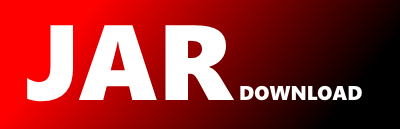
com.pulumi.azure.dataprotection.kotlin.BackupPolicyKubernetesClusterArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.dataprotection.kotlin
import com.pulumi.azure.dataprotection.BackupPolicyKubernetesClusterArgs.builder
import com.pulumi.azure.dataprotection.kotlin.inputs.BackupPolicyKubernetesClusterDefaultRetentionRuleArgs
import com.pulumi.azure.dataprotection.kotlin.inputs.BackupPolicyKubernetesClusterDefaultRetentionRuleArgsBuilder
import com.pulumi.azure.dataprotection.kotlin.inputs.BackupPolicyKubernetesClusterRetentionRuleArgs
import com.pulumi.azure.dataprotection.kotlin.inputs.BackupPolicyKubernetesClusterRetentionRuleArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages a Backup Policy to back up Kubernetes Cluster.
* ## Example Usage
*
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleBackupVault:
* type: azure:dataprotection:BackupVault
* name: example
* properties:
* name: example-backup-vault
* resourceGroupName: ${example.name}
* location: ${example.location}
* datastoreType: VaultStore
* redundancy: LocallyRedundant
* exampleBackupPolicyKubernetesCluster:
* type: azure:dataprotection:BackupPolicyKubernetesCluster
* name: example
* properties:
* name: example-backup-policy
* resourceGroupName: ${example.name}
* vaultName: ${exampleBackupVault.name}
* backupRepeatingTimeIntervals:
* - R/2021-05-23T02:30:00+00:00/P1W
* timeZone: India Standard Time
* defaultRetentionDuration: P4M
* retentionRules:
* - name: Daily
* priority: 25
* lifeCycles:
* - duration: P84D
* dataStoreType: OperationalStore
* criteria:
* absoluteCriteria: FirstOfDay
* defaultRetentionRule:
* lifeCycles:
* - duration: P7D
* dataStoreType: OperationalStore
* ```
*
* ## Import
* Backup Policy Kubernetes Cluster's can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:dataprotection/backupPolicyKubernetesCluster:BackupPolicyKubernetesCluster example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.DataProtection/backupVaults/vault1/backupPolicies/backupPolicy1
* ```
* @property backupRepeatingTimeIntervals Specifies a list of repeating time interval. It supports weekly back. It should follow `ISO 8601` repeating time interval. Changing this forces a new resource to be created.
* @property defaultRetentionRule A `default_retention_rule` block as defined below. Changing this forces a new resource to be created.
* @property name The name which should be used for the Backup Policy Kubernetes Cluster. Changing this forces a new resource to be created.
* @property resourceGroupName The name of the Resource Group where the Backup Policy Kubernetes Cluster should exist. Changing this forces a new resource to be created.
* @property retentionRules One or more `retention_rule` blocks as defined below. Changing this forces a new resource to be created.
* @property timeZone Specifies the Time Zone which should be used by the backup schedule. Changing this forces a new resource to be created.
* @property vaultName The name of the Backup Vault where the Backup Policy Kubernetes Cluster should exist. Changing this forces a new resource to be created.
*/
public data class BackupPolicyKubernetesClusterArgs(
public val backupRepeatingTimeIntervals: Output>? = null,
public val defaultRetentionRule: Output? =
null,
public val name: Output? = null,
public val resourceGroupName: Output? = null,
public val retentionRules: Output>? = null,
public val timeZone: Output? = null,
public val vaultName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.dataprotection.BackupPolicyKubernetesClusterArgs =
com.pulumi.azure.dataprotection.BackupPolicyKubernetesClusterArgs.builder()
.backupRepeatingTimeIntervals(
backupRepeatingTimeIntervals?.applyValue({ args0 ->
args0.map({ args0 -> args0 })
}),
)
.defaultRetentionRule(
defaultRetentionRule?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.retentionRules(
retentionRules?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.timeZone(timeZone?.applyValue({ args0 -> args0 }))
.vaultName(vaultName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackupPolicyKubernetesClusterArgs].
*/
@PulumiTagMarker
public class BackupPolicyKubernetesClusterArgsBuilder internal constructor() {
private var backupRepeatingTimeIntervals: Output>? = null
private var defaultRetentionRule: Output? =
null
private var name: Output? = null
private var resourceGroupName: Output? = null
private var retentionRules: Output>? = null
private var timeZone: Output? = null
private var vaultName: Output? = null
/**
* @param value Specifies a list of repeating time interval. It supports weekly back. It should follow `ISO 8601` repeating time interval. Changing this forces a new resource to be created.
*/
@JvmName("qpctbbyidbkiebqw")
public suspend fun backupRepeatingTimeIntervals(`value`: Output>) {
this.backupRepeatingTimeIntervals = value
}
@JvmName("cwxjlaqpuekngfui")
public suspend fun backupRepeatingTimeIntervals(vararg values: Output) {
this.backupRepeatingTimeIntervals = Output.all(values.asList())
}
/**
* @param values Specifies a list of repeating time interval. It supports weekly back. It should follow `ISO 8601` repeating time interval. Changing this forces a new resource to be created.
*/
@JvmName("vqkpkktuoigahpbj")
public suspend fun backupRepeatingTimeIntervals(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy