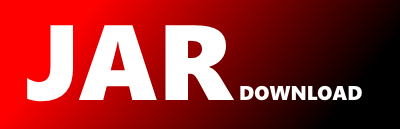
com.pulumi.azure.compute.kotlin.ManagedDiskSasToken.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [ManagedDiskSasToken].
*/
@PulumiTagMarker
public class ManagedDiskSasTokenResourceBuilder internal constructor() {
public var name: String? = null
public var args: ManagedDiskSasTokenArgs = ManagedDiskSasTokenArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ManagedDiskSasTokenArgsBuilder.() -> Unit) {
val builder = ManagedDiskSasTokenArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ManagedDiskSasToken {
val builtJavaResource = com.pulumi.azure.compute.ManagedDiskSasToken(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ManagedDiskSasToken(builtJavaResource)
}
}
/**
* Manages a Disk SAS Token.
* Use this resource to obtain a Shared Access Signature (SAS Token) for an existing Managed Disk.
* Shared access signatures allow fine-grained, ephemeral access control to various aspects of Managed Disk similar to blob/storage account container.
* With the help of this resource, data from the disk can be copied from managed disk to a storage blob or to some other system without the need of azcopy.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const test = new azure.core.ResourceGroup("test", {
* name: "testrg",
* location: "West Europe",
* });
* const testManagedDisk = new azure.compute.ManagedDisk("test", {
* name: "tst-disk-export",
* location: test.location,
* resourceGroupName: test.name,
* storageAccountType: "Standard_LRS",
* createOption: "Empty",
* diskSizeGb: 1,
* });
* const testManagedDiskSasToken = new azure.compute.ManagedDiskSasToken("test", {
* managedDiskId: testManagedDisk.id,
* durationInSeconds: 300,
* accessLevel: "Read",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* test = azure.core.ResourceGroup("test",
* name="testrg",
* location="West Europe")
* test_managed_disk = azure.compute.ManagedDisk("test",
* name="tst-disk-export",
* location=test.location,
* resource_group_name=test.name,
* storage_account_type="Standard_LRS",
* create_option="Empty",
* disk_size_gb=1)
* test_managed_disk_sas_token = azure.compute.ManagedDiskSasToken("test",
* managed_disk_id=test_managed_disk.id,
* duration_in_seconds=300,
* access_level="Read")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var test = new Azure.Core.ResourceGroup("test", new()
* {
* Name = "testrg",
* Location = "West Europe",
* });
* var testManagedDisk = new Azure.Compute.ManagedDisk("test", new()
* {
* Name = "tst-disk-export",
* Location = test.Location,
* ResourceGroupName = test.Name,
* StorageAccountType = "Standard_LRS",
* CreateOption = "Empty",
* DiskSizeGb = 1,
* });
* var testManagedDiskSasToken = new Azure.Compute.ManagedDiskSasToken("test", new()
* {
* ManagedDiskId = testManagedDisk.Id,
* DurationInSeconds = 300,
* AccessLevel = "Read",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/compute"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* test, err := core.NewResourceGroup(ctx, "test", &core.ResourceGroupArgs{
* Name: pulumi.String("testrg"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* testManagedDisk, err := compute.NewManagedDisk(ctx, "test", &compute.ManagedDiskArgs{
* Name: pulumi.String("tst-disk-export"),
* Location: test.Location,
* ResourceGroupName: test.Name,
* StorageAccountType: pulumi.String("Standard_LRS"),
* CreateOption: pulumi.String("Empty"),
* DiskSizeGb: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewManagedDiskSasToken(ctx, "test", &compute.ManagedDiskSasTokenArgs{
* ManagedDiskId: testManagedDisk.ID(),
* DurationInSeconds: pulumi.Int(300),
* AccessLevel: pulumi.String("Read"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.compute.ManagedDisk;
* import com.pulumi.azure.compute.ManagedDiskArgs;
* import com.pulumi.azure.compute.ManagedDiskSasToken;
* import com.pulumi.azure.compute.ManagedDiskSasTokenArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new ResourceGroup("test", ResourceGroupArgs.builder()
* .name("testrg")
* .location("West Europe")
* .build());
* var testManagedDisk = new ManagedDisk("testManagedDisk", ManagedDiskArgs.builder()
* .name("tst-disk-export")
* .location(test.location())
* .resourceGroupName(test.name())
* .storageAccountType("Standard_LRS")
* .createOption("Empty")
* .diskSizeGb("1")
* .build());
* var testManagedDiskSasToken = new ManagedDiskSasToken("testManagedDiskSasToken", ManagedDiskSasTokenArgs.builder()
* .managedDiskId(testManagedDisk.id())
* .durationInSeconds(300)
* .accessLevel("Read")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: azure:core:ResourceGroup
* properties:
* name: testrg
* location: West Europe
* testManagedDisk:
* type: azure:compute:ManagedDisk
* name: test
* properties:
* name: tst-disk-export
* location: ${test.location}
* resourceGroupName: ${test.name}
* storageAccountType: Standard_LRS
* createOption: Empty
* diskSizeGb: '1'
* testManagedDiskSasToken:
* type: azure:compute:ManagedDiskSasToken
* name: test
* properties:
* managedDiskId: ${testManagedDisk.id}
* durationInSeconds: 300
* accessLevel: Read
* ```
*
* ## Import
* Disk SAS Token can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:compute/managedDiskSasToken:ManagedDiskSasToken example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/mygroup1/providers/Microsoft.Compute/disks/manageddisk1
* ```
*/
public class ManagedDiskSasToken internal constructor(
override val javaResource: com.pulumi.azure.compute.ManagedDiskSasToken,
) : KotlinCustomResource(javaResource, ManagedDiskSasTokenMapper) {
/**
* The level of access required on the disk. Supported are Read, Write. Changing this forces a new resource to be created.
* Refer to the [SAS creation reference from Azure](https://docs.microsoft.com/rest/api/compute/disks/grant-access)
* for additional details on the fields above.
*/
public val accessLevel: Output
get() = javaResource.accessLevel().applyValue({ args0 -> args0 })
/**
* The duration for which the export should be allowed. Should be between 30 & 4294967295 seconds. Changing this forces a new resource to be created.
*/
public val durationInSeconds: Output
get() = javaResource.durationInSeconds().applyValue({ args0 -> args0 })
/**
* The ID of an existing Managed Disk which should be exported. Changing this forces a new resource to be created.
*/
public val managedDiskId: Output
get() = javaResource.managedDiskId().applyValue({ args0 -> args0 })
/**
* The computed Shared Access Signature (SAS) of the Managed Disk.
*/
public val sasUrl: Output
get() = javaResource.sasUrl().applyValue({ args0 -> args0 })
}
public object ManagedDiskSasTokenMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azure.compute.ManagedDiskSasToken::class == javaResource::class
override fun map(javaResource: Resource): ManagedDiskSasToken = ManagedDiskSasToken(
javaResource
as com.pulumi.azure.compute.ManagedDiskSasToken,
)
}
/**
* @see [ManagedDiskSasToken].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ManagedDiskSasToken].
*/
public suspend fun managedDiskSasToken(
name: String,
block: suspend ManagedDiskSasTokenResourceBuilder.() -> Unit,
): ManagedDiskSasToken {
val builder = ManagedDiskSasTokenResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ManagedDiskSasToken].
* @param name The _unique_ name of the resulting resource.
*/
public fun managedDiskSasToken(name: String): ManagedDiskSasToken {
val builder = ManagedDiskSasTokenResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy