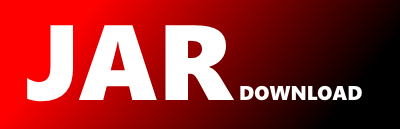
com.pulumi.azure.apimanagement.kotlin.BackendArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.apimanagement.kotlin
import com.pulumi.azure.apimanagement.BackendArgs.builder
import com.pulumi.azure.apimanagement.kotlin.inputs.BackendCredentialsArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.BackendCredentialsArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.BackendProxyArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.BackendProxyArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.BackendServiceFabricClusterArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.BackendServiceFabricClusterArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.BackendTlsArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.BackendTlsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Manages a backend within an API Management Service.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleService = new azure.apimanagement.Service("example", {
* name: "example-apim",
* location: example.location,
* resourceGroupName: example.name,
* publisherName: "My Company",
* publisherEmail: "[email protected]",
* skuName: "Developer_1",
* });
* const exampleBackend = new azure.apimanagement.Backend("example", {
* name: "example-backend",
* resourceGroupName: example.name,
* apiManagementName: exampleService.name,
* protocol: "http",
* url: "https://backend",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_service = azure.apimanagement.Service("example",
* name="example-apim",
* location=example.location,
* resource_group_name=example.name,
* publisher_name="My Company",
* publisher_email="[email protected]",
* sku_name="Developer_1")
* example_backend = azure.apimanagement.Backend("example",
* name="example-backend",
* resource_group_name=example.name,
* api_management_name=example_service.name,
* protocol="http",
* url="https://backend")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleService = new Azure.ApiManagement.Service("example", new()
* {
* Name = "example-apim",
* Location = example.Location,
* ResourceGroupName = example.Name,
* PublisherName = "My Company",
* PublisherEmail = "[email protected]",
* SkuName = "Developer_1",
* });
* var exampleBackend = new Azure.ApiManagement.Backend("example", new()
* {
* Name = "example-backend",
* ResourceGroupName = example.Name,
* ApiManagementName = exampleService.Name,
* Protocol = "http",
* Url = "https://backend",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/apimanagement"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleService, err := apimanagement.NewService(ctx, "example", &apimanagement.ServiceArgs{
* Name: pulumi.String("example-apim"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* PublisherName: pulumi.String("My Company"),
* PublisherEmail: pulumi.String("[email protected]"),
* SkuName: pulumi.String("Developer_1"),
* })
* if err != nil {
* return err
* }
* _, err = apimanagement.NewBackend(ctx, "example", &apimanagement.BackendArgs{
* Name: pulumi.String("example-backend"),
* ResourceGroupName: example.Name,
* ApiManagementName: exampleService.Name,
* Protocol: pulumi.String("http"),
* Url: pulumi.String("https://backend"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.apimanagement.Service;
* import com.pulumi.azure.apimanagement.ServiceArgs;
* import com.pulumi.azure.apimanagement.Backend;
* import com.pulumi.azure.apimanagement.BackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleService = new Service("exampleService", ServiceArgs.builder()
* .name("example-apim")
* .location(example.location())
* .resourceGroupName(example.name())
* .publisherName("My Company")
* .publisherEmail("[email protected]")
* .skuName("Developer_1")
* .build());
* var exampleBackend = new Backend("exampleBackend", BackendArgs.builder()
* .name("example-backend")
* .resourceGroupName(example.name())
* .apiManagementName(exampleService.name())
* .protocol("http")
* .url("https://backend")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleService:
* type: azure:apimanagement:Service
* name: example
* properties:
* name: example-apim
* location: ${example.location}
* resourceGroupName: ${example.name}
* publisherName: My Company
* publisherEmail: [email protected]
* skuName: Developer_1
* exampleBackend:
* type: azure:apimanagement:Backend
* name: example
* properties:
* name: example-backend
* resourceGroupName: ${example.name}
* apiManagementName: ${exampleService.name}
* protocol: http
* url: https://backend
* ```
*
* ## Import
* API Management backends can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:apimanagement/backend:Backend example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/mygroup1/providers/Microsoft.ApiManagement/service/instance1/backends/backend1
* ```
* @property apiManagementName The Name of the API Management Service where this backend should be created. Changing this forces a new resource to be created.
* @property credentials A `credentials` block as documented below.
* @property description The description of the backend.
* @property name The name of the API Management backend. Changing this forces a new resource to be created.
* @property protocol The protocol used by the backend host. Possible values are `http` or `soap`.
* @property proxy A `proxy` block as documented below.
* @property resourceGroupName The Name of the Resource Group where the API Management Service exists. Changing this forces a new resource to be created.
* @property resourceId The management URI of the backend host in an external system. This URI can be the ARM Resource ID of Logic Apps, Function Apps or API Apps, or the management endpoint of a Service Fabric cluster.
* @property serviceFabricCluster A `service_fabric_cluster` block as documented below.
* @property title The title of the backend.
* @property tls A `tls` block as documented below.
* @property url The URL of the backend host.
*/
public data class BackendArgs(
public val apiManagementName: Output? = null,
public val credentials: Output? = null,
public val description: Output? = null,
public val name: Output? = null,
public val protocol: Output? = null,
public val proxy: Output? = null,
public val resourceGroupName: Output? = null,
public val resourceId: Output? = null,
public val serviceFabricCluster: Output? = null,
public val title: Output? = null,
public val tls: Output? = null,
public val url: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.apimanagement.BackendArgs =
com.pulumi.azure.apimanagement.BackendArgs.builder()
.apiManagementName(apiManagementName?.applyValue({ args0 -> args0 }))
.credentials(credentials?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.protocol(protocol?.applyValue({ args0 -> args0 }))
.proxy(proxy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.resourceId(resourceId?.applyValue({ args0 -> args0 }))
.serviceFabricCluster(
serviceFabricCluster?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.title(title?.applyValue({ args0 -> args0 }))
.tls(tls?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.url(url?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackendArgs].
*/
@PulumiTagMarker
public class BackendArgsBuilder internal constructor() {
private var apiManagementName: Output? = null
private var credentials: Output? = null
private var description: Output? = null
private var name: Output? = null
private var protocol: Output? = null
private var proxy: Output? = null
private var resourceGroupName: Output? = null
private var resourceId: Output? = null
private var serviceFabricCluster: Output? = null
private var title: Output? = null
private var tls: Output? = null
private var url: Output? = null
/**
* @param value The Name of the API Management Service where this backend should be created. Changing this forces a new resource to be created.
*/
@JvmName("yshxucmxcgsvtpwl")
public suspend fun apiManagementName(`value`: Output) {
this.apiManagementName = value
}
/**
* @param value A `credentials` block as documented below.
*/
@JvmName("ngkreyqxivorrjtb")
public suspend fun credentials(`value`: Output) {
this.credentials = value
}
/**
* @param value The description of the backend.
*/
@JvmName("qjgteabifkqsuewk")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The name of the API Management backend. Changing this forces a new resource to be created.
*/
@JvmName("lokauhrasflwvwrw")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The protocol used by the backend host. Possible values are `http` or `soap`.
*/
@JvmName("uixjyhsfobbrkpql")
public suspend fun protocol(`value`: Output) {
this.protocol = value
}
/**
* @param value A `proxy` block as documented below.
*/
@JvmName("mwxianfljslmjcms")
public suspend fun proxy(`value`: Output) {
this.proxy = value
}
/**
* @param value The Name of the Resource Group where the API Management Service exists. Changing this forces a new resource to be created.
*/
@JvmName("qeqvqmbbdqiutrhf")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The management URI of the backend host in an external system. This URI can be the ARM Resource ID of Logic Apps, Function Apps or API Apps, or the management endpoint of a Service Fabric cluster.
*/
@JvmName("jqutgxslkesjuwkl")
public suspend fun resourceId(`value`: Output) {
this.resourceId = value
}
/**
* @param value A `service_fabric_cluster` block as documented below.
*/
@JvmName("nsxhatitsrmjpyoj")
public suspend fun serviceFabricCluster(`value`: Output) {
this.serviceFabricCluster = value
}
/**
* @param value The title of the backend.
*/
@JvmName("ksrydfvevunmtxmn")
public suspend fun title(`value`: Output) {
this.title = value
}
/**
* @param value A `tls` block as documented below.
*/
@JvmName("oscdmesoosassqim")
public suspend fun tls(`value`: Output) {
this.tls = value
}
/**
* @param value The URL of the backend host.
*/
@JvmName("pmkewxkqupuswdyk")
public suspend fun url(`value`: Output) {
this.url = value
}
/**
* @param value The Name of the API Management Service where this backend should be created. Changing this forces a new resource to be created.
*/
@JvmName("ydgixmvbfbmdngdt")
public suspend fun apiManagementName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiManagementName = mapped
}
/**
* @param value A `credentials` block as documented below.
*/
@JvmName("tnxniieuuligdawb")
public suspend fun credentials(`value`: BackendCredentialsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.credentials = mapped
}
/**
* @param argument A `credentials` block as documented below.
*/
@JvmName("bsngaoqjniayhuyt")
public suspend fun credentials(argument: suspend BackendCredentialsArgsBuilder.() -> Unit) {
val toBeMapped = BackendCredentialsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.credentials = mapped
}
/**
* @param value The description of the backend.
*/
@JvmName("ypdmclmqjclrfuln")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value The name of the API Management backend. Changing this forces a new resource to be created.
*/
@JvmName("nkiurrvbidhaqeqy")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The protocol used by the backend host. Possible values are `http` or `soap`.
*/
@JvmName("iqgfcublmonvlfcw")
public suspend fun protocol(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.protocol = mapped
}
/**
* @param value A `proxy` block as documented below.
*/
@JvmName("xrwtcgjdxkhurrka")
public suspend fun proxy(`value`: BackendProxyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.proxy = mapped
}
/**
* @param argument A `proxy` block as documented below.
*/
@JvmName("cutfvvfpdihstwvc")
public suspend fun proxy(argument: suspend BackendProxyArgsBuilder.() -> Unit) {
val toBeMapped = BackendProxyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.proxy = mapped
}
/**
* @param value The Name of the Resource Group where the API Management Service exists. Changing this forces a new resource to be created.
*/
@JvmName("xhtjindknedismtr")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value The management URI of the backend host in an external system. This URI can be the ARM Resource ID of Logic Apps, Function Apps or API Apps, or the management endpoint of a Service Fabric cluster.
*/
@JvmName("gcytnftwyrdheepu")
public suspend fun resourceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceId = mapped
}
/**
* @param value A `service_fabric_cluster` block as documented below.
*/
@JvmName("obvkvseoadkndkdt")
public suspend fun serviceFabricCluster(`value`: BackendServiceFabricClusterArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceFabricCluster = mapped
}
/**
* @param argument A `service_fabric_cluster` block as documented below.
*/
@JvmName("xibydugfsvgjmfsd")
public suspend fun serviceFabricCluster(argument: suspend BackendServiceFabricClusterArgsBuilder.() -> Unit) {
val toBeMapped = BackendServiceFabricClusterArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.serviceFabricCluster = mapped
}
/**
* @param value The title of the backend.
*/
@JvmName("mbfdjdduslltgdgi")
public suspend fun title(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.title = mapped
}
/**
* @param value A `tls` block as documented below.
*/
@JvmName("jxufedopncfnpddy")
public suspend fun tls(`value`: BackendTlsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tls = mapped
}
/**
* @param argument A `tls` block as documented below.
*/
@JvmName("plbjfqoqnmhqnvwh")
public suspend fun tls(argument: suspend BackendTlsArgsBuilder.() -> Unit) {
val toBeMapped = BackendTlsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.tls = mapped
}
/**
* @param value The URL of the backend host.
*/
@JvmName("vgjmofftchoprulp")
public suspend fun url(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.url = mapped
}
internal fun build(): BackendArgs = BackendArgs(
apiManagementName = apiManagementName,
credentials = credentials,
description = description,
name = name,
protocol = protocol,
proxy = proxy,
resourceGroupName = resourceGroupName,
resourceId = resourceId,
serviceFabricCluster = serviceFabricCluster,
title = title,
tls = tls,
url = url,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy