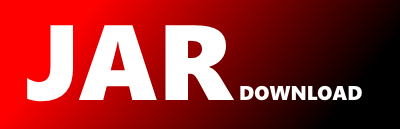
com.pulumi.azure.appservice.kotlin.inputs.WindowsFunctionAppSlotSiteConfigApplicationStackArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.inputs
import com.pulumi.azure.appservice.inputs.WindowsFunctionAppSlotSiteConfigApplicationStackArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property dotnetVersion The version of .Net. Possible values are `v3.0`, `v4.0`, `v6.0`, `v7.0` and `v8.0`. Defaults to `v4.0`.
* @property javaVersion The version of Java to use. Possible values are `1.8`, `11` and `17` (In-Preview).
* @property nodeVersion The version of Node to use. Possible values are `~12`, `~14`, `~16`, `~18` and `~20`.
* @property powershellCoreVersion The PowerShell Core version to use. Possible values are `7`, `7.2`, and `7.4`.
* @property useCustomRuntime Does the Function App use a custom Application Stack?
* @property useDotnetIsolatedRuntime Should the DotNet process use an isolated runtime. Defaults to `false`.
*/
public data class WindowsFunctionAppSlotSiteConfigApplicationStackArgs(
public val dotnetVersion: Output? = null,
public val javaVersion: Output? = null,
public val nodeVersion: Output? = null,
public val powershellCoreVersion: Output? = null,
public val useCustomRuntime: Output? = null,
public val useDotnetIsolatedRuntime: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azure.appservice.inputs.WindowsFunctionAppSlotSiteConfigApplicationStackArgs =
com.pulumi.azure.appservice.inputs.WindowsFunctionAppSlotSiteConfigApplicationStackArgs.builder()
.dotnetVersion(dotnetVersion?.applyValue({ args0 -> args0 }))
.javaVersion(javaVersion?.applyValue({ args0 -> args0 }))
.nodeVersion(nodeVersion?.applyValue({ args0 -> args0 }))
.powershellCoreVersion(powershellCoreVersion?.applyValue({ args0 -> args0 }))
.useCustomRuntime(useCustomRuntime?.applyValue({ args0 -> args0 }))
.useDotnetIsolatedRuntime(useDotnetIsolatedRuntime?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [WindowsFunctionAppSlotSiteConfigApplicationStackArgs].
*/
@PulumiTagMarker
public class WindowsFunctionAppSlotSiteConfigApplicationStackArgsBuilder internal constructor() {
private var dotnetVersion: Output? = null
private var javaVersion: Output? = null
private var nodeVersion: Output? = null
private var powershellCoreVersion: Output? = null
private var useCustomRuntime: Output? = null
private var useDotnetIsolatedRuntime: Output? = null
/**
* @param value The version of .Net. Possible values are `v3.0`, `v4.0`, `v6.0`, `v7.0` and `v8.0`. Defaults to `v4.0`.
*/
@JvmName("pmiryuxckjmvoqla")
public suspend fun dotnetVersion(`value`: Output) {
this.dotnetVersion = value
}
/**
* @param value The version of Java to use. Possible values are `1.8`, `11` and `17` (In-Preview).
*/
@JvmName("dfcvfyesikxjgbbm")
public suspend fun javaVersion(`value`: Output) {
this.javaVersion = value
}
/**
* @param value The version of Node to use. Possible values are `~12`, `~14`, `~16`, `~18` and `~20`.
*/
@JvmName("llbcjgvxqxhqerit")
public suspend fun nodeVersion(`value`: Output) {
this.nodeVersion = value
}
/**
* @param value The PowerShell Core version to use. Possible values are `7`, `7.2`, and `7.4`.
*/
@JvmName("rcqepxmbvpkqtxpx")
public suspend fun powershellCoreVersion(`value`: Output) {
this.powershellCoreVersion = value
}
/**
* @param value Does the Function App use a custom Application Stack?
*/
@JvmName("alprgetmdeghugll")
public suspend fun useCustomRuntime(`value`: Output) {
this.useCustomRuntime = value
}
/**
* @param value Should the DotNet process use an isolated runtime. Defaults to `false`.
*/
@JvmName("tjvkkatwepkhulib")
public suspend fun useDotnetIsolatedRuntime(`value`: Output) {
this.useDotnetIsolatedRuntime = value
}
/**
* @param value The version of .Net. Possible values are `v3.0`, `v4.0`, `v6.0`, `v7.0` and `v8.0`. Defaults to `v4.0`.
*/
@JvmName("wmdcbrblgbjasixk")
public suspend fun dotnetVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dotnetVersion = mapped
}
/**
* @param value The version of Java to use. Possible values are `1.8`, `11` and `17` (In-Preview).
*/
@JvmName("dqngfivroifewjkl")
public suspend fun javaVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.javaVersion = mapped
}
/**
* @param value The version of Node to use. Possible values are `~12`, `~14`, `~16`, `~18` and `~20`.
*/
@JvmName("cldnfsovghbheqja")
public suspend fun nodeVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nodeVersion = mapped
}
/**
* @param value The PowerShell Core version to use. Possible values are `7`, `7.2`, and `7.4`.
*/
@JvmName("splvkorondihfcys")
public suspend fun powershellCoreVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.powershellCoreVersion = mapped
}
/**
* @param value Does the Function App use a custom Application Stack?
*/
@JvmName("xpitmqqucsymdphi")
public suspend fun useCustomRuntime(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useCustomRuntime = mapped
}
/**
* @param value Should the DotNet process use an isolated runtime. Defaults to `false`.
*/
@JvmName("hckncofkkvpviwii")
public suspend fun useDotnetIsolatedRuntime(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useDotnetIsolatedRuntime = mapped
}
internal fun build(): WindowsFunctionAppSlotSiteConfigApplicationStackArgs =
WindowsFunctionAppSlotSiteConfigApplicationStackArgs(
dotnetVersion = dotnetVersion,
javaVersion = javaVersion,
nodeVersion = nodeVersion,
powershellCoreVersion = powershellCoreVersion,
useCustomRuntime = useCustomRuntime,
useDotnetIsolatedRuntime = useDotnetIsolatedRuntime,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy