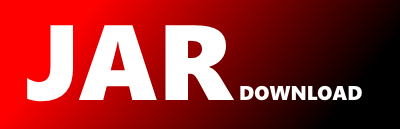
com.pulumi.azure.containerservice.kotlin.FluxConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin
import com.pulumi.azure.containerservice.FluxConfigurationArgs.builder
import com.pulumi.azure.containerservice.kotlin.inputs.FluxConfigurationBlobStorageArgs
import com.pulumi.azure.containerservice.kotlin.inputs.FluxConfigurationBlobStorageArgsBuilder
import com.pulumi.azure.containerservice.kotlin.inputs.FluxConfigurationBucketArgs
import com.pulumi.azure.containerservice.kotlin.inputs.FluxConfigurationBucketArgsBuilder
import com.pulumi.azure.containerservice.kotlin.inputs.FluxConfigurationGitRepositoryArgs
import com.pulumi.azure.containerservice.kotlin.inputs.FluxConfigurationGitRepositoryArgsBuilder
import com.pulumi.azure.containerservice.kotlin.inputs.FluxConfigurationKustomizationArgs
import com.pulumi.azure.containerservice.kotlin.inputs.FluxConfigurationKustomizationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages a Kubernetes Flux Configuration.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleKubernetesCluster = new azure.containerservice.KubernetesCluster("example", {
* name: "example-aks",
* location: "West Europe",
* resourceGroupName: example.name,
* dnsPrefix: "example-aks",
* defaultNodePool: {
* name: "default",
* nodeCount: 1,
* vmSize: "Standard_DS2_v2",
* },
* identity: {
* type: "SystemAssigned",
* },
* });
* const exampleKubernetesClusterExtension = new azure.containerservice.KubernetesClusterExtension("example", {
* name: "example-ext",
* clusterId: test.id,
* extensionType: "microsoft.flux",
* });
* const exampleFluxConfiguration = new azure.containerservice.FluxConfiguration("example", {
* name: "example-fc",
* clusterId: test.id,
* namespace: "flux",
* gitRepository: {
* url: "https://github.com/Azure/arc-k8s-demo",
* referenceType: "branch",
* referenceValue: "main",
* },
* kustomizations: [{
* name: "kustomization-1",
* }],
* }, {
* dependsOn: [exampleKubernetesClusterExtension],
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_kubernetes_cluster = azure.containerservice.KubernetesCluster("example",
* name="example-aks",
* location="West Europe",
* resource_group_name=example.name,
* dns_prefix="example-aks",
* default_node_pool={
* "name": "default",
* "node_count": 1,
* "vm_size": "Standard_DS2_v2",
* },
* identity={
* "type": "SystemAssigned",
* })
* example_kubernetes_cluster_extension = azure.containerservice.KubernetesClusterExtension("example",
* name="example-ext",
* cluster_id=test["id"],
* extension_type="microsoft.flux")
* example_flux_configuration = azure.containerservice.FluxConfiguration("example",
* name="example-fc",
* cluster_id=test["id"],
* namespace="flux",
* git_repository={
* "url": "https://github.com/Azure/arc-k8s-demo",
* "reference_type": "branch",
* "reference_value": "main",
* },
* kustomizations=[{
* "name": "kustomization-1",
* }],
* opts = pulumi.ResourceOptions(depends_on=[example_kubernetes_cluster_extension]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleKubernetesCluster = new Azure.ContainerService.KubernetesCluster("example", new()
* {
* Name = "example-aks",
* Location = "West Europe",
* ResourceGroupName = example.Name,
* DnsPrefix = "example-aks",
* DefaultNodePool = new Azure.ContainerService.Inputs.KubernetesClusterDefaultNodePoolArgs
* {
* Name = "default",
* NodeCount = 1,
* VmSize = "Standard_DS2_v2",
* },
* Identity = new Azure.ContainerService.Inputs.KubernetesClusterIdentityArgs
* {
* Type = "SystemAssigned",
* },
* });
* var exampleKubernetesClusterExtension = new Azure.ContainerService.KubernetesClusterExtension("example", new()
* {
* Name = "example-ext",
* ClusterId = test.Id,
* ExtensionType = "microsoft.flux",
* });
* var exampleFluxConfiguration = new Azure.ContainerService.FluxConfiguration("example", new()
* {
* Name = "example-fc",
* ClusterId = test.Id,
* Namespace = "flux",
* GitRepository = new Azure.ContainerService.Inputs.FluxConfigurationGitRepositoryArgs
* {
* Url = "https://github.com/Azure/arc-k8s-demo",
* ReferenceType = "branch",
* ReferenceValue = "main",
* },
* Kustomizations = new[]
* {
* new Azure.ContainerService.Inputs.FluxConfigurationKustomizationArgs
* {
* Name = "kustomization-1",
* },
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* exampleKubernetesClusterExtension,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* _, err = containerservice.NewKubernetesCluster(ctx, "example", &containerservice.KubernetesClusterArgs{
* Name: pulumi.String("example-aks"),
* Location: pulumi.String("West Europe"),
* ResourceGroupName: example.Name,
* DnsPrefix: pulumi.String("example-aks"),
* DefaultNodePool: &containerservice.KubernetesClusterDefaultNodePoolArgs{
* Name: pulumi.String("default"),
* NodeCount: pulumi.Int(1),
* VmSize: pulumi.String("Standard_DS2_v2"),
* },
* Identity: &containerservice.KubernetesClusterIdentityArgs{
* Type: pulumi.String("SystemAssigned"),
* },
* })
* if err != nil {
* return err
* }
* exampleKubernetesClusterExtension, err := containerservice.NewKubernetesClusterExtension(ctx, "example", &containerservice.KubernetesClusterExtensionArgs{
* Name: pulumi.String("example-ext"),
* ClusterId: pulumi.Any(test.Id),
* ExtensionType: pulumi.String("microsoft.flux"),
* })
* if err != nil {
* return err
* }
* _, err = containerservice.NewFluxConfiguration(ctx, "example", &containerservice.FluxConfigurationArgs{
* Name: pulumi.String("example-fc"),
* ClusterId: pulumi.Any(test.Id),
* Namespace: pulumi.String("flux"),
* GitRepository: &containerservice.FluxConfigurationGitRepositoryArgs{
* Url: pulumi.String("https://github.com/Azure/arc-k8s-demo"),
* ReferenceType: pulumi.String("branch"),
* ReferenceValue: pulumi.String("main"),
* },
* Kustomizations: containerservice.FluxConfigurationKustomizationArray{
* &containerservice.FluxConfigurationKustomizationArgs{
* Name: pulumi.String("kustomization-1"),
* },
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* exampleKubernetesClusterExtension,
* }))
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.containerservice.KubernetesCluster;
* import com.pulumi.azure.containerservice.KubernetesClusterArgs;
* import com.pulumi.azure.containerservice.inputs.KubernetesClusterDefaultNodePoolArgs;
* import com.pulumi.azure.containerservice.inputs.KubernetesClusterIdentityArgs;
* import com.pulumi.azure.containerservice.KubernetesClusterExtension;
* import com.pulumi.azure.containerservice.KubernetesClusterExtensionArgs;
* import com.pulumi.azure.containerservice.FluxConfiguration;
* import com.pulumi.azure.containerservice.FluxConfigurationArgs;
* import com.pulumi.azure.containerservice.inputs.FluxConfigurationGitRepositoryArgs;
* import com.pulumi.azure.containerservice.inputs.FluxConfigurationKustomizationArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleKubernetesCluster = new KubernetesCluster("exampleKubernetesCluster", KubernetesClusterArgs.builder()
* .name("example-aks")
* .location("West Europe")
* .resourceGroupName(example.name())
* .dnsPrefix("example-aks")
* .defaultNodePool(KubernetesClusterDefaultNodePoolArgs.builder()
* .name("default")
* .nodeCount(1)
* .vmSize("Standard_DS2_v2")
* .build())
* .identity(KubernetesClusterIdentityArgs.builder()
* .type("SystemAssigned")
* .build())
* .build());
* var exampleKubernetesClusterExtension = new KubernetesClusterExtension("exampleKubernetesClusterExtension", KubernetesClusterExtensionArgs.builder()
* .name("example-ext")
* .clusterId(test.id())
* .extensionType("microsoft.flux")
* .build());
* var exampleFluxConfiguration = new FluxConfiguration("exampleFluxConfiguration", FluxConfigurationArgs.builder()
* .name("example-fc")
* .clusterId(test.id())
* .namespace("flux")
* .gitRepository(FluxConfigurationGitRepositoryArgs.builder()
* .url("https://github.com/Azure/arc-k8s-demo")
* .referenceType("branch")
* .referenceValue("main")
* .build())
* .kustomizations(FluxConfigurationKustomizationArgs.builder()
* .name("kustomization-1")
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(exampleKubernetesClusterExtension)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleKubernetesCluster:
* type: azure:containerservice:KubernetesCluster
* name: example
* properties:
* name: example-aks
* location: West Europe
* resourceGroupName: ${example.name}
* dnsPrefix: example-aks
* defaultNodePool:
* name: default
* nodeCount: 1
* vmSize: Standard_DS2_v2
* identity:
* type: SystemAssigned
* exampleKubernetesClusterExtension:
* type: azure:containerservice:KubernetesClusterExtension
* name: example
* properties:
* name: example-ext
* clusterId: ${test.id}
* extensionType: microsoft.flux
* exampleFluxConfiguration:
* type: azure:containerservice:FluxConfiguration
* name: example
* properties:
* name: example-fc
* clusterId: ${test.id}
* namespace: flux
* gitRepository:
* url: https://github.com/Azure/arc-k8s-demo
* referenceType: branch
* referenceValue: main
* kustomizations:
* - name: kustomization-1
* options:
* dependson:
* - ${exampleKubernetesClusterExtension}
* ```
*
* ## Import
* Kubernetes Flux Configuration can be imported using the `resource id` for different `cluster_resource_name`, e.g.
* ```sh
* $ pulumi import azure:containerservice/fluxConfiguration:FluxConfiguration example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resourceGroup1/providers/Microsoft.ContainerService/managedClusters/cluster1/providers/Microsoft.KubernetesConfiguration/fluxConfigurations/fluxConfiguration1
* ```
* @property blobStorage An `blob_storage` block as defined below.
* @property bucket A `bucket` block as defined below.
* @property clusterId Specifies the Cluster ID. Changing this forces a new Kubernetes Cluster Extension to be created.
* @property continuousReconciliationEnabled Whether the configuration will keep its reconciliation of its kustomizations and sources with the repository. Defaults to `true`.
* @property gitRepository A `git_repository` block as defined below.
* @property kustomizations A `kustomizations` block as defined below.
* @property name Specifies the name which should be used for this Kubernetes Flux Configuration. Changing this forces a new Kubernetes Flux Configuration to be created.
* @property namespace Specifies the namespace to which this configuration is installed to. Changing this forces a new Kubernetes Flux Configuration to be created.
* @property scope Specifies the scope at which the operator will be installed. Possible values are `cluster` and `namespace`. Defaults to `namespace`. Changing this forces a new Kubernetes Flux Configuration to be created.
*/
public data class FluxConfigurationArgs(
public val blobStorage: Output? = null,
public val bucket: Output? = null,
public val clusterId: Output? = null,
public val continuousReconciliationEnabled: Output? = null,
public val gitRepository: Output? = null,
public val kustomizations: Output>? = null,
public val name: Output? = null,
public val namespace: Output? = null,
public val scope: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.containerservice.FluxConfigurationArgs =
com.pulumi.azure.containerservice.FluxConfigurationArgs.builder()
.blobStorage(blobStorage?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.bucket(bucket?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.clusterId(clusterId?.applyValue({ args0 -> args0 }))
.continuousReconciliationEnabled(continuousReconciliationEnabled?.applyValue({ args0 -> args0 }))
.gitRepository(gitRepository?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.kustomizations(
kustomizations?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.scope(scope?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FluxConfigurationArgs].
*/
@PulumiTagMarker
public class FluxConfigurationArgsBuilder internal constructor() {
private var blobStorage: Output? = null
private var bucket: Output? = null
private var clusterId: Output? = null
private var continuousReconciliationEnabled: Output? = null
private var gitRepository: Output? = null
private var kustomizations: Output>? = null
private var name: Output? = null
private var namespace: Output? = null
private var scope: Output? = null
/**
* @param value An `blob_storage` block as defined below.
*/
@JvmName("cgvqlbeshbcngjjy")
public suspend fun blobStorage(`value`: Output) {
this.blobStorage = value
}
/**
* @param value A `bucket` block as defined below.
*/
@JvmName("yuslnlckxkyltema")
public suspend fun bucket(`value`: Output) {
this.bucket = value
}
/**
* @param value Specifies the Cluster ID. Changing this forces a new Kubernetes Cluster Extension to be created.
*/
@JvmName("kowhqupqiklhvehy")
public suspend fun clusterId(`value`: Output) {
this.clusterId = value
}
/**
* @param value Whether the configuration will keep its reconciliation of its kustomizations and sources with the repository. Defaults to `true`.
*/
@JvmName("eocuskfssrxehyyt")
public suspend fun continuousReconciliationEnabled(`value`: Output) {
this.continuousReconciliationEnabled = value
}
/**
* @param value A `git_repository` block as defined below.
*/
@JvmName("lgdbkwivsanbfolt")
public suspend fun gitRepository(`value`: Output) {
this.gitRepository = value
}
/**
* @param value A `kustomizations` block as defined below.
*/
@JvmName("dytqxipbhpbktxph")
public suspend fun kustomizations(`value`: Output>) {
this.kustomizations = value
}
@JvmName("gfuesgfapyukikep")
public suspend fun kustomizations(vararg values: Output) {
this.kustomizations = Output.all(values.asList())
}
/**
* @param values A `kustomizations` block as defined below.
*/
@JvmName("vucutfaciisrhjel")
public suspend fun kustomizations(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy