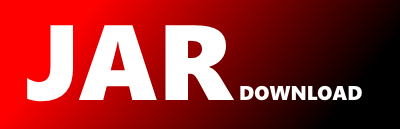
com.pulumi.azure.appplatform.kotlin.inputs.SpringCloudAppIngressSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appplatform.kotlin.inputs
import com.pulumi.azure.appplatform.inputs.SpringCloudAppIngressSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property backendProtocol Specifies how ingress should communicate with this app backend service. Allowed values are `GRPC` and `Default`. Defaults to `Default`.
* @property readTimeoutInSeconds Specifies the ingress read time out in seconds. Defaults to `300`.
* @property sendTimeoutInSeconds Specifies the ingress send time out in seconds. Defaults to `60`.
* @property sessionAffinity Specifies the type of the affinity, set this to `Cookie` to enable session affinity. Allowed values are `Cookie` and `None`. Defaults to `None`.
* @property sessionCookieMaxAge Specifies the time in seconds until the cookie expires.
*/
public data class SpringCloudAppIngressSettingsArgs(
public val backendProtocol: Output? = null,
public val readTimeoutInSeconds: Output? = null,
public val sendTimeoutInSeconds: Output? = null,
public val sessionAffinity: Output? = null,
public val sessionCookieMaxAge: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.appplatform.inputs.SpringCloudAppIngressSettingsArgs =
com.pulumi.azure.appplatform.inputs.SpringCloudAppIngressSettingsArgs.builder()
.backendProtocol(backendProtocol?.applyValue({ args0 -> args0 }))
.readTimeoutInSeconds(readTimeoutInSeconds?.applyValue({ args0 -> args0 }))
.sendTimeoutInSeconds(sendTimeoutInSeconds?.applyValue({ args0 -> args0 }))
.sessionAffinity(sessionAffinity?.applyValue({ args0 -> args0 }))
.sessionCookieMaxAge(sessionCookieMaxAge?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SpringCloudAppIngressSettingsArgs].
*/
@PulumiTagMarker
public class SpringCloudAppIngressSettingsArgsBuilder internal constructor() {
private var backendProtocol: Output? = null
private var readTimeoutInSeconds: Output? = null
private var sendTimeoutInSeconds: Output? = null
private var sessionAffinity: Output? = null
private var sessionCookieMaxAge: Output? = null
/**
* @param value Specifies how ingress should communicate with this app backend service. Allowed values are `GRPC` and `Default`. Defaults to `Default`.
*/
@JvmName("jqvbkssmvskdpekg")
public suspend fun backendProtocol(`value`: Output) {
this.backendProtocol = value
}
/**
* @param value Specifies the ingress read time out in seconds. Defaults to `300`.
*/
@JvmName("beplkposjqjbsouc")
public suspend fun readTimeoutInSeconds(`value`: Output) {
this.readTimeoutInSeconds = value
}
/**
* @param value Specifies the ingress send time out in seconds. Defaults to `60`.
*/
@JvmName("mvmmvoctsowwdsjs")
public suspend fun sendTimeoutInSeconds(`value`: Output) {
this.sendTimeoutInSeconds = value
}
/**
* @param value Specifies the type of the affinity, set this to `Cookie` to enable session affinity. Allowed values are `Cookie` and `None`. Defaults to `None`.
*/
@JvmName("rhuaoecbhtsqbtnq")
public suspend fun sessionAffinity(`value`: Output) {
this.sessionAffinity = value
}
/**
* @param value Specifies the time in seconds until the cookie expires.
*/
@JvmName("wpyeobayyogxlhwk")
public suspend fun sessionCookieMaxAge(`value`: Output) {
this.sessionCookieMaxAge = value
}
/**
* @param value Specifies how ingress should communicate with this app backend service. Allowed values are `GRPC` and `Default`. Defaults to `Default`.
*/
@JvmName("qxwxmaroepnptilu")
public suspend fun backendProtocol(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backendProtocol = mapped
}
/**
* @param value Specifies the ingress read time out in seconds. Defaults to `300`.
*/
@JvmName("kbmthdwxfvcsaptd")
public suspend fun readTimeoutInSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.readTimeoutInSeconds = mapped
}
/**
* @param value Specifies the ingress send time out in seconds. Defaults to `60`.
*/
@JvmName("xbocctrhcujlvbno")
public suspend fun sendTimeoutInSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sendTimeoutInSeconds = mapped
}
/**
* @param value Specifies the type of the affinity, set this to `Cookie` to enable session affinity. Allowed values are `Cookie` and `None`. Defaults to `None`.
*/
@JvmName("qaicogufmpwligat")
public suspend fun sessionAffinity(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sessionAffinity = mapped
}
/**
* @param value Specifies the time in seconds until the cookie expires.
*/
@JvmName("keunfvioxsnfptnn")
public suspend fun sessionCookieMaxAge(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sessionCookieMaxAge = mapped
}
internal fun build(): SpringCloudAppIngressSettingsArgs = SpringCloudAppIngressSettingsArgs(
backendProtocol = backendProtocol,
readTimeoutInSeconds = readTimeoutInSeconds,
sendTimeoutInSeconds = sendTimeoutInSeconds,
sessionAffinity = sessionAffinity,
sessionCookieMaxAge = sessionCookieMaxAge,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy