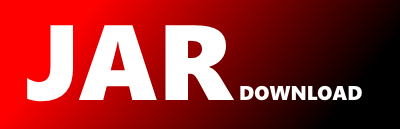
com.pulumi.azure.workloadssap.kotlin.SingleNodeVirtualInstanceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.workloadssap.kotlin
import com.pulumi.azure.workloadssap.SingleNodeVirtualInstanceArgs.builder
import com.pulumi.azure.workloadssap.kotlin.inputs.SingleNodeVirtualInstanceIdentityArgs
import com.pulumi.azure.workloadssap.kotlin.inputs.SingleNodeVirtualInstanceIdentityArgsBuilder
import com.pulumi.azure.workloadssap.kotlin.inputs.SingleNodeVirtualInstanceSingleServerConfigurationArgs
import com.pulumi.azure.workloadssap.kotlin.inputs.SingleNodeVirtualInstanceSingleServerConfigurationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages an SAP Single Node Virtual Instance with new SAP System.
* > **Note:** Before using this resource, it's required to submit the request of registering the Resource Provider with Azure CLI `az provider register --namespace "Microsoft.Workloads"`. The Resource Provider can take a while to register, you can check the status by running `az provider show --namespace "Microsoft.Workloads" --query "registrationState"`. Once this outputs "Registered" the Resource Provider is available for use.
* ## Import
* SAP Single Node Virtual Instances with new SAP Systems can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:workloadssap/singleNodeVirtualInstance:SingleNodeVirtualInstance example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.Workloads/sapVirtualInstances/vis1
* ```
* @property appLocation The Geo-Location where the SAP system is to be created. Changing this forces a new resource to be created.
* @property environment The environment type for the SAP Single Node Virtual Instance. Possible values are `NonProd` and `Prod`. Changing this forces a new resource to be created.
* @property identity An `identity` block as defined below.
* @property location The Azure Region where the SAP Single Node Virtual Instance should exist. Changing this forces a new resource to be created.
* @property managedResourceGroupName The name of the managed Resource Group for the SAP Single Node Virtual Instance. Changing this forces a new resource to be created.
* @property name Specifies the name of this SAP Single Node Virtual Instance. Changing this forces a new resource to be created.
* @property resourceGroupName The name of the Resource Group where the SAP Single Node Virtual Instance should exist. Changing this forces a new resource to be created.
* @property sapFqdn The fully qualified domain name for the SAP system. Changing this forces a new resource to be created.
* @property sapProduct The SAP Product type for the SAP Single Node Virtual Instance. Possible values are `ECC`, `Other` and `S4HANA`. Changing this forces a new resource to be created.
* @property singleServerConfiguration A `single_server_configuration` block as defined below. Changing this forces a new resource to be created.
* @property tags A mapping of tags which should be assigned to the SAP Single Node Virtual Instance.
*/
public data class SingleNodeVirtualInstanceArgs(
public val appLocation: Output? = null,
public val environment: Output? = null,
public val identity: Output? = null,
public val location: Output? = null,
public val managedResourceGroupName: Output? = null,
public val name: Output? = null,
public val resourceGroupName: Output? = null,
public val sapFqdn: Output? = null,
public val sapProduct: Output? = null,
public val singleServerConfiguration:
Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy