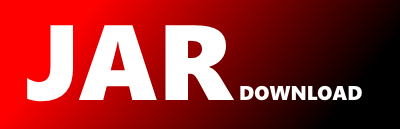
com.pulumi.azure.bot.kotlin.ChannelSmsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.bot.kotlin
import com.pulumi.azure.bot.ChannelSmsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages a SMS integration for a Bot Channel
* > **Note** A bot can only have a single SMS Channel associated with it.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const current = azure.core.getClientConfig({});
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleChannelsRegistration = new azure.bot.ChannelsRegistration("example", {
* name: "example-bcr",
* location: "global",
* resourceGroupName: example.name,
* sku: "F0",
* microsoftAppId: current.then(current => current.clientId),
* });
* const exampleChannelSms = new azure.bot.ChannelSms("example", {
* botName: exampleChannelsRegistration.name,
* location: exampleChannelsRegistration.location,
* resourceGroupName: example.name,
* smsChannelAccountSecurityId: "BG61f7cf5157f439b084e98256409c2815",
* smsChannelAuthToken: "jh8980432610052ed4e29565c5e232f",
* phoneNumber: "+12313803556",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* current = azure.core.get_client_config()
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_channels_registration = azure.bot.ChannelsRegistration("example",
* name="example-bcr",
* location="global",
* resource_group_name=example.name,
* sku="F0",
* microsoft_app_id=current.client_id)
* example_channel_sms = azure.bot.ChannelSms("example",
* bot_name=example_channels_registration.name,
* location=example_channels_registration.location,
* resource_group_name=example.name,
* sms_channel_account_security_id="BG61f7cf5157f439b084e98256409c2815",
* sms_channel_auth_token="jh8980432610052ed4e29565c5e232f",
* phone_number="+12313803556")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var current = Azure.Core.GetClientConfig.Invoke();
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleChannelsRegistration = new Azure.Bot.ChannelsRegistration("example", new()
* {
* Name = "example-bcr",
* Location = "global",
* ResourceGroupName = example.Name,
* Sku = "F0",
* MicrosoftAppId = current.Apply(getClientConfigResult => getClientConfigResult.ClientId),
* });
* var exampleChannelSms = new Azure.Bot.ChannelSms("example", new()
* {
* BotName = exampleChannelsRegistration.Name,
* Location = exampleChannelsRegistration.Location,
* ResourceGroupName = example.Name,
* SmsChannelAccountSecurityId = "BG61f7cf5157f439b084e98256409c2815",
* SmsChannelAuthToken = "jh8980432610052ed4e29565c5e232f",
* PhoneNumber = "+12313803556",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/bot"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* current, err := core.GetClientConfig(ctx, nil, nil)
* if err != nil {
* return err
* }
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleChannelsRegistration, err := bot.NewChannelsRegistration(ctx, "example", &bot.ChannelsRegistrationArgs{
* Name: pulumi.String("example-bcr"),
* Location: pulumi.String("global"),
* ResourceGroupName: example.Name,
* Sku: pulumi.String("F0"),
* MicrosoftAppId: pulumi.String(current.ClientId),
* })
* if err != nil {
* return err
* }
* _, err = bot.NewChannelSms(ctx, "example", &bot.ChannelSmsArgs{
* BotName: exampleChannelsRegistration.Name,
* Location: exampleChannelsRegistration.Location,
* ResourceGroupName: example.Name,
* SmsChannelAccountSecurityId: pulumi.String("BG61f7cf5157f439b084e98256409c2815"),
* SmsChannelAuthToken: pulumi.String("jh8980432610052ed4e29565c5e232f"),
* PhoneNumber: pulumi.String("+12313803556"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.bot.ChannelsRegistration;
* import com.pulumi.azure.bot.ChannelsRegistrationArgs;
* import com.pulumi.azure.bot.ChannelSms;
* import com.pulumi.azure.bot.ChannelSmsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = CoreFunctions.getClientConfig();
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleChannelsRegistration = new ChannelsRegistration("exampleChannelsRegistration", ChannelsRegistrationArgs.builder()
* .name("example-bcr")
* .location("global")
* .resourceGroupName(example.name())
* .sku("F0")
* .microsoftAppId(current.applyValue(getClientConfigResult -> getClientConfigResult.clientId()))
* .build());
* var exampleChannelSms = new ChannelSms("exampleChannelSms", ChannelSmsArgs.builder()
* .botName(exampleChannelsRegistration.name())
* .location(exampleChannelsRegistration.location())
* .resourceGroupName(example.name())
* .smsChannelAccountSecurityId("BG61f7cf5157f439b084e98256409c2815")
* .smsChannelAuthToken("jh8980432610052ed4e29565c5e232f")
* .phoneNumber("+12313803556")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleChannelsRegistration:
* type: azure:bot:ChannelsRegistration
* name: example
* properties:
* name: example-bcr
* location: global
* resourceGroupName: ${example.name}
* sku: F0
* microsoftAppId: ${current.clientId}
* exampleChannelSms:
* type: azure:bot:ChannelSms
* name: example
* properties:
* botName: ${exampleChannelsRegistration.name}
* location: ${exampleChannelsRegistration.location}
* resourceGroupName: ${example.name}
* smsChannelAccountSecurityId: BG61f7cf5157f439b084e98256409c2815
* smsChannelAuthToken: jh8980432610052ed4e29565c5e232f
* phoneNumber: '+12313803556'
* variables:
* current:
* fn::invoke:
* Function: azure:core:getClientConfig
* Arguments: {}
* ```
*
* ## Import
* The SMS Integration for a Bot Channel can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:bot/channelSms:ChannelSms example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.BotService/botServices/botService1/channels/SmsChannel
* ```
* @property botName The name of the Bot Resource this channel will be associated with. Changing this forces a new resource to be created.
* @property location Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
* @property phoneNumber The phone number for the SMS Channel.
* @property resourceGroupName The name of the resource group where the SMS Channel should be created. Changing this forces a new resource to be created.
* @property smsChannelAccountSecurityId The account security identifier (SID) for the SMS Channel.
* @property smsChannelAuthToken The authorization token for the SMS Channel.
*/
public data class ChannelSmsArgs(
public val botName: Output? = null,
public val location: Output? = null,
public val phoneNumber: Output? = null,
public val resourceGroupName: Output? = null,
public val smsChannelAccountSecurityId: Output? = null,
public val smsChannelAuthToken: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.bot.ChannelSmsArgs =
com.pulumi.azure.bot.ChannelSmsArgs.builder()
.botName(botName?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.phoneNumber(phoneNumber?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.smsChannelAccountSecurityId(smsChannelAccountSecurityId?.applyValue({ args0 -> args0 }))
.smsChannelAuthToken(smsChannelAuthToken?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ChannelSmsArgs].
*/
@PulumiTagMarker
public class ChannelSmsArgsBuilder internal constructor() {
private var botName: Output? = null
private var location: Output? = null
private var phoneNumber: Output? = null
private var resourceGroupName: Output? = null
private var smsChannelAccountSecurityId: Output? = null
private var smsChannelAuthToken: Output? = null
/**
* @param value The name of the Bot Resource this channel will be associated with. Changing this forces a new resource to be created.
*/
@JvmName("qdgxaicohidbwknl")
public suspend fun botName(`value`: Output) {
this.botName = value
}
/**
* @param value Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
*/
@JvmName("qiltqeymfgqupeuf")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The phone number for the SMS Channel.
*/
@JvmName("jnvfbuwvrlejmcla")
public suspend fun phoneNumber(`value`: Output) {
this.phoneNumber = value
}
/**
* @param value The name of the resource group where the SMS Channel should be created. Changing this forces a new resource to be created.
*/
@JvmName("cpqsuglrsorivvka")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The account security identifier (SID) for the SMS Channel.
*/
@JvmName("hbhbqniwhilpekjv")
public suspend fun smsChannelAccountSecurityId(`value`: Output) {
this.smsChannelAccountSecurityId = value
}
/**
* @param value The authorization token for the SMS Channel.
*/
@JvmName("njugdkqifoistvhb")
public suspend fun smsChannelAuthToken(`value`: Output) {
this.smsChannelAuthToken = value
}
/**
* @param value The name of the Bot Resource this channel will be associated with. Changing this forces a new resource to be created.
*/
@JvmName("onsdhwsoukmhevyo")
public suspend fun botName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.botName = mapped
}
/**
* @param value Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
*/
@JvmName("rcaxbodqhwscjdsr")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value The phone number for the SMS Channel.
*/
@JvmName("xpmqokuqixrneebu")
public suspend fun phoneNumber(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.phoneNumber = mapped
}
/**
* @param value The name of the resource group where the SMS Channel should be created. Changing this forces a new resource to be created.
*/
@JvmName("cfwddfjxhurirsif")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value The account security identifier (SID) for the SMS Channel.
*/
@JvmName("ufcgxfdbgykfkbtx")
public suspend fun smsChannelAccountSecurityId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.smsChannelAccountSecurityId = mapped
}
/**
* @param value The authorization token for the SMS Channel.
*/
@JvmName("rnpugfdpgsvmiwrf")
public suspend fun smsChannelAuthToken(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.smsChannelAuthToken = mapped
}
internal fun build(): ChannelSmsArgs = ChannelSmsArgs(
botName = botName,
location = location,
phoneNumber = phoneNumber,
resourceGroupName = resourceGroupName,
smsChannelAccountSecurityId = smsChannelAccountSecurityId,
smsChannelAuthToken = smsChannelAuthToken,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy