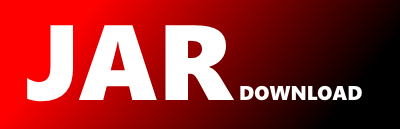
com.pulumi.azure.voice.kotlin.ServicesCommunicationsGatewayArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.voice.kotlin
import com.pulumi.azure.voice.ServicesCommunicationsGatewayArgs.builder
import com.pulumi.azure.voice.kotlin.inputs.ServicesCommunicationsGatewayServiceLocationArgs
import com.pulumi.azure.voice.kotlin.inputs.ServicesCommunicationsGatewayServiceLocationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages a Voice Services Communications Gateways.
* !> **NOTE:** You must have signed an Operator Connect agreement with Microsoft to use this resource. For more information, see [`Prerequisites`](https://learn.microsoft.com/en-us/azure/communications-gateway/prepare-to-deploy#prerequisites).
* !> **NOTE:** Access to Azure Communications Gateway is restricted, see [`Get access to Azure Communications Gateway for your Azure subscription`](https://learn.microsoft.com/en-us/azure/communications-gateway/prepare-to-deploy#9-get-access-to-azure-communications-gateway-for-your-azure-subscription) for details.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleServicesCommunicationsGateway = new azure.voice.ServicesCommunicationsGateway("example", {
* name: "example-vcg",
* location: "West Europe",
* resourceGroupName: example.name,
* connectivity: "PublicAddress",
* codecs: "PCMA",
* e911Type: "DirectToEsrp",
* platforms: [
* "OperatorConnect",
* "TeamsPhoneMobile",
* ],
* serviceLocations: [
* {
* location: "eastus",
* allowedMediaSourceAddressPrefixes: ["10.1.2.0/24"],
* allowedSignalingSourceAddressPrefixes: ["10.1.1.0/24"],
* esrpAddresses: ["198.51.100.3"],
* operatorAddresses: ["198.51.100.1"],
* },
* {
* location: "eastus2",
* allowedMediaSourceAddressPrefixes: ["10.2.2.0/24"],
* allowedSignalingSourceAddressPrefixes: ["10.2.1.0/24"],
* esrpAddresses: ["198.51.100.4"],
* operatorAddresses: ["198.51.100.2"],
* },
* ],
* autoGeneratedDomainNameLabelScope: "SubscriptionReuse",
* apiBridge: JSON.stringify({}),
* emergencyDialStrings: [
* "911",
* "933",
* ],
* onPremMcpEnabled: false,
* tags: {
* key: "value",
* },
* microsoftTeamsVoicemailPilotNumber: "1",
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_services_communications_gateway = azure.voice.ServicesCommunicationsGateway("example",
* name="example-vcg",
* location="West Europe",
* resource_group_name=example.name,
* connectivity="PublicAddress",
* codecs="PCMA",
* e911_type="DirectToEsrp",
* platforms=[
* "OperatorConnect",
* "TeamsPhoneMobile",
* ],
* service_locations=[
* azure.voice.ServicesCommunicationsGatewayServiceLocationArgs(
* location="eastus",
* allowed_media_source_address_prefixes=["10.1.2.0/24"],
* allowed_signaling_source_address_prefixes=["10.1.1.0/24"],
* esrp_addresses=["198.51.100.3"],
* operator_addresses=["198.51.100.1"],
* ),
* azure.voice.ServicesCommunicationsGatewayServiceLocationArgs(
* location="eastus2",
* allowed_media_source_address_prefixes=["10.2.2.0/24"],
* allowed_signaling_source_address_prefixes=["10.2.1.0/24"],
* esrp_addresses=["198.51.100.4"],
* operator_addresses=["198.51.100.2"],
* ),
* ],
* auto_generated_domain_name_label_scope="SubscriptionReuse",
* api_bridge=json.dumps({}),
* emergency_dial_strings=[
* "911",
* "933",
* ],
* on_prem_mcp_enabled=False,
* tags={
* "key": "value",
* },
* microsoft_teams_voicemail_pilot_number="1")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleServicesCommunicationsGateway = new Azure.Voice.ServicesCommunicationsGateway("example", new()
* {
* Name = "example-vcg",
* Location = "West Europe",
* ResourceGroupName = example.Name,
* Connectivity = "PublicAddress",
* Codecs = "PCMA",
* E911Type = "DirectToEsrp",
* Platforms = new[]
* {
* "OperatorConnect",
* "TeamsPhoneMobile",
* },
* ServiceLocations = new[]
* {
* new Azure.Voice.Inputs.ServicesCommunicationsGatewayServiceLocationArgs
* {
* Location = "eastus",
* AllowedMediaSourceAddressPrefixes = new[]
* {
* "10.1.2.0/24",
* },
* AllowedSignalingSourceAddressPrefixes = new[]
* {
* "10.1.1.0/24",
* },
* EsrpAddresses = new[]
* {
* "198.51.100.3",
* },
* OperatorAddresses = new[]
* {
* "198.51.100.1",
* },
* },
* new Azure.Voice.Inputs.ServicesCommunicationsGatewayServiceLocationArgs
* {
* Location = "eastus2",
* AllowedMediaSourceAddressPrefixes = new[]
* {
* "10.2.2.0/24",
* },
* AllowedSignalingSourceAddressPrefixes = new[]
* {
* "10.2.1.0/24",
* },
* EsrpAddresses = new[]
* {
* "198.51.100.4",
* },
* OperatorAddresses = new[]
* {
* "198.51.100.2",
* },
* },
* },
* AutoGeneratedDomainNameLabelScope = "SubscriptionReuse",
* ApiBridge = JsonSerializer.Serialize(new Dictionary
* {
* }),
* EmergencyDialStrings = new[]
* {
* "911",
* "933",
* },
* OnPremMcpEnabled = false,
* Tags =
* {
* { "key", "value" },
* },
* MicrosoftTeamsVoicemailPilotNumber = "1",
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/voice"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* tmpJSON0, err := json.Marshal(nil)
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = voice.NewServicesCommunicationsGateway(ctx, "example", &voice.ServicesCommunicationsGatewayArgs{
* Name: pulumi.String("example-vcg"),
* Location: pulumi.String("West Europe"),
* ResourceGroupName: example.Name,
* Connectivity: pulumi.String("PublicAddress"),
* Codecs: pulumi.String("PCMA"),
* E911Type: pulumi.String("DirectToEsrp"),
* Platforms: pulumi.StringArray{
* pulumi.String("OperatorConnect"),
* pulumi.String("TeamsPhoneMobile"),
* },
* ServiceLocations: voice.ServicesCommunicationsGatewayServiceLocationArray{
* &voice.ServicesCommunicationsGatewayServiceLocationArgs{
* Location: pulumi.String("eastus"),
* AllowedMediaSourceAddressPrefixes: pulumi.StringArray{
* pulumi.String("10.1.2.0/24"),
* },
* AllowedSignalingSourceAddressPrefixes: pulumi.StringArray{
* pulumi.String("10.1.1.0/24"),
* },
* EsrpAddresses: pulumi.StringArray{
* pulumi.String("198.51.100.3"),
* },
* OperatorAddresses: pulumi.StringArray{
* pulumi.String("198.51.100.1"),
* },
* },
* &voice.ServicesCommunicationsGatewayServiceLocationArgs{
* Location: pulumi.String("eastus2"),
* AllowedMediaSourceAddressPrefixes: pulumi.StringArray{
* pulumi.String("10.2.2.0/24"),
* },
* AllowedSignalingSourceAddressPrefixes: pulumi.StringArray{
* pulumi.String("10.2.1.0/24"),
* },
* EsrpAddresses: pulumi.StringArray{
* pulumi.String("198.51.100.4"),
* },
* OperatorAddresses: pulumi.StringArray{
* pulumi.String("198.51.100.2"),
* },
* },
* },
* AutoGeneratedDomainNameLabelScope: pulumi.String("SubscriptionReuse"),
* ApiBridge: pulumi.String(json0),
* EmergencyDialStrings: pulumi.StringArray{
* pulumi.String("911"),
* pulumi.String("933"),
* },
* OnPremMcpEnabled: pulumi.Bool(false),
* Tags: pulumi.StringMap{
* "key": pulumi.String("value"),
* },
* MicrosoftTeamsVoicemailPilotNumber: pulumi.String("1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.voice.ServicesCommunicationsGateway;
* import com.pulumi.azure.voice.ServicesCommunicationsGatewayArgs;
* import com.pulumi.azure.voice.inputs.ServicesCommunicationsGatewayServiceLocationArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleServicesCommunicationsGateway = new ServicesCommunicationsGateway("exampleServicesCommunicationsGateway", ServicesCommunicationsGatewayArgs.builder()
* .name("example-vcg")
* .location("West Europe")
* .resourceGroupName(example.name())
* .connectivity("PublicAddress")
* .codecs("PCMA")
* .e911Type("DirectToEsrp")
* .platforms(
* "OperatorConnect",
* "TeamsPhoneMobile")
* .serviceLocations(
* ServicesCommunicationsGatewayServiceLocationArgs.builder()
* .location("eastus")
* .allowedMediaSourceAddressPrefixes("10.1.2.0/24")
* .allowedSignalingSourceAddressPrefixes("10.1.1.0/24")
* .esrpAddresses("198.51.100.3")
* .operatorAddresses("198.51.100.1")
* .build(),
* ServicesCommunicationsGatewayServiceLocationArgs.builder()
* .location("eastus2")
* .allowedMediaSourceAddressPrefixes("10.2.2.0/24")
* .allowedSignalingSourceAddressPrefixes("10.2.1.0/24")
* .esrpAddresses("198.51.100.4")
* .operatorAddresses("198.51.100.2")
* .build())
* .autoGeneratedDomainNameLabelScope("SubscriptionReuse")
* .apiBridge(serializeJson(
* jsonObject(
* )))
* .emergencyDialStrings(
* "911",
* "933")
* .onPremMcpEnabled(false)
* .tags(Map.of("key", "value"))
* .microsoftTeamsVoicemailPilotNumber("1")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleServicesCommunicationsGateway:
* type: azure:voice:ServicesCommunicationsGateway
* name: example
* properties:
* name: example-vcg
* location: West Europe
* resourceGroupName: ${example.name}
* connectivity: PublicAddress
* codecs: PCMA
* e911Type: DirectToEsrp
* platforms:
* - OperatorConnect
* - TeamsPhoneMobile
* serviceLocations:
* - location: eastus
* allowedMediaSourceAddressPrefixes:
* - 10.1.2.0/24
* allowedSignalingSourceAddressPrefixes:
* - 10.1.1.0/24
* esrpAddresses:
* - 198.51.100.3
* operatorAddresses:
* - 198.51.100.1
* - location: eastus2
* allowedMediaSourceAddressPrefixes:
* - 10.2.2.0/24
* allowedSignalingSourceAddressPrefixes:
* - 10.2.1.0/24
* esrpAddresses:
* - 198.51.100.4
* operatorAddresses:
* - 198.51.100.2
* autoGeneratedDomainNameLabelScope: SubscriptionReuse
* apiBridge:
* fn::toJSON: {}
* emergencyDialStrings:
* - '911'
* - '933'
* onPremMcpEnabled: false
* tags:
* key: value
* microsoftTeamsVoicemailPilotNumber: '1'
* ```
*
* ## Import
* Voice Services Communications Gateways can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:voice/servicesCommunicationsGateway:ServicesCommunicationsGateway example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resourceGroup1/providers/Microsoft.VoiceServices/communicationsGateways/communicationsGateway1
* ```
* @property apiBridge Details of API bridge functionality, if required.
* @property autoGeneratedDomainNameLabelScope Specifies the scope at which the auto-generated domain name can be re-used. Possible values are `TenantReuse`, `SubscriptionReuse`, `ResourceGroupReuse` and `NoReuse` . Changing this forces a new resource to be created. Defaults to `TenantReuse`.
* @property codecs The voice codecs expected for communication with Teams. Possible values are `PCMA`, `PCMU`,`G722`,`G722_2`,`SILK_8` and `SILK_16`.
* @property connectivity How to connect back to the operator network, e.g. MAPS. Possible values is `PublicAddress`. Changing this forces a new Voice Services Communications Gateways to be created.
* @property e911Type How to handle 911 calls. Possible values are `Standard` and `DirectToEsrp`.
* @property emergencyDialStrings A list of dial strings used for emergency calling.
* @property location Specifies the Azure Region where the Voice Services Communications Gateways should exist. Changing this forces a new resource to be created.
* @property microsoftTeamsVoicemailPilotNumber This number is used in Teams Phone Mobile scenarios for access to the voicemail IVR from the native dialer.
* @property name Specifies the name which should be used for this Voice Services Communications Gateways. Changing this forces a new resource to be created.
* @property onPremMcpEnabled Whether an on-premises Mobile Control Point is in use.
* @property platforms The Voice Services Communications GatewaysAvailable supports platform types. Possible values are `OperatorConnect`, `TeamsPhoneMobile`.
* @property resourceGroupName Specifies the name of the Resource Group where the Voice Services Communications Gateways should exist. Changing this forces a new resource to be created.
* @property serviceLocations A `service_location` block as defined below.
* @property tags A mapping of tags which should be assigned to the Voice Services Communications Gateways.
*/
public data class ServicesCommunicationsGatewayArgs(
public val apiBridge: Output? = null,
public val autoGeneratedDomainNameLabelScope: Output? = null,
public val codecs: Output? = null,
public val connectivity: Output? = null,
public val e911Type: Output? = null,
public val emergencyDialStrings: Output>? = null,
public val location: Output? = null,
public val microsoftTeamsVoicemailPilotNumber: Output? = null,
public val name: Output? = null,
public val onPremMcpEnabled: Output? = null,
public val platforms: Output>? = null,
public val resourceGroupName: Output? = null,
public val serviceLocations: Output>? =
null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy