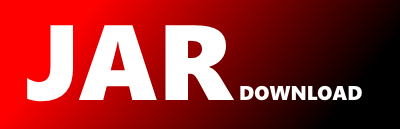
com.pulumi.azure.desktopvirtualization.kotlin.inputs.HostPoolScheduledAgentUpdatesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.desktopvirtualization.kotlin.inputs
import com.pulumi.azure.desktopvirtualization.inputs.HostPoolScheduledAgentUpdatesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property enabled Enables or disables scheduled updates of the AVD agent components (RDAgent, Geneva Monitoring agent, and side-by-side stack) on session hosts. If this is enabled then up to two `schedule` blocks must be defined. Default is `false`.
* > **NOTE:** if `enabled` is set to `true` then at least one and a maximum of two `schedule` blocks must be provided.
* @property schedules A `schedule` block as defined below. A maximum of two blocks can be added.
* @property timezone Specifies the time zone in which the agent update schedule will apply, [the possible values are defined here](https://jackstromberg.com/2017/01/list-of-time-zones-consumed-by-azure/). If `use_session_host_timezone` is enabled then it will override this setting. Default is `UTC`
* @property useSessionHostTimezone Specifies whether scheduled agent updates should be applied based on the timezone of the affected session host. If configured then this setting overrides `timezone`. Default is `false`.
*/
public data class HostPoolScheduledAgentUpdatesArgs(
public val enabled: Output? = null,
public val schedules: Output>? = null,
public val timezone: Output? = null,
public val useSessionHostTimezone: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azure.desktopvirtualization.inputs.HostPoolScheduledAgentUpdatesArgs =
com.pulumi.azure.desktopvirtualization.inputs.HostPoolScheduledAgentUpdatesArgs.builder()
.enabled(enabled?.applyValue({ args0 -> args0 }))
.schedules(
schedules?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.timezone(timezone?.applyValue({ args0 -> args0 }))
.useSessionHostTimezone(useSessionHostTimezone?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [HostPoolScheduledAgentUpdatesArgs].
*/
@PulumiTagMarker
public class HostPoolScheduledAgentUpdatesArgsBuilder internal constructor() {
private var enabled: Output? = null
private var schedules: Output>? = null
private var timezone: Output? = null
private var useSessionHostTimezone: Output? = null
/**
* @param value Enables or disables scheduled updates of the AVD agent components (RDAgent, Geneva Monitoring agent, and side-by-side stack) on session hosts. If this is enabled then up to two `schedule` blocks must be defined. Default is `false`.
* > **NOTE:** if `enabled` is set to `true` then at least one and a maximum of two `schedule` blocks must be provided.
*/
@JvmName("rqenctxlrmwxtfci")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value A `schedule` block as defined below. A maximum of two blocks can be added.
*/
@JvmName("loffhicuedjbpfyj")
public suspend fun schedules(`value`: Output>) {
this.schedules = value
}
@JvmName("lnqtmjjwdmvtbvvq")
public suspend fun schedules(vararg values: Output) {
this.schedules = Output.all(values.asList())
}
/**
* @param values A `schedule` block as defined below. A maximum of two blocks can be added.
*/
@JvmName("qavobtyrpkekyfku")
public suspend fun schedules(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy