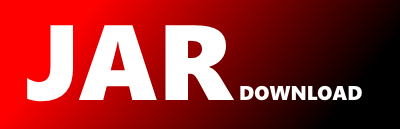
com.pulumi.azure.storage.kotlin.MoverSourceEndpointArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.storage.kotlin
import com.pulumi.azure.storage.MoverSourceEndpointArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages a Storage Mover Source Endpoint.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleMover = new azure.storage.Mover("example", {
* name: "example-ssm",
* resourceGroupName: example.name,
* location: "West Europe",
* });
* const exampleMoverSourceEndpoint = new azure.storage.MoverSourceEndpoint("example", {
* name: "example-se",
* storageMoverId: exampleMover.id,
* "export": "/",
* host: "192.168.0.1",
* nfsVersion: "NFSv3",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_mover = azure.storage.Mover("example",
* name="example-ssm",
* resource_group_name=example.name,
* location="West Europe")
* example_mover_source_endpoint = azure.storage.MoverSourceEndpoint("example",
* name="example-se",
* storage_mover_id=example_mover.id,
* export="/",
* host="192.168.0.1",
* nfs_version="NFSv3")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleMover = new Azure.Storage.Mover("example", new()
* {
* Name = "example-ssm",
* ResourceGroupName = example.Name,
* Location = "West Europe",
* });
* var exampleMoverSourceEndpoint = new Azure.Storage.MoverSourceEndpoint("example", new()
* {
* Name = "example-se",
* StorageMoverId = exampleMover.Id,
* Export = "/",
* Host = "192.168.0.1",
* NfsVersion = "NFSv3",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleMover, err := storage.NewMover(ctx, "example", &storage.MoverArgs{
* Name: pulumi.String("example-ssm"),
* ResourceGroupName: example.Name,
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* _, err = storage.NewMoverSourceEndpoint(ctx, "example", &storage.MoverSourceEndpointArgs{
* Name: pulumi.String("example-se"),
* StorageMoverId: exampleMover.ID(),
* Export: pulumi.String("/"),
* Host: pulumi.String("192.168.0.1"),
* NfsVersion: pulumi.String("NFSv3"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.storage.Mover;
* import com.pulumi.azure.storage.MoverArgs;
* import com.pulumi.azure.storage.MoverSourceEndpoint;
* import com.pulumi.azure.storage.MoverSourceEndpointArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleMover = new Mover("exampleMover", MoverArgs.builder()
* .name("example-ssm")
* .resourceGroupName(example.name())
* .location("West Europe")
* .build());
* var exampleMoverSourceEndpoint = new MoverSourceEndpoint("exampleMoverSourceEndpoint", MoverSourceEndpointArgs.builder()
* .name("example-se")
* .storageMoverId(exampleMover.id())
* .export("/")
* .host("192.168.0.1")
* .nfsVersion("NFSv3")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleMover:
* type: azure:storage:Mover
* name: example
* properties:
* name: example-ssm
* resourceGroupName: ${example.name}
* location: West Europe
* exampleMoverSourceEndpoint:
* type: azure:storage:MoverSourceEndpoint
* name: example
* properties:
* name: example-se
* storageMoverId: ${exampleMover.id}
* export: /
* host: 192.168.0.1
* nfsVersion: NFSv3
* ```
*
* ## Import
* Storage Mover Source Endpoint can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:storage/moverSourceEndpoint:MoverSourceEndpoint example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resourceGroup1/providers/Microsoft.StorageMover/storageMovers/storageMover1/endpoints/endpoint1
* ```
* @property description Specifies a description for the Storage Mover Source Endpoint.
* @property export Specifies the directory being exported from the server. Changing this forces a new resource to be created.
* @property host Specifies the host name or IP address of the server exporting the file system. Changing this forces a new resource to be created.
* @property name Specifies the name which should be used for this Storage Mover Source Endpoint. Changing this forces a new resource to be created.
* @property nfsVersion Specifies the NFS protocol version. Possible values are `NFSauto`, `NFSv3` and `NFSv4`. Defaults to `NFSauto`. Changing this forces a new resource to be created.
* @property storageMoverId Specifies the ID of the Storage Mover for this Storage Mover Source Endpoint. Changing this forces a new resource to be created.
*/
public data class MoverSourceEndpointArgs(
public val description: Output? = null,
public val export: Output? = null,
public val host: Output? = null,
public val name: Output? = null,
public val nfsVersion: Output? = null,
public val storageMoverId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.storage.MoverSourceEndpointArgs =
com.pulumi.azure.storage.MoverSourceEndpointArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.export(export?.applyValue({ args0 -> args0 }))
.host(host?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.nfsVersion(nfsVersion?.applyValue({ args0 -> args0 }))
.storageMoverId(storageMoverId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MoverSourceEndpointArgs].
*/
@PulumiTagMarker
public class MoverSourceEndpointArgsBuilder internal constructor() {
private var description: Output? = null
private var export: Output? = null
private var host: Output? = null
private var name: Output? = null
private var nfsVersion: Output? = null
private var storageMoverId: Output? = null
/**
* @param value Specifies a description for the Storage Mover Source Endpoint.
*/
@JvmName("wmirmudgvbsweyvw")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Specifies the directory being exported from the server. Changing this forces a new resource to be created.
*/
@JvmName("xwobyrovtqcfkgbu")
public suspend fun export(`value`: Output) {
this.export = value
}
/**
* @param value Specifies the host name or IP address of the server exporting the file system. Changing this forces a new resource to be created.
*/
@JvmName("ifgkdwwjrmlkkdss")
public suspend fun host(`value`: Output) {
this.host = value
}
/**
* @param value Specifies the name which should be used for this Storage Mover Source Endpoint. Changing this forces a new resource to be created.
*/
@JvmName("wwfhdfremwvmnbmi")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Specifies the NFS protocol version. Possible values are `NFSauto`, `NFSv3` and `NFSv4`. Defaults to `NFSauto`. Changing this forces a new resource to be created.
*/
@JvmName("iuabniwbaytbtdon")
public suspend fun nfsVersion(`value`: Output) {
this.nfsVersion = value
}
/**
* @param value Specifies the ID of the Storage Mover for this Storage Mover Source Endpoint. Changing this forces a new resource to be created.
*/
@JvmName("grypldwyrkyiveib")
public suspend fun storageMoverId(`value`: Output) {
this.storageMoverId = value
}
/**
* @param value Specifies a description for the Storage Mover Source Endpoint.
*/
@JvmName("yduiwwuruaorbyqk")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Specifies the directory being exported from the server. Changing this forces a new resource to be created.
*/
@JvmName("kymlskefdikhcmyk")
public suspend fun export(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.export = mapped
}
/**
* @param value Specifies the host name or IP address of the server exporting the file system. Changing this forces a new resource to be created.
*/
@JvmName("tgqwcyakfxfdyomo")
public suspend fun host(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.host = mapped
}
/**
* @param value Specifies the name which should be used for this Storage Mover Source Endpoint. Changing this forces a new resource to be created.
*/
@JvmName("smdlwxlewwisawwb")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Specifies the NFS protocol version. Possible values are `NFSauto`, `NFSv3` and `NFSv4`. Defaults to `NFSauto`. Changing this forces a new resource to be created.
*/
@JvmName("wjlfyeeomdimkuer")
public suspend fun nfsVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nfsVersion = mapped
}
/**
* @param value Specifies the ID of the Storage Mover for this Storage Mover Source Endpoint. Changing this forces a new resource to be created.
*/
@JvmName("uprnieepmesmacja")
public suspend fun storageMoverId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageMoverId = mapped
}
internal fun build(): MoverSourceEndpointArgs = MoverSourceEndpointArgs(
description = description,
export = export,
host = host,
name = name,
nfsVersion = nfsVersion,
storageMoverId = storageMoverId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy