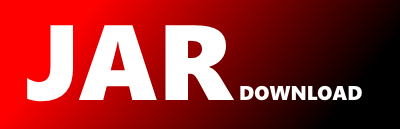
com.pulumi.azure.cosmosdb.kotlin.CassandraTableArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.cosmosdb.kotlin
import com.pulumi.azure.cosmosdb.CassandraTableArgs.builder
import com.pulumi.azure.cosmosdb.kotlin.inputs.CassandraTableAutoscaleSettingsArgs
import com.pulumi.azure.cosmosdb.kotlin.inputs.CassandraTableAutoscaleSettingsArgsBuilder
import com.pulumi.azure.cosmosdb.kotlin.inputs.CassandraTableSchemaArgs
import com.pulumi.azure.cosmosdb.kotlin.inputs.CassandraTableSchemaArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Manages a Cassandra Table within a Cosmos DB Cassandra Keyspace.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "tflex-cosmosdb-account-rg",
* location: "West Europe",
* });
* const exampleAccount = new azure.cosmosdb.Account("example", {
* name: "tfex-cosmosdb-account",
* resourceGroupName: example.name,
* location: example.location,
* offerType: "Standard",
* capabilities: [{
* name: "EnableCassandra",
* }],
* consistencyPolicy: {
* consistencyLevel: "Strong",
* },
* geoLocations: [{
* location: example.location,
* failoverPriority: 0,
* }],
* });
* const exampleCassandraKeyspace = new azure.cosmosdb.CassandraKeyspace("example", {
* name: "tfex-cosmos-cassandra-keyspace",
* resourceGroupName: exampleAccount.resourceGroupName,
* accountName: exampleAccount.name,
* throughput: 400,
* });
* const exampleCassandraTable = new azure.cosmosdb.CassandraTable("example", {
* name: "testtable",
* cassandraKeyspaceId: exampleCassandraKeyspace.id,
* schema: {
* columns: [
* {
* name: "test1",
* type: "ascii",
* },
* {
* name: "test2",
* type: "int",
* },
* ],
* partitionKeys: [{
* name: "test1",
* }],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="tflex-cosmosdb-account-rg",
* location="West Europe")
* example_account = azure.cosmosdb.Account("example",
* name="tfex-cosmosdb-account",
* resource_group_name=example.name,
* location=example.location,
* offer_type="Standard",
* capabilities=[{
* "name": "EnableCassandra",
* }],
* consistency_policy={
* "consistency_level": "Strong",
* },
* geo_locations=[{
* "location": example.location,
* "failover_priority": 0,
* }])
* example_cassandra_keyspace = azure.cosmosdb.CassandraKeyspace("example",
* name="tfex-cosmos-cassandra-keyspace",
* resource_group_name=example_account.resource_group_name,
* account_name=example_account.name,
* throughput=400)
* example_cassandra_table = azure.cosmosdb.CassandraTable("example",
* name="testtable",
* cassandra_keyspace_id=example_cassandra_keyspace.id,
* schema={
* "columns": [
* {
* "name": "test1",
* "type": "ascii",
* },
* {
* "name": "test2",
* "type": "int",
* },
* ],
* "partition_keys": [{
* "name": "test1",
* }],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "tflex-cosmosdb-account-rg",
* Location = "West Europe",
* });
* var exampleAccount = new Azure.CosmosDB.Account("example", new()
* {
* Name = "tfex-cosmosdb-account",
* ResourceGroupName = example.Name,
* Location = example.Location,
* OfferType = "Standard",
* Capabilities = new[]
* {
* new Azure.CosmosDB.Inputs.AccountCapabilityArgs
* {
* Name = "EnableCassandra",
* },
* },
* ConsistencyPolicy = new Azure.CosmosDB.Inputs.AccountConsistencyPolicyArgs
* {
* ConsistencyLevel = "Strong",
* },
* GeoLocations = new[]
* {
* new Azure.CosmosDB.Inputs.AccountGeoLocationArgs
* {
* Location = example.Location,
* FailoverPriority = 0,
* },
* },
* });
* var exampleCassandraKeyspace = new Azure.CosmosDB.CassandraKeyspace("example", new()
* {
* Name = "tfex-cosmos-cassandra-keyspace",
* ResourceGroupName = exampleAccount.ResourceGroupName,
* AccountName = exampleAccount.Name,
* Throughput = 400,
* });
* var exampleCassandraTable = new Azure.CosmosDB.CassandraTable("example", new()
* {
* Name = "testtable",
* CassandraKeyspaceId = exampleCassandraKeyspace.Id,
* Schema = new Azure.CosmosDB.Inputs.CassandraTableSchemaArgs
* {
* Columns = new[]
* {
* new Azure.CosmosDB.Inputs.CassandraTableSchemaColumnArgs
* {
* Name = "test1",
* Type = "ascii",
* },
* new Azure.CosmosDB.Inputs.CassandraTableSchemaColumnArgs
* {
* Name = "test2",
* Type = "int",
* },
* },
* PartitionKeys = new[]
* {
* new Azure.CosmosDB.Inputs.CassandraTableSchemaPartitionKeyArgs
* {
* Name = "test1",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/cosmosdb"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("tflex-cosmosdb-account-rg"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleAccount, err := cosmosdb.NewAccount(ctx, "example", &cosmosdb.AccountArgs{
* Name: pulumi.String("tfex-cosmosdb-account"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* OfferType: pulumi.String("Standard"),
* Capabilities: cosmosdb.AccountCapabilityArray{
* &cosmosdb.AccountCapabilityArgs{
* Name: pulumi.String("EnableCassandra"),
* },
* },
* ConsistencyPolicy: &cosmosdb.AccountConsistencyPolicyArgs{
* ConsistencyLevel: pulumi.String("Strong"),
* },
* GeoLocations: cosmosdb.AccountGeoLocationArray{
* &cosmosdb.AccountGeoLocationArgs{
* Location: example.Location,
* FailoverPriority: pulumi.Int(0),
* },
* },
* })
* if err != nil {
* return err
* }
* exampleCassandraKeyspace, err := cosmosdb.NewCassandraKeyspace(ctx, "example", &cosmosdb.CassandraKeyspaceArgs{
* Name: pulumi.String("tfex-cosmos-cassandra-keyspace"),
* ResourceGroupName: exampleAccount.ResourceGroupName,
* AccountName: exampleAccount.Name,
* Throughput: pulumi.Int(400),
* })
* if err != nil {
* return err
* }
* _, err = cosmosdb.NewCassandraTable(ctx, "example", &cosmosdb.CassandraTableArgs{
* Name: pulumi.String("testtable"),
* CassandraKeyspaceId: exampleCassandraKeyspace.ID(),
* Schema: &cosmosdb.CassandraTableSchemaArgs{
* Columns: cosmosdb.CassandraTableSchemaColumnArray{
* &cosmosdb.CassandraTableSchemaColumnArgs{
* Name: pulumi.String("test1"),
* Type: pulumi.String("ascii"),
* },
* &cosmosdb.CassandraTableSchemaColumnArgs{
* Name: pulumi.String("test2"),
* Type: pulumi.String("int"),
* },
* },
* PartitionKeys: cosmosdb.CassandraTableSchemaPartitionKeyArray{
* &cosmosdb.CassandraTableSchemaPartitionKeyArgs{
* Name: pulumi.String("test1"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.cosmosdb.Account;
* import com.pulumi.azure.cosmosdb.AccountArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountCapabilityArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountConsistencyPolicyArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountGeoLocationArgs;
* import com.pulumi.azure.cosmosdb.CassandraKeyspace;
* import com.pulumi.azure.cosmosdb.CassandraKeyspaceArgs;
* import com.pulumi.azure.cosmosdb.CassandraTable;
* import com.pulumi.azure.cosmosdb.CassandraTableArgs;
* import com.pulumi.azure.cosmosdb.inputs.CassandraTableSchemaArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("tflex-cosmosdb-account-rg")
* .location("West Europe")
* .build());
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("tfex-cosmosdb-account")
* .resourceGroupName(example.name())
* .location(example.location())
* .offerType("Standard")
* .capabilities(AccountCapabilityArgs.builder()
* .name("EnableCassandra")
* .build())
* .consistencyPolicy(AccountConsistencyPolicyArgs.builder()
* .consistencyLevel("Strong")
* .build())
* .geoLocations(AccountGeoLocationArgs.builder()
* .location(example.location())
* .failoverPriority(0)
* .build())
* .build());
* var exampleCassandraKeyspace = new CassandraKeyspace("exampleCassandraKeyspace", CassandraKeyspaceArgs.builder()
* .name("tfex-cosmos-cassandra-keyspace")
* .resourceGroupName(exampleAccount.resourceGroupName())
* .accountName(exampleAccount.name())
* .throughput(400)
* .build());
* var exampleCassandraTable = new CassandraTable("exampleCassandraTable", CassandraTableArgs.builder()
* .name("testtable")
* .cassandraKeyspaceId(exampleCassandraKeyspace.id())
* .schema(CassandraTableSchemaArgs.builder()
* .columns(
* CassandraTableSchemaColumnArgs.builder()
* .name("test1")
* .type("ascii")
* .build(),
* CassandraTableSchemaColumnArgs.builder()
* .name("test2")
* .type("int")
* .build())
* .partitionKeys(CassandraTableSchemaPartitionKeyArgs.builder()
* .name("test1")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: tflex-cosmosdb-account-rg
* location: West Europe
* exampleAccount:
* type: azure:cosmosdb:Account
* name: example
* properties:
* name: tfex-cosmosdb-account
* resourceGroupName: ${example.name}
* location: ${example.location}
* offerType: Standard
* capabilities:
* - name: EnableCassandra
* consistencyPolicy:
* consistencyLevel: Strong
* geoLocations:
* - location: ${example.location}
* failoverPriority: 0
* exampleCassandraKeyspace:
* type: azure:cosmosdb:CassandraKeyspace
* name: example
* properties:
* name: tfex-cosmos-cassandra-keyspace
* resourceGroupName: ${exampleAccount.resourceGroupName}
* accountName: ${exampleAccount.name}
* throughput: 400
* exampleCassandraTable:
* type: azure:cosmosdb:CassandraTable
* name: example
* properties:
* name: testtable
* cassandraKeyspaceId: ${exampleCassandraKeyspace.id}
* schema:
* columns:
* - name: test1
* type: ascii
* - name: test2
* type: int
* partitionKeys:
* - name: test1
* ```
*
* ## Import
* Cosmos Cassandra Table can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:cosmosdb/cassandraTable:CassandraTable ks1 /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DocumentDB/databaseAccounts/account1/cassandraKeyspaces/ks1/tables/table1
* ```
* @property analyticalStorageTtl Time to live of the Analytical Storage. Possible values are between `-1` and `2147483647` except `0`. `-1` means the Analytical Storage never expires. Changing this forces a new resource to be created.
* > **Note:** throughput has a maximum value of `1000000` unless a higher limit is requested via Azure Support
* @property autoscaleSettings
* @property cassandraKeyspaceId The ID of the Cosmos DB Cassandra Keyspace to create the table within. Changing this forces a new resource to be created.
* @property defaultTtl Time to live of the Cosmos DB Cassandra table. Possible values are at least `-1`. `-1` means the Cassandra table never expires.
* @property name Specifies the name of the Cosmos DB Cassandra Table. Changing this forces a new resource to be created.
* @property schema A `schema` block as defined below.
* @property throughput
*/
public data class CassandraTableArgs(
public val analyticalStorageTtl: Output? = null,
public val autoscaleSettings: Output? = null,
public val cassandraKeyspaceId: Output? = null,
public val defaultTtl: Output? = null,
public val name: Output? = null,
public val schema: Output? = null,
public val throughput: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.cosmosdb.CassandraTableArgs =
com.pulumi.azure.cosmosdb.CassandraTableArgs.builder()
.analyticalStorageTtl(analyticalStorageTtl?.applyValue({ args0 -> args0 }))
.autoscaleSettings(autoscaleSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.cassandraKeyspaceId(cassandraKeyspaceId?.applyValue({ args0 -> args0 }))
.defaultTtl(defaultTtl?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.schema(schema?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.throughput(throughput?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CassandraTableArgs].
*/
@PulumiTagMarker
public class CassandraTableArgsBuilder internal constructor() {
private var analyticalStorageTtl: Output? = null
private var autoscaleSettings: Output? = null
private var cassandraKeyspaceId: Output? = null
private var defaultTtl: Output? = null
private var name: Output? = null
private var schema: Output? = null
private var throughput: Output? = null
/**
* @param value Time to live of the Analytical Storage. Possible values are between `-1` and `2147483647` except `0`. `-1` means the Analytical Storage never expires. Changing this forces a new resource to be created.
* > **Note:** throughput has a maximum value of `1000000` unless a higher limit is requested via Azure Support
*/
@JvmName("qepwngeccabaamec")
public suspend fun analyticalStorageTtl(`value`: Output) {
this.analyticalStorageTtl = value
}
/**
* @param value
*/
@JvmName("bjxwumlrwtyrqbqq")
public suspend fun autoscaleSettings(`value`: Output) {
this.autoscaleSettings = value
}
/**
* @param value The ID of the Cosmos DB Cassandra Keyspace to create the table within. Changing this forces a new resource to be created.
*/
@JvmName("nlqnlvaashqounkg")
public suspend fun cassandraKeyspaceId(`value`: Output) {
this.cassandraKeyspaceId = value
}
/**
* @param value Time to live of the Cosmos DB Cassandra table. Possible values are at least `-1`. `-1` means the Cassandra table never expires.
*/
@JvmName("hmbhgmjexcbkgcbp")
public suspend fun defaultTtl(`value`: Output) {
this.defaultTtl = value
}
/**
* @param value Specifies the name of the Cosmos DB Cassandra Table. Changing this forces a new resource to be created.
*/
@JvmName("pceghprdjrhfiklp")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value A `schema` block as defined below.
*/
@JvmName("hkmweqauhoepridc")
public suspend fun schema(`value`: Output) {
this.schema = value
}
/**
* @param value
*/
@JvmName("pobcociniqymuvfl")
public suspend fun throughput(`value`: Output) {
this.throughput = value
}
/**
* @param value Time to live of the Analytical Storage. Possible values are between `-1` and `2147483647` except `0`. `-1` means the Analytical Storage never expires. Changing this forces a new resource to be created.
* > **Note:** throughput has a maximum value of `1000000` unless a higher limit is requested via Azure Support
*/
@JvmName("foiafkahiojgmhft")
public suspend fun analyticalStorageTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.analyticalStorageTtl = mapped
}
/**
* @param value
*/
@JvmName("ymrfgardkuvyyqvy")
public suspend fun autoscaleSettings(`value`: CassandraTableAutoscaleSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autoscaleSettings = mapped
}
/**
* @param argument
*/
@JvmName("vmrofyddeytdbaue")
public suspend fun autoscaleSettings(argument: suspend CassandraTableAutoscaleSettingsArgsBuilder.() -> Unit) {
val toBeMapped = CassandraTableAutoscaleSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.autoscaleSettings = mapped
}
/**
* @param value The ID of the Cosmos DB Cassandra Keyspace to create the table within. Changing this forces a new resource to be created.
*/
@JvmName("vqabepebiklulass")
public suspend fun cassandraKeyspaceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cassandraKeyspaceId = mapped
}
/**
* @param value Time to live of the Cosmos DB Cassandra table. Possible values are at least `-1`. `-1` means the Cassandra table never expires.
*/
@JvmName("jotgkqpvnfihqbul")
public suspend fun defaultTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultTtl = mapped
}
/**
* @param value Specifies the name of the Cosmos DB Cassandra Table. Changing this forces a new resource to be created.
*/
@JvmName("kbcmhywnlnykcvha")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value A `schema` block as defined below.
*/
@JvmName("dqciqnewjqyyxdfb")
public suspend fun schema(`value`: CassandraTableSchemaArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.schema = mapped
}
/**
* @param argument A `schema` block as defined below.
*/
@JvmName("avpqrcwxxhpmfbdx")
public suspend fun schema(argument: suspend CassandraTableSchemaArgsBuilder.() -> Unit) {
val toBeMapped = CassandraTableSchemaArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.schema = mapped
}
/**
* @param value
*/
@JvmName("bppmfkdtcupsnsje")
public suspend fun throughput(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.throughput = mapped
}
internal fun build(): CassandraTableArgs = CassandraTableArgs(
analyticalStorageTtl = analyticalStorageTtl,
autoscaleSettings = autoscaleSettings,
cassandraKeyspaceId = cassandraKeyspaceId,
defaultTtl = defaultTtl,
name = name,
schema = schema,
throughput = throughput,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy