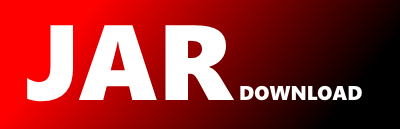
com.pulumi.azure.apimanagement.kotlin.SubscriptionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.apimanagement.kotlin
import com.pulumi.azure.apimanagement.SubscriptionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages a Subscription within a API Management Service.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.apimanagement.getService({
* name: "example-apim",
* resourceGroupName: "example-resources",
* });
* const exampleGetProduct = Promise.all([example, example]).then(([example, example1]) => azure.apimanagement.getProduct({
* productId: "00000000-0000-0000-0000-000000000000",
* apiManagementName: example.name,
* resourceGroupName: example1.resourceGroupName,
* }));
* const exampleGetUser = Promise.all([example, example]).then(([example, example1]) => azure.apimanagement.getUser({
* userId: "11111111-1111-1111-1111-111111111111",
* apiManagementName: example.name,
* resourceGroupName: example1.resourceGroupName,
* }));
* const exampleSubscription = new azure.apimanagement.Subscription("example", {
* apiManagementName: example.then(example => example.name),
* resourceGroupName: example.then(example => example.resourceGroupName),
* userId: exampleGetUser.then(exampleGetUser => exampleGetUser.id),
* productId: exampleGetProduct.then(exampleGetProduct => exampleGetProduct.id),
* displayName: "Parser API",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.apimanagement.get_service(name="example-apim",
* resource_group_name="example-resources")
* example_get_product = azure.apimanagement.get_product(product_id="00000000-0000-0000-0000-000000000000",
* api_management_name=example.name,
* resource_group_name=example.resource_group_name)
* example_get_user = azure.apimanagement.get_user(user_id="11111111-1111-1111-1111-111111111111",
* api_management_name=example.name,
* resource_group_name=example.resource_group_name)
* example_subscription = azure.apimanagement.Subscription("example",
* api_management_name=example.name,
* resource_group_name=example.resource_group_name,
* user_id=example_get_user.id,
* product_id=example_get_product.id,
* display_name="Parser API")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ApiManagement.GetService.Invoke(new()
* {
* Name = "example-apim",
* ResourceGroupName = "example-resources",
* });
* var exampleGetProduct = Azure.ApiManagement.GetProduct.Invoke(new()
* {
* ProductId = "00000000-0000-0000-0000-000000000000",
* ApiManagementName = example.Apply(getServiceResult => getServiceResult.Name),
* ResourceGroupName = example.Apply(getServiceResult => getServiceResult.ResourceGroupName),
* });
* var exampleGetUser = Azure.ApiManagement.GetUser.Invoke(new()
* {
* UserId = "11111111-1111-1111-1111-111111111111",
* ApiManagementName = example.Apply(getServiceResult => getServiceResult.Name),
* ResourceGroupName = example.Apply(getServiceResult => getServiceResult.ResourceGroupName),
* });
* var exampleSubscription = new Azure.ApiManagement.Subscription("example", new()
* {
* ApiManagementName = example.Apply(getServiceResult => getServiceResult.Name),
* ResourceGroupName = example.Apply(getServiceResult => getServiceResult.ResourceGroupName),
* UserId = exampleGetUser.Apply(getUserResult => getUserResult.Id),
* ProductId = exampleGetProduct.Apply(getProductResult => getProductResult.Id),
* DisplayName = "Parser API",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/apimanagement"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := apimanagement.LookupService(ctx, &apimanagement.LookupServiceArgs{
* Name: "example-apim",
* ResourceGroupName: "example-resources",
* }, nil)
* if err != nil {
* return err
* }
* exampleGetProduct, err := apimanagement.LookupProduct(ctx, &apimanagement.LookupProductArgs{
* ProductId: "00000000-0000-0000-0000-000000000000",
* ApiManagementName: example.Name,
* ResourceGroupName: example.ResourceGroupName,
* }, nil)
* if err != nil {
* return err
* }
* exampleGetUser, err := apimanagement.LookupUser(ctx, &apimanagement.LookupUserArgs{
* UserId: "11111111-1111-1111-1111-111111111111",
* ApiManagementName: example.Name,
* ResourceGroupName: example.ResourceGroupName,
* }, nil)
* if err != nil {
* return err
* }
* _, err = apimanagement.NewSubscription(ctx, "example", &apimanagement.SubscriptionArgs{
* ApiManagementName: pulumi.String(example.Name),
* ResourceGroupName: pulumi.String(example.ResourceGroupName),
* UserId: pulumi.String(exampleGetUser.Id),
* ProductId: pulumi.String(exampleGetProduct.Id),
* DisplayName: pulumi.String("Parser API"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.apimanagement.ApimanagementFunctions;
* import com.pulumi.azure.apimanagement.inputs.GetServiceArgs;
* import com.pulumi.azure.apimanagement.inputs.GetProductArgs;
* import com.pulumi.azure.apimanagement.inputs.GetUserArgs;
* import com.pulumi.azure.apimanagement.Subscription;
* import com.pulumi.azure.apimanagement.SubscriptionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ApimanagementFunctions.getService(GetServiceArgs.builder()
* .name("example-apim")
* .resourceGroupName("example-resources")
* .build());
* final var exampleGetProduct = ApimanagementFunctions.getProduct(GetProductArgs.builder()
* .productId("00000000-0000-0000-0000-000000000000")
* .apiManagementName(example.applyValue(getServiceResult -> getServiceResult.name()))
* .resourceGroupName(example.applyValue(getServiceResult -> getServiceResult.resourceGroupName()))
* .build());
* final var exampleGetUser = ApimanagementFunctions.getUser(GetUserArgs.builder()
* .userId("11111111-1111-1111-1111-111111111111")
* .apiManagementName(example.applyValue(getServiceResult -> getServiceResult.name()))
* .resourceGroupName(example.applyValue(getServiceResult -> getServiceResult.resourceGroupName()))
* .build());
* var exampleSubscription = new Subscription("exampleSubscription", SubscriptionArgs.builder()
* .apiManagementName(example.applyValue(getServiceResult -> getServiceResult.name()))
* .resourceGroupName(example.applyValue(getServiceResult -> getServiceResult.resourceGroupName()))
* .userId(exampleGetUser.applyValue(getUserResult -> getUserResult.id()))
* .productId(exampleGetProduct.applyValue(getProductResult -> getProductResult.id()))
* .displayName("Parser API")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleSubscription:
* type: azure:apimanagement:Subscription
* name: example
* properties:
* apiManagementName: ${example.name}
* resourceGroupName: ${example.resourceGroupName}
* userId: ${exampleGetUser.id}
* productId: ${exampleGetProduct.id}
* displayName: Parser API
* variables:
* example:
* fn::invoke:
* Function: azure:apimanagement:getService
* Arguments:
* name: example-apim
* resourceGroupName: example-resources
* exampleGetProduct:
* fn::invoke:
* Function: azure:apimanagement:getProduct
* Arguments:
* productId: 00000000-0000-0000-0000-000000000000
* apiManagementName: ${example.name}
* resourceGroupName: ${example.resourceGroupName}
* exampleGetUser:
* fn::invoke:
* Function: azure:apimanagement:getUser
* Arguments:
* userId: 11111111-1111-1111-1111-111111111111
* apiManagementName: ${example.name}
* resourceGroupName: ${example.resourceGroupName}
* ```
*
* ## Import
* API Management Subscriptions can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:apimanagement/subscription:Subscription example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/example-resources/providers/Microsoft.ApiManagement/service/example-apim/subscriptions/subscription-name
* ```
* @property allowTracing Determines whether tracing can be enabled. Defaults to `true`.
* @property apiId The ID of the API which should be assigned to this Subscription. Changing this forces a new resource to be created.
* > **Info:** Only one of `product_id` and `api_id` can be set. If both are missing `/apis` scope is used for the subscription and all apis are accessible.
* @property apiManagementName The name of the API Management Service where this Subscription should be created. Changing this forces a new resource to be created.
* @property displayName The display name of this Subscription.
* @property primaryKey The primary subscription key to use for the subscription.
* @property productId The ID of the Product which should be assigned to this Subscription. Changing this forces a new resource to be created.
* > **Info:** Only one of `product_id` and `api_id` can be set. If both are missing `all_apis` scope is used for the subscription.
* @property resourceGroupName The name of the Resource Group in which the API Management Service exists. Changing this forces a new resource to be created.
* @property secondaryKey The secondary subscription key to use for the subscription.
* @property state The state of this Subscription. Possible values are `active`, `cancelled`, `expired`, `rejected`, `submitted` and `suspended`. Defaults to `submitted`.
* @property subscriptionId An Identifier which should used as the ID of this Subscription. If not specified a new Subscription ID will be generated. Changing this forces a new resource to be created.
* @property userId The ID of the User which should be assigned to this Subscription. Changing this forces a new resource to be created.
*/
public data class SubscriptionArgs(
public val allowTracing: Output? = null,
public val apiId: Output? = null,
public val apiManagementName: Output? = null,
public val displayName: Output? = null,
public val primaryKey: Output? = null,
public val productId: Output? = null,
public val resourceGroupName: Output? = null,
public val secondaryKey: Output? = null,
public val state: Output? = null,
public val subscriptionId: Output? = null,
public val userId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.apimanagement.SubscriptionArgs =
com.pulumi.azure.apimanagement.SubscriptionArgs.builder()
.allowTracing(allowTracing?.applyValue({ args0 -> args0 }))
.apiId(apiId?.applyValue({ args0 -> args0 }))
.apiManagementName(apiManagementName?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.primaryKey(primaryKey?.applyValue({ args0 -> args0 }))
.productId(productId?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.secondaryKey(secondaryKey?.applyValue({ args0 -> args0 }))
.state(state?.applyValue({ args0 -> args0 }))
.subscriptionId(subscriptionId?.applyValue({ args0 -> args0 }))
.userId(userId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SubscriptionArgs].
*/
@PulumiTagMarker
public class SubscriptionArgsBuilder internal constructor() {
private var allowTracing: Output? = null
private var apiId: Output? = null
private var apiManagementName: Output? = null
private var displayName: Output? = null
private var primaryKey: Output? = null
private var productId: Output? = null
private var resourceGroupName: Output? = null
private var secondaryKey: Output? = null
private var state: Output? = null
private var subscriptionId: Output? = null
private var userId: Output? = null
/**
* @param value Determines whether tracing can be enabled. Defaults to `true`.
*/
@JvmName("aethsthmsbgbagbp")
public suspend fun allowTracing(`value`: Output) {
this.allowTracing = value
}
/**
* @param value The ID of the API which should be assigned to this Subscription. Changing this forces a new resource to be created.
* > **Info:** Only one of `product_id` and `api_id` can be set. If both are missing `/apis` scope is used for the subscription and all apis are accessible.
*/
@JvmName("bbbasqkxjqdlpklt")
public suspend fun apiId(`value`: Output) {
this.apiId = value
}
/**
* @param value The name of the API Management Service where this Subscription should be created. Changing this forces a new resource to be created.
*/
@JvmName("mmdjemhmlaqxgxjr")
public suspend fun apiManagementName(`value`: Output) {
this.apiManagementName = value
}
/**
* @param value The display name of this Subscription.
*/
@JvmName("wlmfcdqiapwwonjv")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value The primary subscription key to use for the subscription.
*/
@JvmName("ctwtfilhhdcpismh")
public suspend fun primaryKey(`value`: Output) {
this.primaryKey = value
}
/**
* @param value The ID of the Product which should be assigned to this Subscription. Changing this forces a new resource to be created.
* > **Info:** Only one of `product_id` and `api_id` can be set. If both are missing `all_apis` scope is used for the subscription.
*/
@JvmName("jnpiebdjrogwbbma")
public suspend fun productId(`value`: Output) {
this.productId = value
}
/**
* @param value The name of the Resource Group in which the API Management Service exists. Changing this forces a new resource to be created.
*/
@JvmName("tesbqjwrkxmwqjtu")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The secondary subscription key to use for the subscription.
*/
@JvmName("ekpkfhmayssdaacr")
public suspend fun secondaryKey(`value`: Output) {
this.secondaryKey = value
}
/**
* @param value The state of this Subscription. Possible values are `active`, `cancelled`, `expired`, `rejected`, `submitted` and `suspended`. Defaults to `submitted`.
*/
@JvmName("yswsdsaluacjqiqa")
public suspend fun state(`value`: Output) {
this.state = value
}
/**
* @param value An Identifier which should used as the ID of this Subscription. If not specified a new Subscription ID will be generated. Changing this forces a new resource to be created.
*/
@JvmName("vslllcjxspvxsvso")
public suspend fun subscriptionId(`value`: Output) {
this.subscriptionId = value
}
/**
* @param value The ID of the User which should be assigned to this Subscription. Changing this forces a new resource to be created.
*/
@JvmName("ftbibvynmpqmnvkh")
public suspend fun userId(`value`: Output) {
this.userId = value
}
/**
* @param value Determines whether tracing can be enabled. Defaults to `true`.
*/
@JvmName("xfixcmxarepmuspd")
public suspend fun allowTracing(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowTracing = mapped
}
/**
* @param value The ID of the API which should be assigned to this Subscription. Changing this forces a new resource to be created.
* > **Info:** Only one of `product_id` and `api_id` can be set. If both are missing `/apis` scope is used for the subscription and all apis are accessible.
*/
@JvmName("vylanidawhohbjmm")
public suspend fun apiId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiId = mapped
}
/**
* @param value The name of the API Management Service where this Subscription should be created. Changing this forces a new resource to be created.
*/
@JvmName("vdaplgbmyxjqfeub")
public suspend fun apiManagementName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiManagementName = mapped
}
/**
* @param value The display name of this Subscription.
*/
@JvmName("wwrwoeskfsbtuqda")
public suspend fun displayName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayName = mapped
}
/**
* @param value The primary subscription key to use for the subscription.
*/
@JvmName("omhqrnhxoffcablk")
public suspend fun primaryKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.primaryKey = mapped
}
/**
* @param value The ID of the Product which should be assigned to this Subscription. Changing this forces a new resource to be created.
* > **Info:** Only one of `product_id` and `api_id` can be set. If both are missing `all_apis` scope is used for the subscription.
*/
@JvmName("jlfqqapolerjrmws")
public suspend fun productId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.productId = mapped
}
/**
* @param value The name of the Resource Group in which the API Management Service exists. Changing this forces a new resource to be created.
*/
@JvmName("kvryncvhlwmhwttr")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value The secondary subscription key to use for the subscription.
*/
@JvmName("ltcqftdbhjnqcqml")
public suspend fun secondaryKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secondaryKey = mapped
}
/**
* @param value The state of this Subscription. Possible values are `active`, `cancelled`, `expired`, `rejected`, `submitted` and `suspended`. Defaults to `submitted`.
*/
@JvmName("tvxaaeaxigpslaof")
public suspend fun state(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.state = mapped
}
/**
* @param value An Identifier which should used as the ID of this Subscription. If not specified a new Subscription ID will be generated. Changing this forces a new resource to be created.
*/
@JvmName("xhikdoqyfywmgifn")
public suspend fun subscriptionId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subscriptionId = mapped
}
/**
* @param value The ID of the User which should be assigned to this Subscription. Changing this forces a new resource to be created.
*/
@JvmName("pturmsubjajdmhrj")
public suspend fun userId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.userId = mapped
}
internal fun build(): SubscriptionArgs = SubscriptionArgs(
allowTracing = allowTracing,
apiId = apiId,
apiManagementName = apiManagementName,
displayName = displayName,
primaryKey = primaryKey,
productId = productId,
resourceGroupName = resourceGroupName,
secondaryKey = secondaryKey,
state = state,
subscriptionId = subscriptionId,
userId = userId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy