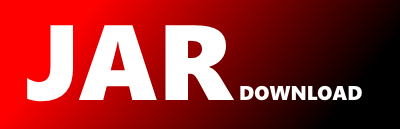
com.pulumi.azure.storage.kotlin.outputs.ManagementPolicyRuleActionsBaseBlob.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.storage.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.Suppress
/**
*
* @property autoTierToHotFromCoolEnabled Whether a blob should automatically be tiered from cool back to hot if it's accessed again after being tiered to cool. Defaults to `false`.
* > **Note:** The `auto_tier_to_hot_from_cool_enabled` must be used together with `tier_to_cool_after_days_since_last_access_time_greater_than`.
* @property deleteAfterDaysSinceCreationGreaterThan The age in days after creation to delete the blob. Must be between `0` and `99999`. Defaults to `-1`.
* > **Note:** The `delete_after_days_since_modification_greater_than`, `delete_after_days_since_last_access_time_greater_than` and `delete_after_days_since_creation_greater_than` can not be set at the same time.
* > **Note:** The `last_access_time_enabled` must be set to `true` in the `azure.storage.Account` in order to use `tier_to_cool_after_days_since_last_access_time_greater_than`, `tier_to_archive_after_days_since_last_access_time_greater_than` and `delete_after_days_since_last_access_time_greater_than`.
* @property deleteAfterDaysSinceLastAccessTimeGreaterThan The age in days after last access time to delete the blob. Must be between `0` and `99999`. Defaults to `-1`.
* @property deleteAfterDaysSinceModificationGreaterThan The age in days after last modification to delete the blob. Must be between 0 and 99999. Defaults to `-1`.
* @property tierToArchiveAfterDaysSinceCreationGreaterThan The age in days after creation to archive storage. Supports blob currently at Hot or Cool tier. Must be between `0` and`99999`. Defaults to `-1`.
* > **Note:** The `tier_to_archive_after_days_since_modification_greater_than`, `tier_to_archive_after_days_since_last_access_time_greater_than` and `tier_to_archive_after_days_since_creation_greater_than` can not be set at the same time.
* @property tierToArchiveAfterDaysSinceLastAccessTimeGreaterThan The age in days after last access time to tier blobs to archive storage. Supports blob currently at Hot or Cool tier. Must be between `0` and`99999`. Defaults to `-1`.
* @property tierToArchiveAfterDaysSinceLastTierChangeGreaterThan The age in days after last tier change to the blobs to skip to be archved. Must be between 0 and 99999. Defaults to `-1`.
* @property tierToArchiveAfterDaysSinceModificationGreaterThan The age in days after last modification to tier blobs to archive storage. Supports blob currently at Hot or Cool tier. Must be between 0 and 99999. Defaults to `-1`.
* @property tierToColdAfterDaysSinceCreationGreaterThan The age in days after creation to cold storage. Supports blob currently at Hot tier. Must be between `0` and `99999`. Defaults to `-1`.
* > **Note:** The `tier_to_cool_after_days_since_modification_greater_than`, `tier_to_cool_after_days_since_last_access_time_greater_than` and `tier_to_cool_after_days_since_creation_greater_than` can not be set at the same time.
* @property tierToColdAfterDaysSinceLastAccessTimeGreaterThan The age in days after last access time to tier blobs to cold storage. Supports blob currently at Hot tier. Must be between `0` and `99999`. Defaults to `-1`.
* @property tierToColdAfterDaysSinceModificationGreaterThan The age in days after last modification to tier blobs to cold storage. Supports blob currently at Hot tier. Must be between 0 and 99999. Defaults to `-1`.
* @property tierToCoolAfterDaysSinceCreationGreaterThan The age in days after creation to cool storage. Supports blob currently at Hot tier. Must be between `0` and `99999`. Defaults to `-1`.
* > **Note:** The `tier_to_cool_after_days_since_modification_greater_than`, `tier_to_cool_after_days_since_last_access_time_greater_than` and `tier_to_cool_after_days_since_creation_greater_than` can not be set at the same time.
* @property tierToCoolAfterDaysSinceLastAccessTimeGreaterThan The age in days after last access time to tier blobs to cool storage. Supports blob currently at Hot tier. Must be between `0` and `99999`. Defaults to `-1`.
* @property tierToCoolAfterDaysSinceModificationGreaterThan The age in days after last modification to tier blobs to cool storage. Supports blob currently at Hot tier. Must be between 0 and 99999. Defaults to `-1`.
*/
public data class ManagementPolicyRuleActionsBaseBlob(
public val autoTierToHotFromCoolEnabled: Boolean? = null,
public val deleteAfterDaysSinceCreationGreaterThan: Int? = null,
public val deleteAfterDaysSinceLastAccessTimeGreaterThan: Int? = null,
public val deleteAfterDaysSinceModificationGreaterThan: Int? = null,
public val tierToArchiveAfterDaysSinceCreationGreaterThan: Int? = null,
public val tierToArchiveAfterDaysSinceLastAccessTimeGreaterThan: Int? = null,
public val tierToArchiveAfterDaysSinceLastTierChangeGreaterThan: Int? = null,
public val tierToArchiveAfterDaysSinceModificationGreaterThan: Int? = null,
public val tierToColdAfterDaysSinceCreationGreaterThan: Int? = null,
public val tierToColdAfterDaysSinceLastAccessTimeGreaterThan: Int? = null,
public val tierToColdAfterDaysSinceModificationGreaterThan: Int? = null,
public val tierToCoolAfterDaysSinceCreationGreaterThan: Int? = null,
public val tierToCoolAfterDaysSinceLastAccessTimeGreaterThan: Int? = null,
public val tierToCoolAfterDaysSinceModificationGreaterThan: Int? = null,
) {
public companion object {
public
fun toKotlin(javaType: com.pulumi.azure.storage.outputs.ManagementPolicyRuleActionsBaseBlob):
ManagementPolicyRuleActionsBaseBlob = ManagementPolicyRuleActionsBaseBlob(
autoTierToHotFromCoolEnabled = javaType.autoTierToHotFromCoolEnabled().map({ args0 ->
args0
}).orElse(null),
deleteAfterDaysSinceCreationGreaterThan = javaType.deleteAfterDaysSinceCreationGreaterThan().map({ args0 ->
args0
}).orElse(null),
deleteAfterDaysSinceLastAccessTimeGreaterThan = javaType.deleteAfterDaysSinceLastAccessTimeGreaterThan().map({ args0 ->
args0
}).orElse(null),
deleteAfterDaysSinceModificationGreaterThan = javaType.deleteAfterDaysSinceModificationGreaterThan().map({ args0 ->
args0
}).orElse(null),
tierToArchiveAfterDaysSinceCreationGreaterThan = javaType.tierToArchiveAfterDaysSinceCreationGreaterThan().map({ args0 ->
args0
}).orElse(null),
tierToArchiveAfterDaysSinceLastAccessTimeGreaterThan = javaType.tierToArchiveAfterDaysSinceLastAccessTimeGreaterThan().map({ args0 ->
args0
}).orElse(null),
tierToArchiveAfterDaysSinceLastTierChangeGreaterThan = javaType.tierToArchiveAfterDaysSinceLastTierChangeGreaterThan().map({ args0 ->
args0
}).orElse(null),
tierToArchiveAfterDaysSinceModificationGreaterThan = javaType.tierToArchiveAfterDaysSinceModificationGreaterThan().map({ args0 ->
args0
}).orElse(null),
tierToColdAfterDaysSinceCreationGreaterThan = javaType.tierToColdAfterDaysSinceCreationGreaterThan().map({ args0 ->
args0
}).orElse(null),
tierToColdAfterDaysSinceLastAccessTimeGreaterThan = javaType.tierToColdAfterDaysSinceLastAccessTimeGreaterThan().map({ args0 ->
args0
}).orElse(null),
tierToColdAfterDaysSinceModificationGreaterThan = javaType.tierToColdAfterDaysSinceModificationGreaterThan().map({ args0 ->
args0
}).orElse(null),
tierToCoolAfterDaysSinceCreationGreaterThan = javaType.tierToCoolAfterDaysSinceCreationGreaterThan().map({ args0 ->
args0
}).orElse(null),
tierToCoolAfterDaysSinceLastAccessTimeGreaterThan = javaType.tierToCoolAfterDaysSinceLastAccessTimeGreaterThan().map({ args0 ->
args0
}).orElse(null),
tierToCoolAfterDaysSinceModificationGreaterThan = javaType.tierToCoolAfterDaysSinceModificationGreaterThan().map({ args0 ->
args0
}).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy