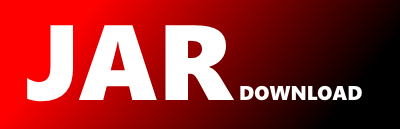
com.pulumi.azure.containerservice.kotlin.outputs.GetClusterNodePoolResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin.outputs
import kotlin.Boolean
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getClusterNodePool.
* @property autoScalingEnabled
* @property enableAutoScaling Does this Node Pool have Auto-Scaling enabled?
* @property enableNodePublicIp Do nodes in this Node Pool have a Public IP Address?
* @property evictionPolicy The eviction policy used for Virtual Machines in the Virtual Machine Scale Set, when `priority` is set to `Spot`.
* @property id The provider-assigned unique ID for this managed resource.
* @property kubernetesClusterName
* @property maxCount The maximum number of Nodes allowed when auto-scaling is enabled.
* @property maxPods The maximum number of Pods allowed on each Node in this Node Pool.
* @property minCount The minimum number of Nodes allowed when auto-scaling is enabled.
* @property mode The Mode for this Node Pool, specifying how these Nodes should be used (for either System or User resources).
* @property name
* @property nodeCount The current number of Nodes in the Node Pool.
* @property nodeLabels A map of Kubernetes Labels applied to each Node in this Node Pool.
* @property nodePublicIpEnabled
* @property nodePublicIpPrefixId Resource ID for the Public IP Addresses Prefix for the nodes in this Agent Pool.
* @property nodeTaints A map of Kubernetes Taints applied to each Node in this Node Pool.
* @property orchestratorVersion The version of Kubernetes configured on each Node in this Node Pool.
* @property osDiskSizeGb The size of the OS Disk on each Node in this Node Pool.
* @property osDiskType The type of the OS Disk on each Node in this Node Pool.
* @property osType The operating system used on each Node in this Node Pool.
* @property priority The priority of the Virtual Machines in the Virtual Machine Scale Set backing this Node Pool.
* @property proximityPlacementGroupId The ID of the Proximity Placement Group where the Virtual Machine Scale Set backing this Node Pool will be placed.
* @property resourceGroupName
* @property spotMaxPrice The maximum price being paid for Virtual Machines in this Scale Set. `-1` means the current on-demand price for a Virtual Machine.
* @property tags A mapping of tags assigned to the Kubernetes Cluster Node Pool.
* @property upgradeSettings A `upgrade_settings` block as documented below.
* @property vmSize The size of the Virtual Machines used in the Virtual Machine Scale Set backing this Node Pool.
* @property vnetSubnetId The ID of the Subnet in which this Node Pool exists.
* @property zones A list of the Availability Zones where the Nodes in this Node Pool exist.
*/
public data class GetClusterNodePoolResult(
public val autoScalingEnabled: Boolean,
public val enableAutoScaling: Boolean,
public val enableNodePublicIp: Boolean,
public val evictionPolicy: String,
public val id: String,
public val kubernetesClusterName: String,
public val maxCount: Int,
public val maxPods: Int,
public val minCount: Int,
public val mode: String,
public val name: String,
public val nodeCount: Int,
public val nodeLabels: Map,
public val nodePublicIpEnabled: Boolean,
public val nodePublicIpPrefixId: String,
public val nodeTaints: List,
public val orchestratorVersion: String,
public val osDiskSizeGb: Int,
public val osDiskType: String,
public val osType: String,
public val priority: String,
public val proximityPlacementGroupId: String,
public val resourceGroupName: String,
public val spotMaxPrice: Double,
public val tags: Map,
public val upgradeSettings: List,
public val vmSize: String,
public val vnetSubnetId: String,
public val zones: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.containerservice.outputs.GetClusterNodePoolResult): GetClusterNodePoolResult = GetClusterNodePoolResult(
autoScalingEnabled = javaType.autoScalingEnabled(),
enableAutoScaling = javaType.enableAutoScaling(),
enableNodePublicIp = javaType.enableNodePublicIp(),
evictionPolicy = javaType.evictionPolicy(),
id = javaType.id(),
kubernetesClusterName = javaType.kubernetesClusterName(),
maxCount = javaType.maxCount(),
maxPods = javaType.maxPods(),
minCount = javaType.minCount(),
mode = javaType.mode(),
name = javaType.name(),
nodeCount = javaType.nodeCount(),
nodeLabels = javaType.nodeLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
nodePublicIpEnabled = javaType.nodePublicIpEnabled(),
nodePublicIpPrefixId = javaType.nodePublicIpPrefixId(),
nodeTaints = javaType.nodeTaints().map({ args0 -> args0 }),
orchestratorVersion = javaType.orchestratorVersion(),
osDiskSizeGb = javaType.osDiskSizeGb(),
osDiskType = javaType.osDiskType(),
osType = javaType.osType(),
priority = javaType.priority(),
proximityPlacementGroupId = javaType.proximityPlacementGroupId(),
resourceGroupName = javaType.resourceGroupName(),
spotMaxPrice = javaType.spotMaxPrice(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
upgradeSettings = javaType.upgradeSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetClusterNodePoolUpgradeSetting.Companion.toKotlin(args0)
})
}),
vmSize = javaType.vmSize(),
vnetSubnetId = javaType.vnetSubnetId(),
zones = javaType.zones().map({ args0 -> args0 }),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy