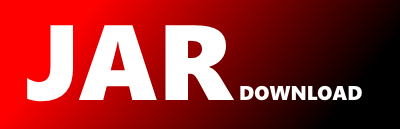
com.pulumi.azure.elasticsan.kotlin.ElasticsanFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.elasticsan.kotlin
import com.pulumi.azure.elasticsan.ElasticsanFunctions.getPlain
import com.pulumi.azure.elasticsan.ElasticsanFunctions.getVolumeGroupPlain
import com.pulumi.azure.elasticsan.ElasticsanFunctions.getVolumeSnapshotPlain
import com.pulumi.azure.elasticsan.kotlin.inputs.GetPlainArgs
import com.pulumi.azure.elasticsan.kotlin.inputs.GetPlainArgsBuilder
import com.pulumi.azure.elasticsan.kotlin.inputs.GetVolumeGroupPlainArgs
import com.pulumi.azure.elasticsan.kotlin.inputs.GetVolumeGroupPlainArgsBuilder
import com.pulumi.azure.elasticsan.kotlin.inputs.GetVolumeSnapshotPlainArgs
import com.pulumi.azure.elasticsan.kotlin.inputs.GetVolumeSnapshotPlainArgsBuilder
import com.pulumi.azure.elasticsan.kotlin.outputs.GetResult
import com.pulumi.azure.elasticsan.kotlin.outputs.GetVolumeGroupResult
import com.pulumi.azure.elasticsan.kotlin.outputs.GetVolumeSnapshotResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azure.elasticsan.kotlin.outputs.GetResult.Companion.toKotlin as getResultToKotlin
import com.pulumi.azure.elasticsan.kotlin.outputs.GetVolumeGroupResult.Companion.toKotlin as getVolumeGroupResultToKotlin
import com.pulumi.azure.elasticsan.kotlin.outputs.GetVolumeSnapshotResult.Companion.toKotlin as getVolumeSnapshotResultToKotlin
public object ElasticsanFunctions {
/**
* Use this data source to access information about an existing Elastic SAN.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.elasticsan.get({
* name: "existing",
* resourceGroupName: "existing",
* });
* export const id = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.elasticsan.get(name="existing",
* resource_group_name="existing")
* pulumi.export("id", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ElasticSan.Get.Invoke(new()
* {
* Name = "existing",
* ResourceGroupName = "existing",
* });
* return new Dictionary
* {
* ["id"] = example.Apply(getResult => getResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/elasticsan"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := elasticsan.Get(ctx, &elasticsan.GetArgs{
* Name: "existing",
* ResourceGroupName: "existing",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("id", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.elasticsan.ElasticsanFunctions;
* import com.pulumi.azure.elasticsan.inputs.GetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ElasticsanFunctions.get(GetArgs.builder()
* .name("existing")
* .resourceGroupName("existing")
* .build());
* ctx.export("id", example.applyValue(getResult -> getResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:elasticsan:get
* Arguments:
* name: existing
* resourceGroupName: existing
* outputs:
* id: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking get.
* @return A collection of values returned by get.
*/
public suspend fun `get`(argument: GetPlainArgs): GetResult =
getResultToKotlin(getPlain(argument.toJava()).await())
/**
* @see [get].
* @param name The name of this Elastic SAN.
* @param resourceGroupName The name of the Resource Group where the Elastic SAN exists.
* @return A collection of values returned by get.
*/
public suspend fun `get`(name: String, resourceGroupName: String): GetResult {
val argument = GetPlainArgs(
name = name,
resourceGroupName = resourceGroupName,
)
return getResultToKotlin(getPlain(argument.toJava()).await())
}
/**
* @see [get].
* @param argument Builder for [com.pulumi.azure.elasticsan.kotlin.inputs.GetPlainArgs].
* @return A collection of values returned by get.
*/
public suspend fun `get`(argument: suspend GetPlainArgsBuilder.() -> Unit): GetResult {
val builder = GetPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getResultToKotlin(getPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Elastic SAN Volume Group.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.elasticsan.get({
* name: "existing",
* resourceGroupName: "existing",
* });
* const exampleGetVolumeGroup = example.then(example => azure.elasticsan.getVolumeGroup({
* name: "existing",
* elasticSanId: example.id,
* }));
* export const id = exampleGetVolumeGroup.then(exampleGetVolumeGroup => exampleGetVolumeGroup.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.elasticsan.get(name="existing",
* resource_group_name="existing")
* example_get_volume_group = azure.elasticsan.get_volume_group(name="existing",
* elastic_san_id=example.id)
* pulumi.export("id", example_get_volume_group.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ElasticSan.Get.Invoke(new()
* {
* Name = "existing",
* ResourceGroupName = "existing",
* });
* var exampleGetVolumeGroup = Azure.ElasticSan.GetVolumeGroup.Invoke(new()
* {
* Name = "existing",
* ElasticSanId = example.Apply(getResult => getResult.Id),
* });
* return new Dictionary
* {
* ["id"] = exampleGetVolumeGroup.Apply(getVolumeGroupResult => getVolumeGroupResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/elasticsan"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := elasticsan.Get(ctx, &elasticsan.GetArgs{
* Name: "existing",
* ResourceGroupName: "existing",
* }, nil)
* if err != nil {
* return err
* }
* exampleGetVolumeGroup, err := elasticsan.LookupVolumeGroup(ctx, &elasticsan.LookupVolumeGroupArgs{
* Name: "existing",
* ElasticSanId: example.Id,
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("id", exampleGetVolumeGroup.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.elasticsan.ElasticsanFunctions;
* import com.pulumi.azure.elasticsan.inputs.GetArgs;
* import com.pulumi.azure.elasticsan.inputs.GetVolumeGroupArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ElasticsanFunctions.get(GetArgs.builder()
* .name("existing")
* .resourceGroupName("existing")
* .build());
* final var exampleGetVolumeGroup = ElasticsanFunctions.getVolumeGroup(GetVolumeGroupArgs.builder()
* .name("existing")
* .elasticSanId(example.applyValue(getResult -> getResult.id()))
* .build());
* ctx.export("id", exampleGetVolumeGroup.applyValue(getVolumeGroupResult -> getVolumeGroupResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:elasticsan:get
* Arguments:
* name: existing
* resourceGroupName: existing
* exampleGetVolumeGroup:
* fn::invoke:
* Function: azure:elasticsan:getVolumeGroup
* Arguments:
* name: existing
* elasticSanId: ${example.id}
* outputs:
* id: ${exampleGetVolumeGroup.id}
* ```
*
* @param argument A collection of arguments for invoking getVolumeGroup.
* @return A collection of values returned by getVolumeGroup.
*/
public suspend fun getVolumeGroup(argument: GetVolumeGroupPlainArgs): GetVolumeGroupResult =
getVolumeGroupResultToKotlin(getVolumeGroupPlain(argument.toJava()).await())
/**
* @see [getVolumeGroup].
* @param elasticSanId The Elastic SAN ID within which the Elastic SAN Volume Group exists.
* @param name The name of the Elastic SAN Volume Group.
* @return A collection of values returned by getVolumeGroup.
*/
public suspend fun getVolumeGroup(elasticSanId: String, name: String): GetVolumeGroupResult {
val argument = GetVolumeGroupPlainArgs(
elasticSanId = elasticSanId,
name = name,
)
return getVolumeGroupResultToKotlin(getVolumeGroupPlain(argument.toJava()).await())
}
/**
* @see [getVolumeGroup].
* @param argument Builder for [com.pulumi.azure.elasticsan.kotlin.inputs.GetVolumeGroupPlainArgs].
* @return A collection of values returned by getVolumeGroup.
*/
public suspend fun getVolumeGroup(argument: suspend GetVolumeGroupPlainArgsBuilder.() -> Unit): GetVolumeGroupResult {
val builder = GetVolumeGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getVolumeGroupResultToKotlin(getVolumeGroupPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Elastic SAN Volume Snapshot.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.elasticsan.get({
* name: "existing",
* resourceGroupName: "existing",
* });
* const exampleGetVolumeGroup = example.then(example => azure.elasticsan.getVolumeGroup({
* name: "existing",
* elasticSanId: example.id,
* }));
* const exampleGetVolumeSnapshot = exampleGetVolumeGroup.then(exampleGetVolumeGroup => azure.elasticsan.getVolumeSnapshot({
* name: "existing",
* volumeGroupId: exampleGetVolumeGroup.id,
* }));
* export const id = exampleGetVolumeSnapshot.then(exampleGetVolumeSnapshot => exampleGetVolumeSnapshot.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.elasticsan.get(name="existing",
* resource_group_name="existing")
* example_get_volume_group = azure.elasticsan.get_volume_group(name="existing",
* elastic_san_id=example.id)
* example_get_volume_snapshot = azure.elasticsan.get_volume_snapshot(name="existing",
* volume_group_id=example_get_volume_group.id)
* pulumi.export("id", example_get_volume_snapshot.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ElasticSan.Get.Invoke(new()
* {
* Name = "existing",
* ResourceGroupName = "existing",
* });
* var exampleGetVolumeGroup = Azure.ElasticSan.GetVolumeGroup.Invoke(new()
* {
* Name = "existing",
* ElasticSanId = example.Apply(getResult => getResult.Id),
* });
* var exampleGetVolumeSnapshot = Azure.ElasticSan.GetVolumeSnapshot.Invoke(new()
* {
* Name = "existing",
* VolumeGroupId = exampleGetVolumeGroup.Apply(getVolumeGroupResult => getVolumeGroupResult.Id),
* });
* return new Dictionary
* {
* ["id"] = exampleGetVolumeSnapshot.Apply(getVolumeSnapshotResult => getVolumeSnapshotResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/elasticsan"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := elasticsan.Get(ctx, &elasticsan.GetArgs{
* Name: "existing",
* ResourceGroupName: "existing",
* }, nil)
* if err != nil {
* return err
* }
* exampleGetVolumeGroup, err := elasticsan.LookupVolumeGroup(ctx, &elasticsan.LookupVolumeGroupArgs{
* Name: "existing",
* ElasticSanId: example.Id,
* }, nil)
* if err != nil {
* return err
* }
* exampleGetVolumeSnapshot, err := elasticsan.GetVolumeSnapshot(ctx, &elasticsan.GetVolumeSnapshotArgs{
* Name: "existing",
* VolumeGroupId: exampleGetVolumeGroup.Id,
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("id", exampleGetVolumeSnapshot.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.elasticsan.ElasticsanFunctions;
* import com.pulumi.azure.elasticsan.inputs.GetArgs;
* import com.pulumi.azure.elasticsan.inputs.GetVolumeGroupArgs;
* import com.pulumi.azure.elasticsan.inputs.GetVolumeSnapshotArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ElasticsanFunctions.get(GetArgs.builder()
* .name("existing")
* .resourceGroupName("existing")
* .build());
* final var exampleGetVolumeGroup = ElasticsanFunctions.getVolumeGroup(GetVolumeGroupArgs.builder()
* .name("existing")
* .elasticSanId(example.applyValue(getResult -> getResult.id()))
* .build());
* final var exampleGetVolumeSnapshot = ElasticsanFunctions.getVolumeSnapshot(GetVolumeSnapshotArgs.builder()
* .name("existing")
* .volumeGroupId(exampleGetVolumeGroup.applyValue(getVolumeGroupResult -> getVolumeGroupResult.id()))
* .build());
* ctx.export("id", exampleGetVolumeSnapshot.applyValue(getVolumeSnapshotResult -> getVolumeSnapshotResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:elasticsan:get
* Arguments:
* name: existing
* resourceGroupName: existing
* exampleGetVolumeGroup:
* fn::invoke:
* Function: azure:elasticsan:getVolumeGroup
* Arguments:
* name: existing
* elasticSanId: ${example.id}
* exampleGetVolumeSnapshot:
* fn::invoke:
* Function: azure:elasticsan:getVolumeSnapshot
* Arguments:
* name: existing
* volumeGroupId: ${exampleGetVolumeGroup.id}
* outputs:
* id: ${exampleGetVolumeSnapshot.id}
* ```
*
* @param argument A collection of arguments for invoking getVolumeSnapshot.
* @return A collection of values returned by getVolumeSnapshot.
*/
public suspend fun getVolumeSnapshot(argument: GetVolumeSnapshotPlainArgs): GetVolumeSnapshotResult =
getVolumeSnapshotResultToKotlin(getVolumeSnapshotPlain(argument.toJava()).await())
/**
* @see [getVolumeSnapshot].
* @param name The name of the Elastic SAN Volume Snapshot.
* @param volumeGroupId The Elastic SAN Volume Group ID within which the Elastic SAN Volume Snapshot exists.
* @return A collection of values returned by getVolumeSnapshot.
*/
public suspend fun getVolumeSnapshot(name: String, volumeGroupId: String): GetVolumeSnapshotResult {
val argument = GetVolumeSnapshotPlainArgs(
name = name,
volumeGroupId = volumeGroupId,
)
return getVolumeSnapshotResultToKotlin(getVolumeSnapshotPlain(argument.toJava()).await())
}
/**
* @see [getVolumeSnapshot].
* @param argument Builder for [com.pulumi.azure.elasticsan.kotlin.inputs.GetVolumeSnapshotPlainArgs].
* @return A collection of values returned by getVolumeSnapshot.
*/
public suspend fun getVolumeSnapshot(argument: suspend GetVolumeSnapshotPlainArgsBuilder.() -> Unit): GetVolumeSnapshotResult {
val builder = GetVolumeSnapshotPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getVolumeSnapshotResultToKotlin(getVolumeSnapshotPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy