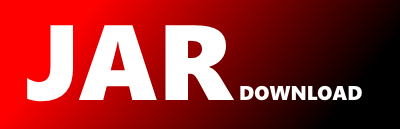
com.pulumi.azure.sentinel.kotlin.ThreatIntelligenceIndicatorArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.sentinel.kotlin
import com.pulumi.azure.sentinel.ThreatIntelligenceIndicatorArgs.builder
import com.pulumi.azure.sentinel.kotlin.inputs.ThreatIntelligenceIndicatorExternalReferenceArgs
import com.pulumi.azure.sentinel.kotlin.inputs.ThreatIntelligenceIndicatorExternalReferenceArgsBuilder
import com.pulumi.azure.sentinel.kotlin.inputs.ThreatIntelligenceIndicatorGranularMarkingArgs
import com.pulumi.azure.sentinel.kotlin.inputs.ThreatIntelligenceIndicatorGranularMarkingArgsBuilder
import com.pulumi.azure.sentinel.kotlin.inputs.ThreatIntelligenceIndicatorKillChainPhaseArgs
import com.pulumi.azure.sentinel.kotlin.inputs.ThreatIntelligenceIndicatorKillChainPhaseArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages a Sentinel Threat Intelligence Indicator.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-rg",
* location: "east us",
* });
* const exampleAnalyticsWorkspace = new azure.operationalinsights.AnalyticsWorkspace("example", {
* name: "example-law",
* location: example.location,
* resourceGroupName: example.name,
* sku: "PerGB2018",
* retentionInDays: 30,
* });
* const exampleLogAnalyticsWorkspaceOnboarding = new azure.sentinel.LogAnalyticsWorkspaceOnboarding("example", {
* resourceGroupName: example.name,
* workspaceName: exampleAnalyticsWorkspace.name,
* });
* const exampleThreatIntelligenceIndicator = new azure.sentinel.ThreatIntelligenceIndicator("example", {
* workspaceId: exampleAnalyticsWorkspace.id,
* patternType: "domain-name",
* pattern: "http://example.com",
* source: "Microsoft Sentinel",
* validateFromUtc: "2022-12-14T16:00:00Z",
* displayName: "example-indicator",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-rg",
* location="east us")
* example_analytics_workspace = azure.operationalinsights.AnalyticsWorkspace("example",
* name="example-law",
* location=example.location,
* resource_group_name=example.name,
* sku="PerGB2018",
* retention_in_days=30)
* example_log_analytics_workspace_onboarding = azure.sentinel.LogAnalyticsWorkspaceOnboarding("example",
* resource_group_name=example.name,
* workspace_name=example_analytics_workspace.name)
* example_threat_intelligence_indicator = azure.sentinel.ThreatIntelligenceIndicator("example",
* workspace_id=example_analytics_workspace.id,
* pattern_type="domain-name",
* pattern="http://example.com",
* source="Microsoft Sentinel",
* validate_from_utc="2022-12-14T16:00:00Z",
* display_name="example-indicator")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-rg",
* Location = "east us",
* });
* var exampleAnalyticsWorkspace = new Azure.OperationalInsights.AnalyticsWorkspace("example", new()
* {
* Name = "example-law",
* Location = example.Location,
* ResourceGroupName = example.Name,
* Sku = "PerGB2018",
* RetentionInDays = 30,
* });
* var exampleLogAnalyticsWorkspaceOnboarding = new Azure.Sentinel.LogAnalyticsWorkspaceOnboarding("example", new()
* {
* ResourceGroupName = example.Name,
* WorkspaceName = exampleAnalyticsWorkspace.Name,
* });
* var exampleThreatIntelligenceIndicator = new Azure.Sentinel.ThreatIntelligenceIndicator("example", new()
* {
* WorkspaceId = exampleAnalyticsWorkspace.Id,
* PatternType = "domain-name",
* Pattern = "http://example.com",
* Source = "Microsoft Sentinel",
* ValidateFromUtc = "2022-12-14T16:00:00Z",
* DisplayName = "example-indicator",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/operationalinsights"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/sentinel"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-rg"),
* Location: pulumi.String("east us"),
* })
* if err != nil {
* return err
* }
* exampleAnalyticsWorkspace, err := operationalinsights.NewAnalyticsWorkspace(ctx, "example", &operationalinsights.AnalyticsWorkspaceArgs{
* Name: pulumi.String("example-law"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* Sku: pulumi.String("PerGB2018"),
* RetentionInDays: pulumi.Int(30),
* })
* if err != nil {
* return err
* }
* _, err = sentinel.NewLogAnalyticsWorkspaceOnboarding(ctx, "example", &sentinel.LogAnalyticsWorkspaceOnboardingArgs{
* ResourceGroupName: example.Name,
* WorkspaceName: exampleAnalyticsWorkspace.Name,
* })
* if err != nil {
* return err
* }
* _, err = sentinel.NewThreatIntelligenceIndicator(ctx, "example", &sentinel.ThreatIntelligenceIndicatorArgs{
* WorkspaceId: exampleAnalyticsWorkspace.ID(),
* PatternType: pulumi.String("domain-name"),
* Pattern: pulumi.String("http://example.com"),
* Source: pulumi.String("Microsoft Sentinel"),
* ValidateFromUtc: pulumi.String("2022-12-14T16:00:00Z"),
* DisplayName: pulumi.String("example-indicator"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.operationalinsights.AnalyticsWorkspace;
* import com.pulumi.azure.operationalinsights.AnalyticsWorkspaceArgs;
* import com.pulumi.azure.sentinel.LogAnalyticsWorkspaceOnboarding;
* import com.pulumi.azure.sentinel.LogAnalyticsWorkspaceOnboardingArgs;
* import com.pulumi.azure.sentinel.ThreatIntelligenceIndicator;
* import com.pulumi.azure.sentinel.ThreatIntelligenceIndicatorArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-rg")
* .location("east us")
* .build());
* var exampleAnalyticsWorkspace = new AnalyticsWorkspace("exampleAnalyticsWorkspace", AnalyticsWorkspaceArgs.builder()
* .name("example-law")
* .location(example.location())
* .resourceGroupName(example.name())
* .sku("PerGB2018")
* .retentionInDays(30)
* .build());
* var exampleLogAnalyticsWorkspaceOnboarding = new LogAnalyticsWorkspaceOnboarding("exampleLogAnalyticsWorkspaceOnboarding", LogAnalyticsWorkspaceOnboardingArgs.builder()
* .resourceGroupName(example.name())
* .workspaceName(exampleAnalyticsWorkspace.name())
* .build());
* var exampleThreatIntelligenceIndicator = new ThreatIntelligenceIndicator("exampleThreatIntelligenceIndicator", ThreatIntelligenceIndicatorArgs.builder()
* .workspaceId(exampleAnalyticsWorkspace.id())
* .patternType("domain-name")
* .pattern("http://example.com")
* .source("Microsoft Sentinel")
* .validateFromUtc("2022-12-14T16:00:00Z")
* .displayName("example-indicator")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-rg
* location: east us
* exampleAnalyticsWorkspace:
* type: azure:operationalinsights:AnalyticsWorkspace
* name: example
* properties:
* name: example-law
* location: ${example.location}
* resourceGroupName: ${example.name}
* sku: PerGB2018
* retentionInDays: 30
* exampleLogAnalyticsWorkspaceOnboarding:
* type: azure:sentinel:LogAnalyticsWorkspaceOnboarding
* name: example
* properties:
* resourceGroupName: ${example.name}
* workspaceName: ${exampleAnalyticsWorkspace.name}
* exampleThreatIntelligenceIndicator:
* type: azure:sentinel:ThreatIntelligenceIndicator
* name: example
* properties:
* workspaceId: ${exampleAnalyticsWorkspace.id}
* patternType: domain-name
* pattern: http://example.com
* source: Microsoft Sentinel
* validateFromUtc: 2022-12-14T16:00:00Z
* displayName: example-indicator
* ```
*
* ## Import
* Sentinel Threat Intelligence Indicators can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:sentinel/threatIntelligenceIndicator:ThreatIntelligenceIndicator example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resourcegroup1/providers/Microsoft.OperationalInsights/workspaces/workspace1/providers/Microsoft.SecurityInsights/threatIntelligence/main/indicators/indicator1
* ```
* @property confidence Confidence levels of the Threat Intelligence Indicator.
* @property createdBy The creator of the Threat Intelligence Indicator.
* @property description The description of the Threat Intelligence Indicator.
* @property displayName The display name of the Threat Intelligence Indicator.
* @property extension The extension config of the Threat Intelligence Indicator in JSON format.
* @property externalReferences One or more `external_reference` blocks as defined below.
* @property granularMarkings One or more `granular_marking` blocks as defined below.
* @property killChainPhases One or more `kill_chain_phase` blocks as defined below.
* @property language The language of the Threat Intelligence Indicator.
* @property objectMarkingRefs Specifies a list of Threat Intelligence marking references.
* @property pattern The pattern used by the Threat Intelligence Indicator. When `pattern_type` set to `file`, `pattern` must be specified with `:` format, such as `MD5:78ecc5c05cd8b79af480df2f8fba0b9d`.
* @property patternType The type of pattern used by the Threat Intelligence Indicator. Possible values are `domain-name`, `file`, `ipv4-addr`, `ipv6-addr` and `url`.
* @property patternVersion The version of a Threat Intelligence entity.
* @property revoked Whether the Threat Intelligence entity revoked.
* @property source Source of the Threat Intelligence Indicator. Changing this forces a new resource to be created.
* @property tags Specifies a list of tags of the Threat Intelligence Indicator.
* @property threatTypes Specifies a list of threat types of this Threat Intelligence Indicator.
* @property validateFromUtc The start of validate date in RFC3339.
* @property validateUntilUtc The end of validate date of the Threat Intelligence Indicator in RFC3339 format.
* @property workspaceId The ID of the Log Analytics Workspace. Changing this forces a new Sentinel Threat Intelligence Indicator to be created.
*/
public data class ThreatIntelligenceIndicatorArgs(
public val confidence: Output? = null,
public val createdBy: Output? = null,
public val description: Output? = null,
public val displayName: Output? = null,
public val extension: Output? = null,
public val externalReferences: Output>? =
null,
public val granularMarkings: Output>? = null,
public val killChainPhases: Output>? = null,
public val language: Output? = null,
public val objectMarkingRefs: Output>? = null,
public val pattern: Output? = null,
public val patternType: Output? = null,
public val patternVersion: Output? = null,
public val revoked: Output? = null,
public val source: Output? = null,
public val tags: Output>? = null,
public val threatTypes: Output>? = null,
public val validateFromUtc: Output? = null,
public val validateUntilUtc: Output? = null,
public val workspaceId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.sentinel.ThreatIntelligenceIndicatorArgs =
com.pulumi.azure.sentinel.ThreatIntelligenceIndicatorArgs.builder()
.confidence(confidence?.applyValue({ args0 -> args0 }))
.createdBy(createdBy?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.extension(extension?.applyValue({ args0 -> args0 }))
.externalReferences(
externalReferences?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.granularMarkings(
granularMarkings?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.killChainPhases(
killChainPhases?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.language(language?.applyValue({ args0 -> args0 }))
.objectMarkingRefs(objectMarkingRefs?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.pattern(pattern?.applyValue({ args0 -> args0 }))
.patternType(patternType?.applyValue({ args0 -> args0 }))
.patternVersion(patternVersion?.applyValue({ args0 -> args0 }))
.revoked(revoked?.applyValue({ args0 -> args0 }))
.source(source?.applyValue({ args0 -> args0 }))
.tags(tags?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.threatTypes(threatTypes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.validateFromUtc(validateFromUtc?.applyValue({ args0 -> args0 }))
.validateUntilUtc(validateUntilUtc?.applyValue({ args0 -> args0 }))
.workspaceId(workspaceId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ThreatIntelligenceIndicatorArgs].
*/
@PulumiTagMarker
public class ThreatIntelligenceIndicatorArgsBuilder internal constructor() {
private var confidence: Output? = null
private var createdBy: Output? = null
private var description: Output? = null
private var displayName: Output? = null
private var extension: Output? = null
private var externalReferences: Output>? =
null
private var granularMarkings: Output>? = null
private var killChainPhases: Output>? = null
private var language: Output? = null
private var objectMarkingRefs: Output>? = null
private var pattern: Output? = null
private var patternType: Output? = null
private var patternVersion: Output? = null
private var revoked: Output? = null
private var source: Output? = null
private var tags: Output>? = null
private var threatTypes: Output>? = null
private var validateFromUtc: Output? = null
private var validateUntilUtc: Output? = null
private var workspaceId: Output? = null
/**
* @param value Confidence levels of the Threat Intelligence Indicator.
*/
@JvmName("xmahnpcovyqrlxig")
public suspend fun confidence(`value`: Output) {
this.confidence = value
}
/**
* @param value The creator of the Threat Intelligence Indicator.
*/
@JvmName("anrndyvvmpghnpvu")
public suspend fun createdBy(`value`: Output) {
this.createdBy = value
}
/**
* @param value The description of the Threat Intelligence Indicator.
*/
@JvmName("ypdnnoipksatvjsj")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The display name of the Threat Intelligence Indicator.
*/
@JvmName("asoewbgicbrtwvyt")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value The extension config of the Threat Intelligence Indicator in JSON format.
*/
@JvmName("uwmnnkawqnqtpgua")
public suspend fun extension(`value`: Output) {
this.extension = value
}
/**
* @param value One or more `external_reference` blocks as defined below.
*/
@JvmName("duwnnrkkowqttbor")
public suspend
fun externalReferences(`value`: Output>) {
this.externalReferences = value
}
@JvmName("dssmrywevqfrgfrs")
public suspend fun externalReferences(
vararg
values: Output,
) {
this.externalReferences = Output.all(values.asList())
}
/**
* @param values One or more `external_reference` blocks as defined below.
*/
@JvmName("vvklpostwcuoyqmg")
public suspend
fun externalReferences(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy