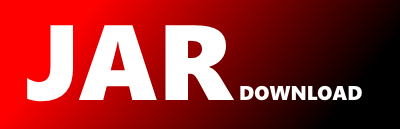
com.pulumi.azure.containerservice.kotlin.RegistryCacheRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin
import com.pulumi.azure.containerservice.RegistryCacheRuleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages an Azure Container Registry Cache Rule.
* > **Note:** All arguments including the access key will be stored in the raw state as plain-text.
* [Read more about sensitive data in state](https://www.terraform.io/docs/state/sensitive-data.html).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const acr = new azure.containerservice.Registry("acr", {
* name: "containerRegistry1",
* resourceGroupName: example.name,
* location: example.location,
* sku: "Basic",
* });
* const cacheRule = new azure.containerservice.RegistryCacheRule("cache_rule", {
* name: "cacherule",
* containerRegistryId: acr.id,
* targetRepo: "target",
* sourceRepo: "docker.io/hello-world",
* credentialSetId: pulumi.interpolate`${acr.id}/credentialSets/example`,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* acr = azure.containerservice.Registry("acr",
* name="containerRegistry1",
* resource_group_name=example.name,
* location=example.location,
* sku="Basic")
* cache_rule = azure.containerservice.RegistryCacheRule("cache_rule",
* name="cacherule",
* container_registry_id=acr.id,
* target_repo="target",
* source_repo="docker.io/hello-world",
* credential_set_id=acr.id.apply(lambda id: f"{id}/credentialSets/example"))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var acr = new Azure.ContainerService.Registry("acr", new()
* {
* Name = "containerRegistry1",
* ResourceGroupName = example.Name,
* Location = example.Location,
* Sku = "Basic",
* });
* var cacheRule = new Azure.ContainerService.RegistryCacheRule("cache_rule", new()
* {
* Name = "cacherule",
* ContainerRegistryId = acr.Id,
* TargetRepo = "target",
* SourceRepo = "docker.io/hello-world",
* CredentialSetId = acr.Id.Apply(id => $"{id}/credentialSets/example"),
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* acr, err := containerservice.NewRegistry(ctx, "acr", &containerservice.RegistryArgs{
* Name: pulumi.String("containerRegistry1"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* Sku: pulumi.String("Basic"),
* })
* if err != nil {
* return err
* }
* _, err = containerservice.NewRegistryCacheRule(ctx, "cache_rule", &containerservice.RegistryCacheRuleArgs{
* Name: pulumi.String("cacherule"),
* ContainerRegistryId: acr.ID(),
* TargetRepo: pulumi.String("target"),
* SourceRepo: pulumi.String("docker.io/hello-world"),
* CredentialSetId: acr.ID().ApplyT(func(id string) (string, error) {
* return fmt.Sprintf("%v/credentialSets/example", id), nil
* }).(pulumi.StringOutput),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.containerservice.Registry;
* import com.pulumi.azure.containerservice.RegistryArgs;
* import com.pulumi.azure.containerservice.RegistryCacheRule;
* import com.pulumi.azure.containerservice.RegistryCacheRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var acr = new Registry("acr", RegistryArgs.builder()
* .name("containerRegistry1")
* .resourceGroupName(example.name())
* .location(example.location())
* .sku("Basic")
* .build());
* var cacheRule = new RegistryCacheRule("cacheRule", RegistryCacheRuleArgs.builder()
* .name("cacherule")
* .containerRegistryId(acr.id())
* .targetRepo("target")
* .sourceRepo("docker.io/hello-world")
* .credentialSetId(acr.id().applyValue(id -> String.format("%s/credentialSets/example", id)))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* acr:
* type: azure:containerservice:Registry
* properties:
* name: containerRegistry1
* resourceGroupName: ${example.name}
* location: ${example.location}
* sku: Basic
* cacheRule:
* type: azure:containerservice:RegistryCacheRule
* name: cache_rule
* properties:
* name: cacherule
* containerRegistryId: ${acr.id}
* targetRepo: target
* sourceRepo: docker.io/hello-world
* credentialSetId: ${acr.id}/credentialSets/example
* ```
*
* ## Import
* Container Registry Cache Rules can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:containerservice/registryCacheRule:RegistryCacheRule example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myResourceGroup/providers/Microsoft.ContainerRegistry/registries/myRegistry/cacheRules/myCacheRule
* ```
* @property containerRegistryId The ID of the Container Registry where the Cache Rule should apply. Changing this forces a new resource to be created.
* @property credentialSetId The ARM resource ID of the Credential Store which is associated with the Cache Rule.
* @property name Specifies the name of the Container Registry Cache Rule. Only Alphanumeric characters allowed. Changing this forces a new resource to be created.
* @property sourceRepo The name of the source repository path. Changing this forces a new resource to be created.
* @property targetRepo The name of the new repository path to store artifacts. Changing this forces a new resource to be created.
*/
public data class RegistryCacheRuleArgs(
public val containerRegistryId: Output? = null,
public val credentialSetId: Output? = null,
public val name: Output? = null,
public val sourceRepo: Output? = null,
public val targetRepo: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.containerservice.RegistryCacheRuleArgs =
com.pulumi.azure.containerservice.RegistryCacheRuleArgs.builder()
.containerRegistryId(containerRegistryId?.applyValue({ args0 -> args0 }))
.credentialSetId(credentialSetId?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.sourceRepo(sourceRepo?.applyValue({ args0 -> args0 }))
.targetRepo(targetRepo?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RegistryCacheRuleArgs].
*/
@PulumiTagMarker
public class RegistryCacheRuleArgsBuilder internal constructor() {
private var containerRegistryId: Output? = null
private var credentialSetId: Output? = null
private var name: Output? = null
private var sourceRepo: Output? = null
private var targetRepo: Output? = null
/**
* @param value The ID of the Container Registry where the Cache Rule should apply. Changing this forces a new resource to be created.
*/
@JvmName("ydptdnjdnlidfsfa")
public suspend fun containerRegistryId(`value`: Output) {
this.containerRegistryId = value
}
/**
* @param value The ARM resource ID of the Credential Store which is associated with the Cache Rule.
*/
@JvmName("jfqxuqykptnyjylq")
public suspend fun credentialSetId(`value`: Output) {
this.credentialSetId = value
}
/**
* @param value Specifies the name of the Container Registry Cache Rule. Only Alphanumeric characters allowed. Changing this forces a new resource to be created.
*/
@JvmName("miyhjgdgwefgqdkb")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The name of the source repository path. Changing this forces a new resource to be created.
*/
@JvmName("bxqyrmdaltuwsgjk")
public suspend fun sourceRepo(`value`: Output) {
this.sourceRepo = value
}
/**
* @param value The name of the new repository path to store artifacts. Changing this forces a new resource to be created.
*/
@JvmName("niiavaxkvbublefg")
public suspend fun targetRepo(`value`: Output) {
this.targetRepo = value
}
/**
* @param value The ID of the Container Registry where the Cache Rule should apply. Changing this forces a new resource to be created.
*/
@JvmName("vihqxolbrqrnuchq")
public suspend fun containerRegistryId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.containerRegistryId = mapped
}
/**
* @param value The ARM resource ID of the Credential Store which is associated with the Cache Rule.
*/
@JvmName("lhvxlldrjnwdtabl")
public suspend fun credentialSetId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.credentialSetId = mapped
}
/**
* @param value Specifies the name of the Container Registry Cache Rule. Only Alphanumeric characters allowed. Changing this forces a new resource to be created.
*/
@JvmName("lkkbkvryfyiuxpsr")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The name of the source repository path. Changing this forces a new resource to be created.
*/
@JvmName("rfqcdptdsxtlaqlt")
public suspend fun sourceRepo(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceRepo = mapped
}
/**
* @param value The name of the new repository path to store artifacts. Changing this forces a new resource to be created.
*/
@JvmName("upawiycftbufkrju")
public suspend fun targetRepo(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetRepo = mapped
}
internal fun build(): RegistryCacheRuleArgs = RegistryCacheRuleArgs(
containerRegistryId = containerRegistryId,
credentialSetId = credentialSetId,
name = name,
sourceRepo = sourceRepo,
targetRepo = targetRepo,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy