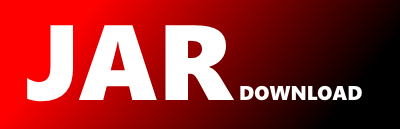
com.pulumi.azure.compute.kotlin.inputs.OrchestratedVirtualMachineScaleSetOsProfileLinuxConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.compute.kotlin.inputs
import com.pulumi.azure.compute.inputs.OrchestratedVirtualMachineScaleSetOsProfileLinuxConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property adminPassword The Password which should be used for the local-administrator on this Virtual Machine. Changing this forces a new resource to be created.
* @property adminSshKeys A `admin_ssh_key` block as documented below.
* @property adminUsername The username of the local administrator on each Virtual Machine Scale Set instance. Changing this forces a new resource to be created.
* @property computerNamePrefix The prefix which should be used for the name of the Virtual Machines in this Scale Set. If unspecified this defaults to the value for the name field. If the value of the name field is not a valid `computer_name_prefix`, then you must specify `computer_name_prefix`. Changing this forces a new resource to be created.
* @property disablePasswordAuthentication When an `admin_password` is specified `disable_password_authentication` must be set to `false`. Defaults to `true`.
* > **Note:** Either `admin_password` or `admin_ssh_key` must be specified.
* @property patchAssessmentMode Specifies the mode of VM Guest Patching for the virtual machines that are associated to the Virtual Machine Scale Set. Possible values are `AutomaticByPlatform` or `ImageDefault`. Defaults to `ImageDefault`.
* > **Note:** If the `patch_assessment_mode` is set to `AutomaticByPlatform` then the `provision_vm_agent` field must be set to `true`.
* @property patchMode Specifies the mode of in-guest patching of this Windows Virtual Machine. Possible values are `ImageDefault` or `AutomaticByPlatform`. Defaults to `ImageDefault`. For more information on patch modes please see the [product documentation](https://docs.microsoft.com/azure/virtual-machines/automatic-vm-guest-patching#patch-orchestration-modes).
* > **Note:** If `patch_mode` is set to `AutomaticByPlatform` the `provision_vm_agent` must be set to `true` and the `extension` must contain at least one application health extension. An example of how to correctly configure a Virtual Machine Scale Set to provision a Linux Virtual Machine with Automatic VM Guest Patching enabled can be found in the `./examples/orchestrated-vm-scale-set/automatic-vm-guest-patching` directory within the GitHub Repository.
* @property provisionVmAgent Should the Azure VM Agent be provisioned on each Virtual Machine in the Scale Set? Defaults to `true`. Changing this value forces a new resource to be created.
* @property secrets One or more `secret` blocks as defined below.
*/
public data class OrchestratedVirtualMachineScaleSetOsProfileLinuxConfigurationArgs(
public val adminPassword: Output? = null,
public val adminSshKeys: Output>? =
null,
public val adminUsername: Output,
public val computerNamePrefix: Output? = null,
public val disablePasswordAuthentication: Output? = null,
public val patchAssessmentMode: Output? = null,
public val patchMode: Output? = null,
public val provisionVmAgent: Output? = null,
public val secrets: Output>? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azure.compute.inputs.OrchestratedVirtualMachineScaleSetOsProfileLinuxConfigurationArgs =
com.pulumi.azure.compute.inputs.OrchestratedVirtualMachineScaleSetOsProfileLinuxConfigurationArgs.builder()
.adminPassword(adminPassword?.applyValue({ args0 -> args0 }))
.adminSshKeys(
adminSshKeys?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.adminUsername(adminUsername.applyValue({ args0 -> args0 }))
.computerNamePrefix(computerNamePrefix?.applyValue({ args0 -> args0 }))
.disablePasswordAuthentication(disablePasswordAuthentication?.applyValue({ args0 -> args0 }))
.patchAssessmentMode(patchAssessmentMode?.applyValue({ args0 -> args0 }))
.patchMode(patchMode?.applyValue({ args0 -> args0 }))
.provisionVmAgent(provisionVmAgent?.applyValue({ args0 -> args0 }))
.secrets(
secrets?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [OrchestratedVirtualMachineScaleSetOsProfileLinuxConfigurationArgs].
*/
@PulumiTagMarker
public class OrchestratedVirtualMachineScaleSetOsProfileLinuxConfigurationArgsBuilder internal constructor() {
private var adminPassword: Output? = null
private var adminSshKeys:
Output>? =
null
private var adminUsername: Output? = null
private var computerNamePrefix: Output? = null
private var disablePasswordAuthentication: Output? = null
private var patchAssessmentMode: Output? = null
private var patchMode: Output? = null
private var provisionVmAgent: Output? = null
private var secrets:
Output>? = null
/**
* @param value The Password which should be used for the local-administrator on this Virtual Machine. Changing this forces a new resource to be created.
*/
@JvmName("cpahjaakrwnmbnic")
public suspend fun adminPassword(`value`: Output) {
this.adminPassword = value
}
/**
* @param value A `admin_ssh_key` block as documented below.
*/
@JvmName("dbulwbwnrmiicvbx")
public suspend fun adminSshKeys(`value`: Output>) {
this.adminSshKeys = value
}
@JvmName("jelfadenjuuqkkvq")
public suspend fun adminSshKeys(vararg values: Output) {
this.adminSshKeys = Output.all(values.asList())
}
/**
* @param values A `admin_ssh_key` block as documented below.
*/
@JvmName("okqffjecrgoygago")
public suspend fun adminSshKeys(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy