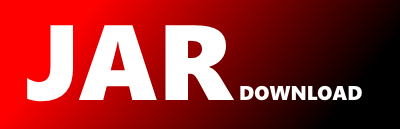
com.pulumi.azure.redis.kotlin.RedisFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.redis.kotlin
import com.pulumi.azure.redis.RedisFunctions.getCachePlain
import com.pulumi.azure.redis.RedisFunctions.getEnterpriseDatabasePlain
import com.pulumi.azure.redis.kotlin.inputs.GetCachePlainArgs
import com.pulumi.azure.redis.kotlin.inputs.GetCachePlainArgsBuilder
import com.pulumi.azure.redis.kotlin.inputs.GetEnterpriseDatabasePlainArgs
import com.pulumi.azure.redis.kotlin.inputs.GetEnterpriseDatabasePlainArgsBuilder
import com.pulumi.azure.redis.kotlin.outputs.GetCacheResult
import com.pulumi.azure.redis.kotlin.outputs.GetEnterpriseDatabaseResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azure.redis.kotlin.outputs.GetCacheResult.Companion.toKotlin as getCacheResultToKotlin
import com.pulumi.azure.redis.kotlin.outputs.GetEnterpriseDatabaseResult.Companion.toKotlin as getEnterpriseDatabaseResultToKotlin
public object RedisFunctions {
/**
* Use this data source to access information about an existing Redis Cache
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.redis.getCache({
* name: "myrediscache",
* resourceGroupName: "redis-cache",
* });
* export const primaryAccessKey = example.then(example => example.primaryAccessKey);
* export const hostname = example.then(example => example.hostname);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.redis.get_cache(name="myrediscache",
* resource_group_name="redis-cache")
* pulumi.export("primaryAccessKey", example.primary_access_key)
* pulumi.export("hostname", example.hostname)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.Redis.GetCache.Invoke(new()
* {
* Name = "myrediscache",
* ResourceGroupName = "redis-cache",
* });
* return new Dictionary
* {
* ["primaryAccessKey"] = example.Apply(getCacheResult => getCacheResult.PrimaryAccessKey),
* ["hostname"] = example.Apply(getCacheResult => getCacheResult.Hostname),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/redis"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := redis.LookupCache(ctx, &redis.LookupCacheArgs{
* Name: "myrediscache",
* ResourceGroupName: "redis-cache",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("primaryAccessKey", example.PrimaryAccessKey)
* ctx.Export("hostname", example.Hostname)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.redis.RedisFunctions;
* import com.pulumi.azure.redis.inputs.GetCacheArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = RedisFunctions.getCache(GetCacheArgs.builder()
* .name("myrediscache")
* .resourceGroupName("redis-cache")
* .build());
* ctx.export("primaryAccessKey", example.applyValue(getCacheResult -> getCacheResult.primaryAccessKey()));
* ctx.export("hostname", example.applyValue(getCacheResult -> getCacheResult.hostname()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:redis:getCache
* Arguments:
* name: myrediscache
* resourceGroupName: redis-cache
* outputs:
* primaryAccessKey: ${example.primaryAccessKey}
* hostname: ${example.hostname}
* ```
*
* @param argument A collection of arguments for invoking getCache.
* @return A collection of values returned by getCache.
*/
public suspend fun getCache(argument: GetCachePlainArgs): GetCacheResult =
getCacheResultToKotlin(getCachePlain(argument.toJava()).await())
/**
* @see [getCache].
* @param name The name of the Redis cache
* @param resourceGroupName The name of the resource group the Redis cache instance is located in.
* @return A collection of values returned by getCache.
*/
public suspend fun getCache(name: String, resourceGroupName: String): GetCacheResult {
val argument = GetCachePlainArgs(
name = name,
resourceGroupName = resourceGroupName,
)
return getCacheResultToKotlin(getCachePlain(argument.toJava()).await())
}
/**
* @see [getCache].
* @param argument Builder for [com.pulumi.azure.redis.kotlin.inputs.GetCachePlainArgs].
* @return A collection of values returned by getCache.
*/
public suspend fun getCache(argument: suspend GetCachePlainArgsBuilder.() -> Unit):
GetCacheResult {
val builder = GetCachePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getCacheResultToKotlin(getCachePlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Redis Enterprise Database
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.redis.getEnterpriseDatabase({
* name: "default",
* resourceGroupName: exampleAzurermResourceGroup.name,
* clusterId: exampleAzurermRedisEnterpriseCluster.id,
* });
* export const redisEnterpriseDatabasePrimaryKey = example.then(example => example.primaryAccessKey);
* export const redisEnterpriseDatabaseSecondaryKey = example.then(example => example.secondaryAccessKey);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.redis.get_enterprise_database(name="default",
* resource_group_name=example_azurerm_resource_group["name"],
* cluster_id=example_azurerm_redis_enterprise_cluster["id"])
* pulumi.export("redisEnterpriseDatabasePrimaryKey", example.primary_access_key)
* pulumi.export("redisEnterpriseDatabaseSecondaryKey", example.secondary_access_key)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.Redis.GetEnterpriseDatabase.Invoke(new()
* {
* Name = "default",
* ResourceGroupName = exampleAzurermResourceGroup.Name,
* ClusterId = exampleAzurermRedisEnterpriseCluster.Id,
* });
* return new Dictionary
* {
* ["redisEnterpriseDatabasePrimaryKey"] = example.Apply(getEnterpriseDatabaseResult => getEnterpriseDatabaseResult.PrimaryAccessKey),
* ["redisEnterpriseDatabaseSecondaryKey"] = example.Apply(getEnterpriseDatabaseResult => getEnterpriseDatabaseResult.SecondaryAccessKey),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/redis"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := redis.LookupEnterpriseDatabase(ctx, &redis.LookupEnterpriseDatabaseArgs{
* Name: "default",
* ResourceGroupName: pulumi.StringRef(exampleAzurermResourceGroup.Name),
* ClusterId: exampleAzurermRedisEnterpriseCluster.Id,
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("redisEnterpriseDatabasePrimaryKey", example.PrimaryAccessKey)
* ctx.Export("redisEnterpriseDatabaseSecondaryKey", example.SecondaryAccessKey)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.redis.RedisFunctions;
* import com.pulumi.azure.redis.inputs.GetEnterpriseDatabaseArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = RedisFunctions.getEnterpriseDatabase(GetEnterpriseDatabaseArgs.builder()
* .name("default")
* .resourceGroupName(exampleAzurermResourceGroup.name())
* .clusterId(exampleAzurermRedisEnterpriseCluster.id())
* .build());
* ctx.export("redisEnterpriseDatabasePrimaryKey", example.applyValue(getEnterpriseDatabaseResult -> getEnterpriseDatabaseResult.primaryAccessKey()));
* ctx.export("redisEnterpriseDatabaseSecondaryKey", example.applyValue(getEnterpriseDatabaseResult -> getEnterpriseDatabaseResult.secondaryAccessKey()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:redis:getEnterpriseDatabase
* Arguments:
* name: default
* resourceGroupName: ${exampleAzurermResourceGroup.name}
* clusterId: ${exampleAzurermRedisEnterpriseCluster.id}
* outputs:
* redisEnterpriseDatabasePrimaryKey: ${example.primaryAccessKey}
* redisEnterpriseDatabaseSecondaryKey: ${example.secondaryAccessKey}
* ```
*
* @param argument A collection of arguments for invoking getEnterpriseDatabase.
* @return A collection of values returned by getEnterpriseDatabase.
*/
public suspend fun getEnterpriseDatabase(argument: GetEnterpriseDatabasePlainArgs):
GetEnterpriseDatabaseResult =
getEnterpriseDatabaseResultToKotlin(getEnterpriseDatabasePlain(argument.toJava()).await())
/**
* @see [getEnterpriseDatabase].
* @param clusterId The resource ID of Redis Enterprise Cluster which hosts the Redis Enterprise Database instance.
* @param name The name of the Redis Enterprise Database.
* @param resourceGroupName The name of the resource group the Redis Enterprise Database instance is located in.
* @return A collection of values returned by getEnterpriseDatabase.
*/
public suspend fun getEnterpriseDatabase(
clusterId: String,
name: String,
resourceGroupName: String? = null,
): GetEnterpriseDatabaseResult {
val argument = GetEnterpriseDatabasePlainArgs(
clusterId = clusterId,
name = name,
resourceGroupName = resourceGroupName,
)
return getEnterpriseDatabaseResultToKotlin(getEnterpriseDatabasePlain(argument.toJava()).await())
}
/**
* @see [getEnterpriseDatabase].
* @param argument Builder for [com.pulumi.azure.redis.kotlin.inputs.GetEnterpriseDatabasePlainArgs].
* @return A collection of values returned by getEnterpriseDatabase.
*/
public suspend
fun getEnterpriseDatabase(argument: suspend GetEnterpriseDatabasePlainArgsBuilder.() -> Unit):
GetEnterpriseDatabaseResult {
val builder = GetEnterpriseDatabasePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getEnterpriseDatabaseResultToKotlin(getEnterpriseDatabasePlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy