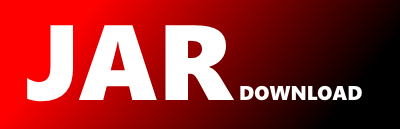
com.pulumi.azure.datafactory.kotlin.LinkedServiceSqlServer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.datafactory.kotlin
import com.pulumi.azure.datafactory.kotlin.outputs.LinkedServiceSqlServerKeyVaultConnectionString
import com.pulumi.azure.datafactory.kotlin.outputs.LinkedServiceSqlServerKeyVaultPassword
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azure.datafactory.kotlin.outputs.LinkedServiceSqlServerKeyVaultConnectionString.Companion.toKotlin as linkedServiceSqlServerKeyVaultConnectionStringToKotlin
import com.pulumi.azure.datafactory.kotlin.outputs.LinkedServiceSqlServerKeyVaultPassword.Companion.toKotlin as linkedServiceSqlServerKeyVaultPasswordToKotlin
/**
* Builder for [LinkedServiceSqlServer].
*/
@PulumiTagMarker
public class LinkedServiceSqlServerResourceBuilder internal constructor() {
public var name: String? = null
public var args: LinkedServiceSqlServerArgs = LinkedServiceSqlServerArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend LinkedServiceSqlServerArgsBuilder.() -> Unit) {
val builder = LinkedServiceSqlServerArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): LinkedServiceSqlServer {
val builtJavaResource =
com.pulumi.azure.datafactory.LinkedServiceSqlServer(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return LinkedServiceSqlServer(builtJavaResource)
}
}
/**
* Manages a Linked Service (connection) between a SQL Server and Azure Data Factory.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleFactory = new azure.datafactory.Factory("example", {
* name: "example",
* location: example.location,
* resourceGroupName: example.name,
* });
* const exampleLinkedServiceSqlServer = new azure.datafactory.LinkedServiceSqlServer("example", {
* name: "example",
* dataFactoryId: exampleFactory.id,
* connectionString: "Integrated Security=False;Data Source=test;Initial Catalog=test;User ID=test;Password=test",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_factory = azure.datafactory.Factory("example",
* name="example",
* location=example.location,
* resource_group_name=example.name)
* example_linked_service_sql_server = azure.datafactory.LinkedServiceSqlServer("example",
* name="example",
* data_factory_id=example_factory.id,
* connection_string="Integrated Security=False;Data Source=test;Initial Catalog=test;User ID=test;Password=test")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleFactory = new Azure.DataFactory.Factory("example", new()
* {
* Name = "example",
* Location = example.Location,
* ResourceGroupName = example.Name,
* });
* var exampleLinkedServiceSqlServer = new Azure.DataFactory.LinkedServiceSqlServer("example", new()
* {
* Name = "example",
* DataFactoryId = exampleFactory.Id,
* ConnectionString = "Integrated Security=False;Data Source=test;Initial Catalog=test;User ID=test;Password=test",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/datafactory"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleFactory, err := datafactory.NewFactory(ctx, "example", &datafactory.FactoryArgs{
* Name: pulumi.String("example"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* })
* if err != nil {
* return err
* }
* _, err = datafactory.NewLinkedServiceSqlServer(ctx, "example", &datafactory.LinkedServiceSqlServerArgs{
* Name: pulumi.String("example"),
* DataFactoryId: exampleFactory.ID(),
* ConnectionString: pulumi.String("Integrated Security=False;Data Source=test;Initial Catalog=test;User ID=test;Password=test"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.datafactory.Factory;
* import com.pulumi.azure.datafactory.FactoryArgs;
* import com.pulumi.azure.datafactory.LinkedServiceSqlServer;
* import com.pulumi.azure.datafactory.LinkedServiceSqlServerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleFactory = new Factory("exampleFactory", FactoryArgs.builder()
* .name("example")
* .location(example.location())
* .resourceGroupName(example.name())
* .build());
* var exampleLinkedServiceSqlServer = new LinkedServiceSqlServer("exampleLinkedServiceSqlServer", LinkedServiceSqlServerArgs.builder()
* .name("example")
* .dataFactoryId(exampleFactory.id())
* .connectionString("Integrated Security=False;Data Source=test;Initial Catalog=test;User ID=test;Password=test")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleFactory:
* type: azure:datafactory:Factory
* name: example
* properties:
* name: example
* location: ${example.location}
* resourceGroupName: ${example.name}
* exampleLinkedServiceSqlServer:
* type: azure:datafactory:LinkedServiceSqlServer
* name: example
* properties:
* name: example
* dataFactoryId: ${exampleFactory.id}
* connectionString: Integrated Security=False;Data Source=test;Initial Catalog=test;User ID=test;Password=test
* ```
*
* ### With Password In Key Vault
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const current = azure.core.getClientConfig({});
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleKeyVault = new azure.keyvault.KeyVault("example", {
* name: "example",
* location: example.location,
* resourceGroupName: example.name,
* tenantId: current.then(current => current.tenantId),
* skuName: "standard",
* });
* const exampleFactory = new azure.datafactory.Factory("example", {
* name: "example",
* location: example.location,
* resourceGroupName: example.name,
* });
* const exampleLinkedServiceKeyVault = new azure.datafactory.LinkedServiceKeyVault("example", {
* name: "kvlink",
* dataFactoryId: exampleFactory.id,
* keyVaultId: exampleKeyVault.id,
* });
* const exampleLinkedServiceSqlServer = new azure.datafactory.LinkedServiceSqlServer("example", {
* name: "example",
* dataFactoryId: exampleFactory.id,
* connectionString: "Integrated Security=False;Data Source=test;Initial Catalog=test;User ID=test;",
* keyVaultPassword: {
* linkedServiceName: exampleLinkedServiceKeyVault.name,
* secretName: "secret",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* current = azure.core.get_client_config()
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_key_vault = azure.keyvault.KeyVault("example",
* name="example",
* location=example.location,
* resource_group_name=example.name,
* tenant_id=current.tenant_id,
* sku_name="standard")
* example_factory = azure.datafactory.Factory("example",
* name="example",
* location=example.location,
* resource_group_name=example.name)
* example_linked_service_key_vault = azure.datafactory.LinkedServiceKeyVault("example",
* name="kvlink",
* data_factory_id=example_factory.id,
* key_vault_id=example_key_vault.id)
* example_linked_service_sql_server = azure.datafactory.LinkedServiceSqlServer("example",
* name="example",
* data_factory_id=example_factory.id,
* connection_string="Integrated Security=False;Data Source=test;Initial Catalog=test;User ID=test;",
* key_vault_password={
* "linked_service_name": example_linked_service_key_vault.name,
* "secret_name": "secret",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var current = Azure.Core.GetClientConfig.Invoke();
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleKeyVault = new Azure.KeyVault.KeyVault("example", new()
* {
* Name = "example",
* Location = example.Location,
* ResourceGroupName = example.Name,
* TenantId = current.Apply(getClientConfigResult => getClientConfigResult.TenantId),
* SkuName = "standard",
* });
* var exampleFactory = new Azure.DataFactory.Factory("example", new()
* {
* Name = "example",
* Location = example.Location,
* ResourceGroupName = example.Name,
* });
* var exampleLinkedServiceKeyVault = new Azure.DataFactory.LinkedServiceKeyVault("example", new()
* {
* Name = "kvlink",
* DataFactoryId = exampleFactory.Id,
* KeyVaultId = exampleKeyVault.Id,
* });
* var exampleLinkedServiceSqlServer = new Azure.DataFactory.LinkedServiceSqlServer("example", new()
* {
* Name = "example",
* DataFactoryId = exampleFactory.Id,
* ConnectionString = "Integrated Security=False;Data Source=test;Initial Catalog=test;User ID=test;",
* KeyVaultPassword = new Azure.DataFactory.Inputs.LinkedServiceSqlServerKeyVaultPasswordArgs
* {
* LinkedServiceName = exampleLinkedServiceKeyVault.Name,
* SecretName = "secret",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/datafactory"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/keyvault"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* current, err := core.GetClientConfig(ctx, nil, nil)
* if err != nil {
* return err
* }
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleKeyVault, err := keyvault.NewKeyVault(ctx, "example", &keyvault.KeyVaultArgs{
* Name: pulumi.String("example"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* TenantId: pulumi.String(current.TenantId),
* SkuName: pulumi.String("standard"),
* })
* if err != nil {
* return err
* }
* exampleFactory, err := datafactory.NewFactory(ctx, "example", &datafactory.FactoryArgs{
* Name: pulumi.String("example"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* })
* if err != nil {
* return err
* }
* exampleLinkedServiceKeyVault, err := datafactory.NewLinkedServiceKeyVault(ctx, "example", &datafactory.LinkedServiceKeyVaultArgs{
* Name: pulumi.String("kvlink"),
* DataFactoryId: exampleFactory.ID(),
* KeyVaultId: exampleKeyVault.ID(),
* })
* if err != nil {
* return err
* }
* _, err = datafactory.NewLinkedServiceSqlServer(ctx, "example", &datafactory.LinkedServiceSqlServerArgs{
* Name: pulumi.String("example"),
* DataFactoryId: exampleFactory.ID(),
* ConnectionString: pulumi.String("Integrated Security=False;Data Source=test;Initial Catalog=test;User ID=test;"),
* KeyVaultPassword: &datafactory.LinkedServiceSqlServerKeyVaultPasswordArgs{
* LinkedServiceName: exampleLinkedServiceKeyVault.Name,
* SecretName: pulumi.String("secret"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.keyvault.KeyVault;
* import com.pulumi.azure.keyvault.KeyVaultArgs;
* import com.pulumi.azure.datafactory.Factory;
* import com.pulumi.azure.datafactory.FactoryArgs;
* import com.pulumi.azure.datafactory.LinkedServiceKeyVault;
* import com.pulumi.azure.datafactory.LinkedServiceKeyVaultArgs;
* import com.pulumi.azure.datafactory.LinkedServiceSqlServer;
* import com.pulumi.azure.datafactory.LinkedServiceSqlServerArgs;
* import com.pulumi.azure.datafactory.inputs.LinkedServiceSqlServerKeyVaultPasswordArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = CoreFunctions.getClientConfig();
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleKeyVault = new KeyVault("exampleKeyVault", KeyVaultArgs.builder()
* .name("example")
* .location(example.location())
* .resourceGroupName(example.name())
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .skuName("standard")
* .build());
* var exampleFactory = new Factory("exampleFactory", FactoryArgs.builder()
* .name("example")
* .location(example.location())
* .resourceGroupName(example.name())
* .build());
* var exampleLinkedServiceKeyVault = new LinkedServiceKeyVault("exampleLinkedServiceKeyVault", LinkedServiceKeyVaultArgs.builder()
* .name("kvlink")
* .dataFactoryId(exampleFactory.id())
* .keyVaultId(exampleKeyVault.id())
* .build());
* var exampleLinkedServiceSqlServer = new LinkedServiceSqlServer("exampleLinkedServiceSqlServer", LinkedServiceSqlServerArgs.builder()
* .name("example")
* .dataFactoryId(exampleFactory.id())
* .connectionString("Integrated Security=False;Data Source=test;Initial Catalog=test;User ID=test;")
* .keyVaultPassword(LinkedServiceSqlServerKeyVaultPasswordArgs.builder()
* .linkedServiceName(exampleLinkedServiceKeyVault.name())
* .secretName("secret")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleKeyVault:
* type: azure:keyvault:KeyVault
* name: example
* properties:
* name: example
* location: ${example.location}
* resourceGroupName: ${example.name}
* tenantId: ${current.tenantId}
* skuName: standard
* exampleFactory:
* type: azure:datafactory:Factory
* name: example
* properties:
* name: example
* location: ${example.location}
* resourceGroupName: ${example.name}
* exampleLinkedServiceKeyVault:
* type: azure:datafactory:LinkedServiceKeyVault
* name: example
* properties:
* name: kvlink
* dataFactoryId: ${exampleFactory.id}
* keyVaultId: ${exampleKeyVault.id}
* exampleLinkedServiceSqlServer:
* type: azure:datafactory:LinkedServiceSqlServer
* name: example
* properties:
* name: example
* dataFactoryId: ${exampleFactory.id}
* connectionString: Integrated Security=False;Data Source=test;Initial Catalog=test;User ID=test;
* keyVaultPassword:
* linkedServiceName: ${exampleLinkedServiceKeyVault.name}
* secretName: secret
* variables:
* current:
* fn::invoke:
* Function: azure:core:getClientConfig
* Arguments: {}
* ```
*
* ## Import
* Data Factory SQL Server Linked Service's can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:datafactory/linkedServiceSqlServer:LinkedServiceSqlServer example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/example/providers/Microsoft.DataFactory/factories/example/linkedservices/example
* ```
*/
public class LinkedServiceSqlServer internal constructor(
override val javaResource: com.pulumi.azure.datafactory.LinkedServiceSqlServer,
) : KotlinCustomResource(javaResource, LinkedServiceSqlServerMapper) {
/**
* A map of additional properties to associate with the Data Factory Linked Service SQL Server.
*/
public val additionalProperties: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy