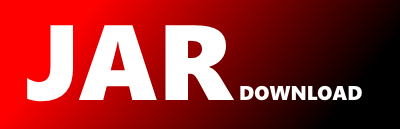
com.pulumi.azure.storage.kotlin.outputs.GetAccountResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.storage.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getAccount.
* @property accessTier The access tier for `BlobStorage` accounts.
* @property accountKind The Kind of account.
* @property accountReplicationType The type of replication used for this storage account.
* @property accountTier The Tier of this storage account.
* @property allowNestedItemsToBePublic Can nested items in the storage account opt into allowing public access?
* @property azureFilesAuthentications A `azure_files_authentication` block as documented below.
* @property customDomains supports the following:
* @property enableHttpsTrafficOnly Is traffic only allowed via HTTPS? See [here](https://docs.microsoft.com/azure/storage/storage-require-secure-transfer/)
* for more information.
* @property id The provider-assigned unique ID for this managed resource.
* @property identities An `identity` block as documented below.
* @property infrastructureEncryptionEnabled Is infrastructure encryption enabled? See [here](https://docs.microsoft.com/azure/storage/common/infrastructure-encryption-enable/)
* for more information.
* @property isHnsEnabled Is Hierarchical Namespace enabled?
* @property location The Azure location where the Storage Account exists
* @property minTlsVersion The minimum supported TLS version for this storage account.
* @property name The Custom Domain Name used for the Storage Account.
* @property nfsv3Enabled Is NFSv3 protocol enabled?
* @property primaryAccessKey The primary access key for the Storage Account.
* @property primaryBlobConnectionString The connection string associated with the primary blob location
* @property primaryBlobEndpoint The endpoint URL for blob storage in the primary location.
* @property primaryBlobHost The hostname with port if applicable for blob storage in the primary location.
* @property primaryBlobInternetEndpoint The internet routing endpoint URL for blob storage in the primary location.
* @property primaryBlobInternetHost The internet routing hostname with port if applicable for blob storage in the primary location.
* @property primaryBlobMicrosoftEndpoint The microsoft routing endpoint URL for blob storage in the primary location.
* @property primaryBlobMicrosoftHost The microsoft routing hostname with port if applicable for blob storage in the primary location.
* @property primaryConnectionString The connection string associated with the primary location
* @property primaryDfsEndpoint The endpoint URL for DFS storage in the primary location.
* @property primaryDfsHost The hostname with port if applicable for DFS storage in the primary location.
* @property primaryDfsInternetEndpoint The internet routing endpoint URL for DFS storage in the primary location.
* @property primaryDfsInternetHost The internet routing hostname with port if applicable for DFS storage in the primary location.
* @property primaryDfsMicrosoftEndpoint The microsoft routing endpoint URL for DFS storage in the primary location.
* @property primaryDfsMicrosoftHost The microsoft routing hostname with port if applicable for DFS storage in the primary location.
* @property primaryFileEndpoint The endpoint URL for file storage in the primary location.
* @property primaryFileHost The hostname with port if applicable for file storage in the primary location.
* @property primaryFileInternetEndpoint The internet routing endpoint URL for file storage in the primary location.
* @property primaryFileInternetHost The internet routing hostname with port if applicable for file storage in the primary location.
* @property primaryFileMicrosoftEndpoint The microsoft routing endpoint URL for file storage in the primary location.
* @property primaryFileMicrosoftHost The microsoft routing hostname with port if applicable for file storage in the primary location.
* @property primaryLocation The primary location of the Storage Account.
* @property primaryQueueEndpoint The endpoint URL for queue storage in the primary location.
* @property primaryQueueHost The hostname with port if applicable for queue storage in the primary location.
* @property primaryQueueMicrosoftEndpoint The microsoft routing endpoint URL for queue storage in the primary location.
* @property primaryQueueMicrosoftHost The microsoft routing hostname with port if applicable for queue storage in the primary location.
* @property primaryTableEndpoint The endpoint URL for table storage in the primary location.
* @property primaryTableHost The hostname with port if applicable for table storage in the primary location.
* @property primaryTableMicrosoftEndpoint The microsoft routing endpoint URL for table storage in the primary location.
* @property primaryTableMicrosoftHost The microsoft routing hostname with port if applicable for table storage in the primary location.
* @property primaryWebEndpoint The endpoint URL for web storage in the primary location.
* @property primaryWebHost The hostname with port if applicable for web storage in the primary location.
* @property primaryWebInternetEndpoint The internet routing endpoint URL for web storage in the primary location.
* @property primaryWebInternetHost The internet routing hostname with port if applicable for web storage in the primary location.
* @property primaryWebMicrosoftEndpoint The microsoft routing endpoint URL for web storage in the primary location.
* @property primaryWebMicrosoftHost The microsoft routing hostname with port if applicable for web storage in the primary location.
* @property queueEncryptionKeyType The encryption key type of the queue.
* @property resourceGroupName
* @property secondaryAccessKey The secondary access key for the Storage Account.
* @property secondaryBlobConnectionString The connection string associated with the secondary blob location
* @property secondaryBlobEndpoint The endpoint URL for blob storage in the secondary location.
* @property secondaryBlobHost The hostname with port if applicable for blob storage in the secondary location.
* @property secondaryBlobInternetEndpoint The internet routing endpoint URL for blob storage in the secondary location.
* @property secondaryBlobInternetHost The internet routing hostname with port if applicable for blob storage in the secondary location.
* @property secondaryBlobMicrosoftEndpoint The microsoft routing endpoint URL for blob storage in the secondary location.
* @property secondaryBlobMicrosoftHost The microsoft routing hostname with port if applicable for blob storage in the secondary location.
* @property secondaryConnectionString The connection string associated with the secondary location
* @property secondaryDfsEndpoint The endpoint URL for DFS storage in the secondary location.
* @property secondaryDfsHost The hostname with port if applicable for DFS storage in the secondary location.
* @property secondaryDfsInternetEndpoint The internet routing endpoint URL for DFS storage in the secondary location.
* @property secondaryDfsInternetHost The internet routing hostname with port if applicable for DFS storage in the secondary location.
* @property secondaryDfsMicrosoftEndpoint The microsoft routing endpoint URL for DFS storage in the secondary location.
* @property secondaryDfsMicrosoftHost The microsoft routing hostname with port if applicable for DFS storage in the secondary location.
* @property secondaryFileEndpoint The endpoint URL for file storage in the secondary location.
* @property secondaryFileHost The hostname with port if applicable for file storage in the secondary location.
* @property secondaryFileInternetEndpoint The internet routing endpoint URL for file storage in the secondary location.
* @property secondaryFileInternetHost The internet routing hostname with port if applicable for file storage in the secondary location.
* @property secondaryFileMicrosoftEndpoint The microsoft routing endpoint URL for file storage in the secondary location.
* @property secondaryFileMicrosoftHost The microsoft routing hostname with port if applicable for file storage in the secondary location.
* @property secondaryLocation The secondary location of the Storage Account.
* @property secondaryQueueEndpoint The endpoint URL for queue storage in the secondary location.
* @property secondaryQueueHost The hostname with port if applicable for queue storage in the secondary location.
* @property secondaryQueueMicrosoftEndpoint The microsoft routing endpoint URL for queue storage in the secondary location.
* @property secondaryQueueMicrosoftHost The microsoft routing hostname with port if applicable for queue storage in the secondary location.
* @property secondaryTableEndpoint The endpoint URL for table storage in the secondary location.
* @property secondaryTableHost The hostname with port if applicable for table storage in the secondary location.
* @property secondaryTableMicrosoftEndpoint The microsoft routing endpoint URL for table storage in the secondary location.
* @property secondaryTableMicrosoftHost The microsoft routing hostname with port if applicable for table storage in the secondary location.
* @property secondaryWebEndpoint The endpoint URL for web storage in the secondary location.
* @property secondaryWebHost The hostname with port if applicable for web storage in the secondary location.
* @property secondaryWebInternetEndpoint The internet routing endpoint URL for web storage in the secondary location.
* @property secondaryWebInternetHost The internet routing hostname with port if applicable for web storage in the secondary location.
* @property secondaryWebMicrosoftEndpoint The microsoft routing endpoint URL for web storage in the secondary location.
* @property secondaryWebMicrosoftHost The microsoft routing hostname with port if applicable for web storage in the secondary location.
* @property tableEncryptionKeyType The encryption key type of the table.
* @property tags A mapping of tags to assigned to the resource.
*/
public data class GetAccountResult(
public val accessTier: String,
public val accountKind: String,
public val accountReplicationType: String,
public val accountTier: String,
public val allowNestedItemsToBePublic: Boolean,
public val azureFilesAuthentications: List,
public val customDomains: List,
public val enableHttpsTrafficOnly: Boolean,
public val id: String,
public val identities: List,
public val infrastructureEncryptionEnabled: Boolean,
public val isHnsEnabled: Boolean,
public val location: String,
public val minTlsVersion: String? = null,
public val name: String,
public val nfsv3Enabled: Boolean,
public val primaryAccessKey: String,
public val primaryBlobConnectionString: String,
public val primaryBlobEndpoint: String,
public val primaryBlobHost: String,
public val primaryBlobInternetEndpoint: String,
public val primaryBlobInternetHost: String,
public val primaryBlobMicrosoftEndpoint: String,
public val primaryBlobMicrosoftHost: String,
public val primaryConnectionString: String,
public val primaryDfsEndpoint: String,
public val primaryDfsHost: String,
public val primaryDfsInternetEndpoint: String,
public val primaryDfsInternetHost: String,
public val primaryDfsMicrosoftEndpoint: String,
public val primaryDfsMicrosoftHost: String,
public val primaryFileEndpoint: String,
public val primaryFileHost: String,
public val primaryFileInternetEndpoint: String,
public val primaryFileInternetHost: String,
public val primaryFileMicrosoftEndpoint: String,
public val primaryFileMicrosoftHost: String,
public val primaryLocation: String,
public val primaryQueueEndpoint: String,
public val primaryQueueHost: String,
public val primaryQueueMicrosoftEndpoint: String,
public val primaryQueueMicrosoftHost: String,
public val primaryTableEndpoint: String,
public val primaryTableHost: String,
public val primaryTableMicrosoftEndpoint: String,
public val primaryTableMicrosoftHost: String,
public val primaryWebEndpoint: String,
public val primaryWebHost: String,
public val primaryWebInternetEndpoint: String,
public val primaryWebInternetHost: String,
public val primaryWebMicrosoftEndpoint: String,
public val primaryWebMicrosoftHost: String,
public val queueEncryptionKeyType: String,
public val resourceGroupName: String? = null,
public val secondaryAccessKey: String,
public val secondaryBlobConnectionString: String,
public val secondaryBlobEndpoint: String,
public val secondaryBlobHost: String,
public val secondaryBlobInternetEndpoint: String,
public val secondaryBlobInternetHost: String,
public val secondaryBlobMicrosoftEndpoint: String,
public val secondaryBlobMicrosoftHost: String,
public val secondaryConnectionString: String,
public val secondaryDfsEndpoint: String,
public val secondaryDfsHost: String,
public val secondaryDfsInternetEndpoint: String,
public val secondaryDfsInternetHost: String,
public val secondaryDfsMicrosoftEndpoint: String,
public val secondaryDfsMicrosoftHost: String,
public val secondaryFileEndpoint: String,
public val secondaryFileHost: String,
public val secondaryFileInternetEndpoint: String,
public val secondaryFileInternetHost: String,
public val secondaryFileMicrosoftEndpoint: String,
public val secondaryFileMicrosoftHost: String,
public val secondaryLocation: String,
public val secondaryQueueEndpoint: String,
public val secondaryQueueHost: String,
public val secondaryQueueMicrosoftEndpoint: String,
public val secondaryQueueMicrosoftHost: String,
public val secondaryTableEndpoint: String,
public val secondaryTableHost: String,
public val secondaryTableMicrosoftEndpoint: String,
public val secondaryTableMicrosoftHost: String,
public val secondaryWebEndpoint: String,
public val secondaryWebHost: String,
public val secondaryWebInternetEndpoint: String,
public val secondaryWebInternetHost: String,
public val secondaryWebMicrosoftEndpoint: String,
public val secondaryWebMicrosoftHost: String,
public val tableEncryptionKeyType: String,
public val tags: Map,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.storage.outputs.GetAccountResult):
GetAccountResult = GetAccountResult(
accessTier = javaType.accessTier(),
accountKind = javaType.accountKind(),
accountReplicationType = javaType.accountReplicationType(),
accountTier = javaType.accountTier(),
allowNestedItemsToBePublic = javaType.allowNestedItemsToBePublic(),
azureFilesAuthentications = javaType.azureFilesAuthentications().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.storage.kotlin.outputs.GetAccountAzureFilesAuthentication.Companion.toKotlin(args0)
})
}),
customDomains = javaType.customDomains().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.storage.kotlin.outputs.GetAccountCustomDomain.Companion.toKotlin(args0)
})
}),
enableHttpsTrafficOnly = javaType.enableHttpsTrafficOnly(),
id = javaType.id(),
identities = javaType.identities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.storage.kotlin.outputs.GetAccountIdentity.Companion.toKotlin(args0)
})
}),
infrastructureEncryptionEnabled = javaType.infrastructureEncryptionEnabled(),
isHnsEnabled = javaType.isHnsEnabled(),
location = javaType.location(),
minTlsVersion = javaType.minTlsVersion().map({ args0 -> args0 }).orElse(null),
name = javaType.name(),
nfsv3Enabled = javaType.nfsv3Enabled(),
primaryAccessKey = javaType.primaryAccessKey(),
primaryBlobConnectionString = javaType.primaryBlobConnectionString(),
primaryBlobEndpoint = javaType.primaryBlobEndpoint(),
primaryBlobHost = javaType.primaryBlobHost(),
primaryBlobInternetEndpoint = javaType.primaryBlobInternetEndpoint(),
primaryBlobInternetHost = javaType.primaryBlobInternetHost(),
primaryBlobMicrosoftEndpoint = javaType.primaryBlobMicrosoftEndpoint(),
primaryBlobMicrosoftHost = javaType.primaryBlobMicrosoftHost(),
primaryConnectionString = javaType.primaryConnectionString(),
primaryDfsEndpoint = javaType.primaryDfsEndpoint(),
primaryDfsHost = javaType.primaryDfsHost(),
primaryDfsInternetEndpoint = javaType.primaryDfsInternetEndpoint(),
primaryDfsInternetHost = javaType.primaryDfsInternetHost(),
primaryDfsMicrosoftEndpoint = javaType.primaryDfsMicrosoftEndpoint(),
primaryDfsMicrosoftHost = javaType.primaryDfsMicrosoftHost(),
primaryFileEndpoint = javaType.primaryFileEndpoint(),
primaryFileHost = javaType.primaryFileHost(),
primaryFileInternetEndpoint = javaType.primaryFileInternetEndpoint(),
primaryFileInternetHost = javaType.primaryFileInternetHost(),
primaryFileMicrosoftEndpoint = javaType.primaryFileMicrosoftEndpoint(),
primaryFileMicrosoftHost = javaType.primaryFileMicrosoftHost(),
primaryLocation = javaType.primaryLocation(),
primaryQueueEndpoint = javaType.primaryQueueEndpoint(),
primaryQueueHost = javaType.primaryQueueHost(),
primaryQueueMicrosoftEndpoint = javaType.primaryQueueMicrosoftEndpoint(),
primaryQueueMicrosoftHost = javaType.primaryQueueMicrosoftHost(),
primaryTableEndpoint = javaType.primaryTableEndpoint(),
primaryTableHost = javaType.primaryTableHost(),
primaryTableMicrosoftEndpoint = javaType.primaryTableMicrosoftEndpoint(),
primaryTableMicrosoftHost = javaType.primaryTableMicrosoftHost(),
primaryWebEndpoint = javaType.primaryWebEndpoint(),
primaryWebHost = javaType.primaryWebHost(),
primaryWebInternetEndpoint = javaType.primaryWebInternetEndpoint(),
primaryWebInternetHost = javaType.primaryWebInternetHost(),
primaryWebMicrosoftEndpoint = javaType.primaryWebMicrosoftEndpoint(),
primaryWebMicrosoftHost = javaType.primaryWebMicrosoftHost(),
queueEncryptionKeyType = javaType.queueEncryptionKeyType(),
resourceGroupName = javaType.resourceGroupName().map({ args0 -> args0 }).orElse(null),
secondaryAccessKey = javaType.secondaryAccessKey(),
secondaryBlobConnectionString = javaType.secondaryBlobConnectionString(),
secondaryBlobEndpoint = javaType.secondaryBlobEndpoint(),
secondaryBlobHost = javaType.secondaryBlobHost(),
secondaryBlobInternetEndpoint = javaType.secondaryBlobInternetEndpoint(),
secondaryBlobInternetHost = javaType.secondaryBlobInternetHost(),
secondaryBlobMicrosoftEndpoint = javaType.secondaryBlobMicrosoftEndpoint(),
secondaryBlobMicrosoftHost = javaType.secondaryBlobMicrosoftHost(),
secondaryConnectionString = javaType.secondaryConnectionString(),
secondaryDfsEndpoint = javaType.secondaryDfsEndpoint(),
secondaryDfsHost = javaType.secondaryDfsHost(),
secondaryDfsInternetEndpoint = javaType.secondaryDfsInternetEndpoint(),
secondaryDfsInternetHost = javaType.secondaryDfsInternetHost(),
secondaryDfsMicrosoftEndpoint = javaType.secondaryDfsMicrosoftEndpoint(),
secondaryDfsMicrosoftHost = javaType.secondaryDfsMicrosoftHost(),
secondaryFileEndpoint = javaType.secondaryFileEndpoint(),
secondaryFileHost = javaType.secondaryFileHost(),
secondaryFileInternetEndpoint = javaType.secondaryFileInternetEndpoint(),
secondaryFileInternetHost = javaType.secondaryFileInternetHost(),
secondaryFileMicrosoftEndpoint = javaType.secondaryFileMicrosoftEndpoint(),
secondaryFileMicrosoftHost = javaType.secondaryFileMicrosoftHost(),
secondaryLocation = javaType.secondaryLocation(),
secondaryQueueEndpoint = javaType.secondaryQueueEndpoint(),
secondaryQueueHost = javaType.secondaryQueueHost(),
secondaryQueueMicrosoftEndpoint = javaType.secondaryQueueMicrosoftEndpoint(),
secondaryQueueMicrosoftHost = javaType.secondaryQueueMicrosoftHost(),
secondaryTableEndpoint = javaType.secondaryTableEndpoint(),
secondaryTableHost = javaType.secondaryTableHost(),
secondaryTableMicrosoftEndpoint = javaType.secondaryTableMicrosoftEndpoint(),
secondaryTableMicrosoftHost = javaType.secondaryTableMicrosoftHost(),
secondaryWebEndpoint = javaType.secondaryWebEndpoint(),
secondaryWebHost = javaType.secondaryWebHost(),
secondaryWebInternetEndpoint = javaType.secondaryWebInternetEndpoint(),
secondaryWebInternetHost = javaType.secondaryWebInternetHost(),
secondaryWebMicrosoftEndpoint = javaType.secondaryWebMicrosoftEndpoint(),
secondaryWebMicrosoftHost = javaType.secondaryWebMicrosoftHost(),
tableEncryptionKeyType = javaType.tableEncryptionKeyType(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy