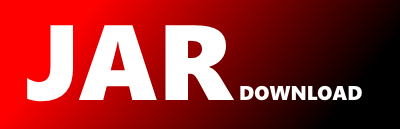
com.pulumi.azure.iot.kotlin.inputs.SecurityDeviceGroupRangeRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.iot.kotlin.inputs
import com.pulumi.azure.iot.inputs.SecurityDeviceGroupRangeRuleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property duration Specifies the time range. represented in ISO 8601 duration format.
* @property max The maximum threshold in the given time window.
* @property min The minimum threshold in the given time window.
* @property type The type of supported rule type. Possible Values are `ActiveConnectionsNotInAllowedRange`, `AmqpC2DMessagesNotInAllowedRange`, `MqttC2DMessagesNotInAllowedRange`, `HttpC2DMessagesNotInAllowedRange`, `AmqpC2DRejectedMessagesNotInAllowedRange`, `MqttC2DRejectedMessagesNotInAllowedRange`, `HttpC2DRejectedMessagesNotInAllowedRange`, `AmqpD2CMessagesNotInAllowedRange`, `MqttD2CMessagesNotInAllowedRange`, `HttpD2CMessagesNotInAllowedRange`, `DirectMethodInvokesNotInAllowedRange`, `FailedLocalLoginsNotInAllowedRange`, `FileUploadsNotInAllowedRange`, `QueuePurgesNotInAllowedRange`, `TwinUpdatesNotInAllowedRange` and `UnauthorizedOperationsNotInAllowedRange`.
*/
public data class SecurityDeviceGroupRangeRuleArgs(
public val duration: Output,
public val max: Output,
public val min: Output,
public val type: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.iot.inputs.SecurityDeviceGroupRangeRuleArgs =
com.pulumi.azure.iot.inputs.SecurityDeviceGroupRangeRuleArgs.builder()
.duration(duration.applyValue({ args0 -> args0 }))
.max(max.applyValue({ args0 -> args0 }))
.min(min.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecurityDeviceGroupRangeRuleArgs].
*/
@PulumiTagMarker
public class SecurityDeviceGroupRangeRuleArgsBuilder internal constructor() {
private var duration: Output? = null
private var max: Output? = null
private var min: Output? = null
private var type: Output? = null
/**
* @param value Specifies the time range. represented in ISO 8601 duration format.
*/
@JvmName("ekrwtvtibyhlxdro")
public suspend fun duration(`value`: Output) {
this.duration = value
}
/**
* @param value The maximum threshold in the given time window.
*/
@JvmName("lysibbfairkavfdu")
public suspend fun max(`value`: Output) {
this.max = value
}
/**
* @param value The minimum threshold in the given time window.
*/
@JvmName("hdnguxjrichkjkbc")
public suspend fun min(`value`: Output) {
this.min = value
}
/**
* @param value The type of supported rule type. Possible Values are `ActiveConnectionsNotInAllowedRange`, `AmqpC2DMessagesNotInAllowedRange`, `MqttC2DMessagesNotInAllowedRange`, `HttpC2DMessagesNotInAllowedRange`, `AmqpC2DRejectedMessagesNotInAllowedRange`, `MqttC2DRejectedMessagesNotInAllowedRange`, `HttpC2DRejectedMessagesNotInAllowedRange`, `AmqpD2CMessagesNotInAllowedRange`, `MqttD2CMessagesNotInAllowedRange`, `HttpD2CMessagesNotInAllowedRange`, `DirectMethodInvokesNotInAllowedRange`, `FailedLocalLoginsNotInAllowedRange`, `FileUploadsNotInAllowedRange`, `QueuePurgesNotInAllowedRange`, `TwinUpdatesNotInAllowedRange` and `UnauthorizedOperationsNotInAllowedRange`.
*/
@JvmName("kvmknyakhrylunwj")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Specifies the time range. represented in ISO 8601 duration format.
*/
@JvmName("swrqywoujutwwfxu")
public suspend fun duration(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.duration = mapped
}
/**
* @param value The maximum threshold in the given time window.
*/
@JvmName("vpwduvyfdqabvwol")
public suspend fun max(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.max = mapped
}
/**
* @param value The minimum threshold in the given time window.
*/
@JvmName("rcokjilefeamohgo")
public suspend fun min(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.min = mapped
}
/**
* @param value The type of supported rule type. Possible Values are `ActiveConnectionsNotInAllowedRange`, `AmqpC2DMessagesNotInAllowedRange`, `MqttC2DMessagesNotInAllowedRange`, `HttpC2DMessagesNotInAllowedRange`, `AmqpC2DRejectedMessagesNotInAllowedRange`, `MqttC2DRejectedMessagesNotInAllowedRange`, `HttpC2DRejectedMessagesNotInAllowedRange`, `AmqpD2CMessagesNotInAllowedRange`, `MqttD2CMessagesNotInAllowedRange`, `HttpD2CMessagesNotInAllowedRange`, `DirectMethodInvokesNotInAllowedRange`, `FailedLocalLoginsNotInAllowedRange`, `FileUploadsNotInAllowedRange`, `QueuePurgesNotInAllowedRange`, `TwinUpdatesNotInAllowedRange` and `UnauthorizedOperationsNotInAllowedRange`.
*/
@JvmName("lqpqqjgpsjotifjn")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): SecurityDeviceGroupRangeRuleArgs = SecurityDeviceGroupRangeRuleArgs(
duration = duration ?: throw PulumiNullFieldException("duration"),
max = max ?: throw PulumiNullFieldException("max"),
min = min ?: throw PulumiNullFieldException("min"),
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy