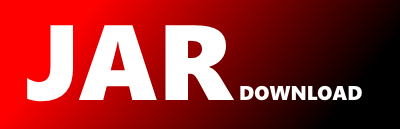
com.pulumi.azure.apimanagement.kotlin.ApimanagementFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.apimanagement.kotlin
import com.pulumi.azure.apimanagement.ApimanagementFunctions.getApiPlain
import com.pulumi.azure.apimanagement.ApimanagementFunctions.getApiVersionSetPlain
import com.pulumi.azure.apimanagement.ApimanagementFunctions.getGatewayHostNameConfigurationPlain
import com.pulumi.azure.apimanagement.ApimanagementFunctions.getGatewayPlain
import com.pulumi.azure.apimanagement.ApimanagementFunctions.getGroupPlain
import com.pulumi.azure.apimanagement.ApimanagementFunctions.getProductPlain
import com.pulumi.azure.apimanagement.ApimanagementFunctions.getServicePlain
import com.pulumi.azure.apimanagement.ApimanagementFunctions.getUserPlain
import com.pulumi.azure.apimanagement.kotlin.inputs.GetApiPlainArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.GetApiPlainArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.GetApiVersionSetPlainArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.GetApiVersionSetPlainArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.GetGatewayHostNameConfigurationPlainArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.GetGatewayHostNameConfigurationPlainArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.GetGatewayPlainArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.GetGatewayPlainArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.GetGroupPlainArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.GetGroupPlainArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.GetProductPlainArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.GetProductPlainArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.GetServicePlainArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.GetServicePlainArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.GetUserPlainArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.GetUserPlainArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.outputs.GetApiResult
import com.pulumi.azure.apimanagement.kotlin.outputs.GetApiVersionSetResult
import com.pulumi.azure.apimanagement.kotlin.outputs.GetGatewayHostNameConfigurationResult
import com.pulumi.azure.apimanagement.kotlin.outputs.GetGatewayResult
import com.pulumi.azure.apimanagement.kotlin.outputs.GetGroupResult
import com.pulumi.azure.apimanagement.kotlin.outputs.GetProductResult
import com.pulumi.azure.apimanagement.kotlin.outputs.GetServiceResult
import com.pulumi.azure.apimanagement.kotlin.outputs.GetUserResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import com.pulumi.azure.apimanagement.kotlin.outputs.GetApiResult.Companion.toKotlin as getApiResultToKotlin
import com.pulumi.azure.apimanagement.kotlin.outputs.GetApiVersionSetResult.Companion.toKotlin as getApiVersionSetResultToKotlin
import com.pulumi.azure.apimanagement.kotlin.outputs.GetGatewayHostNameConfigurationResult.Companion.toKotlin as getGatewayHostNameConfigurationResultToKotlin
import com.pulumi.azure.apimanagement.kotlin.outputs.GetGatewayResult.Companion.toKotlin as getGatewayResultToKotlin
import com.pulumi.azure.apimanagement.kotlin.outputs.GetGroupResult.Companion.toKotlin as getGroupResultToKotlin
import com.pulumi.azure.apimanagement.kotlin.outputs.GetProductResult.Companion.toKotlin as getProductResultToKotlin
import com.pulumi.azure.apimanagement.kotlin.outputs.GetServiceResult.Companion.toKotlin as getServiceResultToKotlin
import com.pulumi.azure.apimanagement.kotlin.outputs.GetUserResult.Companion.toKotlin as getUserResultToKotlin
public object ApimanagementFunctions {
/**
* Use this data source to access information about an existing API Management API.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.apimanagement.getApi({
* name: "search-api",
* apiManagementName: "search-api-management",
* resourceGroupName: "search-service",
* revision: "2",
* });
* export const apiManagementApiId = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.apimanagement.get_api(name="search-api",
* api_management_name="search-api-management",
* resource_group_name="search-service",
* revision="2")
* pulumi.export("apiManagementApiId", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ApiManagement.GetApi.Invoke(new()
* {
* Name = "search-api",
* ApiManagementName = "search-api-management",
* ResourceGroupName = "search-service",
* Revision = "2",
* });
* return new Dictionary
* {
* ["apiManagementApiId"] = example.Apply(getApiResult => getApiResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/apimanagement"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := apimanagement.LookupApi(ctx, &apimanagement.LookupApiArgs{
* Name: "search-api",
* ApiManagementName: "search-api-management",
* ResourceGroupName: "search-service",
* Revision: "2",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("apiManagementApiId", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.apimanagement.ApimanagementFunctions;
* import com.pulumi.azure.apimanagement.inputs.GetApiArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ApimanagementFunctions.getApi(GetApiArgs.builder()
* .name("search-api")
* .apiManagementName("search-api-management")
* .resourceGroupName("search-service")
* .revision("2")
* .build());
* ctx.export("apiManagementApiId", example.applyValue(getApiResult -> getApiResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:apimanagement:getApi
* Arguments:
* name: search-api
* apiManagementName: search-api-management
* resourceGroupName: search-service
* revision: '2'
* outputs:
* apiManagementApiId: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getApi.
* @return A collection of values returned by getApi.
*/
public suspend fun getApi(argument: GetApiPlainArgs): GetApiResult =
getApiResultToKotlin(getApiPlain(argument.toJava()).await())
/**
* @see [getApi].
* @param apiManagementName The name of the API Management Service in which the API Management API exists.
* @param name The name of the API Management API.
* @param resourceGroupName The Name of the Resource Group in which the API Management Service exists.
* @param revision The Revision of the API Management API.
* @return A collection of values returned by getApi.
*/
public suspend fun getApi(
apiManagementName: String,
name: String,
resourceGroupName: String,
revision: String,
): GetApiResult {
val argument = GetApiPlainArgs(
apiManagementName = apiManagementName,
name = name,
resourceGroupName = resourceGroupName,
revision = revision,
)
return getApiResultToKotlin(getApiPlain(argument.toJava()).await())
}
/**
* @see [getApi].
* @param argument Builder for [com.pulumi.azure.apimanagement.kotlin.inputs.GetApiPlainArgs].
* @return A collection of values returned by getApi.
*/
public suspend fun getApi(argument: suspend GetApiPlainArgsBuilder.() -> Unit): GetApiResult {
val builder = GetApiPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getApiResultToKotlin(getApiPlain(builtArgument.toJava()).await())
}
/**
* Uses this data source to access information about an API Version Set within an API Management Service.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.apimanagement.getApiVersionSet({
* resourceGroupName: "example-resources",
* apiManagementName: "example-api",
* name: "example-api-version-set",
* });
* export const apiManagementApiVersionSetId = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.apimanagement.get_api_version_set(resource_group_name="example-resources",
* api_management_name="example-api",
* name="example-api-version-set")
* pulumi.export("apiManagementApiVersionSetId", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ApiManagement.GetApiVersionSet.Invoke(new()
* {
* ResourceGroupName = "example-resources",
* ApiManagementName = "example-api",
* Name = "example-api-version-set",
* });
* return new Dictionary
* {
* ["apiManagementApiVersionSetId"] = example.Apply(getApiVersionSetResult => getApiVersionSetResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/apimanagement"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := apimanagement.LookupApiVersionSet(ctx, &apimanagement.LookupApiVersionSetArgs{
* ResourceGroupName: "example-resources",
* ApiManagementName: "example-api",
* Name: "example-api-version-set",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("apiManagementApiVersionSetId", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.apimanagement.ApimanagementFunctions;
* import com.pulumi.azure.apimanagement.inputs.GetApiVersionSetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ApimanagementFunctions.getApiVersionSet(GetApiVersionSetArgs.builder()
* .resourceGroupName("example-resources")
* .apiManagementName("example-api")
* .name("example-api-version-set")
* .build());
* ctx.export("apiManagementApiVersionSetId", example.applyValue(getApiVersionSetResult -> getApiVersionSetResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:apimanagement:getApiVersionSet
* Arguments:
* resourceGroupName: example-resources
* apiManagementName: example-api
* name: example-api-version-set
* outputs:
* apiManagementApiVersionSetId: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getApiVersionSet.
* @return A collection of values returned by getApiVersionSet.
*/
public suspend fun getApiVersionSet(argument: GetApiVersionSetPlainArgs): GetApiVersionSetResult =
getApiVersionSetResultToKotlin(getApiVersionSetPlain(argument.toJava()).await())
/**
* @see [getApiVersionSet].
* @param apiManagementName The name of the API Management Service where the API Version Set exists.
* @param name The name of the API Version Set.
* @param resourceGroupName The name of the Resource Group in which the parent API Management Service exists.
* @return A collection of values returned by getApiVersionSet.
*/
public suspend fun getApiVersionSet(
apiManagementName: String,
name: String,
resourceGroupName: String,
): GetApiVersionSetResult {
val argument = GetApiVersionSetPlainArgs(
apiManagementName = apiManagementName,
name = name,
resourceGroupName = resourceGroupName,
)
return getApiVersionSetResultToKotlin(getApiVersionSetPlain(argument.toJava()).await())
}
/**
* @see [getApiVersionSet].
* @param argument Builder for [com.pulumi.azure.apimanagement.kotlin.inputs.GetApiVersionSetPlainArgs].
* @return A collection of values returned by getApiVersionSet.
*/
public suspend fun getApiVersionSet(argument: suspend GetApiVersionSetPlainArgsBuilder.() -> Unit): GetApiVersionSetResult {
val builder = GetApiVersionSetPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getApiVersionSetResultToKotlin(getApiVersionSetPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing API Management Gateway.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.apimanagement.getService({
* name: "example-apim",
* resourceGroupName: "example-rg",
* });
* const exampleGetGateway = example.then(example => azure.apimanagement.getGateway({
* name: "example-api-gateway",
* apiManagementId: example.id,
* }));
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.apimanagement.get_service(name="example-apim",
* resource_group_name="example-rg")
* example_get_gateway = azure.apimanagement.get_gateway(name="example-api-gateway",
* api_management_id=example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ApiManagement.GetService.Invoke(new()
* {
* Name = "example-apim",
* ResourceGroupName = "example-rg",
* });
* var exampleGetGateway = Azure.ApiManagement.GetGateway.Invoke(new()
* {
* Name = "example-api-gateway",
* ApiManagementId = example.Apply(getServiceResult => getServiceResult.Id),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/apimanagement"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := apimanagement.LookupService(ctx, &apimanagement.LookupServiceArgs{
* Name: "example-apim",
* ResourceGroupName: "example-rg",
* }, nil)
* if err != nil {
* return err
* }
* _, err = apimanagement.LookupGateway(ctx, &apimanagement.LookupGatewayArgs{
* Name: "example-api-gateway",
* ApiManagementId: example.Id,
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.apimanagement.ApimanagementFunctions;
* import com.pulumi.azure.apimanagement.inputs.GetServiceArgs;
* import com.pulumi.azure.apimanagement.inputs.GetGatewayArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ApimanagementFunctions.getService(GetServiceArgs.builder()
* .name("example-apim")
* .resourceGroupName("example-rg")
* .build());
* final var exampleGetGateway = ApimanagementFunctions.getGateway(GetGatewayArgs.builder()
* .name("example-api-gateway")
* .apiManagementId(example.applyValue(getServiceResult -> getServiceResult.id()))
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:apimanagement:getService
* Arguments:
* name: example-apim
* resourceGroupName: example-rg
* exampleGetGateway:
* fn::invoke:
* Function: azure:apimanagement:getGateway
* Arguments:
* name: example-api-gateway
* apiManagementId: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getGateway.
* @return A collection of values returned by getGateway.
*/
public suspend fun getGateway(argument: GetGatewayPlainArgs): GetGatewayResult =
getGatewayResultToKotlin(getGatewayPlain(argument.toJava()).await())
/**
* @see [getGateway].
* @param apiManagementId The ID of the API Management Service in which the Gateway exists.
* @param name The name of the API Management Gateway.
* @return A collection of values returned by getGateway.
*/
public suspend fun getGateway(apiManagementId: String, name: String): GetGatewayResult {
val argument = GetGatewayPlainArgs(
apiManagementId = apiManagementId,
name = name,
)
return getGatewayResultToKotlin(getGatewayPlain(argument.toJava()).await())
}
/**
* @see [getGateway].
* @param argument Builder for [com.pulumi.azure.apimanagement.kotlin.inputs.GetGatewayPlainArgs].
* @return A collection of values returned by getGateway.
*/
public suspend fun getGateway(argument: suspend GetGatewayPlainArgsBuilder.() -> Unit): GetGatewayResult {
val builder = GetGatewayPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getGatewayResultToKotlin(getGatewayPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing API Management Gateway Host Configuration.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.apimanagement.getService({
* name: "example-apim",
* resourceGroupName: "example-resources",
* });
* const exampleGetGateway = azure.apimanagement.getGateway({
* name: "example-gateway",
* apiManagementId: main.id,
* });
* const exampleGetGatewayHostNameConfiguration = Promise.all([example, exampleGetGateway]).then(([example, exampleGetGateway]) => azure.apimanagement.getGatewayHostNameConfiguration({
* name: "example-host-configuration",
* apiManagementId: example.id,
* gatewayName: exampleGetGateway.name,
* }));
* export const hostName = exampleGetGatewayHostNameConfiguration.then(exampleGetGatewayHostNameConfiguration => exampleGetGatewayHostNameConfiguration.hostName);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.apimanagement.get_service(name="example-apim",
* resource_group_name="example-resources")
* example_get_gateway = azure.apimanagement.get_gateway(name="example-gateway",
* api_management_id=main["id"])
* example_get_gateway_host_name_configuration = azure.apimanagement.get_gateway_host_name_configuration(name="example-host-configuration",
* api_management_id=example.id,
* gateway_name=example_get_gateway.name)
* pulumi.export("hostName", example_get_gateway_host_name_configuration.host_name)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ApiManagement.GetService.Invoke(new()
* {
* Name = "example-apim",
* ResourceGroupName = "example-resources",
* });
* var exampleGetGateway = Azure.ApiManagement.GetGateway.Invoke(new()
* {
* Name = "example-gateway",
* ApiManagementId = main.Id,
* });
* var exampleGetGatewayHostNameConfiguration = Azure.ApiManagement.GetGatewayHostNameConfiguration.Invoke(new()
* {
* Name = "example-host-configuration",
* ApiManagementId = example.Apply(getServiceResult => getServiceResult.Id),
* GatewayName = exampleGetGateway.Apply(getGatewayResult => getGatewayResult.Name),
* });
* return new Dictionary
* {
* ["hostName"] = exampleGetGatewayHostNameConfiguration.Apply(getGatewayHostNameConfigurationResult => getGatewayHostNameConfigurationResult.HostName),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/apimanagement"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := apimanagement.LookupService(ctx, &apimanagement.LookupServiceArgs{
* Name: "example-apim",
* ResourceGroupName: "example-resources",
* }, nil)
* if err != nil {
* return err
* }
* exampleGetGateway, err := apimanagement.LookupGateway(ctx, &apimanagement.LookupGatewayArgs{
* Name: "example-gateway",
* ApiManagementId: main.Id,
* }, nil)
* if err != nil {
* return err
* }
* exampleGetGatewayHostNameConfiguration, err := apimanagement.LookupGatewayHostNameConfiguration(ctx, &apimanagement.LookupGatewayHostNameConfigurationArgs{
* Name: "example-host-configuration",
* ApiManagementId: example.Id,
* GatewayName: exampleGetGateway.Name,
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("hostName", exampleGetGatewayHostNameConfiguration.HostName)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.apimanagement.ApimanagementFunctions;
* import com.pulumi.azure.apimanagement.inputs.GetServiceArgs;
* import com.pulumi.azure.apimanagement.inputs.GetGatewayArgs;
* import com.pulumi.azure.apimanagement.inputs.GetGatewayHostNameConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ApimanagementFunctions.getService(GetServiceArgs.builder()
* .name("example-apim")
* .resourceGroupName("example-resources")
* .build());
* final var exampleGetGateway = ApimanagementFunctions.getGateway(GetGatewayArgs.builder()
* .name("example-gateway")
* .apiManagementId(main.id())
* .build());
* final var exampleGetGatewayHostNameConfiguration = ApimanagementFunctions.getGatewayHostNameConfiguration(GetGatewayHostNameConfigurationArgs.builder()
* .name("example-host-configuration")
* .apiManagementId(example.applyValue(getServiceResult -> getServiceResult.id()))
* .gatewayName(exampleGetGateway.applyValue(getGatewayResult -> getGatewayResult.name()))
* .build());
* ctx.export("hostName", exampleGetGatewayHostNameConfiguration.applyValue(getGatewayHostNameConfigurationResult -> getGatewayHostNameConfigurationResult.hostName()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:apimanagement:getService
* Arguments:
* name: example-apim
* resourceGroupName: example-resources
* exampleGetGateway:
* fn::invoke:
* Function: azure:apimanagement:getGateway
* Arguments:
* name: example-gateway
* apiManagementId: ${main.id}
* exampleGetGatewayHostNameConfiguration:
* fn::invoke:
* Function: azure:apimanagement:getGatewayHostNameConfiguration
* Arguments:
* name: example-host-configuration
* apiManagementId: ${example.id}
* gatewayName: ${exampleGetGateway.name}
* outputs:
* hostName: ${exampleGetGatewayHostNameConfiguration.hostName}
* ```
*
* @param argument A collection of arguments for invoking getGatewayHostNameConfiguration.
* @return A collection of values returned by getGatewayHostNameConfiguration.
*/
public suspend fun getGatewayHostNameConfiguration(argument: GetGatewayHostNameConfigurationPlainArgs): GetGatewayHostNameConfigurationResult =
getGatewayHostNameConfigurationResultToKotlin(getGatewayHostNameConfigurationPlain(argument.toJava()).await())
/**
* @see [getGatewayHostNameConfiguration].
* @param apiManagementId The ID of the API Management Service.
* @param gatewayName The name of the API Management Gateway.
* *
* @param name The name of the API Management Gateway Host Name Configuration.
* @return A collection of values returned by getGatewayHostNameConfiguration.
*/
public suspend fun getGatewayHostNameConfiguration(
apiManagementId: String,
gatewayName: String,
name: String,
): GetGatewayHostNameConfigurationResult {
val argument = GetGatewayHostNameConfigurationPlainArgs(
apiManagementId = apiManagementId,
gatewayName = gatewayName,
name = name,
)
return getGatewayHostNameConfigurationResultToKotlin(getGatewayHostNameConfigurationPlain(argument.toJava()).await())
}
/**
* @see [getGatewayHostNameConfiguration].
* @param argument Builder for [com.pulumi.azure.apimanagement.kotlin.inputs.GetGatewayHostNameConfigurationPlainArgs].
* @return A collection of values returned by getGatewayHostNameConfiguration.
*/
public suspend fun getGatewayHostNameConfiguration(argument: suspend GetGatewayHostNameConfigurationPlainArgsBuilder.() -> Unit): GetGatewayHostNameConfigurationResult {
val builder = GetGatewayHostNameConfigurationPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getGatewayHostNameConfigurationResultToKotlin(getGatewayHostNameConfigurationPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing API Management Group.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.apimanagement.getGroup({
* name: "my-group",
* apiManagementName: "example-apim",
* resourceGroupName: "search-service",
* });
* export const groupType = example.then(example => example.type);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.apimanagement.get_group(name="my-group",
* api_management_name="example-apim",
* resource_group_name="search-service")
* pulumi.export("groupType", example.type)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ApiManagement.GetGroup.Invoke(new()
* {
* Name = "my-group",
* ApiManagementName = "example-apim",
* ResourceGroupName = "search-service",
* });
* return new Dictionary
* {
* ["groupType"] = example.Apply(getGroupResult => getGroupResult.Type),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/apimanagement"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := apimanagement.LookupGroup(ctx, &apimanagement.LookupGroupArgs{
* Name: "my-group",
* ApiManagementName: "example-apim",
* ResourceGroupName: "search-service",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("groupType", example.Type)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.apimanagement.ApimanagementFunctions;
* import com.pulumi.azure.apimanagement.inputs.GetGroupArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ApimanagementFunctions.getGroup(GetGroupArgs.builder()
* .name("my-group")
* .apiManagementName("example-apim")
* .resourceGroupName("search-service")
* .build());
* ctx.export("groupType", example.applyValue(getGroupResult -> getGroupResult.type()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:apimanagement:getGroup
* Arguments:
* name: my-group
* apiManagementName: example-apim
* resourceGroupName: search-service
* outputs:
* groupType: ${example.type}
* ```
*
* @param argument A collection of arguments for invoking getGroup.
* @return A collection of values returned by getGroup.
*/
public suspend fun getGroup(argument: GetGroupPlainArgs): GetGroupResult =
getGroupResultToKotlin(getGroupPlain(argument.toJava()).await())
/**
* @see [getGroup].
* @param apiManagementName The Name of the API Management Service in which this Group exists.
* @param name The Name of the API Management Group.
* @param resourceGroupName The Name of the Resource Group in which the API Management Service exists.
* @return A collection of values returned by getGroup.
*/
public suspend fun getGroup(
apiManagementName: String,
name: String,
resourceGroupName: String,
): GetGroupResult {
val argument = GetGroupPlainArgs(
apiManagementName = apiManagementName,
name = name,
resourceGroupName = resourceGroupName,
)
return getGroupResultToKotlin(getGroupPlain(argument.toJava()).await())
}
/**
* @see [getGroup].
* @param argument Builder for [com.pulumi.azure.apimanagement.kotlin.inputs.GetGroupPlainArgs].
* @return A collection of values returned by getGroup.
*/
public suspend fun getGroup(argument: suspend GetGroupPlainArgsBuilder.() -> Unit): GetGroupResult {
val builder = GetGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getGroupResultToKotlin(getGroupPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing API Management Product.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.apimanagement.getProduct({
* productId: "my-product",
* apiManagementName: "example-apim",
* resourceGroupName: "search-service",
* });
* export const productTerms = example.then(example => example.terms);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.apimanagement.get_product(product_id="my-product",
* api_management_name="example-apim",
* resource_group_name="search-service")
* pulumi.export("productTerms", example.terms)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ApiManagement.GetProduct.Invoke(new()
* {
* ProductId = "my-product",
* ApiManagementName = "example-apim",
* ResourceGroupName = "search-service",
* });
* return new Dictionary
* {
* ["productTerms"] = example.Apply(getProductResult => getProductResult.Terms),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/apimanagement"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := apimanagement.LookupProduct(ctx, &apimanagement.LookupProductArgs{
* ProductId: "my-product",
* ApiManagementName: "example-apim",
* ResourceGroupName: "search-service",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("productTerms", example.Terms)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.apimanagement.ApimanagementFunctions;
* import com.pulumi.azure.apimanagement.inputs.GetProductArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ApimanagementFunctions.getProduct(GetProductArgs.builder()
* .productId("my-product")
* .apiManagementName("example-apim")
* .resourceGroupName("search-service")
* .build());
* ctx.export("productTerms", example.applyValue(getProductResult -> getProductResult.terms()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:apimanagement:getProduct
* Arguments:
* productId: my-product
* apiManagementName: example-apim
* resourceGroupName: search-service
* outputs:
* productTerms: ${example.terms}
* ```
*
* @param argument A collection of arguments for invoking getProduct.
* @return A collection of values returned by getProduct.
*/
public suspend fun getProduct(argument: GetProductPlainArgs): GetProductResult =
getProductResultToKotlin(getProductPlain(argument.toJava()).await())
/**
* @see [getProduct].
* @param apiManagementName The Name of the API Management Service in which this Product exists.
* @param productId The Identifier for the API Management Product.
* @param resourceGroupName The Name of the Resource Group in which the API Management Service exists.
* @return A collection of values returned by getProduct.
*/
public suspend fun getProduct(
apiManagementName: String,
productId: String,
resourceGroupName: String,
): GetProductResult {
val argument = GetProductPlainArgs(
apiManagementName = apiManagementName,
productId = productId,
resourceGroupName = resourceGroupName,
)
return getProductResultToKotlin(getProductPlain(argument.toJava()).await())
}
/**
* @see [getProduct].
* @param argument Builder for [com.pulumi.azure.apimanagement.kotlin.inputs.GetProductPlainArgs].
* @return A collection of values returned by getProduct.
*/
public suspend fun getProduct(argument: suspend GetProductPlainArgsBuilder.() -> Unit): GetProductResult {
val builder = GetProductPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getProductResultToKotlin(getProductPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing API Management Service.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.apimanagement.getService({
* name: "search-api",
* resourceGroupName: "search-service",
* });
* export const apiManagementId = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.apimanagement.get_service(name="search-api",
* resource_group_name="search-service")
* pulumi.export("apiManagementId", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ApiManagement.GetService.Invoke(new()
* {
* Name = "search-api",
* ResourceGroupName = "search-service",
* });
* return new Dictionary
* {
* ["apiManagementId"] = example.Apply(getServiceResult => getServiceResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/apimanagement"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := apimanagement.LookupService(ctx, &apimanagement.LookupServiceArgs{
* Name: "search-api",
* ResourceGroupName: "search-service",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("apiManagementId", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.apimanagement.ApimanagementFunctions;
* import com.pulumi.azure.apimanagement.inputs.GetServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ApimanagementFunctions.getService(GetServiceArgs.builder()
* .name("search-api")
* .resourceGroupName("search-service")
* .build());
* ctx.export("apiManagementId", example.applyValue(getServiceResult -> getServiceResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:apimanagement:getService
* Arguments:
* name: search-api
* resourceGroupName: search-service
* outputs:
* apiManagementId: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getService.
* @return A collection of values returned by getService.
*/
public suspend fun getService(argument: GetServicePlainArgs): GetServiceResult =
getServiceResultToKotlin(getServicePlain(argument.toJava()).await())
/**
* @see [getService].
* @param name The name of the API Management service.
* @param resourceGroupName The Name of the Resource Group in which the API Management Service exists.
* @param tags A mapping of tags assigned to the resource.
* @return A collection of values returned by getService.
*/
public suspend fun getService(
name: String,
resourceGroupName: String,
tags: Map? = null,
): GetServiceResult {
val argument = GetServicePlainArgs(
name = name,
resourceGroupName = resourceGroupName,
tags = tags,
)
return getServiceResultToKotlin(getServicePlain(argument.toJava()).await())
}
/**
* @see [getService].
* @param argument Builder for [com.pulumi.azure.apimanagement.kotlin.inputs.GetServicePlainArgs].
* @return A collection of values returned by getService.
*/
public suspend fun getService(argument: suspend GetServicePlainArgsBuilder.() -> Unit): GetServiceResult {
val builder = GetServicePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getServiceResultToKotlin(getServicePlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing API Management User.
* @param argument A collection of arguments for invoking getUser.
* @return A collection of values returned by getUser.
*/
public suspend fun getUser(argument: GetUserPlainArgs): GetUserResult =
getUserResultToKotlin(getUserPlain(argument.toJava()).await())
/**
* @see [getUser].
* @param apiManagementName The Name of the API Management Service in which this User exists.
* @param resourceGroupName The Name of the Resource Group in which the API Management Service exists.
* @param userId The Identifier for the User.
* @return A collection of values returned by getUser.
*/
public suspend fun getUser(
apiManagementName: String,
resourceGroupName: String,
userId: String,
): GetUserResult {
val argument = GetUserPlainArgs(
apiManagementName = apiManagementName,
resourceGroupName = resourceGroupName,
userId = userId,
)
return getUserResultToKotlin(getUserPlain(argument.toJava()).await())
}
/**
* @see [getUser].
* @param argument Builder for [com.pulumi.azure.apimanagement.kotlin.inputs.GetUserPlainArgs].
* @return A collection of values returned by getUser.
*/
public suspend fun getUser(argument: suspend GetUserPlainArgsBuilder.() -> Unit): GetUserResult {
val builder = GetUserPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getUserResultToKotlin(getUserPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy