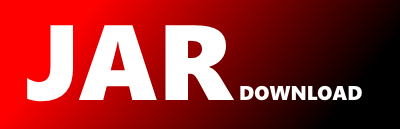
com.pulumi.azure.containerservice.kotlin.outputs.GroupContainer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin.outputs
import kotlin.Deprecated
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property commands A list of commands which should be run on the container. Changing this forces a new resource to be created.
* @property cpu The required number of CPU cores of the containers. Changing this forces a new resource to be created.
* @property cpuLimit The upper limit of the number of CPU cores of the containers.
* @property environmentVariables A list of environment variables to be set on the container. Specified as a map of name/value pairs. Changing this forces a new resource to be created.
* @property gpu
* @property gpuLimit
* @property image The container image name. Changing this forces a new resource to be created.
* @property livenessProbe The definition of a readiness probe for this container as documented in the `liveness_probe` block below. Changing this forces a new resource to be created.
* @property memory The required memory of the containers in GB. Changing this forces a new resource to be created.
* @property memoryLimit The upper limit of the memory of the containers in GB.
* @property name Specifies the name of the Container. Changing this forces a new resource to be created.
* @property ports A set of public ports for the container. Changing this forces a new resource to be created. Set as documented in the `ports` block below.
* @property readinessProbe The definition of a readiness probe for this container as documented in the `readiness_probe` block below. Changing this forces a new resource to be created.
* @property secureEnvironmentVariables A list of sensitive environment variables to be set on the container. Specified as a map of name/value pairs. Changing this forces a new resource to be created.
* @property securities The definition of the security context for this container as documented in the `security` block below. Changing this forces a new resource to be created.
* @property volumes The definition of a volume mount for this container as documented in the `volume` block below. Changing this forces a new resource to be created.
*/
public data class GroupContainer(
public val commands: List? = null,
public val cpu: Double,
public val cpuLimit: Double? = null,
public val environmentVariables: Map? = null,
@Deprecated(
message = """
The `gpu` block has been deprecated since K80 and P100 GPU Skus have been retired and remaining
GPU resources are not fully supported and not appropriate for production workloads. This block
will be removed in v4.0 of the AzureRM provider.
""",
)
public val gpu: GroupContainerGpu? = null,
@Deprecated(
message = """
The `gpu_limit` block has been deprecated since K80 and P100 GPU Skus have been retired and
remaining GPU resources are not fully supported and not appropriate for production workloads.
This block will be removed in v4.0 of the AzureRM provider.
""",
)
public val gpuLimit: GroupContainerGpuLimit? = null,
public val image: String,
public val livenessProbe: GroupContainerLivenessProbe? = null,
public val memory: Double,
public val memoryLimit: Double? = null,
public val name: String,
public val ports: List? = null,
public val readinessProbe: GroupContainerReadinessProbe? = null,
public val secureEnvironmentVariables: Map? = null,
public val securities: List? = null,
public val volumes: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.containerservice.outputs.GroupContainer): GroupContainer = GroupContainer(
commands = javaType.commands().map({ args0 -> args0 }),
cpu = javaType.cpu(),
cpuLimit = javaType.cpuLimit().map({ args0 -> args0 }).orElse(null),
environmentVariables = javaType.environmentVariables().map({ args0 ->
args0.key.to(args0.value)
}).toMap(),
gpu = javaType.gpu().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GroupContainerGpu.Companion.toKotlin(args0)
})
}).orElse(null),
gpuLimit = javaType.gpuLimit().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GroupContainerGpuLimit.Companion.toKotlin(args0)
})
}).orElse(null),
image = javaType.image(),
livenessProbe = javaType.livenessProbe().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GroupContainerLivenessProbe.Companion.toKotlin(args0)
})
}).orElse(null),
memory = javaType.memory(),
memoryLimit = javaType.memoryLimit().map({ args0 -> args0 }).orElse(null),
name = javaType.name(),
ports = javaType.ports().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GroupContainerPort.Companion.toKotlin(args0)
})
}),
readinessProbe = javaType.readinessProbe().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GroupContainerReadinessProbe.Companion.toKotlin(args0)
})
}).orElse(null),
secureEnvironmentVariables = javaType.secureEnvironmentVariables().map({ args0 ->
args0.key.to(args0.value)
}).toMap(),
securities = javaType.securities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GroupContainerSecurity.Companion.toKotlin(args0)
})
}),
volumes = javaType.volumes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.containerservice.kotlin.outputs.GroupContainerVolume.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy