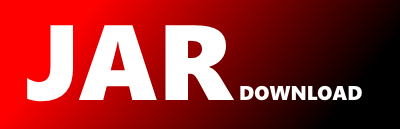
com.pulumi.azure.sentinel.kotlin.LogAnalyticsWorkspaceOnboardingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.sentinel.kotlin
import com.pulumi.azure.sentinel.LogAnalyticsWorkspaceOnboardingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages a Security Insights Sentinel Onboarding.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleAnalyticsWorkspace = new azure.operationalinsights.AnalyticsWorkspace("example", {
* name: "example-law",
* location: example.location,
* resourceGroupName: example.name,
* sku: "PerGB2018",
* });
* const exampleLogAnalyticsWorkspaceOnboarding = new azure.sentinel.LogAnalyticsWorkspaceOnboarding("example", {
* resourceGroupName: example.name,
* workspaceName: exampleAnalyticsWorkspace.name,
* customerManagedKeyEnabled: false,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_analytics_workspace = azure.operationalinsights.AnalyticsWorkspace("example",
* name="example-law",
* location=example.location,
* resource_group_name=example.name,
* sku="PerGB2018")
* example_log_analytics_workspace_onboarding = azure.sentinel.LogAnalyticsWorkspaceOnboarding("example",
* resource_group_name=example.name,
* workspace_name=example_analytics_workspace.name,
* customer_managed_key_enabled=False)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleAnalyticsWorkspace = new Azure.OperationalInsights.AnalyticsWorkspace("example", new()
* {
* Name = "example-law",
* Location = example.Location,
* ResourceGroupName = example.Name,
* Sku = "PerGB2018",
* });
* var exampleLogAnalyticsWorkspaceOnboarding = new Azure.Sentinel.LogAnalyticsWorkspaceOnboarding("example", new()
* {
* ResourceGroupName = example.Name,
* WorkspaceName = exampleAnalyticsWorkspace.Name,
* CustomerManagedKeyEnabled = false,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/operationalinsights"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/sentinel"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleAnalyticsWorkspace, err := operationalinsights.NewAnalyticsWorkspace(ctx, "example", &operationalinsights.AnalyticsWorkspaceArgs{
* Name: pulumi.String("example-law"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* Sku: pulumi.String("PerGB2018"),
* })
* if err != nil {
* return err
* }
* _, err = sentinel.NewLogAnalyticsWorkspaceOnboarding(ctx, "example", &sentinel.LogAnalyticsWorkspaceOnboardingArgs{
* ResourceGroupName: example.Name,
* WorkspaceName: exampleAnalyticsWorkspace.Name,
* CustomerManagedKeyEnabled: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.operationalinsights.AnalyticsWorkspace;
* import com.pulumi.azure.operationalinsights.AnalyticsWorkspaceArgs;
* import com.pulumi.azure.sentinel.LogAnalyticsWorkspaceOnboarding;
* import com.pulumi.azure.sentinel.LogAnalyticsWorkspaceOnboardingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleAnalyticsWorkspace = new AnalyticsWorkspace("exampleAnalyticsWorkspace", AnalyticsWorkspaceArgs.builder()
* .name("example-law")
* .location(example.location())
* .resourceGroupName(example.name())
* .sku("PerGB2018")
* .build());
* var exampleLogAnalyticsWorkspaceOnboarding = new LogAnalyticsWorkspaceOnboarding("exampleLogAnalyticsWorkspaceOnboarding", LogAnalyticsWorkspaceOnboardingArgs.builder()
* .resourceGroupName(example.name())
* .workspaceName(exampleAnalyticsWorkspace.name())
* .customerManagedKeyEnabled(false)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleAnalyticsWorkspace:
* type: azure:operationalinsights:AnalyticsWorkspace
* name: example
* properties:
* name: example-law
* location: ${example.location}
* resourceGroupName: ${example.name}
* sku: PerGB2018
* exampleLogAnalyticsWorkspaceOnboarding:
* type: azure:sentinel:LogAnalyticsWorkspaceOnboarding
* name: example
* properties:
* resourceGroupName: ${example.name}
* workspaceName: ${exampleAnalyticsWorkspace.name}
* customerManagedKeyEnabled: false
* ```
*
* ## Import
* Security Insights Sentinel Onboarding States can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:sentinel/logAnalyticsWorkspaceOnboarding:LogAnalyticsWorkspaceOnboarding example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resourceGroup1/providers/Microsoft.OperationalInsights/workspaces/workspace1/providers/Microsoft.SecurityInsights/onboardingStates/defaults
* ```
* @property customerManagedKeyEnabled Specifies if the Workspace is using Customer managed key. Defaults to `false`. Changing this forces a new resource to be created.
* > **Note:** To set up Microsoft Sentinel customer-managed key it needs to enable CMK on the workspace and add access policy to your Azure Key Vault. Details could be found on [this document](https://learn.microsoft.com/en-us/azure/sentinel/customer-managed-keys)
* > **Note:** Once a workspace is onboarded to Microsoft Sentinel with `customer_managed_key_enabled` set to true, it will not be able to be onboarded again with `customer_managed_key_enabled` set to false.
* @property resourceGroupName Specifies the name of the Resource Group where the Security Insights Sentinel Onboarding States should exist. Changing this forces the Log Analytics Workspace off the board and onboard again.
* @property workspaceId
* @property workspaceName Specifies the Workspace Name. Changing this forces the Log Analytics Workspace off the board and onboard again. Changing this forces a new resource to be created.
*/
public data class LogAnalyticsWorkspaceOnboardingArgs(
public val customerManagedKeyEnabled: Output? = null,
@Deprecated(
message = """
this property has been deprecated in favour of `workspace_id`
""",
)
public val resourceGroupName: Output? = null,
public val workspaceId: Output? = null,
@Deprecated(
message = """
this property will be removed in favour of `workspace_id` in version 4.0 of the AzureRM Provider
""",
)
public val workspaceName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.sentinel.LogAnalyticsWorkspaceOnboardingArgs =
com.pulumi.azure.sentinel.LogAnalyticsWorkspaceOnboardingArgs.builder()
.customerManagedKeyEnabled(customerManagedKeyEnabled?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.workspaceId(workspaceId?.applyValue({ args0 -> args0 }))
.workspaceName(workspaceName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LogAnalyticsWorkspaceOnboardingArgs].
*/
@PulumiTagMarker
public class LogAnalyticsWorkspaceOnboardingArgsBuilder internal constructor() {
private var customerManagedKeyEnabled: Output? = null
private var resourceGroupName: Output? = null
private var workspaceId: Output? = null
private var workspaceName: Output? = null
/**
* @param value Specifies if the Workspace is using Customer managed key. Defaults to `false`. Changing this forces a new resource to be created.
* > **Note:** To set up Microsoft Sentinel customer-managed key it needs to enable CMK on the workspace and add access policy to your Azure Key Vault. Details could be found on [this document](https://learn.microsoft.com/en-us/azure/sentinel/customer-managed-keys)
* > **Note:** Once a workspace is onboarded to Microsoft Sentinel with `customer_managed_key_enabled` set to true, it will not be able to be onboarded again with `customer_managed_key_enabled` set to false.
*/
@JvmName("aslttnisjurxvxju")
public suspend fun customerManagedKeyEnabled(`value`: Output) {
this.customerManagedKeyEnabled = value
}
/**
* @param value Specifies the name of the Resource Group where the Security Insights Sentinel Onboarding States should exist. Changing this forces the Log Analytics Workspace off the board and onboard again.
*/
@Deprecated(
message = """
this property has been deprecated in favour of `workspace_id`
""",
)
@JvmName("gaqmmnjrrqoobjpl")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value
*/
@JvmName("fduknxsrjqiwltur")
public suspend fun workspaceId(`value`: Output) {
this.workspaceId = value
}
/**
* @param value Specifies the Workspace Name. Changing this forces the Log Analytics Workspace off the board and onboard again. Changing this forces a new resource to be created.
*/
@Deprecated(
message = """
this property will be removed in favour of `workspace_id` in version 4.0 of the AzureRM Provider
""",
)
@JvmName("arogoyxfellohexp")
public suspend fun workspaceName(`value`: Output) {
this.workspaceName = value
}
/**
* @param value Specifies if the Workspace is using Customer managed key. Defaults to `false`. Changing this forces a new resource to be created.
* > **Note:** To set up Microsoft Sentinel customer-managed key it needs to enable CMK on the workspace and add access policy to your Azure Key Vault. Details could be found on [this document](https://learn.microsoft.com/en-us/azure/sentinel/customer-managed-keys)
* > **Note:** Once a workspace is onboarded to Microsoft Sentinel with `customer_managed_key_enabled` set to true, it will not be able to be onboarded again with `customer_managed_key_enabled` set to false.
*/
@JvmName("oafsxkechicprttn")
public suspend fun customerManagedKeyEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customerManagedKeyEnabled = mapped
}
/**
* @param value Specifies the name of the Resource Group where the Security Insights Sentinel Onboarding States should exist. Changing this forces the Log Analytics Workspace off the board and onboard again.
*/
@Deprecated(
message = """
this property has been deprecated in favour of `workspace_id`
""",
)
@JvmName("fngphxtqinppkvpm")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value
*/
@JvmName("jlacdqdwelkrjgbr")
public suspend fun workspaceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.workspaceId = mapped
}
/**
* @param value Specifies the Workspace Name. Changing this forces the Log Analytics Workspace off the board and onboard again. Changing this forces a new resource to be created.
*/
@Deprecated(
message = """
this property will be removed in favour of `workspace_id` in version 4.0 of the AzureRM Provider
""",
)
@JvmName("lhtjicfutgjoiori")
public suspend fun workspaceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.workspaceName = mapped
}
internal fun build(): LogAnalyticsWorkspaceOnboardingArgs = LogAnalyticsWorkspaceOnboardingArgs(
customerManagedKeyEnabled = customerManagedKeyEnabled,
resourceGroupName = resourceGroupName,
workspaceId = workspaceId,
workspaceName = workspaceName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy