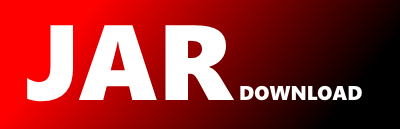
com.pulumi.azure.appservice.kotlin.inputs.WindowsWebAppSiteConfigApplicationStackArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.inputs
import com.pulumi.azure.appservice.inputs.WindowsWebAppSiteConfigApplicationStackArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property currentStack The Application Stack for the Windows Web App. Possible values include `dotnet`, `dotnetcore`, `node`, `python`, `php`, and `java`.
* > **NOTE:** Whilst this property is Optional omitting it can cause unexpected behaviour, in particular for display of settings in the Azure Portal.
* > **NOTE:** Windows Web apps can configure multiple `app_stack` properties, it is recommended to always configure this `Optional` value and set it to the primary application stack of your app to ensure correct operation of this resource and display the correct metadata in the Azure Portal.
* @property dockerContainerName The name of the container to be used. This value is required with `docker_container_tag`.
* @property dockerContainerRegistry
* @property dockerContainerTag The tag of the container to be used. This value is required with `docker_container_name`.
* @property dockerImageName The docker image, including tag, to be used. e.g. `azure-app-service/windows/parkingpage:latest`.
* @property dockerRegistryPassword The User Name to use for authentication against the registry to pull the image.
* > **NOTE:** `docker_registry_url`, `docker_registry_username`, and `docker_registry_password` replace the use of the `app_settings` values of `DOCKER_REGISTRY_SERVER_URL`, `DOCKER_REGISTRY_SERVER_USERNAME` and `DOCKER_REGISTRY_SERVER_PASSWORD` respectively, these values will be managed by the provider and should not be specified in the `app_settings` map.
* @property dockerRegistryUrl The URL of the container registry where the `docker_image_name` is located. e.g. `https://index.docker.io` or `https://mcr.microsoft.com`. This value is required with `docker_image_name`.
* @property dockerRegistryUsername The User Name to use for authentication against the registry to pull the image.
* @property dotnetCoreVersion The version of .NET to use when `current_stack` is set to `dotnetcore`. Possible values include `v4.0`.
* @property dotnetVersion The version of .NET to use when `current_stack` is set to `dotnet`. Possible values include `v2.0`,`v3.0`, `v4.0`, `v5.0`, `v6.0`, `v7.0` and `v8.0`.
* > **NOTE:** The Portal displayed values and the actual underlying API values differ for this setting, as follows:
* Portal Value | API value
* :--|--:
* ASP.NET V3.5 | v2.0
* ASP.NET V4.8 | v4.0
* .NET 6 (LTS) | v6.0
* .NET 7 (STS) | v7.0
* .NET 8 (LTS) | v8.0
* @property javaContainer
* @property javaContainerVersion
* @property javaEmbeddedServerEnabled Should the Java Embedded Server (Java SE) be used to run the app.
* @property javaVersion The version of Java to use when `current_stack` is set to `java`.
* > **NOTE:** For currently supported versions, please see the official documentation. Some example values include: `1.8`, `1.8.0_322`, `11`, `11.0.14`, `17` and `17.0.2`
* @property nodeVersion The version of node to use when `current_stack` is set to `node`. Possible values are `~12`, `~14`, `~16`, `~18` and `~20`.
* > **NOTE:** This property conflicts with `java_version`.
* @property phpVersion The version of PHP to use when `current_stack` is set to `php`. Possible values are `7.1`, `7.4` and `Off`.
* > **NOTE:** The value `Off` is used to signify latest supported by the service.
* @property python Specifies whether this is a Python app. Defaults to `false`.
* @property pythonVersion
* @property tomcatVersion The version of Tomcat the Java App should use. Conflicts with `java_embedded_server_enabled`
* > **NOTE:** See the official documentation for current supported versions. Some example valuess include: `10.0`, `10.0.20`.
*/
public data class WindowsWebAppSiteConfigApplicationStackArgs(
public val currentStack: Output? = null,
public val dockerContainerName: Output? = null,
@Deprecated(
message = """
This property has been deprecated and will be removed in a future release of the provider.
""",
)
public val dockerContainerRegistry: Output? = null,
public val dockerContainerTag: Output? = null,
public val dockerImageName: Output? = null,
public val dockerRegistryPassword: Output? = null,
public val dockerRegistryUrl: Output? = null,
public val dockerRegistryUsername: Output? = null,
public val dotnetCoreVersion: Output? = null,
public val dotnetVersion: Output? = null,
@Deprecated(
message = """
this property has been deprecated in favour of `tomcat_version` and `java_embedded_server_enabled`
""",
)
public val javaContainer: Output? = null,
@Deprecated(
message = """
This property has been deprecated in favour of `tomcat_version` and `java_embedded_server_enabled`
""",
)
public val javaContainerVersion: Output? = null,
public val javaEmbeddedServerEnabled: Output? = null,
public val javaVersion: Output? = null,
public val nodeVersion: Output? = null,
public val phpVersion: Output? = null,
public val python: Output? = null,
@Deprecated(
message = """
This property is deprecated. Values set are not used by the service.
""",
)
public val pythonVersion: Output? = null,
public val tomcatVersion: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azure.appservice.inputs.WindowsWebAppSiteConfigApplicationStackArgs =
com.pulumi.azure.appservice.inputs.WindowsWebAppSiteConfigApplicationStackArgs.builder()
.currentStack(currentStack?.applyValue({ args0 -> args0 }))
.dockerContainerName(dockerContainerName?.applyValue({ args0 -> args0 }))
.dockerContainerRegistry(dockerContainerRegistry?.applyValue({ args0 -> args0 }))
.dockerContainerTag(dockerContainerTag?.applyValue({ args0 -> args0 }))
.dockerImageName(dockerImageName?.applyValue({ args0 -> args0 }))
.dockerRegistryPassword(dockerRegistryPassword?.applyValue({ args0 -> args0 }))
.dockerRegistryUrl(dockerRegistryUrl?.applyValue({ args0 -> args0 }))
.dockerRegistryUsername(dockerRegistryUsername?.applyValue({ args0 -> args0 }))
.dotnetCoreVersion(dotnetCoreVersion?.applyValue({ args0 -> args0 }))
.dotnetVersion(dotnetVersion?.applyValue({ args0 -> args0 }))
.javaContainer(javaContainer?.applyValue({ args0 -> args0 }))
.javaContainerVersion(javaContainerVersion?.applyValue({ args0 -> args0 }))
.javaEmbeddedServerEnabled(javaEmbeddedServerEnabled?.applyValue({ args0 -> args0 }))
.javaVersion(javaVersion?.applyValue({ args0 -> args0 }))
.nodeVersion(nodeVersion?.applyValue({ args0 -> args0 }))
.phpVersion(phpVersion?.applyValue({ args0 -> args0 }))
.python(python?.applyValue({ args0 -> args0 }))
.pythonVersion(pythonVersion?.applyValue({ args0 -> args0 }))
.tomcatVersion(tomcatVersion?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [WindowsWebAppSiteConfigApplicationStackArgs].
*/
@PulumiTagMarker
public class WindowsWebAppSiteConfigApplicationStackArgsBuilder internal constructor() {
private var currentStack: Output? = null
private var dockerContainerName: Output? = null
private var dockerContainerRegistry: Output? = null
private var dockerContainerTag: Output? = null
private var dockerImageName: Output? = null
private var dockerRegistryPassword: Output? = null
private var dockerRegistryUrl: Output? = null
private var dockerRegistryUsername: Output? = null
private var dotnetCoreVersion: Output? = null
private var dotnetVersion: Output? = null
private var javaContainer: Output? = null
private var javaContainerVersion: Output? = null
private var javaEmbeddedServerEnabled: Output? = null
private var javaVersion: Output? = null
private var nodeVersion: Output? = null
private var phpVersion: Output? = null
private var python: Output? = null
private var pythonVersion: Output? = null
private var tomcatVersion: Output? = null
/**
* @param value The Application Stack for the Windows Web App. Possible values include `dotnet`, `dotnetcore`, `node`, `python`, `php`, and `java`.
* > **NOTE:** Whilst this property is Optional omitting it can cause unexpected behaviour, in particular for display of settings in the Azure Portal.
* > **NOTE:** Windows Web apps can configure multiple `app_stack` properties, it is recommended to always configure this `Optional` value and set it to the primary application stack of your app to ensure correct operation of this resource and display the correct metadata in the Azure Portal.
*/
@JvmName("mtjgaeysagadwynf")
public suspend fun currentStack(`value`: Output) {
this.currentStack = value
}
/**
* @param value The name of the container to be used. This value is required with `docker_container_tag`.
*/
@JvmName("tukqsdmptuhaveki")
public suspend fun dockerContainerName(`value`: Output) {
this.dockerContainerName = value
}
/**
* @param value
*/
@Deprecated(
message = """
This property has been deprecated and will be removed in a future release of the provider.
""",
)
@JvmName("mufjgmsxkijadsta")
public suspend fun dockerContainerRegistry(`value`: Output) {
this.dockerContainerRegistry = value
}
/**
* @param value The tag of the container to be used. This value is required with `docker_container_name`.
*/
@JvmName("jjitucmawmvsagwn")
public suspend fun dockerContainerTag(`value`: Output) {
this.dockerContainerTag = value
}
/**
* @param value The docker image, including tag, to be used. e.g. `azure-app-service/windows/parkingpage:latest`.
*/
@JvmName("jbciqgkbmbfbcjlu")
public suspend fun dockerImageName(`value`: Output) {
this.dockerImageName = value
}
/**
* @param value The User Name to use for authentication against the registry to pull the image.
* > **NOTE:** `docker_registry_url`, `docker_registry_username`, and `docker_registry_password` replace the use of the `app_settings` values of `DOCKER_REGISTRY_SERVER_URL`, `DOCKER_REGISTRY_SERVER_USERNAME` and `DOCKER_REGISTRY_SERVER_PASSWORD` respectively, these values will be managed by the provider and should not be specified in the `app_settings` map.
*/
@JvmName("cidbqnifuxkvylkc")
public suspend fun dockerRegistryPassword(`value`: Output) {
this.dockerRegistryPassword = value
}
/**
* @param value The URL of the container registry where the `docker_image_name` is located. e.g. `https://index.docker.io` or `https://mcr.microsoft.com`. This value is required with `docker_image_name`.
*/
@JvmName("gknvvpwtncedtjml")
public suspend fun dockerRegistryUrl(`value`: Output) {
this.dockerRegistryUrl = value
}
/**
* @param value The User Name to use for authentication against the registry to pull the image.
*/
@JvmName("llkagqgnmprxsaqs")
public suspend fun dockerRegistryUsername(`value`: Output) {
this.dockerRegistryUsername = value
}
/**
* @param value The version of .NET to use when `current_stack` is set to `dotnetcore`. Possible values include `v4.0`.
*/
@JvmName("ussptqjuojjkvdxm")
public suspend fun dotnetCoreVersion(`value`: Output) {
this.dotnetCoreVersion = value
}
/**
* @param value The version of .NET to use when `current_stack` is set to `dotnet`. Possible values include `v2.0`,`v3.0`, `v4.0`, `v5.0`, `v6.0`, `v7.0` and `v8.0`.
* > **NOTE:** The Portal displayed values and the actual underlying API values differ for this setting, as follows:
* Portal Value | API value
* :--|--:
* ASP.NET V3.5 | v2.0
* ASP.NET V4.8 | v4.0
* .NET 6 (LTS) | v6.0
* .NET 7 (STS) | v7.0
* .NET 8 (LTS) | v8.0
*/
@JvmName("uyhyjdetkjablkap")
public suspend fun dotnetVersion(`value`: Output) {
this.dotnetVersion = value
}
/**
* @param value
*/
@Deprecated(
message = """
this property has been deprecated in favour of `tomcat_version` and `java_embedded_server_enabled`
""",
)
@JvmName("lmgukdqltdvkwnwk")
public suspend fun javaContainer(`value`: Output) {
this.javaContainer = value
}
/**
* @param value
*/
@Deprecated(
message = """
This property has been deprecated in favour of `tomcat_version` and `java_embedded_server_enabled`
""",
)
@JvmName("eyjqevifkufaybro")
public suspend fun javaContainerVersion(`value`: Output) {
this.javaContainerVersion = value
}
/**
* @param value Should the Java Embedded Server (Java SE) be used to run the app.
*/
@JvmName("qiubecsbqrrosguv")
public suspend fun javaEmbeddedServerEnabled(`value`: Output) {
this.javaEmbeddedServerEnabled = value
}
/**
* @param value The version of Java to use when `current_stack` is set to `java`.
* > **NOTE:** For currently supported versions, please see the official documentation. Some example values include: `1.8`, `1.8.0_322`, `11`, `11.0.14`, `17` and `17.0.2`
*/
@JvmName("ucmiwfwqlhcjhswv")
public suspend fun javaVersion(`value`: Output) {
this.javaVersion = value
}
/**
* @param value The version of node to use when `current_stack` is set to `node`. Possible values are `~12`, `~14`, `~16`, `~18` and `~20`.
* > **NOTE:** This property conflicts with `java_version`.
*/
@JvmName("jmvtilcaffkhtwef")
public suspend fun nodeVersion(`value`: Output) {
this.nodeVersion = value
}
/**
* @param value The version of PHP to use when `current_stack` is set to `php`. Possible values are `7.1`, `7.4` and `Off`.
* > **NOTE:** The value `Off` is used to signify latest supported by the service.
*/
@JvmName("hplydsttlucwtorp")
public suspend fun phpVersion(`value`: Output) {
this.phpVersion = value
}
/**
* @param value Specifies whether this is a Python app. Defaults to `false`.
*/
@JvmName("ybocqggaqxdykgqg")
public suspend fun python(`value`: Output) {
this.python = value
}
/**
* @param value
*/
@Deprecated(
message = """
This property is deprecated. Values set are not used by the service.
""",
)
@JvmName("hrcgffknhjpjgpog")
public suspend fun pythonVersion(`value`: Output) {
this.pythonVersion = value
}
/**
* @param value The version of Tomcat the Java App should use. Conflicts with `java_embedded_server_enabled`
* > **NOTE:** See the official documentation for current supported versions. Some example valuess include: `10.0`, `10.0.20`.
*/
@JvmName("woljvbwriqbgpgcu")
public suspend fun tomcatVersion(`value`: Output) {
this.tomcatVersion = value
}
/**
* @param value The Application Stack for the Windows Web App. Possible values include `dotnet`, `dotnetcore`, `node`, `python`, `php`, and `java`.
* > **NOTE:** Whilst this property is Optional omitting it can cause unexpected behaviour, in particular for display of settings in the Azure Portal.
* > **NOTE:** Windows Web apps can configure multiple `app_stack` properties, it is recommended to always configure this `Optional` value and set it to the primary application stack of your app to ensure correct operation of this resource and display the correct metadata in the Azure Portal.
*/
@JvmName("pihdjbrkvqdwnnys")
public suspend fun currentStack(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.currentStack = mapped
}
/**
* @param value The name of the container to be used. This value is required with `docker_container_tag`.
*/
@JvmName("rwqkreapiaembyqu")
public suspend fun dockerContainerName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerContainerName = mapped
}
/**
* @param value
*/
@Deprecated(
message = """
This property has been deprecated and will be removed in a future release of the provider.
""",
)
@JvmName("tacnndwgbtykwnxi")
public suspend fun dockerContainerRegistry(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerContainerRegistry = mapped
}
/**
* @param value The tag of the container to be used. This value is required with `docker_container_name`.
*/
@JvmName("ehaqgnqpodrpgbjp")
public suspend fun dockerContainerTag(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerContainerTag = mapped
}
/**
* @param value The docker image, including tag, to be used. e.g. `azure-app-service/windows/parkingpage:latest`.
*/
@JvmName("qmqemlnuvlrgtfow")
public suspend fun dockerImageName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerImageName = mapped
}
/**
* @param value The User Name to use for authentication against the registry to pull the image.
* > **NOTE:** `docker_registry_url`, `docker_registry_username`, and `docker_registry_password` replace the use of the `app_settings` values of `DOCKER_REGISTRY_SERVER_URL`, `DOCKER_REGISTRY_SERVER_USERNAME` and `DOCKER_REGISTRY_SERVER_PASSWORD` respectively, these values will be managed by the provider and should not be specified in the `app_settings` map.
*/
@JvmName("ebmmgtyrxjcsxsnc")
public suspend fun dockerRegistryPassword(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerRegistryPassword = mapped
}
/**
* @param value The URL of the container registry where the `docker_image_name` is located. e.g. `https://index.docker.io` or `https://mcr.microsoft.com`. This value is required with `docker_image_name`.
*/
@JvmName("dsyrrocptvnaxaww")
public suspend fun dockerRegistryUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerRegistryUrl = mapped
}
/**
* @param value The User Name to use for authentication against the registry to pull the image.
*/
@JvmName("ddsjalyromkactho")
public suspend fun dockerRegistryUsername(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dockerRegistryUsername = mapped
}
/**
* @param value The version of .NET to use when `current_stack` is set to `dotnetcore`. Possible values include `v4.0`.
*/
@JvmName("olyayggmweellsuy")
public suspend fun dotnetCoreVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dotnetCoreVersion = mapped
}
/**
* @param value The version of .NET to use when `current_stack` is set to `dotnet`. Possible values include `v2.0`,`v3.0`, `v4.0`, `v5.0`, `v6.0`, `v7.0` and `v8.0`.
* > **NOTE:** The Portal displayed values and the actual underlying API values differ for this setting, as follows:
* Portal Value | API value
* :--|--:
* ASP.NET V3.5 | v2.0
* ASP.NET V4.8 | v4.0
* .NET 6 (LTS) | v6.0
* .NET 7 (STS) | v7.0
* .NET 8 (LTS) | v8.0
*/
@JvmName("cqtgpwriadwskabh")
public suspend fun dotnetVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dotnetVersion = mapped
}
/**
* @param value
*/
@Deprecated(
message = """
this property has been deprecated in favour of `tomcat_version` and `java_embedded_server_enabled`
""",
)
@JvmName("jxhnufjxvxcdlyud")
public suspend fun javaContainer(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.javaContainer = mapped
}
/**
* @param value
*/
@Deprecated(
message = """
This property has been deprecated in favour of `tomcat_version` and `java_embedded_server_enabled`
""",
)
@JvmName("sqfngpbjgmidfomr")
public suspend fun javaContainerVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.javaContainerVersion = mapped
}
/**
* @param value Should the Java Embedded Server (Java SE) be used to run the app.
*/
@JvmName("syhgxmgqcpwhcpnt")
public suspend fun javaEmbeddedServerEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.javaEmbeddedServerEnabled = mapped
}
/**
* @param value The version of Java to use when `current_stack` is set to `java`.
* > **NOTE:** For currently supported versions, please see the official documentation. Some example values include: `1.8`, `1.8.0_322`, `11`, `11.0.14`, `17` and `17.0.2`
*/
@JvmName("gnnlqhiapjlxmhpx")
public suspend fun javaVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.javaVersion = mapped
}
/**
* @param value The version of node to use when `current_stack` is set to `node`. Possible values are `~12`, `~14`, `~16`, `~18` and `~20`.
* > **NOTE:** This property conflicts with `java_version`.
*/
@JvmName("gmnuyaonneivcjly")
public suspend fun nodeVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nodeVersion = mapped
}
/**
* @param value The version of PHP to use when `current_stack` is set to `php`. Possible values are `7.1`, `7.4` and `Off`.
* > **NOTE:** The value `Off` is used to signify latest supported by the service.
*/
@JvmName("dehpbykebpocboxl")
public suspend fun phpVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.phpVersion = mapped
}
/**
* @param value Specifies whether this is a Python app. Defaults to `false`.
*/
@JvmName("jsutqfernfarxaoj")
public suspend fun python(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.python = mapped
}
/**
* @param value
*/
@Deprecated(
message = """
This property is deprecated. Values set are not used by the service.
""",
)
@JvmName("glawcqyqsjaeaiso")
public suspend fun pythonVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pythonVersion = mapped
}
/**
* @param value The version of Tomcat the Java App should use. Conflicts with `java_embedded_server_enabled`
* > **NOTE:** See the official documentation for current supported versions. Some example valuess include: `10.0`, `10.0.20`.
*/
@JvmName("exefrnpxhdrjbkev")
public suspend fun tomcatVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tomcatVersion = mapped
}
internal fun build(): WindowsWebAppSiteConfigApplicationStackArgs =
WindowsWebAppSiteConfigApplicationStackArgs(
currentStack = currentStack,
dockerContainerName = dockerContainerName,
dockerContainerRegistry = dockerContainerRegistry,
dockerContainerTag = dockerContainerTag,
dockerImageName = dockerImageName,
dockerRegistryPassword = dockerRegistryPassword,
dockerRegistryUrl = dockerRegistryUrl,
dockerRegistryUsername = dockerRegistryUsername,
dotnetCoreVersion = dotnetCoreVersion,
dotnetVersion = dotnetVersion,
javaContainer = javaContainer,
javaContainerVersion = javaContainerVersion,
javaEmbeddedServerEnabled = javaEmbeddedServerEnabled,
javaVersion = javaVersion,
nodeVersion = nodeVersion,
phpVersion = phpVersion,
python = python,
pythonVersion = pythonVersion,
tomcatVersion = tomcatVersion,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy