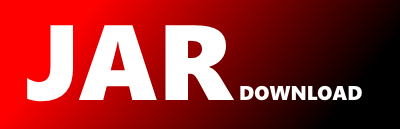
com.pulumi.azure.containerservice.kotlin.inputs.KubernetesClusterNodePoolKubeletConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin.inputs
import com.pulumi.azure.containerservice.inputs.KubernetesClusterNodePoolKubeletConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property allowedUnsafeSysctls Specifies the allow list of unsafe sysctls command or patterns (ending in `*`). Changing this forces a new resource to be created.
* @property containerLogMaxLine Specifies the maximum number of container log files that can be present for a container. must be at least 2. Changing this forces a new resource to be created.
* @property containerLogMaxSizeMb Specifies the maximum size (e.g. 10MB) of container log file before it is rotated. Changing this forces a new resource to be created.
* @property cpuCfsQuotaEnabled Is CPU CFS quota enforcement for containers enabled? Changing this forces a new resource to be created.
* @property cpuCfsQuotaPeriod Specifies the CPU CFS quota period value. Changing this forces a new resource to be created.
* @property cpuManagerPolicy Specifies the CPU Manager policy to use. Possible values are `none` and `static`, Changing this forces a new resource to be created.
* @property imageGcHighThreshold Specifies the percent of disk usage above which image garbage collection is always run. Must be between `0` and `100`. Changing this forces a new resource to be created.
* @property imageGcLowThreshold Specifies the percent of disk usage lower than which image garbage collection is never run. Must be between `0` and `100`. Changing this forces a new resource to be created.
* @property podMaxPid Specifies the maximum number of processes per pod. Changing this forces a new resource to be created.
* @property topologyManagerPolicy Specifies the Topology Manager policy to use. Possible values are `none`, `best-effort`, `restricted` or `single-numa-node`. Changing this forces a new resource to be created.
*/
public data class KubernetesClusterNodePoolKubeletConfigArgs(
public val allowedUnsafeSysctls: Output>? = null,
public val containerLogMaxLine: Output? = null,
public val containerLogMaxSizeMb: Output? = null,
public val cpuCfsQuotaEnabled: Output? = null,
public val cpuCfsQuotaPeriod: Output? = null,
public val cpuManagerPolicy: Output? = null,
public val imageGcHighThreshold: Output? = null,
public val imageGcLowThreshold: Output? = null,
public val podMaxPid: Output? = null,
public val topologyManagerPolicy: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azure.containerservice.inputs.KubernetesClusterNodePoolKubeletConfigArgs =
com.pulumi.azure.containerservice.inputs.KubernetesClusterNodePoolKubeletConfigArgs.builder()
.allowedUnsafeSysctls(allowedUnsafeSysctls?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.containerLogMaxLine(containerLogMaxLine?.applyValue({ args0 -> args0 }))
.containerLogMaxSizeMb(containerLogMaxSizeMb?.applyValue({ args0 -> args0 }))
.cpuCfsQuotaEnabled(cpuCfsQuotaEnabled?.applyValue({ args0 -> args0 }))
.cpuCfsQuotaPeriod(cpuCfsQuotaPeriod?.applyValue({ args0 -> args0 }))
.cpuManagerPolicy(cpuManagerPolicy?.applyValue({ args0 -> args0 }))
.imageGcHighThreshold(imageGcHighThreshold?.applyValue({ args0 -> args0 }))
.imageGcLowThreshold(imageGcLowThreshold?.applyValue({ args0 -> args0 }))
.podMaxPid(podMaxPid?.applyValue({ args0 -> args0 }))
.topologyManagerPolicy(topologyManagerPolicy?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [KubernetesClusterNodePoolKubeletConfigArgs].
*/
@PulumiTagMarker
public class KubernetesClusterNodePoolKubeletConfigArgsBuilder internal constructor() {
private var allowedUnsafeSysctls: Output>? = null
private var containerLogMaxLine: Output? = null
private var containerLogMaxSizeMb: Output? = null
private var cpuCfsQuotaEnabled: Output? = null
private var cpuCfsQuotaPeriod: Output? = null
private var cpuManagerPolicy: Output? = null
private var imageGcHighThreshold: Output? = null
private var imageGcLowThreshold: Output? = null
private var podMaxPid: Output? = null
private var topologyManagerPolicy: Output? = null
/**
* @param value Specifies the allow list of unsafe sysctls command or patterns (ending in `*`). Changing this forces a new resource to be created.
*/
@JvmName("yielgcehbmdsbana")
public suspend fun allowedUnsafeSysctls(`value`: Output>) {
this.allowedUnsafeSysctls = value
}
@JvmName("ujgtoiqupynaylex")
public suspend fun allowedUnsafeSysctls(vararg values: Output) {
this.allowedUnsafeSysctls = Output.all(values.asList())
}
/**
* @param values Specifies the allow list of unsafe sysctls command or patterns (ending in `*`). Changing this forces a new resource to be created.
*/
@JvmName("sxvweqyefhabibnv")
public suspend fun allowedUnsafeSysctls(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy