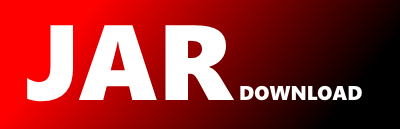
com.pulumi.azure.servicebus.kotlin.TopicArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.servicebus.kotlin
import com.pulumi.azure.servicebus.TopicArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages a ServiceBus Topic.
* **Note** Topics can only be created in Namespaces with an SKU of `standard` or higher.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "tfex-servicebus-topic",
* location: "West Europe",
* });
* const exampleNamespace = new azure.servicebus.Namespace("example", {
* name: "tfex-servicebus-namespace",
* location: example.location,
* resourceGroupName: example.name,
* sku: "Standard",
* tags: {
* source: "example",
* },
* });
* const exampleTopic = new azure.servicebus.Topic("example", {
* name: "tfex_servicebus_topic",
* namespaceId: exampleNamespace.id,
* enablePartitioning: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="tfex-servicebus-topic",
* location="West Europe")
* example_namespace = azure.servicebus.Namespace("example",
* name="tfex-servicebus-namespace",
* location=example.location,
* resource_group_name=example.name,
* sku="Standard",
* tags={
* "source": "example",
* })
* example_topic = azure.servicebus.Topic("example",
* name="tfex_servicebus_topic",
* namespace_id=example_namespace.id,
* enable_partitioning=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "tfex-servicebus-topic",
* Location = "West Europe",
* });
* var exampleNamespace = new Azure.ServiceBus.Namespace("example", new()
* {
* Name = "tfex-servicebus-namespace",
* Location = example.Location,
* ResourceGroupName = example.Name,
* Sku = "Standard",
* Tags =
* {
* { "source", "example" },
* },
* });
* var exampleTopic = new Azure.ServiceBus.Topic("example", new()
* {
* Name = "tfex_servicebus_topic",
* NamespaceId = exampleNamespace.Id,
* EnablePartitioning = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/servicebus"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("tfex-servicebus-topic"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleNamespace, err := servicebus.NewNamespace(ctx, "example", &servicebus.NamespaceArgs{
* Name: pulumi.String("tfex-servicebus-namespace"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* Sku: pulumi.String("Standard"),
* Tags: pulumi.StringMap{
* "source": pulumi.String("example"),
* },
* })
* if err != nil {
* return err
* }
* _, err = servicebus.NewTopic(ctx, "example", &servicebus.TopicArgs{
* Name: pulumi.String("tfex_servicebus_topic"),
* NamespaceId: exampleNamespace.ID(),
* EnablePartitioning: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.servicebus.Namespace;
* import com.pulumi.azure.servicebus.NamespaceArgs;
* import com.pulumi.azure.servicebus.Topic;
* import com.pulumi.azure.servicebus.TopicArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("tfex-servicebus-topic")
* .location("West Europe")
* .build());
* var exampleNamespace = new Namespace("exampleNamespace", NamespaceArgs.builder()
* .name("tfex-servicebus-namespace")
* .location(example.location())
* .resourceGroupName(example.name())
* .sku("Standard")
* .tags(Map.of("source", "example"))
* .build());
* var exampleTopic = new Topic("exampleTopic", TopicArgs.builder()
* .name("tfex_servicebus_topic")
* .namespaceId(exampleNamespace.id())
* .enablePartitioning(true)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: tfex-servicebus-topic
* location: West Europe
* exampleNamespace:
* type: azure:servicebus:Namespace
* name: example
* properties:
* name: tfex-servicebus-namespace
* location: ${example.location}
* resourceGroupName: ${example.name}
* sku: Standard
* tags:
* source: example
* exampleTopic:
* type: azure:servicebus:Topic
* name: example
* properties:
* name: tfex_servicebus_topic
* namespaceId: ${exampleNamespace.id}
* enablePartitioning: true
* ```
*
* ## Import
* Service Bus Topics can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:servicebus/topic:Topic example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/mygroup1/providers/Microsoft.ServiceBus/namespaces/sbns1/topics/sntopic1
* ```
* @property autoDeleteOnIdle The ISO 8601 timespan duration of the idle interval after which the Topic is automatically deleted, minimum of 5 minutes.
* @property defaultMessageTtl The ISO 8601 timespan duration of TTL of messages sent to this topic if no TTL value is set on the message itself.
* @property duplicateDetectionHistoryTimeWindow The ISO 8601 timespan duration during which duplicates can be detected. Defaults to 10 minutes. (`PT10M`)
* @property enableBatchedOperations Boolean flag which controls if server-side batched operations are enabled.
* @property enableExpress Boolean flag which controls whether Express Entities are enabled. An express topic holds a message in memory temporarily before writing it to persistent storage.
* @property enablePartitioning Boolean flag which controls whether to enable the topic to be partitioned across multiple message brokers. Changing this forces a new resource to be created.
* > **NOTE:** Partitioning is available at entity creation for all queues and topics in Basic or Standard SKUs. It is not available for the Premium messaging SKU, but any previously existing partitioned entities in Premium namespaces continue to work as expected. Please [see the documentation](https://docs.microsoft.com/azure/service-bus-messaging/service-bus-partitioning) for more information.
* @property maxMessageSizeInKilobytes Integer value which controls the maximum size of a message allowed on the topic for Premium SKU. For supported values see the "Large messages support" section of [this document](https://docs.microsoft.com/azure/service-bus-messaging/service-bus-premium-messaging#large-messages-support-preview).
* @property maxSizeInMegabytes Integer value which controls the size of memory allocated for the topic. For supported values see the "Queue/topic size" section of [this document](https://docs.microsoft.com/azure/service-bus-messaging/service-bus-quotas).
* @property name Specifies the name of the ServiceBus Topic resource. Changing this forces a new resource to be created.
* @property namespaceId The ID of the ServiceBus Namespace to create this topic in. Changing this forces a new resource to be created.
* @property requiresDuplicateDetection Boolean flag which controls whether the Topic requires duplicate detection. Defaults to `false`. Changing this forces a new resource to be created.
* @property status The Status of the Service Bus Topic. Acceptable values are `Active` or `Disabled`. Defaults to `Active`.
* @property supportOrdering Boolean flag which controls whether the Topic supports ordering.
*/
public data class TopicArgs(
public val autoDeleteOnIdle: Output? = null,
public val defaultMessageTtl: Output? = null,
public val duplicateDetectionHistoryTimeWindow: Output? = null,
public val enableBatchedOperations: Output? = null,
public val enableExpress: Output? = null,
public val enablePartitioning: Output? = null,
public val maxMessageSizeInKilobytes: Output? = null,
public val maxSizeInMegabytes: Output? = null,
public val name: Output? = null,
public val namespaceId: Output? = null,
public val requiresDuplicateDetection: Output? = null,
public val status: Output? = null,
public val supportOrdering: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.servicebus.TopicArgs =
com.pulumi.azure.servicebus.TopicArgs.builder()
.autoDeleteOnIdle(autoDeleteOnIdle?.applyValue({ args0 -> args0 }))
.defaultMessageTtl(defaultMessageTtl?.applyValue({ args0 -> args0 }))
.duplicateDetectionHistoryTimeWindow(
duplicateDetectionHistoryTimeWindow?.applyValue({ args0 ->
args0
}),
)
.enableBatchedOperations(enableBatchedOperations?.applyValue({ args0 -> args0 }))
.enableExpress(enableExpress?.applyValue({ args0 -> args0 }))
.enablePartitioning(enablePartitioning?.applyValue({ args0 -> args0 }))
.maxMessageSizeInKilobytes(maxMessageSizeInKilobytes?.applyValue({ args0 -> args0 }))
.maxSizeInMegabytes(maxSizeInMegabytes?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.namespaceId(namespaceId?.applyValue({ args0 -> args0 }))
.requiresDuplicateDetection(requiresDuplicateDetection?.applyValue({ args0 -> args0 }))
.status(status?.applyValue({ args0 -> args0 }))
.supportOrdering(supportOrdering?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TopicArgs].
*/
@PulumiTagMarker
public class TopicArgsBuilder internal constructor() {
private var autoDeleteOnIdle: Output? = null
private var defaultMessageTtl: Output? = null
private var duplicateDetectionHistoryTimeWindow: Output? = null
private var enableBatchedOperations: Output? = null
private var enableExpress: Output? = null
private var enablePartitioning: Output? = null
private var maxMessageSizeInKilobytes: Output? = null
private var maxSizeInMegabytes: Output? = null
private var name: Output? = null
private var namespaceId: Output? = null
private var requiresDuplicateDetection: Output? = null
private var status: Output? = null
private var supportOrdering: Output? = null
/**
* @param value The ISO 8601 timespan duration of the idle interval after which the Topic is automatically deleted, minimum of 5 minutes.
*/
@JvmName("pflhaeybfuaxhxog")
public suspend fun autoDeleteOnIdle(`value`: Output) {
this.autoDeleteOnIdle = value
}
/**
* @param value The ISO 8601 timespan duration of TTL of messages sent to this topic if no TTL value is set on the message itself.
*/
@JvmName("ggcatqntyoguxkip")
public suspend fun defaultMessageTtl(`value`: Output) {
this.defaultMessageTtl = value
}
/**
* @param value The ISO 8601 timespan duration during which duplicates can be detected. Defaults to 10 minutes. (`PT10M`)
*/
@JvmName("kmslqksedlmjxgam")
public suspend fun duplicateDetectionHistoryTimeWindow(`value`: Output) {
this.duplicateDetectionHistoryTimeWindow = value
}
/**
* @param value Boolean flag which controls if server-side batched operations are enabled.
*/
@JvmName("nbteonkuwhdsnlji")
public suspend fun enableBatchedOperations(`value`: Output) {
this.enableBatchedOperations = value
}
/**
* @param value Boolean flag which controls whether Express Entities are enabled. An express topic holds a message in memory temporarily before writing it to persistent storage.
*/
@JvmName("mpukcblinptuduex")
public suspend fun enableExpress(`value`: Output) {
this.enableExpress = value
}
/**
* @param value Boolean flag which controls whether to enable the topic to be partitioned across multiple message brokers. Changing this forces a new resource to be created.
* > **NOTE:** Partitioning is available at entity creation for all queues and topics in Basic or Standard SKUs. It is not available for the Premium messaging SKU, but any previously existing partitioned entities in Premium namespaces continue to work as expected. Please [see the documentation](https://docs.microsoft.com/azure/service-bus-messaging/service-bus-partitioning) for more information.
*/
@JvmName("qmhsefmlfolnvbqo")
public suspend fun enablePartitioning(`value`: Output) {
this.enablePartitioning = value
}
/**
* @param value Integer value which controls the maximum size of a message allowed on the topic for Premium SKU. For supported values see the "Large messages support" section of [this document](https://docs.microsoft.com/azure/service-bus-messaging/service-bus-premium-messaging#large-messages-support-preview).
*/
@JvmName("tndhebvgkvjtsqfh")
public suspend fun maxMessageSizeInKilobytes(`value`: Output) {
this.maxMessageSizeInKilobytes = value
}
/**
* @param value Integer value which controls the size of memory allocated for the topic. For supported values see the "Queue/topic size" section of [this document](https://docs.microsoft.com/azure/service-bus-messaging/service-bus-quotas).
*/
@JvmName("yciuwaiiiwedkxqh")
public suspend fun maxSizeInMegabytes(`value`: Output) {
this.maxSizeInMegabytes = value
}
/**
* @param value Specifies the name of the ServiceBus Topic resource. Changing this forces a new resource to be created.
*/
@JvmName("gusqblgaxguisfkm")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The ID of the ServiceBus Namespace to create this topic in. Changing this forces a new resource to be created.
*/
@JvmName("emfolleqccbrfglt")
public suspend fun namespaceId(`value`: Output) {
this.namespaceId = value
}
/**
* @param value Boolean flag which controls whether the Topic requires duplicate detection. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("uuuwhyhkyeepldha")
public suspend fun requiresDuplicateDetection(`value`: Output) {
this.requiresDuplicateDetection = value
}
/**
* @param value The Status of the Service Bus Topic. Acceptable values are `Active` or `Disabled`. Defaults to `Active`.
*/
@JvmName("sjysqurwhtuawnaa")
public suspend fun status(`value`: Output) {
this.status = value
}
/**
* @param value Boolean flag which controls whether the Topic supports ordering.
*/
@JvmName("iknpdljwwlaljyig")
public suspend fun supportOrdering(`value`: Output) {
this.supportOrdering = value
}
/**
* @param value The ISO 8601 timespan duration of the idle interval after which the Topic is automatically deleted, minimum of 5 minutes.
*/
@JvmName("ficlvqkxgcgjdody")
public suspend fun autoDeleteOnIdle(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autoDeleteOnIdle = mapped
}
/**
* @param value The ISO 8601 timespan duration of TTL of messages sent to this topic if no TTL value is set on the message itself.
*/
@JvmName("dlubqrldjwtinshf")
public suspend fun defaultMessageTtl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultMessageTtl = mapped
}
/**
* @param value The ISO 8601 timespan duration during which duplicates can be detected. Defaults to 10 minutes. (`PT10M`)
*/
@JvmName("kbarhnxpaiklpveu")
public suspend fun duplicateDetectionHistoryTimeWindow(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.duplicateDetectionHistoryTimeWindow = mapped
}
/**
* @param value Boolean flag which controls if server-side batched operations are enabled.
*/
@JvmName("owagqqwpfqgtdvxm")
public suspend fun enableBatchedOperations(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableBatchedOperations = mapped
}
/**
* @param value Boolean flag which controls whether Express Entities are enabled. An express topic holds a message in memory temporarily before writing it to persistent storage.
*/
@JvmName("cockshribsjyxmhh")
public suspend fun enableExpress(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableExpress = mapped
}
/**
* @param value Boolean flag which controls whether to enable the topic to be partitioned across multiple message brokers. Changing this forces a new resource to be created.
* > **NOTE:** Partitioning is available at entity creation for all queues and topics in Basic or Standard SKUs. It is not available for the Premium messaging SKU, but any previously existing partitioned entities in Premium namespaces continue to work as expected. Please [see the documentation](https://docs.microsoft.com/azure/service-bus-messaging/service-bus-partitioning) for more information.
*/
@JvmName("ckleigntjslpwxio")
public suspend fun enablePartitioning(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enablePartitioning = mapped
}
/**
* @param value Integer value which controls the maximum size of a message allowed on the topic for Premium SKU. For supported values see the "Large messages support" section of [this document](https://docs.microsoft.com/azure/service-bus-messaging/service-bus-premium-messaging#large-messages-support-preview).
*/
@JvmName("awgddviojaqnsmkv")
public suspend fun maxMessageSizeInKilobytes(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxMessageSizeInKilobytes = mapped
}
/**
* @param value Integer value which controls the size of memory allocated for the topic. For supported values see the "Queue/topic size" section of [this document](https://docs.microsoft.com/azure/service-bus-messaging/service-bus-quotas).
*/
@JvmName("cwlingudsdhgainl")
public suspend fun maxSizeInMegabytes(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxSizeInMegabytes = mapped
}
/**
* @param value Specifies the name of the ServiceBus Topic resource. Changing this forces a new resource to be created.
*/
@JvmName("etsqlwdejqtmudws")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The ID of the ServiceBus Namespace to create this topic in. Changing this forces a new resource to be created.
*/
@JvmName("vtcuemmrocssxfly")
public suspend fun namespaceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespaceId = mapped
}
/**
* @param value Boolean flag which controls whether the Topic requires duplicate detection. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("kpcaihxcpcardjcj")
public suspend fun requiresDuplicateDetection(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requiresDuplicateDetection = mapped
}
/**
* @param value The Status of the Service Bus Topic. Acceptable values are `Active` or `Disabled`. Defaults to `Active`.
*/
@JvmName("glmnjtkxsmthiklg")
public suspend fun status(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.status = mapped
}
/**
* @param value Boolean flag which controls whether the Topic supports ordering.
*/
@JvmName("escnkuwyoylnfvyg")
public suspend fun supportOrdering(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.supportOrdering = mapped
}
internal fun build(): TopicArgs = TopicArgs(
autoDeleteOnIdle = autoDeleteOnIdle,
defaultMessageTtl = defaultMessageTtl,
duplicateDetectionHistoryTimeWindow = duplicateDetectionHistoryTimeWindow,
enableBatchedOperations = enableBatchedOperations,
enableExpress = enableExpress,
enablePartitioning = enablePartitioning,
maxMessageSizeInKilobytes = maxMessageSizeInKilobytes,
maxSizeInMegabytes = maxSizeInMegabytes,
name = name,
namespaceId = namespaceId,
requiresDuplicateDetection = requiresDuplicateDetection,
status = status,
supportOrdering = supportOrdering,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy