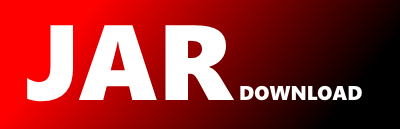
com.pulumi.azure.redis.kotlin.EnterpriseDatabase.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.redis.kotlin
import com.pulumi.azure.redis.kotlin.outputs.EnterpriseDatabaseModule
import com.pulumi.azure.redis.kotlin.outputs.EnterpriseDatabaseModule.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [EnterpriseDatabase].
*/
@PulumiTagMarker
public class EnterpriseDatabaseResourceBuilder internal constructor() {
public var name: String? = null
public var args: EnterpriseDatabaseArgs = EnterpriseDatabaseArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend EnterpriseDatabaseArgsBuilder.() -> Unit) {
val builder = EnterpriseDatabaseArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): EnterpriseDatabase {
val builtJavaResource = com.pulumi.azure.redis.EnterpriseDatabase(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return EnterpriseDatabase(builtJavaResource)
}
}
/**
* Manages a Redis Enterprise Database.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-redisenterprise",
* location: "West Europe",
* });
* const exampleEnterpriseCluster = new azure.redis.EnterpriseCluster("example", {
* name: "example-redisenterprise",
* resourceGroupName: example.name,
* location: example.location,
* skuName: "Enterprise_E20-4",
* });
* const example1 = new azure.redis.EnterpriseCluster("example1", {
* name: "example-redisenterprise1",
* resourceGroupName: example.name,
* location: example.location,
* skuName: "Enterprise_E20-4",
* });
* const exampleEnterpriseDatabase = new azure.redis.EnterpriseDatabase("example", {
* name: "default",
* resourceGroupName: example.name,
* clusterId: exampleEnterpriseCluster.id,
* clientProtocol: "Encrypted",
* clusteringPolicy: "EnterpriseCluster",
* evictionPolicy: "NoEviction",
* port: 10000,
* linkedDatabaseIds: [
* pulumi.interpolate`${exampleEnterpriseCluster.id}/databases/default`,
* pulumi.interpolate`${example1.id}/databases/default`,
* ],
* linkedDatabaseGroupNickname: "tftestGeoGroup",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-redisenterprise",
* location="West Europe")
* example_enterprise_cluster = azure.redis.EnterpriseCluster("example",
* name="example-redisenterprise",
* resource_group_name=example.name,
* location=example.location,
* sku_name="Enterprise_E20-4")
* example1 = azure.redis.EnterpriseCluster("example1",
* name="example-redisenterprise1",
* resource_group_name=example.name,
* location=example.location,
* sku_name="Enterprise_E20-4")
* example_enterprise_database = azure.redis.EnterpriseDatabase("example",
* name="default",
* resource_group_name=example.name,
* cluster_id=example_enterprise_cluster.id,
* client_protocol="Encrypted",
* clustering_policy="EnterpriseCluster",
* eviction_policy="NoEviction",
* port=10000,
* linked_database_ids=[
* example_enterprise_cluster.id.apply(lambda id: f"{id}/databases/default"),
* example1.id.apply(lambda id: f"{id}/databases/default"),
* ],
* linked_database_group_nickname="tftestGeoGroup")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-redisenterprise",
* Location = "West Europe",
* });
* var exampleEnterpriseCluster = new Azure.Redis.EnterpriseCluster("example", new()
* {
* Name = "example-redisenterprise",
* ResourceGroupName = example.Name,
* Location = example.Location,
* SkuName = "Enterprise_E20-4",
* });
* var example1 = new Azure.Redis.EnterpriseCluster("example1", new()
* {
* Name = "example-redisenterprise1",
* ResourceGroupName = example.Name,
* Location = example.Location,
* SkuName = "Enterprise_E20-4",
* });
* var exampleEnterpriseDatabase = new Azure.Redis.EnterpriseDatabase("example", new()
* {
* Name = "default",
* ResourceGroupName = example.Name,
* ClusterId = exampleEnterpriseCluster.Id,
* ClientProtocol = "Encrypted",
* ClusteringPolicy = "EnterpriseCluster",
* EvictionPolicy = "NoEviction",
* Port = 10000,
* LinkedDatabaseIds = new[]
* {
* exampleEnterpriseCluster.Id.Apply(id => $"{id}/databases/default"),
* example1.Id.Apply(id => $"{id}/databases/default"),
* },
* LinkedDatabaseGroupNickname = "tftestGeoGroup",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/redis"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-redisenterprise"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleEnterpriseCluster, err := redis.NewEnterpriseCluster(ctx, "example", &redis.EnterpriseClusterArgs{
* Name: pulumi.String("example-redisenterprise"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* SkuName: pulumi.String("Enterprise_E20-4"),
* })
* if err != nil {
* return err
* }
* example1, err := redis.NewEnterpriseCluster(ctx, "example1", &redis.EnterpriseClusterArgs{
* Name: pulumi.String("example-redisenterprise1"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* SkuName: pulumi.String("Enterprise_E20-4"),
* })
* if err != nil {
* return err
* }
* _, err = redis.NewEnterpriseDatabase(ctx, "example", &redis.EnterpriseDatabaseArgs{
* Name: pulumi.String("default"),
* ResourceGroupName: example.Name,
* ClusterId: exampleEnterpriseCluster.ID(),
* ClientProtocol: pulumi.String("Encrypted"),
* ClusteringPolicy: pulumi.String("EnterpriseCluster"),
* EvictionPolicy: pulumi.String("NoEviction"),
* Port: pulumi.Int(10000),
* LinkedDatabaseIds: pulumi.StringArray{
* exampleEnterpriseCluster.ID().ApplyT(func(id string) (string, error) {
* return fmt.Sprintf("%v/databases/default", id), nil
* }).(pulumi.StringOutput),
* example1.ID().ApplyT(func(id string) (string, error) {
* return fmt.Sprintf("%v/databases/default", id), nil
* }).(pulumi.StringOutput),
* },
* LinkedDatabaseGroupNickname: pulumi.String("tftestGeoGroup"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.redis.EnterpriseCluster;
* import com.pulumi.azure.redis.EnterpriseClusterArgs;
* import com.pulumi.azure.redis.EnterpriseDatabase;
* import com.pulumi.azure.redis.EnterpriseDatabaseArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-redisenterprise")
* .location("West Europe")
* .build());
* var exampleEnterpriseCluster = new EnterpriseCluster("exampleEnterpriseCluster", EnterpriseClusterArgs.builder()
* .name("example-redisenterprise")
* .resourceGroupName(example.name())
* .location(example.location())
* .skuName("Enterprise_E20-4")
* .build());
* var example1 = new EnterpriseCluster("example1", EnterpriseClusterArgs.builder()
* .name("example-redisenterprise1")
* .resourceGroupName(example.name())
* .location(example.location())
* .skuName("Enterprise_E20-4")
* .build());
* var exampleEnterpriseDatabase = new EnterpriseDatabase("exampleEnterpriseDatabase", EnterpriseDatabaseArgs.builder()
* .name("default")
* .resourceGroupName(example.name())
* .clusterId(exampleEnterpriseCluster.id())
* .clientProtocol("Encrypted")
* .clusteringPolicy("EnterpriseCluster")
* .evictionPolicy("NoEviction")
* .port(10000)
* .linkedDatabaseIds(
* exampleEnterpriseCluster.id().applyValue(id -> String.format("%s/databases/default", id)),
* example1.id().applyValue(id -> String.format("%s/databases/default", id)))
* .linkedDatabaseGroupNickname("tftestGeoGroup")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-redisenterprise
* location: West Europe
* exampleEnterpriseCluster:
* type: azure:redis:EnterpriseCluster
* name: example
* properties:
* name: example-redisenterprise
* resourceGroupName: ${example.name}
* location: ${example.location}
* skuName: Enterprise_E20-4
* example1:
* type: azure:redis:EnterpriseCluster
* properties:
* name: example-redisenterprise1
* resourceGroupName: ${example.name}
* location: ${example.location}
* skuName: Enterprise_E20-4
* exampleEnterpriseDatabase:
* type: azure:redis:EnterpriseDatabase
* name: example
* properties:
* name: default
* resourceGroupName: ${example.name}
* clusterId: ${exampleEnterpriseCluster.id}
* clientProtocol: Encrypted
* clusteringPolicy: EnterpriseCluster
* evictionPolicy: NoEviction
* port: 10000
* linkedDatabaseIds:
* - ${exampleEnterpriseCluster.id}/databases/default
* - ${example1.id}/databases/default
* linkedDatabaseGroupNickname: tftestGeoGroup
* ```
*
* ## Import
* Redis Enterprise Databases can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:redis/enterpriseDatabase:EnterpriseDatabase example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.Cache/redisEnterprise/cluster1/databases/database1
* ```
*/
public class EnterpriseDatabase internal constructor(
override val javaResource: com.pulumi.azure.redis.EnterpriseDatabase,
) : KotlinCustomResource(javaResource, EnterpriseDatabaseMapper) {
/**
* Specifies whether redis clients can connect using TLS-encrypted or plaintext redis protocols. Possible values are `Encrypted` and `Plaintext`. Defaults to `Encrypted`. Changing this forces a new Redis Enterprise Database to be created.
*/
public val clientProtocol: Output?
get() = javaResource.clientProtocol().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The resource id of the Redis Enterprise Cluster to deploy this Redis Enterprise Database. Changing this forces a new Redis Enterprise Database to be created.
*/
public val clusterId: Output
get() = javaResource.clusterId().applyValue({ args0 -> args0 })
/**
* Clustering policy Specified at create time. Possible values are `EnterpriseCluster` and `OSSCluster`. Defaults to `OSSCluster`. Changing this forces a new Redis Enterprise Database to be created.
*/
public val clusteringPolicy: Output?
get() = javaResource.clusteringPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Redis eviction policy possible values are `AllKeysLFU`, `AllKeysLRU`, `AllKeysRandom`, `VolatileLRU`, `VolatileLFU`, `VolatileTTL`, `VolatileRandom` and `NoEviction`. Changing this forces a new Redis Enterprise Database to be created. Defaults to `VolatileLRU`.
*/
public val evictionPolicy: Output?
get() = javaResource.evictionPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Nickname of the group of linked databases. Changing this force a new Redis Enterprise Geo Database to be created.
*/
public val linkedDatabaseGroupNickname: Output?
get() = javaResource.linkedDatabaseGroupNickname().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A list of database resources to link with this database with a maximum of 5.
* > **NOTE:** Only the newly created databases can be added to an existing geo-replication group. Existing regular databases or recreated databases cannot be added to the existing geo-replication group. Any linked database be removed from the list will be forcefully unlinked.The only recommended operation is to delete after force-unlink and the recommended scenario of force-unlink is region outrage. The database cannot be linked again after force-unlink.
*/
public val linkedDatabaseIds: Output>?
get() = javaResource.linkedDatabaseIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* A `module` block as defined below. Changing this forces a new resource to be created.
* > **NOTE:** Only `RediSearch` and `RedisJSON` modules are allowed with geo-replication
*/
public val modules: Output>?
get() = javaResource.modules().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> toKotlin(args0) })
})
}).orElse(null)
})
/**
* The name which should be used for this Redis Enterprise Database. Currently the acceptable value for this argument is `default`. Defaults to `default`. Changing this forces a new Redis Enterprise Database to be created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* TCP port of the database endpoint. Specified at create time. Defaults to an available port. Changing this forces a new Redis Enterprise Database to be created. Defaults to `10000`.
*/
public val port: Output?
get() = javaResource.port().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The Primary Access Key for the Redis Enterprise Database Instance.
*/
public val primaryAccessKey: Output
get() = javaResource.primaryAccessKey().applyValue({ args0 -> args0 })
/**
* The name of the Resource Group where the Redis Enterprise Database should exist. Changing this forces a new Redis Enterprise Database to be created.
*/
@Deprecated(
message = """
This field is no longer used and will be removed in the next major version of the Azure Provider
""",
)
public val resourceGroupName: Output
get() = javaResource.resourceGroupName().applyValue({ args0 -> args0 })
/**
* The Secondary Access Key for the Redis Enterprise Database Instance.
*/
public val secondaryAccessKey: Output
get() = javaResource.secondaryAccessKey().applyValue({ args0 -> args0 })
}
public object EnterpriseDatabaseMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azure.redis.EnterpriseDatabase::class == javaResource::class
override fun map(javaResource: Resource): EnterpriseDatabase = EnterpriseDatabase(
javaResource as
com.pulumi.azure.redis.EnterpriseDatabase,
)
}
/**
* @see [EnterpriseDatabase].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [EnterpriseDatabase].
*/
public suspend fun enterpriseDatabase(
name: String,
block: suspend EnterpriseDatabaseResourceBuilder.() -> Unit,
): EnterpriseDatabase {
val builder = EnterpriseDatabaseResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [EnterpriseDatabase].
* @param name The _unique_ name of the resulting resource.
*/
public fun enterpriseDatabase(name: String): EnterpriseDatabase {
val builder = EnterpriseDatabaseResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy