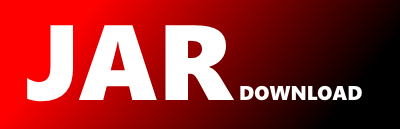
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getKubernetesCluster.
* @property aciConnectorLinuxes An `aci_connector_linux` block as documented below.
* @property agentPoolProfiles An `agent_pool_profile` block as documented below.
* @property apiServerAuthorizedIpRanges The IP ranges to whitelist for incoming traffic to the primaries.
* @property azureActiveDirectoryRoleBasedAccessControls An `azure_active_directory_role_based_access_control` block as documented below.
* @property azurePolicyEnabled Is Azure Policy enabled on this managed Kubernetes Cluster?
* @property currentKubernetesVersion Contains the current version of Kubernetes running on the Cluster.
* @property customCaTrustCertificatesBase64s A list of custom base64 encoded CAs used by this Managed Kubernetes Cluster.
* @property diskEncryptionSetId The ID of the Disk Encryption Set used for the Nodes and Volumes.
* @property dnsPrefix The DNS Prefix of the managed Kubernetes cluster.
* @property fqdn The FQDN of the Azure Kubernetes Managed Cluster.
* @property httpApplicationRoutingEnabled Is HTTP Application Routing enabled for this managed Kubernetes Cluster?
* @property httpApplicationRoutingZoneName The Zone Name of the HTTP Application Routing.
* @property id The provider-assigned unique ID for this managed resource.
* @property identities An `identity` block as documented below.
* @property ingressApplicationGateways An `ingress_application_gateway` block as documented below.
* @property keyManagementServices A `key_management_service` block as documented below.
* @property keyVaultSecretsProviders A `key_vault_secrets_provider` block as documented below.
* @property kubeAdminConfigRaw Raw Kubernetes config for the admin account to be used by [kubectl](https://kubernetes.io/docs/reference/kubectl/overview/) and other compatible tools. This is only available when Role Based Access Control with Azure Active Directory is enabled and local accounts are not disabled.
* @property kubeAdminConfigs A `kube_admin_config` block as defined below. This is only available when Role Based Access Control with Azure Active Directory is enabled and local accounts are not disabled.
* @property kubeConfigRaw Base64 encoded Kubernetes configuration.
* @property kubeConfigs A `kube_config` block as defined below.
* @property kubeletIdentities A `kubelet_identity` block as documented below.
* @property kubernetesVersion The version of Kubernetes used on the managed Kubernetes Cluster.
* @property linuxProfiles A `linux_profile` block as documented below.
* @property location The Azure Region in which the managed Kubernetes Cluster exists.
* @property microsoftDefenders A `microsoft_defender` block as defined below.
* @property name The name assigned to this pool of agents.
* @property networkProfiles A `network_profile` block as documented below.
* @property nodeResourceGroup Auto-generated Resource Group containing AKS Cluster resources.
* @property nodeResourceGroupId The ID of the Resource Group containing the resources for this Managed Kubernetes Cluster.
* @property oidcIssuerEnabled Whether or not the OIDC feature is enabled or disabled.
* @property oidcIssuerUrl The OIDC issuer URL that is associated with the cluster.
* @property omsAgents An `oms_agent` block as documented below.
* @property openServiceMeshEnabled Is Open Service Mesh enabled for this managed Kubernetes Cluster?
* @property privateClusterEnabled If the cluster has the Kubernetes API only exposed on internal IP addresses.
* @property privateFqdn The FQDN of this Kubernetes Cluster when private link has been enabled. This name is only resolvable inside the Virtual Network where the Azure Kubernetes Service is located
* @property resourceGroupName
* @property roleBasedAccessControlEnabled Is Role Based Access Control enabled for this managed Kubernetes Cluster?
* @property serviceMeshProfiles
* @property servicePrincipals A `service_principal` block as documented below.
* @property storageProfiles A `storage_profile` block as documented below.
* @property tags A mapping of tags to assign to the resource.
* @property windowsProfiles A `windows_profile` block as documented below.
*/
public data class GetKubernetesClusterResult(
public val aciConnectorLinuxes: List,
public val agentPoolProfiles: List,
public val apiServerAuthorizedIpRanges: List,
public val azureActiveDirectoryRoleBasedAccessControls: List,
public val azurePolicyEnabled: Boolean,
public val currentKubernetesVersion: String,
public val customCaTrustCertificatesBase64s: List,
public val diskEncryptionSetId: String,
public val dnsPrefix: String,
public val fqdn: String,
public val httpApplicationRoutingEnabled: Boolean,
public val httpApplicationRoutingZoneName: String,
public val id: String,
public val identities: List,
public val ingressApplicationGateways: List,
public val keyManagementServices: List,
public val keyVaultSecretsProviders: List,
public val kubeAdminConfigRaw: String,
public val kubeAdminConfigs: List,
public val kubeConfigRaw: String,
public val kubeConfigs: List,
public val kubeletIdentities: List,
public val kubernetesVersion: String,
public val linuxProfiles: List,
public val location: String,
public val microsoftDefenders: List,
public val name: String,
public val networkProfiles: List,
public val nodeResourceGroup: String,
public val nodeResourceGroupId: String,
public val oidcIssuerEnabled: Boolean,
public val oidcIssuerUrl: String,
public val omsAgents: List,
public val openServiceMeshEnabled: Boolean,
public val privateClusterEnabled: Boolean,
public val privateFqdn: String,
public val resourceGroupName: String,
public val roleBasedAccessControlEnabled: Boolean,
public val serviceMeshProfiles: List,
public val servicePrincipals: List,
public val storageProfiles: List,
public val tags: Map,
public val windowsProfiles: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.containerservice.outputs.GetKubernetesClusterResult): GetKubernetesClusterResult = GetKubernetesClusterResult(
aciConnectorLinuxes = javaType.aciConnectorLinuxes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterAciConnectorLinux.Companion.toKotlin(args0)
})
}),
agentPoolProfiles = javaType.agentPoolProfiles().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterAgentPoolProfile.Companion.toKotlin(args0)
})
}),
apiServerAuthorizedIpRanges = javaType.apiServerAuthorizedIpRanges().map({ args0 -> args0 }),
azureActiveDirectoryRoleBasedAccessControls = javaType.azureActiveDirectoryRoleBasedAccessControls().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterAzureActiveDirectoryRoleBasedAccessControl.Companion.toKotlin(args0)
})
}),
azurePolicyEnabled = javaType.azurePolicyEnabled(),
currentKubernetesVersion = javaType.currentKubernetesVersion(),
customCaTrustCertificatesBase64s = javaType.customCaTrustCertificatesBase64s().map({ args0 ->
args0
}),
diskEncryptionSetId = javaType.diskEncryptionSetId(),
dnsPrefix = javaType.dnsPrefix(),
fqdn = javaType.fqdn(),
httpApplicationRoutingEnabled = javaType.httpApplicationRoutingEnabled(),
httpApplicationRoutingZoneName = javaType.httpApplicationRoutingZoneName(),
id = javaType.id(),
identities = javaType.identities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterIdentity.Companion.toKotlin(args0)
})
}),
ingressApplicationGateways = javaType.ingressApplicationGateways().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterIngressApplicationGateway.Companion.toKotlin(args0)
})
}),
keyManagementServices = javaType.keyManagementServices().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterKeyManagementService.Companion.toKotlin(args0)
})
}),
keyVaultSecretsProviders = javaType.keyVaultSecretsProviders().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterKeyVaultSecretsProvider.Companion.toKotlin(args0)
})
}),
kubeAdminConfigRaw = javaType.kubeAdminConfigRaw(),
kubeAdminConfigs = javaType.kubeAdminConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterKubeAdminConfig.Companion.toKotlin(args0)
})
}),
kubeConfigRaw = javaType.kubeConfigRaw(),
kubeConfigs = javaType.kubeConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterKubeConfig.Companion.toKotlin(args0)
})
}),
kubeletIdentities = javaType.kubeletIdentities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterKubeletIdentity.Companion.toKotlin(args0)
})
}),
kubernetesVersion = javaType.kubernetesVersion(),
linuxProfiles = javaType.linuxProfiles().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterLinuxProfile.Companion.toKotlin(args0)
})
}),
location = javaType.location(),
microsoftDefenders = javaType.microsoftDefenders().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterMicrosoftDefender.Companion.toKotlin(args0)
})
}),
name = javaType.name(),
networkProfiles = javaType.networkProfiles().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterNetworkProfile.Companion.toKotlin(args0)
})
}),
nodeResourceGroup = javaType.nodeResourceGroup(),
nodeResourceGroupId = javaType.nodeResourceGroupId(),
oidcIssuerEnabled = javaType.oidcIssuerEnabled(),
oidcIssuerUrl = javaType.oidcIssuerUrl(),
omsAgents = javaType.omsAgents().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterOmsAgent.Companion.toKotlin(args0)
})
}),
openServiceMeshEnabled = javaType.openServiceMeshEnabled(),
privateClusterEnabled = javaType.privateClusterEnabled(),
privateFqdn = javaType.privateFqdn(),
resourceGroupName = javaType.resourceGroupName(),
roleBasedAccessControlEnabled = javaType.roleBasedAccessControlEnabled(),
serviceMeshProfiles = javaType.serviceMeshProfiles().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterServiceMeshProfile.Companion.toKotlin(args0)
})
}),
servicePrincipals = javaType.servicePrincipals().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterServicePrincipal.Companion.toKotlin(args0)
})
}),
storageProfiles = javaType.storageProfiles().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterStorageProfile.Companion.toKotlin(args0)
})
}),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
windowsProfiles = javaType.windowsProfiles().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterWindowsProfile.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy