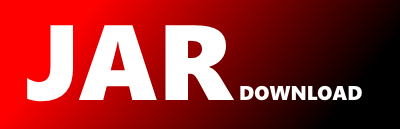
com.pulumi.azure.dataprotection.kotlin.inputs.BackupInstanceKubernetesClusterBackupDatasourceParametersArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.dataprotection.kotlin.inputs
import com.pulumi.azure.dataprotection.inputs.BackupInstanceKubernetesClusterBackupDatasourceParametersArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property clusterScopedResourcesEnabled Whether to include cluster scope resources during backup. Default to `false`. Changing this forces a new resource to be created.
* @property excludedNamespaces Specifies the namespaces to be excluded during backup. Changing this forces a new resource to be created.
* @property excludedResourceTypes Specifies the resource types to be excluded during backup. Changing this forces a new resource to be created.
* @property includedNamespaces Specifies the namespaces to be included during backup. Changing this forces a new resource to be created.
* @property includedResourceTypes Specifies the resource types to be included during backup. Changing this forces a new resource to be created.
* @property labelSelectors Specifies the resources with such label selectors to be included during backup. Changing this forces a new resource to be created.
* @property volumeSnapshotEnabled Whether to take volume snapshots during backup. Default to `false`. Changing this forces a new resource to be created.
*/
public data class BackupInstanceKubernetesClusterBackupDatasourceParametersArgs(
public val clusterScopedResourcesEnabled: Output? = null,
public val excludedNamespaces: Output>? = null,
public val excludedResourceTypes: Output>? = null,
public val includedNamespaces: Output>? = null,
public val includedResourceTypes: Output>? = null,
public val labelSelectors: Output>? = null,
public val volumeSnapshotEnabled: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azure.dataprotection.inputs.BackupInstanceKubernetesClusterBackupDatasourceParametersArgs =
com.pulumi.azure.dataprotection.inputs.BackupInstanceKubernetesClusterBackupDatasourceParametersArgs.builder()
.clusterScopedResourcesEnabled(clusterScopedResourcesEnabled?.applyValue({ args0 -> args0 }))
.excludedNamespaces(excludedNamespaces?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.excludedResourceTypes(excludedResourceTypes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.includedNamespaces(includedNamespaces?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.includedResourceTypes(includedResourceTypes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.labelSelectors(labelSelectors?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.volumeSnapshotEnabled(volumeSnapshotEnabled?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackupInstanceKubernetesClusterBackupDatasourceParametersArgs].
*/
@PulumiTagMarker
public class BackupInstanceKubernetesClusterBackupDatasourceParametersArgsBuilder internal constructor() {
private var clusterScopedResourcesEnabled: Output? = null
private var excludedNamespaces: Output>? = null
private var excludedResourceTypes: Output>? = null
private var includedNamespaces: Output>? = null
private var includedResourceTypes: Output>? = null
private var labelSelectors: Output>? = null
private var volumeSnapshotEnabled: Output? = null
/**
* @param value Whether to include cluster scope resources during backup. Default to `false`. Changing this forces a new resource to be created.
*/
@JvmName("euxtlvyhpgclwger")
public suspend fun clusterScopedResourcesEnabled(`value`: Output) {
this.clusterScopedResourcesEnabled = value
}
/**
* @param value Specifies the namespaces to be excluded during backup. Changing this forces a new resource to be created.
*/
@JvmName("rlglatvqlpbjjrsw")
public suspend fun excludedNamespaces(`value`: Output>) {
this.excludedNamespaces = value
}
@JvmName("ecnpseglrihsorqx")
public suspend fun excludedNamespaces(vararg values: Output) {
this.excludedNamespaces = Output.all(values.asList())
}
/**
* @param values Specifies the namespaces to be excluded during backup. Changing this forces a new resource to be created.
*/
@JvmName("gntkepgmboswnrhg")
public suspend fun excludedNamespaces(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy