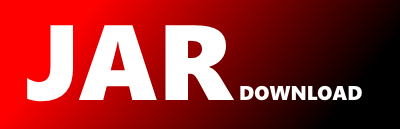
com.pulumi.azure.batch.kotlin.inputs.PoolUserAccountArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.batch.kotlin.inputs
import com.pulumi.azure.batch.inputs.PoolUserAccountArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property elevationLevel The elevation level of the user account. "NonAdmin" - The auto user is a standard user without elevated access. "Admin" - The auto user is a user with elevated access and operates with full Administrator permissions. The default value is nonAdmin.
* @property linuxUserConfigurations The `linux_user_configuration` block defined below is a linux-specific user configuration for the user account. This property is ignored if specified on a Windows pool. If not specified, the user is created with the default options.
* @property name The name of the user account.
* @property password The password for the user account.
* @property windowsUserConfigurations The `windows_user_configuration` block defined below is a windows-specific user configuration for the user account. This property can only be specified if the user is on a Windows pool. If not specified and on a Windows pool, the user is created with the default options.
*/
public data class PoolUserAccountArgs(
public val elevationLevel: Output,
public val linuxUserConfigurations: Output>? =
null,
public val name: Output,
public val password: Output,
public val windowsUserConfigurations: Output>? =
null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.batch.inputs.PoolUserAccountArgs =
com.pulumi.azure.batch.inputs.PoolUserAccountArgs.builder()
.elevationLevel(elevationLevel.applyValue({ args0 -> args0 }))
.linuxUserConfigurations(
linuxUserConfigurations?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.name(name.applyValue({ args0 -> args0 }))
.password(password.applyValue({ args0 -> args0 }))
.windowsUserConfigurations(
windowsUserConfigurations?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [PoolUserAccountArgs].
*/
@PulumiTagMarker
public class PoolUserAccountArgsBuilder internal constructor() {
private var elevationLevel: Output? = null
private var linuxUserConfigurations: Output>? =
null
private var name: Output? = null
private var password: Output? = null
private var windowsUserConfigurations: Output>? =
null
/**
* @param value The elevation level of the user account. "NonAdmin" - The auto user is a standard user without elevated access. "Admin" - The auto user is a user with elevated access and operates with full Administrator permissions. The default value is nonAdmin.
*/
@JvmName("mrovxwhfebycqypp")
public suspend fun elevationLevel(`value`: Output) {
this.elevationLevel = value
}
/**
* @param value The `linux_user_configuration` block defined below is a linux-specific user configuration for the user account. This property is ignored if specified on a Windows pool. If not specified, the user is created with the default options.
*/
@JvmName("twwwcmeybjnircok")
public suspend fun linuxUserConfigurations(`value`: Output>) {
this.linuxUserConfigurations = value
}
@JvmName("aprbqidsrgvasjmg")
public suspend fun linuxUserConfigurations(vararg values: Output) {
this.linuxUserConfigurations = Output.all(values.asList())
}
/**
* @param values The `linux_user_configuration` block defined below is a linux-specific user configuration for the user account. This property is ignored if specified on a Windows pool. If not specified, the user is created with the default options.
*/
@JvmName("uesbavtryovytytk")
public suspend fun linuxUserConfigurations(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy