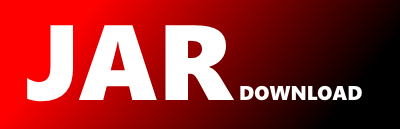
com.pulumi.azure.appplatform.kotlin.SpringCloudAppCosmosDBAssociationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appplatform.kotlin
import com.pulumi.azure.appplatform.SpringCloudAppCosmosDBAssociationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Associates a Spring Cloud Application with a CosmosDB Account.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleSpringCloudService = new azure.appplatform.SpringCloudService("example", {
* name: "example-springcloud",
* resourceGroupName: example.name,
* location: example.location,
* });
* const exampleSpringCloudApp = new azure.appplatform.SpringCloudApp("example", {
* name: "example-springcloudapp",
* resourceGroupName: example.name,
* serviceName: exampleSpringCloudService.name,
* });
* const exampleAccount = new azure.cosmosdb.Account("example", {
* name: "example-cosmosdb-account",
* location: example.location,
* resourceGroupName: example.name,
* offerType: "Standard",
* kind: "GlobalDocumentDB",
* consistencyPolicy: {
* consistencyLevel: "Strong",
* },
* geoLocations: [{
* location: example.location,
* failoverPriority: 0,
* }],
* });
* const exampleSpringCloudAppCosmosDBAssociation = new azure.appplatform.SpringCloudAppCosmosDBAssociation("example", {
* name: "example-bind",
* springCloudAppId: exampleSpringCloudApp.id,
* cosmosdbAccountId: exampleAccount.id,
* apiType: "table",
* cosmosdbAccessKey: exampleAccount.primaryKey,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_spring_cloud_service = azure.appplatform.SpringCloudService("example",
* name="example-springcloud",
* resource_group_name=example.name,
* location=example.location)
* example_spring_cloud_app = azure.appplatform.SpringCloudApp("example",
* name="example-springcloudapp",
* resource_group_name=example.name,
* service_name=example_spring_cloud_service.name)
* example_account = azure.cosmosdb.Account("example",
* name="example-cosmosdb-account",
* location=example.location,
* resource_group_name=example.name,
* offer_type="Standard",
* kind="GlobalDocumentDB",
* consistency_policy={
* "consistency_level": "Strong",
* },
* geo_locations=[{
* "location": example.location,
* "failover_priority": 0,
* }])
* example_spring_cloud_app_cosmos_dbassociation = azure.appplatform.SpringCloudAppCosmosDBAssociation("example",
* name="example-bind",
* spring_cloud_app_id=example_spring_cloud_app.id,
* cosmosdb_account_id=example_account.id,
* api_type="table",
* cosmosdb_access_key=example_account.primary_key)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleSpringCloudService = new Azure.AppPlatform.SpringCloudService("example", new()
* {
* Name = "example-springcloud",
* ResourceGroupName = example.Name,
* Location = example.Location,
* });
* var exampleSpringCloudApp = new Azure.AppPlatform.SpringCloudApp("example", new()
* {
* Name = "example-springcloudapp",
* ResourceGroupName = example.Name,
* ServiceName = exampleSpringCloudService.Name,
* });
* var exampleAccount = new Azure.CosmosDB.Account("example", new()
* {
* Name = "example-cosmosdb-account",
* Location = example.Location,
* ResourceGroupName = example.Name,
* OfferType = "Standard",
* Kind = "GlobalDocumentDB",
* ConsistencyPolicy = new Azure.CosmosDB.Inputs.AccountConsistencyPolicyArgs
* {
* ConsistencyLevel = "Strong",
* },
* GeoLocations = new[]
* {
* new Azure.CosmosDB.Inputs.AccountGeoLocationArgs
* {
* Location = example.Location,
* FailoverPriority = 0,
* },
* },
* });
* var exampleSpringCloudAppCosmosDBAssociation = new Azure.AppPlatform.SpringCloudAppCosmosDBAssociation("example", new()
* {
* Name = "example-bind",
* SpringCloudAppId = exampleSpringCloudApp.Id,
* CosmosdbAccountId = exampleAccount.Id,
* ApiType = "table",
* CosmosdbAccessKey = exampleAccount.PrimaryKey,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/appplatform"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/cosmosdb"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleSpringCloudService, err := appplatform.NewSpringCloudService(ctx, "example", &appplatform.SpringCloudServiceArgs{
* Name: pulumi.String("example-springcloud"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* })
* if err != nil {
* return err
* }
* exampleSpringCloudApp, err := appplatform.NewSpringCloudApp(ctx, "example", &appplatform.SpringCloudAppArgs{
* Name: pulumi.String("example-springcloudapp"),
* ResourceGroupName: example.Name,
* ServiceName: exampleSpringCloudService.Name,
* })
* if err != nil {
* return err
* }
* exampleAccount, err := cosmosdb.NewAccount(ctx, "example", &cosmosdb.AccountArgs{
* Name: pulumi.String("example-cosmosdb-account"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* OfferType: pulumi.String("Standard"),
* Kind: pulumi.String("GlobalDocumentDB"),
* ConsistencyPolicy: &cosmosdb.AccountConsistencyPolicyArgs{
* ConsistencyLevel: pulumi.String("Strong"),
* },
* GeoLocations: cosmosdb.AccountGeoLocationArray{
* &cosmosdb.AccountGeoLocationArgs{
* Location: example.Location,
* FailoverPriority: pulumi.Int(0),
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = appplatform.NewSpringCloudAppCosmosDBAssociation(ctx, "example", &appplatform.SpringCloudAppCosmosDBAssociationArgs{
* Name: pulumi.String("example-bind"),
* SpringCloudAppId: exampleSpringCloudApp.ID(),
* CosmosdbAccountId: exampleAccount.ID(),
* ApiType: pulumi.String("table"),
* CosmosdbAccessKey: exampleAccount.PrimaryKey,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.appplatform.SpringCloudService;
* import com.pulumi.azure.appplatform.SpringCloudServiceArgs;
* import com.pulumi.azure.appplatform.SpringCloudApp;
* import com.pulumi.azure.appplatform.SpringCloudAppArgs;
* import com.pulumi.azure.cosmosdb.Account;
* import com.pulumi.azure.cosmosdb.AccountArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountConsistencyPolicyArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountGeoLocationArgs;
* import com.pulumi.azure.appplatform.SpringCloudAppCosmosDBAssociation;
* import com.pulumi.azure.appplatform.SpringCloudAppCosmosDBAssociationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleSpringCloudService = new SpringCloudService("exampleSpringCloudService", SpringCloudServiceArgs.builder()
* .name("example-springcloud")
* .resourceGroupName(example.name())
* .location(example.location())
* .build());
* var exampleSpringCloudApp = new SpringCloudApp("exampleSpringCloudApp", SpringCloudAppArgs.builder()
* .name("example-springcloudapp")
* .resourceGroupName(example.name())
* .serviceName(exampleSpringCloudService.name())
* .build());
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("example-cosmosdb-account")
* .location(example.location())
* .resourceGroupName(example.name())
* .offerType("Standard")
* .kind("GlobalDocumentDB")
* .consistencyPolicy(AccountConsistencyPolicyArgs.builder()
* .consistencyLevel("Strong")
* .build())
* .geoLocations(AccountGeoLocationArgs.builder()
* .location(example.location())
* .failoverPriority(0)
* .build())
* .build());
* var exampleSpringCloudAppCosmosDBAssociation = new SpringCloudAppCosmosDBAssociation("exampleSpringCloudAppCosmosDBAssociation", SpringCloudAppCosmosDBAssociationArgs.builder()
* .name("example-bind")
* .springCloudAppId(exampleSpringCloudApp.id())
* .cosmosdbAccountId(exampleAccount.id())
* .apiType("table")
* .cosmosdbAccessKey(exampleAccount.primaryKey())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleSpringCloudService:
* type: azure:appplatform:SpringCloudService
* name: example
* properties:
* name: example-springcloud
* resourceGroupName: ${example.name}
* location: ${example.location}
* exampleSpringCloudApp:
* type: azure:appplatform:SpringCloudApp
* name: example
* properties:
* name: example-springcloudapp
* resourceGroupName: ${example.name}
* serviceName: ${exampleSpringCloudService.name}
* exampleAccount:
* type: azure:cosmosdb:Account
* name: example
* properties:
* name: example-cosmosdb-account
* location: ${example.location}
* resourceGroupName: ${example.name}
* offerType: Standard
* kind: GlobalDocumentDB
* consistencyPolicy:
* consistencyLevel: Strong
* geoLocations:
* - location: ${example.location}
* failoverPriority: 0
* exampleSpringCloudAppCosmosDBAssociation:
* type: azure:appplatform:SpringCloudAppCosmosDBAssociation
* name: example
* properties:
* name: example-bind
* springCloudAppId: ${exampleSpringCloudApp.id}
* cosmosdbAccountId: ${exampleAccount.id}
* apiType: table
* cosmosdbAccessKey: ${exampleAccount.primaryKey}
* ```
*
* ## Import
* Spring Cloud Application CosmosDB Association can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:appplatform/springCloudAppCosmosDBAssociation:SpringCloudAppCosmosDBAssociation example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resourcegroup1/providers/Microsoft.AppPlatform/spring/service1/apps/app1/bindings/bind1
* ```
* @property apiType Specifies the API type which should be used when connecting to the CosmosDB Account. Possible values are `cassandra`, `gremlin`, `mongo`, `sql` or `table`. Changing this forces a new resource to be created.
* @property cosmosdbAccessKey Specifies the CosmosDB Account access key.
* @property cosmosdbAccountId Specifies the ID of the CosmosDB Account. Changing this forces a new resource to be created.
* @property cosmosdbCassandraKeyspaceName Specifies the name of the Cassandra Keyspace which the Spring Cloud App should be associated with. Should only be set when `api_type` is `cassandra`.
* @property cosmosdbGremlinDatabaseName Specifies the name of the Gremlin Database which the Spring Cloud App should be associated with. Should only be set when `api_type` is `gremlin`.
* @property cosmosdbGremlinGraphName Specifies the name of the Gremlin Graph which the Spring Cloud App should be associated with. Should only be set when `api_type` is `gremlin`.
* @property cosmosdbMongoDatabaseName Specifies the name of the Mongo Database which the Spring Cloud App should be associated with. Should only be set when `api_type` is `mongo`.
* @property cosmosdbSqlDatabaseName Specifies the name of the SQL Database which the Spring Cloud App should be associated with. Should only be set when `api_type` is `sql`.
* @property name Specifies the name of the Spring Cloud Application Association. Changing this forces a new resource to be created.
* @property springCloudAppId Specifies the ID of the Spring Cloud Application where this Association is created. Changing this forces a new resource to be created.
*/
public data class SpringCloudAppCosmosDBAssociationArgs(
public val apiType: Output? = null,
public val cosmosdbAccessKey: Output? = null,
public val cosmosdbAccountId: Output? = null,
public val cosmosdbCassandraKeyspaceName: Output? = null,
public val cosmosdbGremlinDatabaseName: Output? = null,
public val cosmosdbGremlinGraphName: Output? = null,
public val cosmosdbMongoDatabaseName: Output? = null,
public val cosmosdbSqlDatabaseName: Output? = null,
public val name: Output? = null,
public val springCloudAppId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.appplatform.SpringCloudAppCosmosDBAssociationArgs =
com.pulumi.azure.appplatform.SpringCloudAppCosmosDBAssociationArgs.builder()
.apiType(apiType?.applyValue({ args0 -> args0 }))
.cosmosdbAccessKey(cosmosdbAccessKey?.applyValue({ args0 -> args0 }))
.cosmosdbAccountId(cosmosdbAccountId?.applyValue({ args0 -> args0 }))
.cosmosdbCassandraKeyspaceName(cosmosdbCassandraKeyspaceName?.applyValue({ args0 -> args0 }))
.cosmosdbGremlinDatabaseName(cosmosdbGremlinDatabaseName?.applyValue({ args0 -> args0 }))
.cosmosdbGremlinGraphName(cosmosdbGremlinGraphName?.applyValue({ args0 -> args0 }))
.cosmosdbMongoDatabaseName(cosmosdbMongoDatabaseName?.applyValue({ args0 -> args0 }))
.cosmosdbSqlDatabaseName(cosmosdbSqlDatabaseName?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.springCloudAppId(springCloudAppId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SpringCloudAppCosmosDBAssociationArgs].
*/
@PulumiTagMarker
public class SpringCloudAppCosmosDBAssociationArgsBuilder internal constructor() {
private var apiType: Output? = null
private var cosmosdbAccessKey: Output? = null
private var cosmosdbAccountId: Output? = null
private var cosmosdbCassandraKeyspaceName: Output? = null
private var cosmosdbGremlinDatabaseName: Output? = null
private var cosmosdbGremlinGraphName: Output? = null
private var cosmosdbMongoDatabaseName: Output? = null
private var cosmosdbSqlDatabaseName: Output? = null
private var name: Output? = null
private var springCloudAppId: Output? = null
/**
* @param value Specifies the API type which should be used when connecting to the CosmosDB Account. Possible values are `cassandra`, `gremlin`, `mongo`, `sql` or `table`. Changing this forces a new resource to be created.
*/
@JvmName("lqfwlxwxhlhfjdrl")
public suspend fun apiType(`value`: Output) {
this.apiType = value
}
/**
* @param value Specifies the CosmosDB Account access key.
*/
@JvmName("mlckgicollxgdvbd")
public suspend fun cosmosdbAccessKey(`value`: Output) {
this.cosmosdbAccessKey = value
}
/**
* @param value Specifies the ID of the CosmosDB Account. Changing this forces a new resource to be created.
*/
@JvmName("pvgrgrbomurdcevh")
public suspend fun cosmosdbAccountId(`value`: Output) {
this.cosmosdbAccountId = value
}
/**
* @param value Specifies the name of the Cassandra Keyspace which the Spring Cloud App should be associated with. Should only be set when `api_type` is `cassandra`.
*/
@JvmName("ysubdwsdigiilfwp")
public suspend fun cosmosdbCassandraKeyspaceName(`value`: Output) {
this.cosmosdbCassandraKeyspaceName = value
}
/**
* @param value Specifies the name of the Gremlin Database which the Spring Cloud App should be associated with. Should only be set when `api_type` is `gremlin`.
*/
@JvmName("jhrptcrbflewjphy")
public suspend fun cosmosdbGremlinDatabaseName(`value`: Output) {
this.cosmosdbGremlinDatabaseName = value
}
/**
* @param value Specifies the name of the Gremlin Graph which the Spring Cloud App should be associated with. Should only be set when `api_type` is `gremlin`.
*/
@JvmName("ywnlulyesaxurima")
public suspend fun cosmosdbGremlinGraphName(`value`: Output) {
this.cosmosdbGremlinGraphName = value
}
/**
* @param value Specifies the name of the Mongo Database which the Spring Cloud App should be associated with. Should only be set when `api_type` is `mongo`.
*/
@JvmName("iqkabcyceapvhlsc")
public suspend fun cosmosdbMongoDatabaseName(`value`: Output) {
this.cosmosdbMongoDatabaseName = value
}
/**
* @param value Specifies the name of the SQL Database which the Spring Cloud App should be associated with. Should only be set when `api_type` is `sql`.
*/
@JvmName("qnhetveuddcwjssd")
public suspend fun cosmosdbSqlDatabaseName(`value`: Output) {
this.cosmosdbSqlDatabaseName = value
}
/**
* @param value Specifies the name of the Spring Cloud Application Association. Changing this forces a new resource to be created.
*/
@JvmName("vbrjbabdopaeiriw")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Specifies the ID of the Spring Cloud Application where this Association is created. Changing this forces a new resource to be created.
*/
@JvmName("ytsfpvtvicwdnpwp")
public suspend fun springCloudAppId(`value`: Output) {
this.springCloudAppId = value
}
/**
* @param value Specifies the API type which should be used when connecting to the CosmosDB Account. Possible values are `cassandra`, `gremlin`, `mongo`, `sql` or `table`. Changing this forces a new resource to be created.
*/
@JvmName("cimbjvwvhixtetso")
public suspend fun apiType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiType = mapped
}
/**
* @param value Specifies the CosmosDB Account access key.
*/
@JvmName("boqkdsemwivaljyt")
public suspend fun cosmosdbAccessKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cosmosdbAccessKey = mapped
}
/**
* @param value Specifies the ID of the CosmosDB Account. Changing this forces a new resource to be created.
*/
@JvmName("eswatahmkokagifb")
public suspend fun cosmosdbAccountId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cosmosdbAccountId = mapped
}
/**
* @param value Specifies the name of the Cassandra Keyspace which the Spring Cloud App should be associated with. Should only be set when `api_type` is `cassandra`.
*/
@JvmName("qpsexthtcxmnesqf")
public suspend fun cosmosdbCassandraKeyspaceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cosmosdbCassandraKeyspaceName = mapped
}
/**
* @param value Specifies the name of the Gremlin Database which the Spring Cloud App should be associated with. Should only be set when `api_type` is `gremlin`.
*/
@JvmName("wlmuyrpnupoffspp")
public suspend fun cosmosdbGremlinDatabaseName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cosmosdbGremlinDatabaseName = mapped
}
/**
* @param value Specifies the name of the Gremlin Graph which the Spring Cloud App should be associated with. Should only be set when `api_type` is `gremlin`.
*/
@JvmName("micaiwaargqeilnf")
public suspend fun cosmosdbGremlinGraphName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cosmosdbGremlinGraphName = mapped
}
/**
* @param value Specifies the name of the Mongo Database which the Spring Cloud App should be associated with. Should only be set when `api_type` is `mongo`.
*/
@JvmName("mmvhmymavrakyfdk")
public suspend fun cosmosdbMongoDatabaseName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cosmosdbMongoDatabaseName = mapped
}
/**
* @param value Specifies the name of the SQL Database which the Spring Cloud App should be associated with. Should only be set when `api_type` is `sql`.
*/
@JvmName("idfplrkoryqlmkkt")
public suspend fun cosmosdbSqlDatabaseName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cosmosdbSqlDatabaseName = mapped
}
/**
* @param value Specifies the name of the Spring Cloud Application Association. Changing this forces a new resource to be created.
*/
@JvmName("hlasjjklcjoqxjqp")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Specifies the ID of the Spring Cloud Application where this Association is created. Changing this forces a new resource to be created.
*/
@JvmName("cgfbfdwxdnjiriru")
public suspend fun springCloudAppId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.springCloudAppId = mapped
}
internal fun build(): SpringCloudAppCosmosDBAssociationArgs =
SpringCloudAppCosmosDBAssociationArgs(
apiType = apiType,
cosmosdbAccessKey = cosmosdbAccessKey,
cosmosdbAccountId = cosmosdbAccountId,
cosmosdbCassandraKeyspaceName = cosmosdbCassandraKeyspaceName,
cosmosdbGremlinDatabaseName = cosmosdbGremlinDatabaseName,
cosmosdbGremlinGraphName = cosmosdbGremlinGraphName,
cosmosdbMongoDatabaseName = cosmosdbMongoDatabaseName,
cosmosdbSqlDatabaseName = cosmosdbSqlDatabaseName,
name = name,
springCloudAppId = springCloudAppId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy