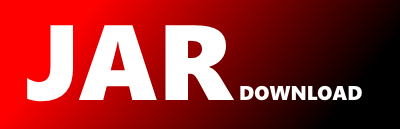
com.pulumi.azure.compute.kotlin.inputs.WindowsVirtualMachineGalleryApplicationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.compute.kotlin.inputs
import com.pulumi.azure.compute.inputs.WindowsVirtualMachineGalleryApplicationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property automaticUpgradeEnabled Specifies whether the version will be automatically updated for the VM when a new Gallery Application version is available in PIR/SIG. Defaults to `false`.
* @property configurationBlobUri Specifies the URI to an Azure Blob that will replace the default configuration for the package if provided.
* @property order Specifies the order in which the packages have to be installed. Possible values are between `0` and `2147483647`. Defaults to `0`.
* @property tag Specifies a passthrough value for more generic context. This field can be any valid `string` value.
* @property treatFailureAsDeploymentFailureEnabled Specifies whether any failure for any operation in the VmApplication will fail the deployment of the VM. Defaults to `false`.
* @property versionId Specifies the Gallery Application Version resource ID.
*/
public data class WindowsVirtualMachineGalleryApplicationArgs(
public val automaticUpgradeEnabled: Output? = null,
public val configurationBlobUri: Output? = null,
public val order: Output? = null,
public val tag: Output? = null,
public val treatFailureAsDeploymentFailureEnabled: Output? = null,
public val versionId: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.compute.inputs.WindowsVirtualMachineGalleryApplicationArgs = com.pulumi.azure.compute.inputs.WindowsVirtualMachineGalleryApplicationArgs.builder()
.automaticUpgradeEnabled(automaticUpgradeEnabled?.applyValue({ args0 -> args0 }))
.configurationBlobUri(configurationBlobUri?.applyValue({ args0 -> args0 }))
.order(order?.applyValue({ args0 -> args0 }))
.tag(tag?.applyValue({ args0 -> args0 }))
.treatFailureAsDeploymentFailureEnabled(
treatFailureAsDeploymentFailureEnabled?.applyValue({ args0 ->
args0
}),
)
.versionId(versionId.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [WindowsVirtualMachineGalleryApplicationArgs].
*/
@PulumiTagMarker
public class WindowsVirtualMachineGalleryApplicationArgsBuilder internal constructor() {
private var automaticUpgradeEnabled: Output? = null
private var configurationBlobUri: Output? = null
private var order: Output? = null
private var tag: Output? = null
private var treatFailureAsDeploymentFailureEnabled: Output? = null
private var versionId: Output? = null
/**
* @param value Specifies whether the version will be automatically updated for the VM when a new Gallery Application version is available in PIR/SIG. Defaults to `false`.
*/
@JvmName("lodrtsptndiwmfvq")
public suspend fun automaticUpgradeEnabled(`value`: Output) {
this.automaticUpgradeEnabled = value
}
/**
* @param value Specifies the URI to an Azure Blob that will replace the default configuration for the package if provided.
*/
@JvmName("sjkbupkfugrfboii")
public suspend fun configurationBlobUri(`value`: Output) {
this.configurationBlobUri = value
}
/**
* @param value Specifies the order in which the packages have to be installed. Possible values are between `0` and `2147483647`. Defaults to `0`.
*/
@JvmName("htvesntnrstynbqa")
public suspend fun order(`value`: Output) {
this.order = value
}
/**
* @param value Specifies a passthrough value for more generic context. This field can be any valid `string` value.
*/
@JvmName("lygencrnkaopllai")
public suspend fun tag(`value`: Output) {
this.tag = value
}
/**
* @param value Specifies whether any failure for any operation in the VmApplication will fail the deployment of the VM. Defaults to `false`.
*/
@JvmName("okwubatwnhshhiqf")
public suspend fun treatFailureAsDeploymentFailureEnabled(`value`: Output) {
this.treatFailureAsDeploymentFailureEnabled = value
}
/**
* @param value Specifies the Gallery Application Version resource ID.
*/
@JvmName("jkdpljgqmjsnmlex")
public suspend fun versionId(`value`: Output) {
this.versionId = value
}
/**
* @param value Specifies whether the version will be automatically updated for the VM when a new Gallery Application version is available in PIR/SIG. Defaults to `false`.
*/
@JvmName("ulaxfqakkdmjuksg")
public suspend fun automaticUpgradeEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.automaticUpgradeEnabled = mapped
}
/**
* @param value Specifies the URI to an Azure Blob that will replace the default configuration for the package if provided.
*/
@JvmName("sipastwkpwvifrlg")
public suspend fun configurationBlobUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.configurationBlobUri = mapped
}
/**
* @param value Specifies the order in which the packages have to be installed. Possible values are between `0` and `2147483647`. Defaults to `0`.
*/
@JvmName("jcpeohbogrlvrmol")
public suspend fun order(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.order = mapped
}
/**
* @param value Specifies a passthrough value for more generic context. This field can be any valid `string` value.
*/
@JvmName("tuflaiqxejjjfwvb")
public suspend fun tag(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tag = mapped
}
/**
* @param value Specifies whether any failure for any operation in the VmApplication will fail the deployment of the VM. Defaults to `false`.
*/
@JvmName("nxqobgaayamhnfcl")
public suspend fun treatFailureAsDeploymentFailureEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.treatFailureAsDeploymentFailureEnabled = mapped
}
/**
* @param value Specifies the Gallery Application Version resource ID.
*/
@JvmName("ofdgcgotmrrpkesb")
public suspend fun versionId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.versionId = mapped
}
internal fun build(): WindowsVirtualMachineGalleryApplicationArgs =
WindowsVirtualMachineGalleryApplicationArgs(
automaticUpgradeEnabled = automaticUpgradeEnabled,
configurationBlobUri = configurationBlobUri,
order = order,
tag = tag,
treatFailureAsDeploymentFailureEnabled = treatFailureAsDeploymentFailureEnabled,
versionId = versionId ?: throw PulumiNullFieldException("versionId"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy