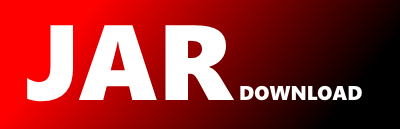
com.pulumi.azure.containerservice.kotlin.outputs.KubernetesClusterAutoScalerProfile.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin.outputs
import kotlin.Boolean
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
/**
*
* @property balanceSimilarNodeGroups Detect similar node groups and balance the number of nodes between them. Defaults to `false`.
* @property emptyBulkDeleteMax Maximum number of empty nodes that can be deleted at the same time. Defaults to `10`.
* @property expander Expander to use. Possible values are `least-waste`, `priority`, `most-pods` and `random`. Defaults to `random`.
* @property maxGracefulTerminationSec Maximum number of seconds the cluster autoscaler waits for pod termination when trying to scale down a node. Defaults to `600`.
* @property maxNodeProvisioningTime Maximum time the autoscaler waits for a node to be provisioned. Defaults to `15m`.
* @property maxUnreadyNodes Maximum Number of allowed unready nodes. Defaults to `3`.
* @property maxUnreadyPercentage Maximum percentage of unready nodes the cluster autoscaler will stop if the percentage is exceeded. Defaults to `45`.
* @property newPodScaleUpDelay For scenarios like burst/batch scale where you don't want CA to act before the kubernetes scheduler could schedule all the pods, you can tell CA to ignore unscheduled pods before they're a certain age. Defaults to `10s`.
* @property scaleDownDelayAfterAdd How long after the scale up of AKS nodes the scale down evaluation resumes. Defaults to `10m`.
* @property scaleDownDelayAfterDelete How long after node deletion that scale down evaluation resumes. Defaults to the value used for `scan_interval`.
* @property scaleDownDelayAfterFailure How long after scale down failure that scale down evaluation resumes. Defaults to `3m`.
* @property scaleDownUnneeded How long a node should be unneeded before it is eligible for scale down. Defaults to `10m`.
* @property scaleDownUnready How long an unready node should be unneeded before it is eligible for scale down. Defaults to `20m`.
* @property scaleDownUtilizationThreshold Node utilization level, defined as sum of requested resources divided by capacity, below which a node can be considered for scale down. Defaults to `0.5`.
* @property scanInterval How often the AKS Cluster should be re-evaluated for scale up/down. Defaults to `10s`.
* @property skipNodesWithLocalStorage If `true` cluster autoscaler will never delete nodes with pods with local storage, for example, EmptyDir or HostPath. Defaults to `true`.
* @property skipNodesWithSystemPods If `true` cluster autoscaler will never delete nodes with pods from kube-system (except for DaemonSet or mirror pods). Defaults to `true`.
*/
public data class KubernetesClusterAutoScalerProfile(
public val balanceSimilarNodeGroups: Boolean? = null,
public val emptyBulkDeleteMax: String? = null,
public val expander: String? = null,
public val maxGracefulTerminationSec: String? = null,
public val maxNodeProvisioningTime: String? = null,
public val maxUnreadyNodes: Int? = null,
public val maxUnreadyPercentage: Double? = null,
public val newPodScaleUpDelay: String? = null,
public val scaleDownDelayAfterAdd: String? = null,
public val scaleDownDelayAfterDelete: String? = null,
public val scaleDownDelayAfterFailure: String? = null,
public val scaleDownUnneeded: String? = null,
public val scaleDownUnready: String? = null,
public val scaleDownUtilizationThreshold: String? = null,
public val scanInterval: String? = null,
public val skipNodesWithLocalStorage: Boolean? = null,
public val skipNodesWithSystemPods: Boolean? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.containerservice.outputs.KubernetesClusterAutoScalerProfile): KubernetesClusterAutoScalerProfile = KubernetesClusterAutoScalerProfile(
balanceSimilarNodeGroups = javaType.balanceSimilarNodeGroups().map({ args0 -> args0 }).orElse(null),
emptyBulkDeleteMax = javaType.emptyBulkDeleteMax().map({ args0 -> args0 }).orElse(null),
expander = javaType.expander().map({ args0 -> args0 }).orElse(null),
maxGracefulTerminationSec = javaType.maxGracefulTerminationSec().map({ args0 ->
args0
}).orElse(null),
maxNodeProvisioningTime = javaType.maxNodeProvisioningTime().map({ args0 -> args0 }).orElse(null),
maxUnreadyNodes = javaType.maxUnreadyNodes().map({ args0 -> args0 }).orElse(null),
maxUnreadyPercentage = javaType.maxUnreadyPercentage().map({ args0 -> args0 }).orElse(null),
newPodScaleUpDelay = javaType.newPodScaleUpDelay().map({ args0 -> args0 }).orElse(null),
scaleDownDelayAfterAdd = javaType.scaleDownDelayAfterAdd().map({ args0 -> args0 }).orElse(null),
scaleDownDelayAfterDelete = javaType.scaleDownDelayAfterDelete().map({ args0 ->
args0
}).orElse(null),
scaleDownDelayAfterFailure = javaType.scaleDownDelayAfterFailure().map({ args0 ->
args0
}).orElse(null),
scaleDownUnneeded = javaType.scaleDownUnneeded().map({ args0 -> args0 }).orElse(null),
scaleDownUnready = javaType.scaleDownUnready().map({ args0 -> args0 }).orElse(null),
scaleDownUtilizationThreshold = javaType.scaleDownUtilizationThreshold().map({ args0 ->
args0
}).orElse(null),
scanInterval = javaType.scanInterval().map({ args0 -> args0 }).orElse(null),
skipNodesWithLocalStorage = javaType.skipNodesWithLocalStorage().map({ args0 ->
args0
}).orElse(null),
skipNodesWithSystemPods = javaType.skipNodesWithSystemPods().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy