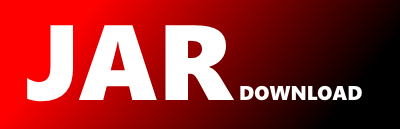
com.pulumi.azure.appservice.kotlin.outputs.FunctionAppSiteConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property alwaysOn Should the Function App be loaded at all times? Defaults to `false`.
* @property appScaleLimit The number of workers this function app can scale out to. Only applicable to apps on the Consumption and Premium plan.
* @property autoSwapSlotName The name of the slot to automatically swap to during deployment
* > **NOTE:** This attribute is only used for slots.
* @property cors A `cors` block as defined below.
* @property dotnetFrameworkVersion The version of the .NET framework's CLR used in this function app. Possible values are `v4.0` (including .NET Core 2.1 and 3.1), `v5.0` and `v6.0`. [For more information on which .NET Framework version to use based on the runtime version you're targeting - please see this table](https://docs.microsoft.com/azure/azure-functions/functions-dotnet-class-library#supported-versions). Defaults to `v4.0`.
* @property elasticInstanceMinimum The number of minimum instances for this function app. Only affects apps on the Premium plan.
* @property ftpsState State of FTP / FTPS service for this function app. Possible values include: `AllAllowed`, `FtpsOnly` and `Disabled`. Defaults to `AllAllowed`.
* @property healthCheckPath Path which will be checked for this function app health.
* @property http2Enabled Specifies whether or not the HTTP2 protocol should be enabled. Defaults to `false`.
* @property ipRestrictions A list of `ip_restriction` objects representing IP restrictions as defined below.
* > **NOTE** User has to explicitly set `ip_restriction` to empty slice (`[]`) to remove it.
* @property javaVersion Java version hosted by the function app in Azure. Possible values are `1.8`, `11` & `17` (In-Preview).
* @property linuxFxVersion Linux App Framework and version for the AppService, e.g. `DOCKER|(golang:latest)`.
* @property minTlsVersion The minimum supported TLS version for the function app. Possible values are `1.0`, `1.1`, and `1.2`. Defaults to `1.2` for new function apps.
* @property preWarmedInstanceCount The number of pre-warmed instances for this function app. Only affects apps on the Premium plan.
* @property runtimeScaleMonitoringEnabled Should Runtime Scale Monitoring be enabled?. Only applicable to apps on the Premium plan. Defaults to `false`.
* @property scmIpRestrictions A list of `scm_ip_restriction` objects representing IP restrictions as defined below.
* > **NOTE** User has to explicitly set `scm_ip_restriction` to empty slice (`[]`) to remove it.
* @property scmType The type of Source Control used by the Function App. Valid values include: `BitBucketGit`, `BitBucketHg`, `CodePlexGit`, `CodePlexHg`, `Dropbox`, `ExternalGit`, `ExternalHg`, `GitHub`, `LocalGit`, `None` (default), `OneDrive`, `Tfs`, `VSO`, and `VSTSRM`.
* > **NOTE:** This setting is incompatible with the `source_control` block which updates this value based on the setting provided.
* @property scmUseMainIpRestriction IP security restrictions for scm to use main. Defaults to `false`.
* > **NOTE** Any `scm_ip_restriction` blocks configured are ignored by the service when `scm_use_main_ip_restriction` is set to `true`. Any scm restrictions will become active if this is subsequently set to `false` or removed.
* @property use32BitWorkerProcess Should the Function App run in 32 bit mode, rather than 64 bit mode? Defaults to `true`.
* > **Note:** when using an App Service Plan in the `Free` or `Shared` Tiers `use_32_bit_worker_process` must be set to `true`.
* @property vnetRouteAllEnabled
* @property websocketsEnabled Should WebSockets be enabled?
*/
public data class FunctionAppSiteConfig(
public val alwaysOn: Boolean? = null,
public val appScaleLimit: Int? = null,
public val autoSwapSlotName: String? = null,
public val cors: FunctionAppSiteConfigCors? = null,
public val dotnetFrameworkVersion: String? = null,
public val elasticInstanceMinimum: Int? = null,
public val ftpsState: String? = null,
public val healthCheckPath: String? = null,
public val http2Enabled: Boolean? = null,
public val ipRestrictions: List? = null,
public val javaVersion: String? = null,
public val linuxFxVersion: String? = null,
public val minTlsVersion: String? = null,
public val preWarmedInstanceCount: Int? = null,
public val runtimeScaleMonitoringEnabled: Boolean? = null,
public val scmIpRestrictions: List? = null,
public val scmType: String? = null,
public val scmUseMainIpRestriction: Boolean? = null,
public val use32BitWorkerProcess: Boolean? = null,
public val vnetRouteAllEnabled: Boolean? = null,
public val websocketsEnabled: Boolean? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.appservice.outputs.FunctionAppSiteConfig): FunctionAppSiteConfig = FunctionAppSiteConfig(
alwaysOn = javaType.alwaysOn().map({ args0 -> args0 }).orElse(null),
appScaleLimit = javaType.appScaleLimit().map({ args0 -> args0 }).orElse(null),
autoSwapSlotName = javaType.autoSwapSlotName().map({ args0 -> args0 }).orElse(null),
cors = javaType.cors().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.FunctionAppSiteConfigCors.Companion.toKotlin(args0)
})
}).orElse(null),
dotnetFrameworkVersion = javaType.dotnetFrameworkVersion().map({ args0 -> args0 }).orElse(null),
elasticInstanceMinimum = javaType.elasticInstanceMinimum().map({ args0 -> args0 }).orElse(null),
ftpsState = javaType.ftpsState().map({ args0 -> args0 }).orElse(null),
healthCheckPath = javaType.healthCheckPath().map({ args0 -> args0 }).orElse(null),
http2Enabled = javaType.http2Enabled().map({ args0 -> args0 }).orElse(null),
ipRestrictions = javaType.ipRestrictions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.FunctionAppSiteConfigIpRestriction.Companion.toKotlin(args0)
})
}),
javaVersion = javaType.javaVersion().map({ args0 -> args0 }).orElse(null),
linuxFxVersion = javaType.linuxFxVersion().map({ args0 -> args0 }).orElse(null),
minTlsVersion = javaType.minTlsVersion().map({ args0 -> args0 }).orElse(null),
preWarmedInstanceCount = javaType.preWarmedInstanceCount().map({ args0 -> args0 }).orElse(null),
runtimeScaleMonitoringEnabled = javaType.runtimeScaleMonitoringEnabled().map({ args0 ->
args0
}).orElse(null),
scmIpRestrictions = javaType.scmIpRestrictions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.FunctionAppSiteConfigScmIpRestriction.Companion.toKotlin(args0)
})
}),
scmType = javaType.scmType().map({ args0 -> args0 }).orElse(null),
scmUseMainIpRestriction = javaType.scmUseMainIpRestriction().map({ args0 -> args0 }).orElse(null),
use32BitWorkerProcess = javaType.use32BitWorkerProcess().map({ args0 -> args0 }).orElse(null),
vnetRouteAllEnabled = javaType.vnetRouteAllEnabled().map({ args0 -> args0 }).orElse(null),
websocketsEnabled = javaType.websocketsEnabled().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy