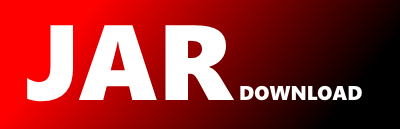
com.pulumi.azure.apimanagement.kotlin.inputs.ApiOperationRequestRepresentationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.apimanagement.kotlin.inputs
import com.pulumi.azure.apimanagement.inputs.ApiOperationRequestRepresentationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property contentType The Content Type of this representation, such as `application/json`.
* @property examples One or more `example` blocks as defined above.
* @property formParameters One or more `form_parameter` block as defined above.
* > **NOTE:** This is Required when `content_type` is set to `application/x-www-form-urlencoded` or `multipart/form-data`.
* @property schemaId The ID of an API Management Schema which represents this Response.
* > **NOTE:** This can only be specified when `content_type` is not set to `application/x-www-form-urlencoded` or `multipart/form-data`.
* @property typeName The Type Name defined by the Schema.
* > **NOTE:** This can only be specified when `content_type` is not set to `application/x-www-form-urlencoded` or `multipart/form-data`.
*/
public data class ApiOperationRequestRepresentationArgs(
public val contentType: Output,
public val examples: Output>? = null,
public val formParameters: Output>? =
null,
public val schemaId: Output? = null,
public val typeName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.apimanagement.inputs.ApiOperationRequestRepresentationArgs = com.pulumi.azure.apimanagement.inputs.ApiOperationRequestRepresentationArgs.builder()
.contentType(contentType.applyValue({ args0 -> args0 }))
.examples(
examples?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.formParameters(
formParameters?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.schemaId(schemaId?.applyValue({ args0 -> args0 }))
.typeName(typeName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ApiOperationRequestRepresentationArgs].
*/
@PulumiTagMarker
public class ApiOperationRequestRepresentationArgsBuilder internal constructor() {
private var contentType: Output? = null
private var examples: Output>? = null
private var formParameters: Output>? =
null
private var schemaId: Output? = null
private var typeName: Output? = null
/**
* @param value The Content Type of this representation, such as `application/json`.
*/
@JvmName("bieddbhbpbvwulwx")
public suspend fun contentType(`value`: Output) {
this.contentType = value
}
/**
* @param value One or more `example` blocks as defined above.
*/
@JvmName("vqoaaqwuahahirbx")
public suspend fun examples(`value`: Output>) {
this.examples = value
}
@JvmName("dqggapsuxxdoicmd")
public suspend fun examples(vararg values: Output) {
this.examples = Output.all(values.asList())
}
/**
* @param values One or more `example` blocks as defined above.
*/
@JvmName("cqbtfeigxmvnloah")
public suspend fun examples(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy