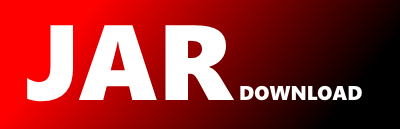
com.pulumi.azure.apimanagement.kotlin.inputs.ServiceSecurityArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.apimanagement.kotlin.inputs
import com.pulumi.azure.apimanagement.inputs.ServiceSecurityArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property enableBackendSsl30 Should SSL 3.0 be enabled on the backend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Backend.Protocols.Ssl30` field
* @property enableBackendTls10 Should TLS 1.0 be enabled on the backend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Backend.Protocols.Tls10` field
* @property enableBackendTls11 Should TLS 1.1 be enabled on the backend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Backend.Protocols.Tls11` field
* @property enableFrontendSsl30 Should SSL 3.0 be enabled on the frontend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Protocols.Ssl30` field
* @property enableFrontendTls10 Should TLS 1.0 be enabled on the frontend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Protocols.Tls10` field
* @property enableFrontendTls11 Should TLS 1.1 be enabled on the frontend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Protocols.Tls11` field
* @property tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled Should the `TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA` field
* @property tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled Should the `TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA` field
* @property tlsEcdheRsaWithAes128CbcShaCiphersEnabled Should the `TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA` field
* @property tlsEcdheRsaWithAes256CbcShaCiphersEnabled Should the `TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA` field
* @property tlsRsaWithAes128CbcSha256CiphersEnabled Should the `TLS_RSA_WITH_AES_128_CBC_SHA256` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_128_CBC_SHA256` field
* @property tlsRsaWithAes128CbcShaCiphersEnabled Should the `TLS_RSA_WITH_AES_128_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_128_CBC_SHA` field
* @property tlsRsaWithAes128GcmSha256CiphersEnabled Should the `TLS_RSA_WITH_AES_128_GCM_SHA256` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_128_GCM_SHA256` field
* @property tlsRsaWithAes256CbcSha256CiphersEnabled Should the `TLS_RSA_WITH_AES_256_CBC_SHA256` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_256_CBC_SHA256` field
* @property tlsRsaWithAes256CbcShaCiphersEnabled Should the `TLS_RSA_WITH_AES_256_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_256_CBC_SHA` field
* @property tlsRsaWithAes256GcmSha384CiphersEnabled Should the `TLS_RSA_WITH_AES_256_GCM_SHA384` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_256_GCM_SHA384` field
* @property tripleDesCiphersEnabled Should the `TLS_RSA_WITH_3DES_EDE_CBC_SHA` cipher be enabled for alL TLS versions (1.0, 1.1 and 1.2)?
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TripleDes168` field
*/
public data class ServiceSecurityArgs(
public val enableBackendSsl30: Output? = null,
public val enableBackendTls10: Output? = null,
public val enableBackendTls11: Output? = null,
public val enableFrontendSsl30: Output? = null,
public val enableFrontendTls10: Output? = null,
public val enableFrontendTls11: Output? = null,
public val tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled: Output? = null,
public val tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled: Output? = null,
public val tlsEcdheRsaWithAes128CbcShaCiphersEnabled: Output? = null,
public val tlsEcdheRsaWithAes256CbcShaCiphersEnabled: Output? = null,
public val tlsRsaWithAes128CbcSha256CiphersEnabled: Output? = null,
public val tlsRsaWithAes128CbcShaCiphersEnabled: Output? = null,
public val tlsRsaWithAes128GcmSha256CiphersEnabled: Output? = null,
public val tlsRsaWithAes256CbcSha256CiphersEnabled: Output? = null,
public val tlsRsaWithAes256CbcShaCiphersEnabled: Output? = null,
public val tlsRsaWithAes256GcmSha384CiphersEnabled: Output? = null,
public val tripleDesCiphersEnabled: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.apimanagement.inputs.ServiceSecurityArgs =
com.pulumi.azure.apimanagement.inputs.ServiceSecurityArgs.builder()
.enableBackendSsl30(enableBackendSsl30?.applyValue({ args0 -> args0 }))
.enableBackendTls10(enableBackendTls10?.applyValue({ args0 -> args0 }))
.enableBackendTls11(enableBackendTls11?.applyValue({ args0 -> args0 }))
.enableFrontendSsl30(enableFrontendSsl30?.applyValue({ args0 -> args0 }))
.enableFrontendTls10(enableFrontendTls10?.applyValue({ args0 -> args0 }))
.enableFrontendTls11(enableFrontendTls11?.applyValue({ args0 -> args0 }))
.tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled(
tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled?.applyValue({ args0 ->
args0
}),
)
.tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled(
tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled?.applyValue({ args0 ->
args0
}),
)
.tlsEcdheRsaWithAes128CbcShaCiphersEnabled(
tlsEcdheRsaWithAes128CbcShaCiphersEnabled?.applyValue({ args0 ->
args0
}),
)
.tlsEcdheRsaWithAes256CbcShaCiphersEnabled(
tlsEcdheRsaWithAes256CbcShaCiphersEnabled?.applyValue({ args0 ->
args0
}),
)
.tlsRsaWithAes128CbcSha256CiphersEnabled(
tlsRsaWithAes128CbcSha256CiphersEnabled?.applyValue({ args0 ->
args0
}),
)
.tlsRsaWithAes128CbcShaCiphersEnabled(
tlsRsaWithAes128CbcShaCiphersEnabled?.applyValue({ args0 ->
args0
}),
)
.tlsRsaWithAes128GcmSha256CiphersEnabled(
tlsRsaWithAes128GcmSha256CiphersEnabled?.applyValue({ args0 ->
args0
}),
)
.tlsRsaWithAes256CbcSha256CiphersEnabled(
tlsRsaWithAes256CbcSha256CiphersEnabled?.applyValue({ args0 ->
args0
}),
)
.tlsRsaWithAes256CbcShaCiphersEnabled(
tlsRsaWithAes256CbcShaCiphersEnabled?.applyValue({ args0 ->
args0
}),
)
.tlsRsaWithAes256GcmSha384CiphersEnabled(
tlsRsaWithAes256GcmSha384CiphersEnabled?.applyValue({ args0 ->
args0
}),
)
.tripleDesCiphersEnabled(tripleDesCiphersEnabled?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServiceSecurityArgs].
*/
@PulumiTagMarker
public class ServiceSecurityArgsBuilder internal constructor() {
private var enableBackendSsl30: Output? = null
private var enableBackendTls10: Output? = null
private var enableBackendTls11: Output? = null
private var enableFrontendSsl30: Output? = null
private var enableFrontendTls10: Output? = null
private var enableFrontendTls11: Output? = null
private var tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled: Output? = null
private var tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled: Output? = null
private var tlsEcdheRsaWithAes128CbcShaCiphersEnabled: Output? = null
private var tlsEcdheRsaWithAes256CbcShaCiphersEnabled: Output? = null
private var tlsRsaWithAes128CbcSha256CiphersEnabled: Output? = null
private var tlsRsaWithAes128CbcShaCiphersEnabled: Output? = null
private var tlsRsaWithAes128GcmSha256CiphersEnabled: Output? = null
private var tlsRsaWithAes256CbcSha256CiphersEnabled: Output? = null
private var tlsRsaWithAes256CbcShaCiphersEnabled: Output? = null
private var tlsRsaWithAes256GcmSha384CiphersEnabled: Output? = null
private var tripleDesCiphersEnabled: Output? = null
/**
* @param value Should SSL 3.0 be enabled on the backend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Backend.Protocols.Ssl30` field
*/
@JvmName("uaipmmystumgqglr")
public suspend fun enableBackendSsl30(`value`: Output) {
this.enableBackendSsl30 = value
}
/**
* @param value Should TLS 1.0 be enabled on the backend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Backend.Protocols.Tls10` field
*/
@JvmName("vewntytfnxevfdwt")
public suspend fun enableBackendTls10(`value`: Output) {
this.enableBackendTls10 = value
}
/**
* @param value Should TLS 1.1 be enabled on the backend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Backend.Protocols.Tls11` field
*/
@JvmName("lklimultfpigtotd")
public suspend fun enableBackendTls11(`value`: Output) {
this.enableBackendTls11 = value
}
/**
* @param value Should SSL 3.0 be enabled on the frontend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Protocols.Ssl30` field
*/
@JvmName("mrrldnmfjdjdnhnd")
public suspend fun enableFrontendSsl30(`value`: Output) {
this.enableFrontendSsl30 = value
}
/**
* @param value Should TLS 1.0 be enabled on the frontend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Protocols.Tls10` field
*/
@JvmName("alrjbgwxijicfkve")
public suspend fun enableFrontendTls10(`value`: Output) {
this.enableFrontendTls10 = value
}
/**
* @param value Should TLS 1.1 be enabled on the frontend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Protocols.Tls11` field
*/
@JvmName("myuvjtkiljlievnu")
public suspend fun enableFrontendTls11(`value`: Output) {
this.enableFrontendTls11 = value
}
/**
* @param value Should the `TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA` field
*/
@JvmName("bxcwkrlsforwbtxl")
public suspend fun tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled(`value`: Output) {
this.tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled = value
}
/**
* @param value Should the `TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA` field
*/
@JvmName("ehaodgucnigqgvhn")
public suspend fun tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled(`value`: Output) {
this.tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled = value
}
/**
* @param value Should the `TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA` field
*/
@JvmName("bhyvqqyyilbdmmja")
public suspend fun tlsEcdheRsaWithAes128CbcShaCiphersEnabled(`value`: Output) {
this.tlsEcdheRsaWithAes128CbcShaCiphersEnabled = value
}
/**
* @param value Should the `TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA` field
*/
@JvmName("viuhbfcmccwxjhww")
public suspend fun tlsEcdheRsaWithAes256CbcShaCiphersEnabled(`value`: Output) {
this.tlsEcdheRsaWithAes256CbcShaCiphersEnabled = value
}
/**
* @param value Should the `TLS_RSA_WITH_AES_128_CBC_SHA256` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_128_CBC_SHA256` field
*/
@JvmName("tbcknhlsbhubeqfc")
public suspend fun tlsRsaWithAes128CbcSha256CiphersEnabled(`value`: Output) {
this.tlsRsaWithAes128CbcSha256CiphersEnabled = value
}
/**
* @param value Should the `TLS_RSA_WITH_AES_128_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_128_CBC_SHA` field
*/
@JvmName("pmjyilxljuowjxtd")
public suspend fun tlsRsaWithAes128CbcShaCiphersEnabled(`value`: Output) {
this.tlsRsaWithAes128CbcShaCiphersEnabled = value
}
/**
* @param value Should the `TLS_RSA_WITH_AES_128_GCM_SHA256` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_128_GCM_SHA256` field
*/
@JvmName("clpxgomkceuiqydp")
public suspend fun tlsRsaWithAes128GcmSha256CiphersEnabled(`value`: Output) {
this.tlsRsaWithAes128GcmSha256CiphersEnabled = value
}
/**
* @param value Should the `TLS_RSA_WITH_AES_256_CBC_SHA256` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_256_CBC_SHA256` field
*/
@JvmName("pxdrjjjqofxoagtj")
public suspend fun tlsRsaWithAes256CbcSha256CiphersEnabled(`value`: Output) {
this.tlsRsaWithAes256CbcSha256CiphersEnabled = value
}
/**
* @param value Should the `TLS_RSA_WITH_AES_256_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_256_CBC_SHA` field
*/
@JvmName("khijhowqspcmveym")
public suspend fun tlsRsaWithAes256CbcShaCiphersEnabled(`value`: Output) {
this.tlsRsaWithAes256CbcShaCiphersEnabled = value
}
/**
* @param value Should the `TLS_RSA_WITH_AES_256_GCM_SHA384` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_256_GCM_SHA384` field
*/
@JvmName("tdxviurojjsexvpd")
public suspend fun tlsRsaWithAes256GcmSha384CiphersEnabled(`value`: Output) {
this.tlsRsaWithAes256GcmSha384CiphersEnabled = value
}
/**
* @param value Should the `TLS_RSA_WITH_3DES_EDE_CBC_SHA` cipher be enabled for alL TLS versions (1.0, 1.1 and 1.2)?
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TripleDes168` field
*/
@JvmName("idqpthlfsaskwgrb")
public suspend fun tripleDesCiphersEnabled(`value`: Output) {
this.tripleDesCiphersEnabled = value
}
/**
* @param value Should SSL 3.0 be enabled on the backend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Backend.Protocols.Ssl30` field
*/
@JvmName("yjwwodrtuoqvnikf")
public suspend fun enableBackendSsl30(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableBackendSsl30 = mapped
}
/**
* @param value Should TLS 1.0 be enabled on the backend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Backend.Protocols.Tls10` field
*/
@JvmName("evvjnfxakyrawmcg")
public suspend fun enableBackendTls10(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableBackendTls10 = mapped
}
/**
* @param value Should TLS 1.1 be enabled on the backend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Backend.Protocols.Tls11` field
*/
@JvmName("vphqcetgyjihegjm")
public suspend fun enableBackendTls11(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableBackendTls11 = mapped
}
/**
* @param value Should SSL 3.0 be enabled on the frontend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Protocols.Ssl30` field
*/
@JvmName("hjmhorqqcxjesixo")
public suspend fun enableFrontendSsl30(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableFrontendSsl30 = mapped
}
/**
* @param value Should TLS 1.0 be enabled on the frontend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Protocols.Tls10` field
*/
@JvmName("uqlopuiruddaisuj")
public suspend fun enableFrontendTls10(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableFrontendTls10 = mapped
}
/**
* @param value Should TLS 1.1 be enabled on the frontend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Protocols.Tls11` field
*/
@JvmName("tqpdqsyhxasogiub")
public suspend fun enableFrontendTls11(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableFrontendTls11 = mapped
}
/**
* @param value Should the `TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA` field
*/
@JvmName("adfhmeysnksdesof")
public suspend fun tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled = mapped
}
/**
* @param value Should the `TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA` field
*/
@JvmName("attcdivahgnsjdyy")
public suspend fun tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled = mapped
}
/**
* @param value Should the `TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA` field
*/
@JvmName("rpxidbcmvdfxjbwl")
public suspend fun tlsEcdheRsaWithAes128CbcShaCiphersEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsEcdheRsaWithAes128CbcShaCiphersEnabled = mapped
}
/**
* @param value Should the `TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA` field
*/
@JvmName("rrwgentttukdkpbq")
public suspend fun tlsEcdheRsaWithAes256CbcShaCiphersEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsEcdheRsaWithAes256CbcShaCiphersEnabled = mapped
}
/**
* @param value Should the `TLS_RSA_WITH_AES_128_CBC_SHA256` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_128_CBC_SHA256` field
*/
@JvmName("wjyeatnwvgqhucvt")
public suspend fun tlsRsaWithAes128CbcSha256CiphersEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsRsaWithAes128CbcSha256CiphersEnabled = mapped
}
/**
* @param value Should the `TLS_RSA_WITH_AES_128_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_128_CBC_SHA` field
*/
@JvmName("wvitflnrhknsgvcj")
public suspend fun tlsRsaWithAes128CbcShaCiphersEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsRsaWithAes128CbcShaCiphersEnabled = mapped
}
/**
* @param value Should the `TLS_RSA_WITH_AES_128_GCM_SHA256` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_128_GCM_SHA256` field
*/
@JvmName("wcoltihifkdqwckq")
public suspend fun tlsRsaWithAes128GcmSha256CiphersEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsRsaWithAes128GcmSha256CiphersEnabled = mapped
}
/**
* @param value Should the `TLS_RSA_WITH_AES_256_CBC_SHA256` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_256_CBC_SHA256` field
*/
@JvmName("rpokfpfcrvofyloj")
public suspend fun tlsRsaWithAes256CbcSha256CiphersEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsRsaWithAes256CbcSha256CiphersEnabled = mapped
}
/**
* @param value Should the `TLS_RSA_WITH_AES_256_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_256_CBC_SHA` field
*/
@JvmName("rkdpwbhnqshebodd")
public suspend fun tlsRsaWithAes256CbcShaCiphersEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsRsaWithAes256CbcShaCiphersEnabled = mapped
}
/**
* @param value Should the `TLS_RSA_WITH_AES_256_GCM_SHA384` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_256_GCM_SHA384` field
*/
@JvmName("mjubrdoiknmcckev")
public suspend fun tlsRsaWithAes256GcmSha384CiphersEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsRsaWithAes256GcmSha384CiphersEnabled = mapped
}
/**
* @param value Should the `TLS_RSA_WITH_3DES_EDE_CBC_SHA` cipher be enabled for alL TLS versions (1.0, 1.1 and 1.2)?
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TripleDes168` field
*/
@JvmName("kirymbbcqnorusay")
public suspend fun tripleDesCiphersEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tripleDesCiphersEnabled = mapped
}
internal fun build(): ServiceSecurityArgs = ServiceSecurityArgs(
enableBackendSsl30 = enableBackendSsl30,
enableBackendTls10 = enableBackendTls10,
enableBackendTls11 = enableBackendTls11,
enableFrontendSsl30 = enableFrontendSsl30,
enableFrontendTls10 = enableFrontendTls10,
enableFrontendTls11 = enableFrontendTls11,
tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled = tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled,
tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled = tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled,
tlsEcdheRsaWithAes128CbcShaCiphersEnabled = tlsEcdheRsaWithAes128CbcShaCiphersEnabled,
tlsEcdheRsaWithAes256CbcShaCiphersEnabled = tlsEcdheRsaWithAes256CbcShaCiphersEnabled,
tlsRsaWithAes128CbcSha256CiphersEnabled = tlsRsaWithAes128CbcSha256CiphersEnabled,
tlsRsaWithAes128CbcShaCiphersEnabled = tlsRsaWithAes128CbcShaCiphersEnabled,
tlsRsaWithAes128GcmSha256CiphersEnabled = tlsRsaWithAes128GcmSha256CiphersEnabled,
tlsRsaWithAes256CbcSha256CiphersEnabled = tlsRsaWithAes256CbcSha256CiphersEnabled,
tlsRsaWithAes256CbcShaCiphersEnabled = tlsRsaWithAes256CbcShaCiphersEnabled,
tlsRsaWithAes256GcmSha384CiphersEnabled = tlsRsaWithAes256GcmSha384CiphersEnabled,
tripleDesCiphersEnabled = tripleDesCiphersEnabled,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy