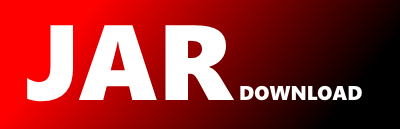
com.pulumi.azure.containerservice.kotlin.KubernetesFleetManagerArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin
import com.pulumi.azure.containerservice.KubernetesFleetManagerArgs.builder
import com.pulumi.azure.containerservice.kotlin.inputs.KubernetesFleetManagerHubProfileArgs
import com.pulumi.azure.containerservice.kotlin.inputs.KubernetesFleetManagerHubProfileArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Deprecated
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* Manages a Kubernetes Fleet Manager.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleKubernetesFleetManager = new azure.containerservice.KubernetesFleetManager("example", {
* location: example.location,
* name: "example",
* resourceGroupName: example.name,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_kubernetes_fleet_manager = azure.containerservice.KubernetesFleetManager("example",
* location=example.location,
* name="example",
* resource_group_name=example.name)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleKubernetesFleetManager = new Azure.ContainerService.KubernetesFleetManager("example", new()
* {
* Location = example.Location,
* Name = "example",
* ResourceGroupName = example.Name,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* _, err = containerservice.NewKubernetesFleetManager(ctx, "example", &containerservice.KubernetesFleetManagerArgs{
* Location: example.Location,
* Name: pulumi.String("example"),
* ResourceGroupName: example.Name,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.containerservice.KubernetesFleetManager;
* import com.pulumi.azure.containerservice.KubernetesFleetManagerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleKubernetesFleetManager = new KubernetesFleetManager("exampleKubernetesFleetManager", KubernetesFleetManagerArgs.builder()
* .location(example.location())
* .name("example")
* .resourceGroupName(example.name())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleKubernetesFleetManager:
* type: azure:containerservice:KubernetesFleetManager
* name: example
* properties:
* location: ${example.location}
* name: example
* resourceGroupName: ${example.name}
* ```
*
* ## Blocks Reference
* ### `hub_profile` Block
* The `hub_profile` block supports the following arguments:
* * `dns_prefix` - (Required)
* In addition to the arguments defined above, the `hub_profile` block exports the following attributes:
* * `fqdn` -
* * `kubernetes_version` -
* ## Import
* An existing Kubernetes Fleet Manager can be imported into Terraform using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:containerservice/kubernetesFleetManager:KubernetesFleetManager example /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ContainerService/fleets/{fleetName}
* ```
* * Where `{subscriptionId}` is the ID of the Azure Subscription where the Kubernetes Fleet Manager exists. For example `12345678-1234-9876-4563-123456789012`.
* * Where `{resourceGroupName}` is the name of Resource Group where this Kubernetes Fleet Manager exists. For example `example-resource-group`.
* * Where `{fleetName}` is the name of the Fleet. For example `fleetValue`.
* @property hubProfile
* @property location The Azure Region where the Kubernetes Fleet Manager should exist. Changing this forces a new Kubernetes Fleet Manager to be created.
* @property name Specifies the name of this Kubernetes Fleet Manager. Changing this forces a new Kubernetes Fleet Manager to be created.
* @property resourceGroupName Specifies the name of the Resource Group within which this Kubernetes Fleet Manager should exist. Changing this forces a new Kubernetes Fleet Manager to be created.
* @property tags A mapping of tags which should be assigned to the Kubernetes Fleet Manager.
*/
public data class KubernetesFleetManagerArgs(
@Deprecated(
message = """
The service team has indicated this field is now deprecated and not to be used, as such we are
marking it as such and no longer sending it to the API, please see url:
https://learn.microsoft.com/en-us/azure/kubernetes-fleet/architectural-overview
""",
)
public val hubProfile: Output? = null,
public val location: Output? = null,
public val name: Output? = null,
public val resourceGroupName: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy