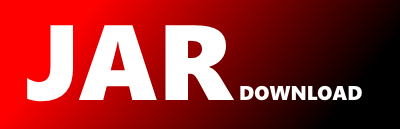
com.pulumi.azure.apimanagement.kotlin.ProductApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.apimanagement.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [ProductApi].
*/
@PulumiTagMarker
public class ProductApiResourceBuilder internal constructor() {
public var name: String? = null
public var args: ProductApiArgs = ProductApiArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ProductApiArgsBuilder.() -> Unit) {
val builder = ProductApiArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ProductApi {
val builtJavaResource = com.pulumi.azure.apimanagement.ProductApi(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ProductApi(builtJavaResource)
}
}
/**
* Manages an API Management API Assignment to a Product.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.apimanagement.getService({
* name: "example-api",
* resourceGroupName: "example-resources",
* });
* const exampleGetApi = Promise.all([example, example]).then(([example, example1]) => azure.apimanagement.getApi({
* name: "search-api",
* apiManagementName: example.name,
* resourceGroupName: example1.resourceGroupName,
* revision: "2",
* }));
* const exampleGetProduct = Promise.all([example, example]).then(([example, example1]) => azure.apimanagement.getProduct({
* productId: "my-product",
* apiManagementName: example.name,
* resourceGroupName: example1.resourceGroupName,
* }));
* const exampleProductApi = new azure.apimanagement.ProductApi("example", {
* apiName: exampleGetApi.then(exampleGetApi => exampleGetApi.name),
* productId: exampleGetProduct.then(exampleGetProduct => exampleGetProduct.productId),
* apiManagementName: example.then(example => example.name),
* resourceGroupName: example.then(example => example.resourceGroupName),
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.apimanagement.get_service(name="example-api",
* resource_group_name="example-resources")
* example_get_api = azure.apimanagement.get_api(name="search-api",
* api_management_name=example.name,
* resource_group_name=example.resource_group_name,
* revision="2")
* example_get_product = azure.apimanagement.get_product(product_id="my-product",
* api_management_name=example.name,
* resource_group_name=example.resource_group_name)
* example_product_api = azure.apimanagement.ProductApi("example",
* api_name=example_get_api.name,
* product_id=example_get_product.product_id,
* api_management_name=example.name,
* resource_group_name=example.resource_group_name)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ApiManagement.GetService.Invoke(new()
* {
* Name = "example-api",
* ResourceGroupName = "example-resources",
* });
* var exampleGetApi = Azure.ApiManagement.GetApi.Invoke(new()
* {
* Name = "search-api",
* ApiManagementName = example.Apply(getServiceResult => getServiceResult.Name),
* ResourceGroupName = example.Apply(getServiceResult => getServiceResult.ResourceGroupName),
* Revision = "2",
* });
* var exampleGetProduct = Azure.ApiManagement.GetProduct.Invoke(new()
* {
* ProductId = "my-product",
* ApiManagementName = example.Apply(getServiceResult => getServiceResult.Name),
* ResourceGroupName = example.Apply(getServiceResult => getServiceResult.ResourceGroupName),
* });
* var exampleProductApi = new Azure.ApiManagement.ProductApi("example", new()
* {
* ApiName = exampleGetApi.Apply(getApiResult => getApiResult.Name),
* ProductId = exampleGetProduct.Apply(getProductResult => getProductResult.ProductId),
* ApiManagementName = example.Apply(getServiceResult => getServiceResult.Name),
* ResourceGroupName = example.Apply(getServiceResult => getServiceResult.ResourceGroupName),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/apimanagement"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := apimanagement.LookupService(ctx, &apimanagement.LookupServiceArgs{
* Name: "example-api",
* ResourceGroupName: "example-resources",
* }, nil)
* if err != nil {
* return err
* }
* exampleGetApi, err := apimanagement.LookupApi(ctx, &apimanagement.LookupApiArgs{
* Name: "search-api",
* ApiManagementName: example.Name,
* ResourceGroupName: example.ResourceGroupName,
* Revision: "2",
* }, nil)
* if err != nil {
* return err
* }
* exampleGetProduct, err := apimanagement.LookupProduct(ctx, &apimanagement.LookupProductArgs{
* ProductId: "my-product",
* ApiManagementName: example.Name,
* ResourceGroupName: example.ResourceGroupName,
* }, nil)
* if err != nil {
* return err
* }
* _, err = apimanagement.NewProductApi(ctx, "example", &apimanagement.ProductApiArgs{
* ApiName: pulumi.String(exampleGetApi.Name),
* ProductId: pulumi.String(exampleGetProduct.ProductId),
* ApiManagementName: pulumi.String(example.Name),
* ResourceGroupName: pulumi.String(example.ResourceGroupName),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.apimanagement.ApimanagementFunctions;
* import com.pulumi.azure.apimanagement.inputs.GetServiceArgs;
* import com.pulumi.azure.apimanagement.inputs.GetApiArgs;
* import com.pulumi.azure.apimanagement.inputs.GetProductArgs;
* import com.pulumi.azure.apimanagement.ProductApi;
* import com.pulumi.azure.apimanagement.ProductApiArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ApimanagementFunctions.getService(GetServiceArgs.builder()
* .name("example-api")
* .resourceGroupName("example-resources")
* .build());
* final var exampleGetApi = ApimanagementFunctions.getApi(GetApiArgs.builder()
* .name("search-api")
* .apiManagementName(example.applyValue(getServiceResult -> getServiceResult.name()))
* .resourceGroupName(example.applyValue(getServiceResult -> getServiceResult.resourceGroupName()))
* .revision("2")
* .build());
* final var exampleGetProduct = ApimanagementFunctions.getProduct(GetProductArgs.builder()
* .productId("my-product")
* .apiManagementName(example.applyValue(getServiceResult -> getServiceResult.name()))
* .resourceGroupName(example.applyValue(getServiceResult -> getServiceResult.resourceGroupName()))
* .build());
* var exampleProductApi = new ProductApi("exampleProductApi", ProductApiArgs.builder()
* .apiName(exampleGetApi.applyValue(getApiResult -> getApiResult.name()))
* .productId(exampleGetProduct.applyValue(getProductResult -> getProductResult.productId()))
* .apiManagementName(example.applyValue(getServiceResult -> getServiceResult.name()))
* .resourceGroupName(example.applyValue(getServiceResult -> getServiceResult.resourceGroupName()))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleProductApi:
* type: azure:apimanagement:ProductApi
* name: example
* properties:
* apiName: ${exampleGetApi.name}
* productId: ${exampleGetProduct.productId}
* apiManagementName: ${example.name}
* resourceGroupName: ${example.resourceGroupName}
* variables:
* example:
* fn::invoke:
* Function: azure:apimanagement:getService
* Arguments:
* name: example-api
* resourceGroupName: example-resources
* exampleGetApi:
* fn::invoke:
* Function: azure:apimanagement:getApi
* Arguments:
* name: search-api
* apiManagementName: ${example.name}
* resourceGroupName: ${example.resourceGroupName}
* revision: '2'
* exampleGetProduct:
* fn::invoke:
* Function: azure:apimanagement:getProduct
* Arguments:
* productId: my-product
* apiManagementName: ${example.name}
* resourceGroupName: ${example.resourceGroupName}
* ```
*
* ## Import
* API Management Product API's can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:apimanagement/productApi:ProductApi example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.ApiManagement/service/service1/products/exampleId/apis/apiId
* ```
*/
public class ProductApi internal constructor(
override val javaResource: com.pulumi.azure.apimanagement.ProductApi,
) : KotlinCustomResource(javaResource, ProductApiMapper) {
/**
* The name of the API Management Service. Changing this forces a new resource to be created.
*/
public val apiManagementName: Output
get() = javaResource.apiManagementName().applyValue({ args0 -> args0 })
/**
* The Name of the API Management API within the API Management Service. Changing this forces a new resource to be created.
*/
public val apiName: Output
get() = javaResource.apiName().applyValue({ args0 -> args0 })
/**
* The ID of the API Management Product within the API Management Service. Changing this forces a new resource to be created.
*/
public val productId: Output
get() = javaResource.productId().applyValue({ args0 -> args0 })
/**
* The name of the Resource Group in which the API Management Service exists. Changing this forces a new resource to be created.
*/
public val resourceGroupName: Output
get() = javaResource.resourceGroupName().applyValue({ args0 -> args0 })
}
public object ProductApiMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azure.apimanagement.ProductApi::class == javaResource::class
override fun map(javaResource: Resource): ProductApi = ProductApi(
javaResource as
com.pulumi.azure.apimanagement.ProductApi,
)
}
/**
* @see [ProductApi].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ProductApi].
*/
public suspend fun productApi(name: String, block: suspend ProductApiResourceBuilder.() -> Unit): ProductApi {
val builder = ProductApiResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ProductApi].
* @param name The _unique_ name of the resulting resource.
*/
public fun productApi(name: String): ProductApi {
val builder = ProductApiResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy