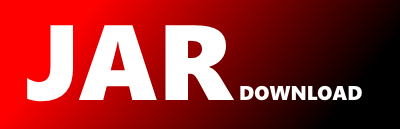
com.pulumi.azure.appservice.kotlin.inputs.LinuxWebAppSlotSiteConfigApplicationStackArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.inputs
import com.pulumi.azure.appservice.inputs.LinuxWebAppSlotSiteConfigApplicationStackArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property dockerImage
* @property dockerImageName The docker image, including tag, to be used. e.g. `appsvc/staticsite:latest`.
* @property dockerImageTag
* @property dockerRegistryPassword The User Name to use for authentication against the registry to pull the image.
* > **NOTE:** `docker_registry_url`, `docker_registry_username`, and `docker_registry_password` replace the use of the `app_settings` values of `DOCKER_REGISTRY_SERVER_URL`, `DOCKER_REGISTRY_SERVER_USERNAME` and `DOCKER_REGISTRY_SERVER_PASSWORD` respectively, these values will be managed by the provider and should not be specified in the `app_settings` map.
* @property dockerRegistryUrl The URL of the container registry where the `docker_image_name` is located. e.g. `https://index.docker.io` or `https://mcr.microsoft.com`. This value is required with `docker_image_name`.
* @property dockerRegistryUsername The User Name to use for authentication against the registry to pull the image.
* @property dotnetVersion The version of .NET to use. Possible values include `3.1`, `5.0`, `6.0`, `7.0` and `8.0`.
* @property goVersion The version of Go to use. Possible values include `1.18`, and `1.19`.
* @property javaServer The Java server type. Possible values include `JAVA`, `TOMCAT`, and `JBOSSEAP`.
* > **NOTE:** `JBOSSEAP` requires a Premium Service Plan SKU to be a valid option.
* @property javaServerVersion The Version of the `java_server` to use.
* @property javaVersion The Version of Java to use. Possible values include `8`, `11`, and `17`.
* > **NOTE:** The valid version combinations for `java_version`, `java_server` and `java_server_version` can be checked from the command line via `az webapp list-runtimes --linux`.
* @property nodeVersion The version of Node to run. Possible values are `12-lts`, `14-lts`, `16-lts`, `18-lts` and `20-lts`. This property conflicts with `java_version`.
* > **NOTE:** 10.x versions have been/are being deprecated so may cease to work for new resources in the future and may be removed from the provider.
* @property phpVersion The version of PHP to run. Possible values are `7.4`, `8.0`, `8.1`, `8.2` and `8.3`.
* > **NOTE:** version `7.4` is deprecated and will be removed from the provider in a future version.
* @property pythonVersion The version of Python to run. Possible values include `3.7`, `3.8`, `3.9`, `3.10`, `3.11` and `3.12`.
* @property rubyVersion The version of Ruby to run. Possible values include `2.6` and `2.7`.
*/
public data class LinuxWebAppSlotSiteConfigApplicationStackArgs(
@Deprecated(
message = """
This property has been deprecated and will be removed in 4.0 of the provider.
""",
)
public val dockerImage: Output? = null,
public val dockerImageName: Output? = null,
@Deprecated(
message = """
This property has been deprecated and will be removed in 4.0 of the provider.
""",
)
public val dockerImageTag: Output? = null,
public val dockerRegistryPassword: Output? = null,
public val dockerRegistryUrl: Output? = null,
public val dockerRegistryUsername: Output? = null,
public val dotnetVersion: Output? = null,
public val goVersion: Output? = null,
public val javaServer: Output? = null,
public val javaServerVersion: Output? = null,
public val javaVersion: Output? = null,
public val nodeVersion: Output? = null,
public val phpVersion: Output? = null,
public val pythonVersion: Output? = null,
public val rubyVersion: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azure.appservice.inputs.LinuxWebAppSlotSiteConfigApplicationStackArgs =
com.pulumi.azure.appservice.inputs.LinuxWebAppSlotSiteConfigApplicationStackArgs.builder()
.dockerImage(dockerImage?.applyValue({ args0 -> args0 }))
.dockerImageName(dockerImageName?.applyValue({ args0 -> args0 }))
.dockerImageTag(dockerImageTag?.applyValue({ args0 -> args0 }))
.dockerRegistryPassword(dockerRegistryPassword?.applyValue({ args0 -> args0 }))
.dockerRegistryUrl(dockerRegistryUrl?.applyValue({ args0 -> args0 }))
.dockerRegistryUsername(dockerRegistryUsername?.applyValue({ args0 -> args0 }))
.dotnetVersion(dotnetVersion?.applyValue({ args0 -> args0 }))
.goVersion(goVersion?.applyValue({ args0 -> args0 }))
.javaServer(javaServer?.applyValue({ args0 -> args0 }))
.javaServerVersion(javaServerVersion?.applyValue({ args0 -> args0 }))
.javaVersion(javaVersion?.applyValue({ args0 -> args0 }))
.nodeVersion(nodeVersion?.applyValue({ args0 -> args0 }))
.phpVersion(phpVersion?.applyValue({ args0 -> args0 }))
.pythonVersion(pythonVersion?.applyValue({ args0 -> args0 }))
.rubyVersion(rubyVersion?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LinuxWebAppSlotSiteConfigApplicationStackArgs].
*/
@PulumiTagMarker
public class LinuxWebAppSlotSiteConfigApplicationStackArgsBuilder internal constructor() {
private var dockerImage: Output? = null
private var dockerImageName: Output? = null
private var dockerImageTag: Output? = null
private var dockerRegistryPassword: Output? = null
private var dockerRegistryUrl: Output? = null
private var dockerRegistryUsername: Output? = null
private var dotnetVersion: Output? = null
private var goVersion: Output? = null
private var javaServer: Output? = null
private var javaServerVersion: Output? = null
private var javaVersion: Output? = null
private var nodeVersion: Output? = null
private var phpVersion: Output? = null
private var pythonVersion: Output? = null
private var rubyVersion: Output? = null
/**
* @param value
*/
@Deprecated(
message = """
This property has been deprecated and will be removed in 4.0 of the provider.
""",
)
@JvmName("hgjfwddrwmxxlbuh")
public suspend fun dockerImage(`value`: Output) {
this.dockerImage = value
}
/**
* @param value The docker image, including tag, to be used. e.g. `appsvc/staticsite:latest`.
*/
@JvmName("nxygrocpinoitpxk")
public suspend fun dockerImageName(`value`: Output) {
this.dockerImageName = value
}
/**
* @param value
*/
@Deprecated(
message = """
This property has been deprecated and will be removed in 4.0 of the provider.
""",
)
@JvmName("lholnfpcamlubdwl")
public suspend fun dockerImageTag(`value`: Output) {
this.dockerImageTag = value
}
/**
* @param value The User Name to use for authentication against the registry to pull the image.
* > **NOTE:** `docker_registry_url`, `docker_registry_username`, and `docker_registry_password` replace the use of the `app_settings` values of `DOCKER_REGISTRY_SERVER_URL`, `DOCKER_REGISTRY_SERVER_USERNAME` and `DOCKER_REGISTRY_SERVER_PASSWORD` respectively, these values will be managed by the provider and should not be specified in the `app_settings` map.
*/
@JvmName("iouipwjohcfrfjuf")
public suspend fun dockerRegistryPassword(`value`: Output) {
this.dockerRegistryPassword = value
}
/**
* @param value The URL of the container registry where the `docker_image_name` is located. e.g. `https://index.docker.io` or `https://mcr.microsoft.com`. This value is required with `docker_image_name`.
*/
@JvmName("ddrbloltxgqhuydb")
public suspend fun dockerRegistryUrl(`value`: Output) {
this.dockerRegistryUrl = value
}
/**
* @param value The User Name to use for authentication against the registry to pull the image.
*/
@JvmName("nxqowydtqiyhexxq")
public suspend fun dockerRegistryUsername(`value`: Output) {
this.dockerRegistryUsername = value
}
/**
* @param value The version of .NET to use. Possible values include `3.1`, `5.0`, `6.0`, `7.0` and `8.0`.
*/
@JvmName("bgstsnejolmyhdnk")
public suspend fun dotnetVersion(`value`: Output) {
this.dotnetVersion = value
}
/**
* @param value The version of Go to use. Possible values include `1.18`, and `1.19`.
*/
@JvmName("foshxgkyvikteadp")
public suspend fun goVersion(`value`: Output) {
this.goVersion = value
}
/**
* @param value The Java server type. Possible values include `JAVA`, `TOMCAT`, and `JBOSSEAP`.
* > **NOTE:** `JBOSSEAP` requires a Premium Service Plan SKU to be a valid option.
*/
@JvmName("cdcuqanmybhghpnc")
public suspend fun javaServer(`value`: Output) {
this.javaServer = value
}
/**
* @param value The Version of the `java_server` to use.
*/
@JvmName("fhnmgtfyynxjebmc")
public suspend fun javaServerVersion(`value`: Output) {
this.javaServerVersion = value
}
/**
* @param value The Version of Java to use. Possible values include `8`, `11`, and `17`.
* > **NOTE:** The valid version combinations for `java_version`, `java_server` and `java_server_version` can be checked from the command line via `az webapp list-runtimes --linux`.
*/
@JvmName("wuxlfobkxrgmtmwo")
public suspend fun javaVersion(`value`: Output) {
this.javaVersion = value
}
/**
* @param value The version of Node to run. Possible values are `12-lts`, `14-lts`, `16-lts`, `18-lts` and `20-lts`. This property conflicts with `java_version`.
* > **NOTE:** 10.x versions have been/are being deprecated so may cease to work for new resources in the future and may be removed from the provider.
*/
@JvmName("yofxgfiyrdesarwl")
public suspend fun nodeVersion(`value`: Output) {
this.nodeVersion = value
}
/**
* @param value The version of PHP to run. Possible values are `7.4`, `8.0`, `8.1`, `8.2` and `8.3`.
* > **NOTE:** version `7.4` is deprecated and will be removed from the provider in a future version.
*/
@JvmName("xkenxhshphqhxnwt")
public suspend fun phpVersion(`value`: Output) {
this.phpVersion = value
}
/**
* @param value The version of Python to run. Possible values include `3.7`, `3.8`, `3.9`, `3.10`, `3.11` and `3.12`.
*/
@JvmName("nibjbsdqvlbrdqqu")
public suspend fun pythonVersion(`value`: Output) {
this.pythonVersion = value
}
/**
* @param value The version of Ruby to run. Possible values include `2.6` and `2.7`.
*/
@JvmName("ajkmrlghnvxxhuvr")
public suspend fun rubyVersion(`value`: Output) {
this.rubyVersion = value
}
/**
* @param value
*/
@Deprecated(
message = """
This property has been deprecated and will be removed in 4.0 of the provider.
""",
)
@JvmName("ahqenkcksctcmxry")
public suspend fun dockerImage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerImage = mapped
}
/**
* @param value The docker image, including tag, to be used. e.g. `appsvc/staticsite:latest`.
*/
@JvmName("loakhtfyjmrpmppu")
public suspend fun dockerImageName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerImageName = mapped
}
/**
* @param value
*/
@Deprecated(
message = """
This property has been deprecated and will be removed in 4.0 of the provider.
""",
)
@JvmName("xrwoupqowmlmsidf")
public suspend fun dockerImageTag(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerImageTag = mapped
}
/**
* @param value The User Name to use for authentication against the registry to pull the image.
* > **NOTE:** `docker_registry_url`, `docker_registry_username`, and `docker_registry_password` replace the use of the `app_settings` values of `DOCKER_REGISTRY_SERVER_URL`, `DOCKER_REGISTRY_SERVER_USERNAME` and `DOCKER_REGISTRY_SERVER_PASSWORD` respectively, these values will be managed by the provider and should not be specified in the `app_settings` map.
*/
@JvmName("coftnktynmyclqck")
public suspend fun dockerRegistryPassword(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerRegistryPassword = mapped
}
/**
* @param value The URL of the container registry where the `docker_image_name` is located. e.g. `https://index.docker.io` or `https://mcr.microsoft.com`. This value is required with `docker_image_name`.
*/
@JvmName("dskfqwhbiutvdqln")
public suspend fun dockerRegistryUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerRegistryUrl = mapped
}
/**
* @param value The User Name to use for authentication against the registry to pull the image.
*/
@JvmName("vfrgrgnhugnuinbv")
public suspend fun dockerRegistryUsername(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerRegistryUsername = mapped
}
/**
* @param value The version of .NET to use. Possible values include `3.1`, `5.0`, `6.0`, `7.0` and `8.0`.
*/
@JvmName("qltptrilhttaemkc")
public suspend fun dotnetVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dotnetVersion = mapped
}
/**
* @param value The version of Go to use. Possible values include `1.18`, and `1.19`.
*/
@JvmName("rarlfqdddrjolsse")
public suspend fun goVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.goVersion = mapped
}
/**
* @param value The Java server type. Possible values include `JAVA`, `TOMCAT`, and `JBOSSEAP`.
* > **NOTE:** `JBOSSEAP` requires a Premium Service Plan SKU to be a valid option.
*/
@JvmName("lwlsmgwktgsgsuag")
public suspend fun javaServer(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.javaServer = mapped
}
/**
* @param value The Version of the `java_server` to use.
*/
@JvmName("ynggouhfbhyxcamj")
public suspend fun javaServerVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.javaServerVersion = mapped
}
/**
* @param value The Version of Java to use. Possible values include `8`, `11`, and `17`.
* > **NOTE:** The valid version combinations for `java_version`, `java_server` and `java_server_version` can be checked from the command line via `az webapp list-runtimes --linux`.
*/
@JvmName("hhtdkpnefyoycsvw")
public suspend fun javaVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.javaVersion = mapped
}
/**
* @param value The version of Node to run. Possible values are `12-lts`, `14-lts`, `16-lts`, `18-lts` and `20-lts`. This property conflicts with `java_version`.
* > **NOTE:** 10.x versions have been/are being deprecated so may cease to work for new resources in the future and may be removed from the provider.
*/
@JvmName("efbadtjuivjolarh")
public suspend fun nodeVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nodeVersion = mapped
}
/**
* @param value The version of PHP to run. Possible values are `7.4`, `8.0`, `8.1`, `8.2` and `8.3`.
* > **NOTE:** version `7.4` is deprecated and will be removed from the provider in a future version.
*/
@JvmName("nkidufxxwelebeeq")
public suspend fun phpVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.phpVersion = mapped
}
/**
* @param value The version of Python to run. Possible values include `3.7`, `3.8`, `3.9`, `3.10`, `3.11` and `3.12`.
*/
@JvmName("yuakqfrsdvwnavgy")
public suspend fun pythonVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pythonVersion = mapped
}
/**
* @param value The version of Ruby to run. Possible values include `2.6` and `2.7`.
*/
@JvmName("deikagceifagoysb")
public suspend fun rubyVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rubyVersion = mapped
}
internal fun build(): LinuxWebAppSlotSiteConfigApplicationStackArgs =
LinuxWebAppSlotSiteConfigApplicationStackArgs(
dockerImage = dockerImage,
dockerImageName = dockerImageName,
dockerImageTag = dockerImageTag,
dockerRegistryPassword = dockerRegistryPassword,
dockerRegistryUrl = dockerRegistryUrl,
dockerRegistryUsername = dockerRegistryUsername,
dotnetVersion = dotnetVersion,
goVersion = goVersion,
javaServer = javaServer,
javaServerVersion = javaServerVersion,
javaVersion = javaVersion,
nodeVersion = nodeVersion,
phpVersion = phpVersion,
pythonVersion = pythonVersion,
rubyVersion = rubyVersion,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy