args0 -> args0 }))
.subnetId(subnetId.applyValue({ args0 -> args0 }))
.vmSize(vmSize.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterWorkerProfileArgs].
*/
@PulumiTagMarker
public class ClusterWorkerProfileArgsBuilder internal constructor() {
private var diskEncryptionSetId: Output? = null
private var diskSizeGb: Output? = null
private var encryptionAtHostEnabled: Output? = null
private var nodeCount: Output? = null
private var subnetId: Output? = null
private var vmSize: Output? = null
/**
* @param value The resource ID of an associated disk encryption set. Changing this forces a new resource to be created.
*/
@JvmName("hrpdqglqcesngguu")
public suspend fun diskEncryptionSetId(`value`: Output) {
this.diskEncryptionSetId = value
}
/**
* @param value The internal OS disk size of the worker Virtual Machines in GB. Changing this forces a new resource to be created.
*/
@JvmName("wckgewffmsqwitaj")
public suspend fun diskSizeGb(`value`: Output) {
this.diskSizeGb = value
}
/**
* @param value Whether worker virtual machines are encrypted at host. Defaults to `false`. Changing this forces a new resource to be created.
* > **NOTE:** `encryption_at_host_enabled` is only available for certain VM sizes and the `EncryptionAtHost` feature must be enabled for your subscription. Please see the [Azure documentation](https://learn.microsoft.com/azure/virtual-machines/disks-enable-host-based-encryption-portal?tabs=azure-powershell) for more information.
*/
@JvmName("ixdsrobqeabupvoy")
public suspend fun encryptionAtHostEnabled(`value`: Output) {
this.encryptionAtHostEnabled = value
}
/**
* @param value The initial number of worker nodes which should exist in the cluster. Changing this forces a new resource to be created.
*/
@JvmName("bocpabtxjtrvwhbx")
public suspend fun nodeCount(`value`: Output) {
this.nodeCount = value
}
/**
* @param value The ID of the subnet where worker nodes will be hosted. Changing this forces a new resource to be created.
*/
@JvmName("ihibyqrcrxyrjhbg")
public suspend fun subnetId(`value`: Output) {
this.subnetId = value
}
/**
* @param value The size of the Virtual Machines for the worker nodes. Changing this forces a new resource to be created.
*/
@JvmName("enqsqmnjxqjkjqdk")
public suspend fun vmSize(`value`: Output) {
this.vmSize = value
}
/**
* @param value The resource ID of an associated disk encryption set. Changing this forces a new resource to be created.
*/
@JvmName("wlqssvfaspounpbb")
public suspend fun diskEncryptionSetId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diskEncryptionSetId = mapped
}
/**
* @param value The internal OS disk size of the worker Virtual Machines in GB. Changing this forces a new resource to be created.
*/
@JvmName("twhemttnfyrarvgl")
public suspend fun diskSizeGb(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.diskSizeGb = mapped
}
/**
* @param value Whether worker virtual machines are encrypted at host. Defaults to `false`. Changing this forces a new resource to be created.
* > **NOTE:** `encryption_at_host_enabled` is only available for certain VM sizes and the `EncryptionAtHost` feature must be enabled for your subscription. Please see the [Azure documentation](https://learn.microsoft.com/azure/virtual-machines/disks-enable-host-based-encryption-portal?tabs=azure-powershell) for more information.
*/
@JvmName("ltcjhrvikwltrqjn")
public suspend fun encryptionAtHostEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionAtHostEnabled = mapped
}
/**
* @param value The initial number of worker nodes which should exist in the cluster. Changing this forces a new resource to be created.
*/
@JvmName("ygtnrswvsccvxxny")
public suspend fun nodeCount(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.nodeCount = mapped
}
/**
* @param value The ID of the subnet where worker nodes will be hosted. Changing this forces a new resource to be created.
*/
@JvmName("acqiiwgmchbysggr")
public suspend fun subnetId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.subnetId = mapped
}
/**
* @param value The size of the Virtual Machines for the worker nodes. Changing this forces a new resource to be created.
*/
@JvmName("jypyyygxxnoqrkrs")
public suspend fun vmSize(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.vmSize = mapped
}
internal fun build(): ClusterWorkerProfileArgs = ClusterWorkerProfileArgs(
diskEncryptionSetId = diskEncryptionSetId,
diskSizeGb = diskSizeGb ?: throw PulumiNullFieldException("diskSizeGb"),
encryptionAtHostEnabled = encryptionAtHostEnabled,
nodeCount = nodeCount ?: throw PulumiNullFieldException("nodeCount"),
subnetId = subnetId ?: throw PulumiNullFieldException("subnetId"),
vmSize = vmSize ?: throw PulumiNullFieldException("vmSize"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy