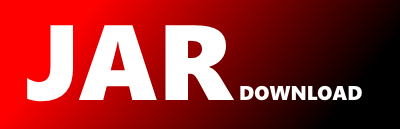
com.pulumi.azure.containerservice.kotlin.inputs.GroupContainerLivenessProbeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin.inputs
import com.pulumi.azure.containerservice.inputs.GroupContainerLivenessProbeArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property execs Commands to be run to validate container readiness. Changing this forces a new resource to be created.
* @property failureThreshold How many times to try the probe before restarting the container (liveness probe) or marking the container as unhealthy (readiness probe). Changing this forces a new resource to be created.
* @property httpGets The definition of the http_get for this container as documented in the `http_get` block below. Changing this forces a new resource to be created.
* @property initialDelaySeconds Number of seconds after the container has started before liveness or readiness probes are initiated. Changing this forces a new resource to be created.
* @property periodSeconds How often (in seconds) to perform the probe. Changing this forces a new resource to be created.
* @property successThreshold Minimum consecutive successes for the probe to be considered successful after having failed. Changing this forces a new resource to be created.
* @property timeoutSeconds Number of seconds after which the probe times out. Changing this forces a new resource to be created.
*/
public data class GroupContainerLivenessProbeArgs(
public val execs: Output>? = null,
public val failureThreshold: Output? = null,
public val httpGets: Output>? = null,
public val initialDelaySeconds: Output? = null,
public val periodSeconds: Output? = null,
public val successThreshold: Output? = null,
public val timeoutSeconds: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.containerservice.inputs.GroupContainerLivenessProbeArgs =
com.pulumi.azure.containerservice.inputs.GroupContainerLivenessProbeArgs.builder()
.execs(execs?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.failureThreshold(failureThreshold?.applyValue({ args0 -> args0 }))
.httpGets(
httpGets?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.initialDelaySeconds(initialDelaySeconds?.applyValue({ args0 -> args0 }))
.periodSeconds(periodSeconds?.applyValue({ args0 -> args0 }))
.successThreshold(successThreshold?.applyValue({ args0 -> args0 }))
.timeoutSeconds(timeoutSeconds?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [GroupContainerLivenessProbeArgs].
*/
@PulumiTagMarker
public class GroupContainerLivenessProbeArgsBuilder internal constructor() {
private var execs: Output>? = null
private var failureThreshold: Output? = null
private var httpGets: Output>? = null
private var initialDelaySeconds: Output? = null
private var periodSeconds: Output? = null
private var successThreshold: Output? = null
private var timeoutSeconds: Output? = null
/**
* @param value Commands to be run to validate container readiness. Changing this forces a new resource to be created.
*/
@JvmName("fkefekxrxswoubhg")
public suspend fun execs(`value`: Output>) {
this.execs = value
}
@JvmName("rhwdrkwbvetrsxgc")
public suspend fun execs(vararg values: Output) {
this.execs = Output.all(values.asList())
}
/**
* @param values Commands to be run to validate container readiness. Changing this forces a new resource to be created.
*/
@JvmName("yxnaflefstjxfjnp")
public suspend fun execs(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy