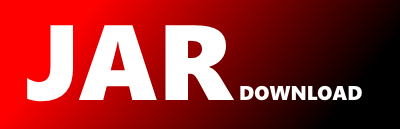
com.pulumi.azure.arckubernetes.kotlin.Cluster.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.arckubernetes.kotlin
import com.pulumi.azure.arckubernetes.kotlin.outputs.ClusterIdentity
import com.pulumi.azure.arckubernetes.kotlin.outputs.ClusterIdentity.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [Cluster].
*/
@PulumiTagMarker
public class ClusterResourceBuilder internal constructor() {
public var name: String? = null
public var args: ClusterArgs = ClusterArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ClusterArgsBuilder.() -> Unit) {
val builder = ClusterArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Cluster {
val builtJavaResource = com.pulumi.azure.arckubernetes.Cluster(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Cluster(builtJavaResource)
}
}
/**
* Manages an Arc Kubernetes Cluster.
* > **Note:** Installing and configuring the Azure Arc Agent on your Kubernetes Cluster to establish connectivity is outside the scope of this document. For more details refer to [Deploy agents to your cluster](https://learn.microsoft.com/en-us/azure/azure-arc/kubernetes/conceptual-agent-overview#deploy-agents-to-your-cluster) and [Connect an existing Kubernetes Cluster](https://learn.microsoft.com/en-us/azure/azure-arc/kubernetes/quickstart-connect-cluster?tabs=azure-cli#connect-an-existing-kubernetes-cluster). If you encounter issues connecting your Kubernetes Cluster to Azure Arc, we'd recommend opening a ticket with Microsoft Support.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* import * as std from "@pulumi/std";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleCluster = new azure.arckubernetes.Cluster("example", {
* name: "example-akcc",
* resourceGroupName: example.name,
* location: "West Europe",
* agentPublicKeyCertificate: std.filebase64({
* input: "testdata/public.cer",
* }).then(invoke => invoke.result),
* identity: {
* type: "SystemAssigned",
* },
* tags: {
* ENV: "Test",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* import pulumi_std as std
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_cluster = azure.arckubernetes.Cluster("example",
* name="example-akcc",
* resource_group_name=example.name,
* location="West Europe",
* agent_public_key_certificate=std.filebase64(input="testdata/public.cer").result,
* identity={
* "type": "SystemAssigned",
* },
* tags={
* "ENV": "Test",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* using Std = Pulumi.Std;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleCluster = new Azure.ArcKubernetes.Cluster("example", new()
* {
* Name = "example-akcc",
* ResourceGroupName = example.Name,
* Location = "West Europe",
* AgentPublicKeyCertificate = Std.Filebase64.Invoke(new()
* {
* Input = "testdata/public.cer",
* }).Apply(invoke => invoke.Result),
* Identity = new Azure.ArcKubernetes.Inputs.ClusterIdentityArgs
* {
* Type = "SystemAssigned",
* },
* Tags =
* {
* { "ENV", "Test" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/arckubernetes"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* invokeFilebase64, err := std.Filebase64(ctx, &std.Filebase64Args{
* Input: "testdata/public.cer",
* }, nil)
* if err != nil {
* return err
* }
* _, err = arckubernetes.NewCluster(ctx, "example", &arckubernetes.ClusterArgs{
* Name: pulumi.String("example-akcc"),
* ResourceGroupName: example.Name,
* Location: pulumi.String("West Europe"),
* AgentPublicKeyCertificate: pulumi.String(invokeFilebase64.Result),
* Identity: &arckubernetes.ClusterIdentityArgs{
* Type: pulumi.String("SystemAssigned"),
* },
* Tags: pulumi.StringMap{
* "ENV": pulumi.String("Test"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.arckubernetes.Cluster;
* import com.pulumi.azure.arckubernetes.ClusterArgs;
* import com.pulumi.azure.arckubernetes.inputs.ClusterIdentityArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleCluster = new Cluster("exampleCluster", ClusterArgs.builder()
* .name("example-akcc")
* .resourceGroupName(example.name())
* .location("West Europe")
* .agentPublicKeyCertificate(StdFunctions.filebase64(Filebase64Args.builder()
* .input("testdata/public.cer")
* .build()).result())
* .identity(ClusterIdentityArgs.builder()
* .type("SystemAssigned")
* .build())
* .tags(Map.of("ENV", "Test"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleCluster:
* type: azure:arckubernetes:Cluster
* name: example
* properties:
* name: example-akcc
* resourceGroupName: ${example.name}
* location: West Europe
* agentPublicKeyCertificate:
* fn::invoke:
* Function: std:filebase64
* Arguments:
* input: testdata/public.cer
* Return: result
* identity:
* type: SystemAssigned
* tags:
* ENV: Test
* ```
*
* > **Note:** An extensive example on connecting the `azure.arckubernetes.Cluster` to an external kubernetes cluster can be found in the `./examples/arckubernetes` directory within the GitHub Repository.
* ## Import
* Arc Kubernetes Cluster can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:arckubernetes/cluster:Cluster example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resourceGroup1/providers/Microsoft.Kubernetes/connectedClusters/cluster1
* ```
*/
public class Cluster internal constructor(
override val javaResource: com.pulumi.azure.arckubernetes.Cluster,
) : KotlinCustomResource(javaResource, ClusterMapper) {
/**
* Specifies the base64-encoded public certificate used by the agent to do the initial handshake to the backend services in Azure. Changing this forces a new Arc Kubernetes Cluster to be created.
*/
public val agentPublicKeyCertificate: Output
get() = javaResource.agentPublicKeyCertificate().applyValue({ args0 -> args0 })
/**
* Version of the agent running on the cluster resource.
*/
public val agentVersion: Output
get() = javaResource.agentVersion().applyValue({ args0 -> args0 })
/**
* The distribution running on this Arc Kubernetes Cluster.
*/
public val distribution: Output
get() = javaResource.distribution().applyValue({ args0 -> args0 })
/**
* An `identity` block as defined below. Changing this forces a new Arc Kubernetes Cluster to be created.
*/
public val identity: Output
get() = javaResource.identity().applyValue({ args0 -> args0.let({ args0 -> toKotlin(args0) }) })
/**
* The infrastructure on which the Arc Kubernetes Cluster is running on.
*/
public val infrastructure: Output
get() = javaResource.infrastructure().applyValue({ args0 -> args0 })
/**
* The Kubernetes version of the cluster resource.
*/
public val kubernetesVersion: Output
get() = javaResource.kubernetesVersion().applyValue({ args0 -> args0 })
/**
* Specifies the Azure Region where the Arc Kubernetes Cluster should exist. Changing this forces a new Arc Kubernetes Cluster to be created.
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* Specifies the name which should be used for this Arc Kubernetes Cluster. Changing this forces a new Arc Kubernetes Cluster to be created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The cluster offering.
*/
public val offering: Output
get() = javaResource.offering().applyValue({ args0 -> args0 })
/**
* Specifies the name of the Resource Group where the Arc Kubernetes Cluster should exist. Changing this forces a new Arc Kubernetes Cluster to be created.
*/
public val resourceGroupName: Output
get() = javaResource.resourceGroupName().applyValue({ args0 -> args0 })
/**
* A mapping of tags which should be assigned to the Arc Kubernetes Cluster.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy