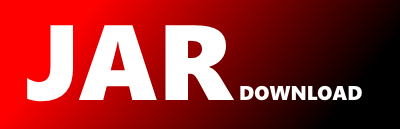
com.pulumi.azure.datafactory.kotlin.inputs.TriggerScheduleScheduleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.datafactory.kotlin.inputs
import com.pulumi.azure.datafactory.inputs.TriggerScheduleScheduleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property daysOfMonths Day(s) of the month on which the trigger is scheduled. This value can be specified with a monthly frequency only.
* @property daysOfWeeks Days of the week on which the trigger is scheduled. This value can be specified only with a weekly frequency.
* @property hours Hours of the day on which the trigger is scheduled.
* @property minutes Minutes of the hour on which the trigger is scheduled.
* @property monthlies A `monthly` block as documented below, which specifies the days of the month on which the trigger is scheduled. The value can be specified only with a monthly frequency.
*/
public data class TriggerScheduleScheduleArgs(
public val daysOfMonths: Output>? = null,
public val daysOfWeeks: Output>? = null,
public val hours: Output>? = null,
public val minutes: Output>? = null,
public val monthlies: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.datafactory.inputs.TriggerScheduleScheduleArgs =
com.pulumi.azure.datafactory.inputs.TriggerScheduleScheduleArgs.builder()
.daysOfMonths(daysOfMonths?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.daysOfWeeks(daysOfWeeks?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.hours(hours?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.minutes(minutes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.monthlies(
monthlies?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [TriggerScheduleScheduleArgs].
*/
@PulumiTagMarker
public class TriggerScheduleScheduleArgsBuilder internal constructor() {
private var daysOfMonths: Output>? = null
private var daysOfWeeks: Output>? = null
private var hours: Output>? = null
private var minutes: Output>? = null
private var monthlies: Output>? = null
/**
* @param value Day(s) of the month on which the trigger is scheduled. This value can be specified with a monthly frequency only.
*/
@JvmName("jyiccmbwbwtfcqqb")
public suspend fun daysOfMonths(`value`: Output>) {
this.daysOfMonths = value
}
@JvmName("tatbigeebblrtspl")
public suspend fun daysOfMonths(vararg values: Output) {
this.daysOfMonths = Output.all(values.asList())
}
/**
* @param values Day(s) of the month on which the trigger is scheduled. This value can be specified with a monthly frequency only.
*/
@JvmName("iankuxqafqrpicrx")
public suspend fun daysOfMonths(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy