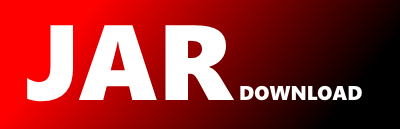
com.pulumi.azure.webpubsub.kotlin.CustomDomainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.webpubsub.kotlin
import com.pulumi.azure.webpubsub.CustomDomainArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages an Azure Web PubSub Custom Domain.
* ## Example Usage
*
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.webpubsub.Service;
* import com.pulumi.azure.webpubsub.ServiceArgs;
* import com.pulumi.azure.webpubsub.inputs.ServiceIdentityArgs;
* import com.pulumi.azure.keyvault.KeyVault;
* import com.pulumi.azure.keyvault.KeyVaultArgs;
* import com.pulumi.azure.keyvault.inputs.KeyVaultAccessPolicyArgs;
* import com.pulumi.azure.keyvault.Certificate;
* import com.pulumi.azure.keyvault.CertificateArgs;
* import com.pulumi.azure.keyvault.inputs.CertificateCertificateArgs;
* import com.pulumi.azure.webpubsub.CustomCertificate;
* import com.pulumi.azure.webpubsub.CustomCertificateArgs;
* import com.pulumi.azure.webpubsub.CustomDomain;
* import com.pulumi.azure.webpubsub.CustomDomainArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = CoreFunctions.getClientConfig();
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleService = new Service("exampleService", ServiceArgs.builder()
* .name("example-webpubsub")
* .location(testAzurermResourceGroup.location())
* .resourceGroupName(testAzurermResourceGroup.name())
* .sku(Map.ofEntries(
* Map.entry("name", "Premium_P1"),
* Map.entry("capacity", 1)
* ))
* .identity(ServiceIdentityArgs.builder()
* .type("SystemAssigned")
* .build())
* .build());
* var exampleKeyVault = new KeyVault("exampleKeyVault", KeyVaultArgs.builder()
* .name("examplekeyvault")
* .location(example.location())
* .resourceGroupName(example.name())
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .skuName("premium")
* .accessPolicies(
* KeyVaultAccessPolicyArgs.builder()
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .objectId(current.applyValue(getClientConfigResult -> getClientConfigResult.objectId()))
* .certificatePermissions(
* "Create",
* "Get",
* "List")
* .secretPermissions(
* "Get",
* "List")
* .build(),
* KeyVaultAccessPolicyArgs.builder()
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .objectId(testAzurermWebPubsub.identity()[0].principalId())
* .certificatePermissions(
* "Create",
* "Get",
* "List")
* .secretPermissions(
* "Get",
* "List")
* .build())
* .build());
* var exampleCertificate = new Certificate("exampleCertificate", CertificateArgs.builder()
* .name("imported-cert")
* .keyVaultId(exampleKeyVault.id())
* .certificate(CertificateCertificateArgs.builder()
* .contents(StdFunctions.filebase64(Filebase64Args.builder()
* .input("certificate-to-import.pfx")
* .build()).result())
* .password("")
* .build())
* .build());
* var test = new CustomCertificate("test", CustomCertificateArgs.builder()
* .name("example-cert")
* .webPubsubId(exampleService.id())
* .customCertificateId(exampleCertificate.id())
* .build());
* var testCustomDomain = new CustomDomain("testCustomDomain", CustomDomainArgs.builder()
* .name("example-domain")
* .domainName("tftest.com")
* .webPubsubId(testAzurermWebPubsub.id())
* .webPubsubCustomCertificateId(test.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleService:
* type: azure:webpubsub:Service
* name: example
* properties:
* name: example-webpubsub
* location: ${testAzurermResourceGroup.location}
* resourceGroupName: ${testAzurermResourceGroup.name}
* sku:
* - name: Premium_P1
* capacity: 1
* identity:
* type: SystemAssigned
* exampleKeyVault:
* type: azure:keyvault:KeyVault
* name: example
* properties:
* name: examplekeyvault
* location: ${example.location}
* resourceGroupName: ${example.name}
* tenantId: ${current.tenantId}
* skuName: premium
* accessPolicies:
* - tenantId: ${current.tenantId}
* objectId: ${current.objectId}
* certificatePermissions:
* - Create
* - Get
* - List
* secretPermissions:
* - Get
* - List
* - tenantId: ${current.tenantId}
* objectId: ${testAzurermWebPubsub.identity[0].principalId}
* certificatePermissions:
* - Create
* - Get
* - List
* secretPermissions:
* - Get
* - List
* exampleCertificate:
* type: azure:keyvault:Certificate
* name: example
* properties:
* name: imported-cert
* keyVaultId: ${exampleKeyVault.id}
* certificate:
* contents:
* fn::invoke:
* Function: std:filebase64
* Arguments:
* input: certificate-to-import.pfx
* Return: result
* password:
* test:
* type: azure:webpubsub:CustomCertificate
* properties:
* name: example-cert
* webPubsubId: ${exampleService.id}
* customCertificateId: ${exampleCertificate.id}
* testCustomDomain:
* type: azure:webpubsub:CustomDomain
* name: test
* properties:
* name: example-domain
* domainName: tftest.com
* webPubsubId: ${testAzurermWebPubsub.id}
* webPubsubCustomCertificateId: ${test.id}
* variables:
* current:
* fn::invoke:
* Function: azure:core:getClientConfig
* Arguments: {}
* ```
*
* ## Import
* Custom Domain for a Web PubSub service can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:webpubsub/customDomain:CustomDomain example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.SignalRService/webPubSub/webpubsub1/customDomains/customDomain1
* ```
* @property domainName Specifies the custom domain name of the Web PubSub Custom Domain. Changing this forces a new resource to be created.
* > **NOTE:** Please ensure the custom domain name is included in the Subject Alternative Names of the selected Web PubSub Custom Certificate.
* @property name Specifies the name of the Web PubSub Custom Domain. Changing this forces a new resource to be created.
* @property webPubsubCustomCertificateId Specifies the Web PubSub Custom Certificate ID of the Web PubSub Custom Domain. Changing this forces a new resource to be created.
* @property webPubsubId Specifies the Web PubSub ID of the Web PubSub Custom Domain. Changing this forces a new resource to be created.
*/
public data class CustomDomainArgs(
public val domainName: Output? = null,
public val name: Output? = null,
public val webPubsubCustomCertificateId: Output? = null,
public val webPubsubId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.webpubsub.CustomDomainArgs =
com.pulumi.azure.webpubsub.CustomDomainArgs.builder()
.domainName(domainName?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.webPubsubCustomCertificateId(webPubsubCustomCertificateId?.applyValue({ args0 -> args0 }))
.webPubsubId(webPubsubId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CustomDomainArgs].
*/
@PulumiTagMarker
public class CustomDomainArgsBuilder internal constructor() {
private var domainName: Output? = null
private var name: Output? = null
private var webPubsubCustomCertificateId: Output? = null
private var webPubsubId: Output? = null
/**
* @param value Specifies the custom domain name of the Web PubSub Custom Domain. Changing this forces a new resource to be created.
* > **NOTE:** Please ensure the custom domain name is included in the Subject Alternative Names of the selected Web PubSub Custom Certificate.
*/
@JvmName("dvebpisbrygrsvvt")
public suspend fun domainName(`value`: Output) {
this.domainName = value
}
/**
* @param value Specifies the name of the Web PubSub Custom Domain. Changing this forces a new resource to be created.
*/
@JvmName("negmnojrwoawkxve")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Specifies the Web PubSub Custom Certificate ID of the Web PubSub Custom Domain. Changing this forces a new resource to be created.
*/
@JvmName("ipaiquargndjwbkk")
public suspend fun webPubsubCustomCertificateId(`value`: Output) {
this.webPubsubCustomCertificateId = value
}
/**
* @param value Specifies the Web PubSub ID of the Web PubSub Custom Domain. Changing this forces a new resource to be created.
*/
@JvmName("vpjbihbmultpojgd")
public suspend fun webPubsubId(`value`: Output) {
this.webPubsubId = value
}
/**
* @param value Specifies the custom domain name of the Web PubSub Custom Domain. Changing this forces a new resource to be created.
* > **NOTE:** Please ensure the custom domain name is included in the Subject Alternative Names of the selected Web PubSub Custom Certificate.
*/
@JvmName("klrbupcmawfukxro")
public suspend fun domainName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.domainName = mapped
}
/**
* @param value Specifies the name of the Web PubSub Custom Domain. Changing this forces a new resource to be created.
*/
@JvmName("umtxgetkfwkjamqa")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Specifies the Web PubSub Custom Certificate ID of the Web PubSub Custom Domain. Changing this forces a new resource to be created.
*/
@JvmName("jwgjfebmkotooege")
public suspend fun webPubsubCustomCertificateId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.webPubsubCustomCertificateId = mapped
}
/**
* @param value Specifies the Web PubSub ID of the Web PubSub Custom Domain. Changing this forces a new resource to be created.
*/
@JvmName("aupltchkuqquxsph")
public suspend fun webPubsubId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.webPubsubId = mapped
}
internal fun build(): CustomDomainArgs = CustomDomainArgs(
domainName = domainName,
name = name,
webPubsubCustomCertificateId = webPubsubCustomCertificateId,
webPubsubId = webPubsubId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy