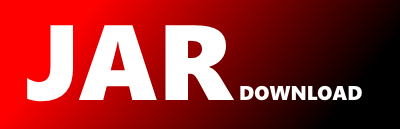
com.pulumi.azure.compute.kotlin.inputs.ScaleSetNetworkProfileIpConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.compute.kotlin.inputs
import com.pulumi.azure.compute.inputs.ScaleSetNetworkProfileIpConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property applicationGatewayBackendAddressPoolIds Specifies an array of references to backend address pools of application gateways. A scale set can reference backend address pools of multiple application gateways. Multiple scale sets can use the same application gateway.
* @property applicationSecurityGroupIds Specifies up to `20` application security group IDs.
* @property loadBalancerBackendAddressPoolIds Specifies an array of references to backend address pools of load balancers. A scale set can reference backend address pools of one public and one internal load balancer. Multiple scale sets cannot use the same load balancer.
* > **NOTE:** When using this field you'll also need to configure a Rule for the Load Balancer, and use a `depends_on` between this resource and the Load Balancer Rule.
* @property loadBalancerInboundNatRulesIds Specifies an array of references to inbound NAT pools for load balancers. A scale set can reference inbound NAT pools of one public and one internal load balancer. Multiple scale sets cannot use the same load balancer.
* > **NOTE:** When using this field you'll also need to configure a Rule for the Load Balancer, and use a `depends_on` between this resource and the Load Balancer Rule.
* @property name Specifies name of the IP configuration.
* @property primary Specifies if this ip_configuration is the primary one.
* @property publicIpAddressConfiguration Describes a virtual machines scale set IP Configuration's PublicIPAddress configuration. The `public_ip_address_configuration` block is documented below.
* @property subnetId Specifies the identifier of the subnet.
*/
public data class ScaleSetNetworkProfileIpConfigurationArgs(
public val applicationGatewayBackendAddressPoolIds: Output>? = null,
public val applicationSecurityGroupIds: Output>? = null,
public val loadBalancerBackendAddressPoolIds: Output>? = null,
public val loadBalancerInboundNatRulesIds: Output>? = null,
public val name: Output,
public val primary: Output,
public val publicIpAddressConfiguration: Output? = null,
public val subnetId: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.compute.inputs.ScaleSetNetworkProfileIpConfigurationArgs =
com.pulumi.azure.compute.inputs.ScaleSetNetworkProfileIpConfigurationArgs.builder()
.applicationGatewayBackendAddressPoolIds(
applicationGatewayBackendAddressPoolIds?.applyValue({ args0 ->
args0.map({ args0 -> args0 })
}),
)
.applicationSecurityGroupIds(
applicationSecurityGroupIds?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
)
.loadBalancerBackendAddressPoolIds(
loadBalancerBackendAddressPoolIds?.applyValue({ args0 ->
args0.map({ args0 -> args0 })
}),
)
.loadBalancerInboundNatRulesIds(
loadBalancerInboundNatRulesIds?.applyValue({ args0 ->
args0.map({ args0 -> args0 })
}),
)
.name(name.applyValue({ args0 -> args0 }))
.primary(primary.applyValue({ args0 -> args0 }))
.publicIpAddressConfiguration(
publicIpAddressConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.subnetId(subnetId.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ScaleSetNetworkProfileIpConfigurationArgs].
*/
@PulumiTagMarker
public class ScaleSetNetworkProfileIpConfigurationArgsBuilder internal constructor() {
private var applicationGatewayBackendAddressPoolIds: Output>? = null
private var applicationSecurityGroupIds: Output>? = null
private var loadBalancerBackendAddressPoolIds: Output>? = null
private var loadBalancerInboundNatRulesIds: Output>? = null
private var name: Output? = null
private var primary: Output? = null
private var publicIpAddressConfiguration:
Output? = null
private var subnetId: Output? = null
/**
* @param value Specifies an array of references to backend address pools of application gateways. A scale set can reference backend address pools of multiple application gateways. Multiple scale sets can use the same application gateway.
*/
@JvmName("koxwffytpaclnytl")
public suspend fun applicationGatewayBackendAddressPoolIds(`value`: Output>) {
this.applicationGatewayBackendAddressPoolIds = value
}
@JvmName("vxwduqcrskegdrvq")
public suspend fun applicationGatewayBackendAddressPoolIds(vararg values: Output) {
this.applicationGatewayBackendAddressPoolIds = Output.all(values.asList())
}
/**
* @param values Specifies an array of references to backend address pools of application gateways. A scale set can reference backend address pools of multiple application gateways. Multiple scale sets can use the same application gateway.
*/
@JvmName("rwcwkdercrknmwuk")
public suspend fun applicationGatewayBackendAddressPoolIds(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy