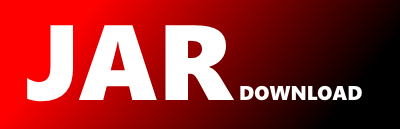
com.pulumi.azure.compute.kotlin.DiskPoolArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.compute.kotlin
import com.pulumi.azure.compute.DiskPoolArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages a Disk Pool.
* !> **Note:** Azure are officially [halting](https://learn.microsoft.com/en-us/azure/azure-vmware/attach-disk-pools-to-azure-vmware-solution-hosts?tabs=azure-cli) the preview of Azure Disk Pools, and it **will not** be made generally available. New customers will not be able to register the Microsoft.StoragePool resource provider on their subscription and deploy new Disk Pools. Existing subscriptions registered with Microsoft.StoragePool may continue to deploy and manage disk pools for the time being.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleVirtualNetwork = new azure.network.VirtualNetwork("example", {
* name: "example-network",
* resourceGroupName: example.name,
* location: example.location,
* addressSpaces: ["10.0.0.0/16"],
* });
* const exampleSubnet = new azure.network.Subnet("example", {
* name: "example-subnet",
* resourceGroupName: exampleVirtualNetwork.resourceGroupName,
* virtualNetworkName: exampleVirtualNetwork.name,
* addressPrefixes: ["10.0.0.0/24"],
* delegations: [{
* name: "diskspool",
* serviceDelegation: {
* actions: ["Microsoft.Network/virtualNetworks/read"],
* name: "Microsoft.StoragePool/diskPools",
* },
* }],
* });
* const exampleDiskPool = new azure.compute.DiskPool("example", {
* name: "example-disk-pool",
* resourceGroupName: example.name,
* location: example.location,
* skuName: "Basic_B1",
* subnetId: exampleSubnet.id,
* zones: ["1"],
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_virtual_network = azure.network.VirtualNetwork("example",
* name="example-network",
* resource_group_name=example.name,
* location=example.location,
* address_spaces=["10.0.0.0/16"])
* example_subnet = azure.network.Subnet("example",
* name="example-subnet",
* resource_group_name=example_virtual_network.resource_group_name,
* virtual_network_name=example_virtual_network.name,
* address_prefixes=["10.0.0.0/24"],
* delegations=[{
* "name": "diskspool",
* "service_delegation": {
* "actions": ["Microsoft.Network/virtualNetworks/read"],
* "name": "Microsoft.StoragePool/diskPools",
* },
* }])
* example_disk_pool = azure.compute.DiskPool("example",
* name="example-disk-pool",
* resource_group_name=example.name,
* location=example.location,
* sku_name="Basic_B1",
* subnet_id=example_subnet.id,
* zones=["1"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleVirtualNetwork = new Azure.Network.VirtualNetwork("example", new()
* {
* Name = "example-network",
* ResourceGroupName = example.Name,
* Location = example.Location,
* AddressSpaces = new[]
* {
* "10.0.0.0/16",
* },
* });
* var exampleSubnet = new Azure.Network.Subnet("example", new()
* {
* Name = "example-subnet",
* ResourceGroupName = exampleVirtualNetwork.ResourceGroupName,
* VirtualNetworkName = exampleVirtualNetwork.Name,
* AddressPrefixes = new[]
* {
* "10.0.0.0/24",
* },
* Delegations = new[]
* {
* new Azure.Network.Inputs.SubnetDelegationArgs
* {
* Name = "diskspool",
* ServiceDelegation = new Azure.Network.Inputs.SubnetDelegationServiceDelegationArgs
* {
* Actions = new[]
* {
* "Microsoft.Network/virtualNetworks/read",
* },
* Name = "Microsoft.StoragePool/diskPools",
* },
* },
* },
* });
* var exampleDiskPool = new Azure.Compute.DiskPool("example", new()
* {
* Name = "example-disk-pool",
* ResourceGroupName = example.Name,
* Location = example.Location,
* SkuName = "Basic_B1",
* SubnetId = exampleSubnet.Id,
* Zones = new[]
* {
* "1",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/compute"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/network"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleVirtualNetwork, err := network.NewVirtualNetwork(ctx, "example", &network.VirtualNetworkArgs{
* Name: pulumi.String("example-network"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* AddressSpaces: pulumi.StringArray{
* pulumi.String("10.0.0.0/16"),
* },
* })
* if err != nil {
* return err
* }
* exampleSubnet, err := network.NewSubnet(ctx, "example", &network.SubnetArgs{
* Name: pulumi.String("example-subnet"),
* ResourceGroupName: exampleVirtualNetwork.ResourceGroupName,
* VirtualNetworkName: exampleVirtualNetwork.Name,
* AddressPrefixes: pulumi.StringArray{
* pulumi.String("10.0.0.0/24"),
* },
* Delegations: network.SubnetDelegationArray{
* &network.SubnetDelegationArgs{
* Name: pulumi.String("diskspool"),
* ServiceDelegation: &network.SubnetDelegationServiceDelegationArgs{
* Actions: pulumi.StringArray{
* pulumi.String("Microsoft.Network/virtualNetworks/read"),
* },
* Name: pulumi.String("Microsoft.StoragePool/diskPools"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = compute.NewDiskPool(ctx, "example", &compute.DiskPoolArgs{
* Name: pulumi.String("example-disk-pool"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* SkuName: pulumi.String("Basic_B1"),
* SubnetId: exampleSubnet.ID(),
* Zones: pulumi.StringArray{
* pulumi.String("1"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.network.VirtualNetwork;
* import com.pulumi.azure.network.VirtualNetworkArgs;
* import com.pulumi.azure.network.Subnet;
* import com.pulumi.azure.network.SubnetArgs;
* import com.pulumi.azure.network.inputs.SubnetDelegationArgs;
* import com.pulumi.azure.network.inputs.SubnetDelegationServiceDelegationArgs;
* import com.pulumi.azure.compute.DiskPool;
* import com.pulumi.azure.compute.DiskPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleVirtualNetwork = new VirtualNetwork("exampleVirtualNetwork", VirtualNetworkArgs.builder()
* .name("example-network")
* .resourceGroupName(example.name())
* .location(example.location())
* .addressSpaces("10.0.0.0/16")
* .build());
* var exampleSubnet = new Subnet("exampleSubnet", SubnetArgs.builder()
* .name("example-subnet")
* .resourceGroupName(exampleVirtualNetwork.resourceGroupName())
* .virtualNetworkName(exampleVirtualNetwork.name())
* .addressPrefixes("10.0.0.0/24")
* .delegations(SubnetDelegationArgs.builder()
* .name("diskspool")
* .serviceDelegation(SubnetDelegationServiceDelegationArgs.builder()
* .actions("Microsoft.Network/virtualNetworks/read")
* .name("Microsoft.StoragePool/diskPools")
* .build())
* .build())
* .build());
* var exampleDiskPool = new DiskPool("exampleDiskPool", DiskPoolArgs.builder()
* .name("example-disk-pool")
* .resourceGroupName(example.name())
* .location(example.location())
* .skuName("Basic_B1")
* .subnetId(exampleSubnet.id())
* .zones("1")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleVirtualNetwork:
* type: azure:network:VirtualNetwork
* name: example
* properties:
* name: example-network
* resourceGroupName: ${example.name}
* location: ${example.location}
* addressSpaces:
* - 10.0.0.0/16
* exampleSubnet:
* type: azure:network:Subnet
* name: example
* properties:
* name: example-subnet
* resourceGroupName: ${exampleVirtualNetwork.resourceGroupName}
* virtualNetworkName: ${exampleVirtualNetwork.name}
* addressPrefixes:
* - 10.0.0.0/24
* delegations:
* - name: diskspool
* serviceDelegation:
* actions:
* - Microsoft.Network/virtualNetworks/read
* name: Microsoft.StoragePool/diskPools
* exampleDiskPool:
* type: azure:compute:DiskPool
* name: example
* properties:
* name: example-disk-pool
* resourceGroupName: ${example.name}
* location: ${example.location}
* skuName: Basic_B1
* subnetId: ${exampleSubnet.id}
* zones:
* - '1'
* ```
*
* ## Import
* Disk Pools can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:compute/diskPool:DiskPool example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resGroup1/providers/Microsoft.StoragePool/diskPools/diskPool1
* ```
* @property location The Azure Region where the Disk Pool should exist. Changing this forces a new Disk Pool to be created.
* @property name The name of the Disk Pool. Changing this forces a new Disk Pool to be created.
* @property resourceGroupName The name of the Resource Group where the Disk Pool should exist. Changing this forces a new Disk Pool to be created.
* @property skuName The SKU of the Disk Pool. Possible values are `Basic_B1`, `Standard_S1` and `Premium_P1`. Changing this forces a new Disk Pool to be created.
* @property subnetId The ID of the Subnet where the Disk Pool should be created. Changing this forces a new Disk Pool to be created.
* @property tags A mapping of tags which should be assigned to the Disk Pool.
* @property zones Specifies a list of Availability Zones in which this Disk Pool should be located. Changing this forces a new Disk Pool to be created.
*/
public data class DiskPoolArgs(
public val location: Output? = null,
public val name: Output? = null,
public val resourceGroupName: Output? = null,
public val skuName: Output? = null,
public val subnetId: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy