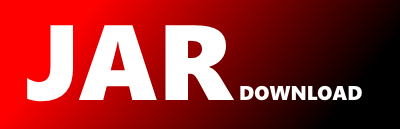
com.pulumi.azure.storage.kotlin.inputs.ManagementPolicyRuleActionsVersionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.storage.kotlin.inputs
import com.pulumi.azure.storage.inputs.ManagementPolicyRuleActionsVersionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property changeTierToArchiveAfterDaysSinceCreation The age in days after creation to tier blob version to archive storage. Must be between 0 and 99999. Defaults to `-1`.
* @property changeTierToCoolAfterDaysSinceCreation The age in days creation create to tier blob version to cool storage. Must be between 0 and 99999. Defaults to `-1`.
* @property deleteAfterDaysSinceCreation The age in days after creation to delete the blob version. Must be between 0 and 99999. Defaults to `-1`.
* @property tierToArchiveAfterDaysSinceLastTierChangeGreaterThan The age in days after last tier change to the blobs to skip to be archved. Must be between 0 and 99999. Defaults to `-1`.
* @property tierToColdAfterDaysSinceCreationGreaterThan The age in days after creation to cold storage. Supports blob currently at Hot tier. Must be between `0` and `99999`. Defaults to `-1`.
*/
public data class ManagementPolicyRuleActionsVersionArgs(
public val changeTierToArchiveAfterDaysSinceCreation: Output? = null,
public val changeTierToCoolAfterDaysSinceCreation: Output? = null,
public val deleteAfterDaysSinceCreation: Output? = null,
public val tierToArchiveAfterDaysSinceLastTierChangeGreaterThan: Output? = null,
public val tierToColdAfterDaysSinceCreationGreaterThan: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.storage.inputs.ManagementPolicyRuleActionsVersionArgs =
com.pulumi.azure.storage.inputs.ManagementPolicyRuleActionsVersionArgs.builder()
.changeTierToArchiveAfterDaysSinceCreation(
changeTierToArchiveAfterDaysSinceCreation?.applyValue({ args0 ->
args0
}),
)
.changeTierToCoolAfterDaysSinceCreation(
changeTierToCoolAfterDaysSinceCreation?.applyValue({ args0 ->
args0
}),
)
.deleteAfterDaysSinceCreation(deleteAfterDaysSinceCreation?.applyValue({ args0 -> args0 }))
.tierToArchiveAfterDaysSinceLastTierChangeGreaterThan(
tierToArchiveAfterDaysSinceLastTierChangeGreaterThan?.applyValue({ args0 ->
args0
}),
)
.tierToColdAfterDaysSinceCreationGreaterThan(
tierToColdAfterDaysSinceCreationGreaterThan?.applyValue({ args0 ->
args0
}),
).build()
}
/**
* Builder for [ManagementPolicyRuleActionsVersionArgs].
*/
@PulumiTagMarker
public class ManagementPolicyRuleActionsVersionArgsBuilder internal constructor() {
private var changeTierToArchiveAfterDaysSinceCreation: Output? = null
private var changeTierToCoolAfterDaysSinceCreation: Output? = null
private var deleteAfterDaysSinceCreation: Output? = null
private var tierToArchiveAfterDaysSinceLastTierChangeGreaterThan: Output? = null
private var tierToColdAfterDaysSinceCreationGreaterThan: Output? = null
/**
* @param value The age in days after creation to tier blob version to archive storage. Must be between 0 and 99999. Defaults to `-1`.
*/
@JvmName("lvidokedninlmbxy")
public suspend fun changeTierToArchiveAfterDaysSinceCreation(`value`: Output) {
this.changeTierToArchiveAfterDaysSinceCreation = value
}
/**
* @param value The age in days creation create to tier blob version to cool storage. Must be between 0 and 99999. Defaults to `-1`.
*/
@JvmName("ckdiisdbacatlnib")
public suspend fun changeTierToCoolAfterDaysSinceCreation(`value`: Output) {
this.changeTierToCoolAfterDaysSinceCreation = value
}
/**
* @param value The age in days after creation to delete the blob version. Must be between 0 and 99999. Defaults to `-1`.
*/
@JvmName("qrigvwswenkgiona")
public suspend fun deleteAfterDaysSinceCreation(`value`: Output) {
this.deleteAfterDaysSinceCreation = value
}
/**
* @param value The age in days after last tier change to the blobs to skip to be archved. Must be between 0 and 99999. Defaults to `-1`.
*/
@JvmName("opigivymvefpdjym")
public suspend fun tierToArchiveAfterDaysSinceLastTierChangeGreaterThan(`value`: Output) {
this.tierToArchiveAfterDaysSinceLastTierChangeGreaterThan = value
}
/**
* @param value The age in days after creation to cold storage. Supports blob currently at Hot tier. Must be between `0` and `99999`. Defaults to `-1`.
*/
@JvmName("flauyoopqylvatab")
public suspend fun tierToColdAfterDaysSinceCreationGreaterThan(`value`: Output) {
this.tierToColdAfterDaysSinceCreationGreaterThan = value
}
/**
* @param value The age in days after creation to tier blob version to archive storage. Must be between 0 and 99999. Defaults to `-1`.
*/
@JvmName("xlesdyengwwowgau")
public suspend fun changeTierToArchiveAfterDaysSinceCreation(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.changeTierToArchiveAfterDaysSinceCreation = mapped
}
/**
* @param value The age in days creation create to tier blob version to cool storage. Must be between 0 and 99999. Defaults to `-1`.
*/
@JvmName("hgjjgqacmsqgyyrf")
public suspend fun changeTierToCoolAfterDaysSinceCreation(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.changeTierToCoolAfterDaysSinceCreation = mapped
}
/**
* @param value The age in days after creation to delete the blob version. Must be between 0 and 99999. Defaults to `-1`.
*/
@JvmName("okftkosgjeryrtwd")
public suspend fun deleteAfterDaysSinceCreation(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deleteAfterDaysSinceCreation = mapped
}
/**
* @param value The age in days after last tier change to the blobs to skip to be archved. Must be between 0 and 99999. Defaults to `-1`.
*/
@JvmName("ljrjspwixawfhjdm")
public suspend fun tierToArchiveAfterDaysSinceLastTierChangeGreaterThan(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tierToArchiveAfterDaysSinceLastTierChangeGreaterThan = mapped
}
/**
* @param value The age in days after creation to cold storage. Supports blob currently at Hot tier. Must be between `0` and `99999`. Defaults to `-1`.
*/
@JvmName("ybaojcjqxiealnsj")
public suspend fun tierToColdAfterDaysSinceCreationGreaterThan(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tierToColdAfterDaysSinceCreationGreaterThan = mapped
}
internal fun build(): ManagementPolicyRuleActionsVersionArgs =
ManagementPolicyRuleActionsVersionArgs(
changeTierToArchiveAfterDaysSinceCreation = changeTierToArchiveAfterDaysSinceCreation,
changeTierToCoolAfterDaysSinceCreation = changeTierToCoolAfterDaysSinceCreation,
deleteAfterDaysSinceCreation = deleteAfterDaysSinceCreation,
tierToArchiveAfterDaysSinceLastTierChangeGreaterThan = tierToArchiveAfterDaysSinceLastTierChangeGreaterThan,
tierToColdAfterDaysSinceCreationGreaterThan = tierToColdAfterDaysSinceCreationGreaterThan,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy